The "Left String" concept in PowerShell allows you to extract a specified number of characters from the beginning of a string using the `Substring` method or the `-replace` operator.
Here’s a code snippet demonstrating how to use `Substring` to get the left part of a string:
$originalString = "Hello, PowerShell!"
$leftString = $originalString.Substring(0, 5) # Gets the first 5 characters
Write-Host $leftString # Outputs: Hello
Understanding Strings in PowerShell
What is a String in PowerShell?
A string in PowerShell is a sequence of characters enclosed in double quotes. It can contain letters, numbers, punctuation, and spaces. Strings are fundamental in programming, as they allow you to store, manipulate, and display text data. For example:
$exampleString = "This is a string in PowerShell."
The Importance of Manipulating Strings
String manipulation is an essential skill in scripting and automation. Many use cases hinge on the ability to extract, replace, or format strings for various tasks. For instance, when processing user input, generating reports, or performing data transformations, effective string manipulation saves time and reduces errors.
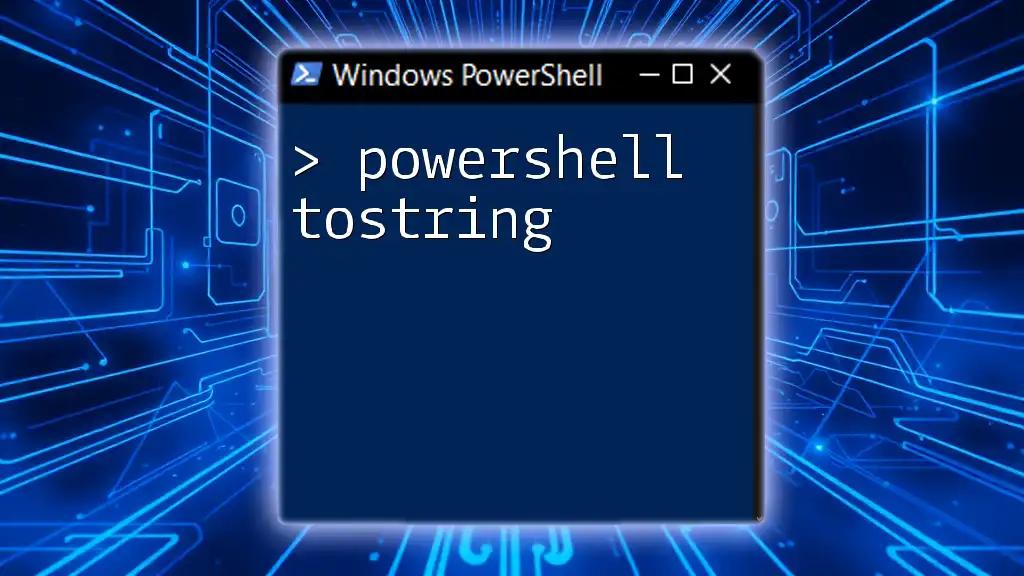
PowerShell Left String: The Basics
What Does "Left String" Mean?
In programming, a left string refers to taking the first few characters from a string, starting from the leftmost character. This is especially useful when you need to extract a specific portion of a string for further processing or analysis.
PowerShell String Functions
PowerShell provides several built-in string manipulation functions that streamline the process of string handling. The most commonly used functions include `Substring()` for extracting parts of strings, as well as others like `-replace` for modifying string content.
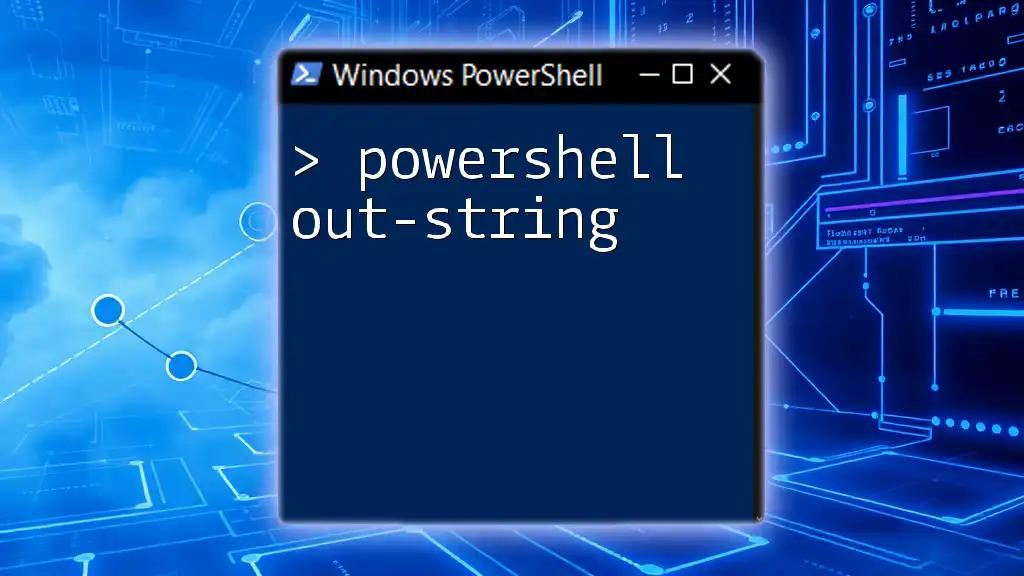
The PowerShell Left Substring Command
Introduction to `Substring()`
The `Substring()` method is a powerful tool for string manipulation in PowerShell. It allows you to extract a portion of a string by specifying the starting index and the length of the substring you want. Here’s the basic syntax:
string.Substring(startIndex, length)
How to Use `Substring()` to Get the Left Part of a String
Basic Example of Left Substring
To extract the left portion of a string, you can start with the following example:
$originalString = "Hello, World!"
$leftSubstring = $originalString.Substring(0, 5) # "Hello"
In this example, `Substring(0, 5)` means you start at index 0 and take the next 5 characters, resulting in the output "Hello". Understanding indices is crucial, as PowerShell is zero-based, meaning the first character has an index of 0.
Extracting Different Lengths
You can easily modify the length to get substrings of varying sizes. For example, if you want only the first three characters, you could write:
$shorterSubstring = $originalString.Substring(0, 3) # "Hel"
In this case, you specify a length of 3, which will give you the substring "Hel". Such functionality can be handy when dealing with input validation or parsing.

Handling Edge Cases
What if the Start Index is Out of Range?
Using `Substring()` with an invalid start index can lead to runtime errors. It is crucial to ensure that your specified start index is within the bounds of the string’s length. For example:
$invalidSubstring = $originalString.Substring(15, 2) # This will throw an error
To handle potential errors effectively, consider a style that checks string length before attempting substring operations.
Dealing with Empty Strings
When working with strings, you might encounter empty strings. To safely use `Substring()` with an empty string, you can implement a simple check:
$emptyString = ""
$leftSubstring = if ($emptyString.Length -gt 0) { $emptyString.Substring(0, 1) } else { "No content" }
In this code, if `$emptyString` has a length greater than zero, the code extracts the first character; otherwise, it returns "No content". This practice helps to avoid runtime exceptions.
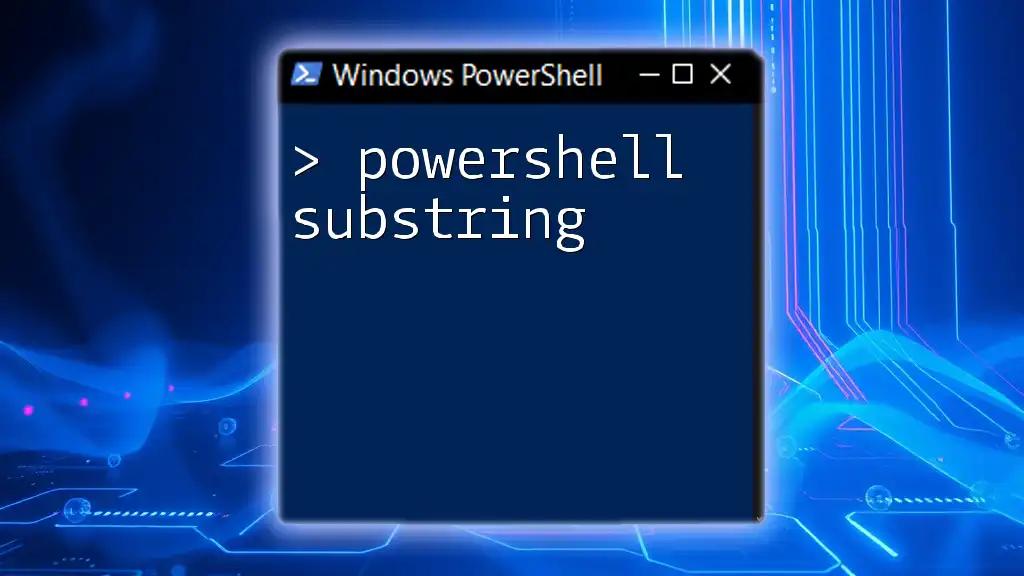
Other Useful String Manipulation Techniques
Using `-replace` Operator for Left Substring
You can creatively use the `-replace` operator to achieve similar results as `Substring()`. For instance:
$leftPart = $originalString -replace " World!", ""
In this case, you're effectively taking out the substring " World!" from the original string, retaining "Hello". This method can be particularly useful when you want to clean up or format output without directly referencing indices.
Combining `Substring()` with Other PowerShell Cmdlets
You can extend the functionality of `Substring()` by combining it with other PowerShell cmdlets like `ForEach-Object`. For example:
$names = "Alice", "Bob", "Charlie"
$names | ForEach-Object { $_.Substring(0, 1) }
This will output the first character of each name, resulting in A, B, and C. Such combinations illustrate the versatility of `Substring()` within various operations.
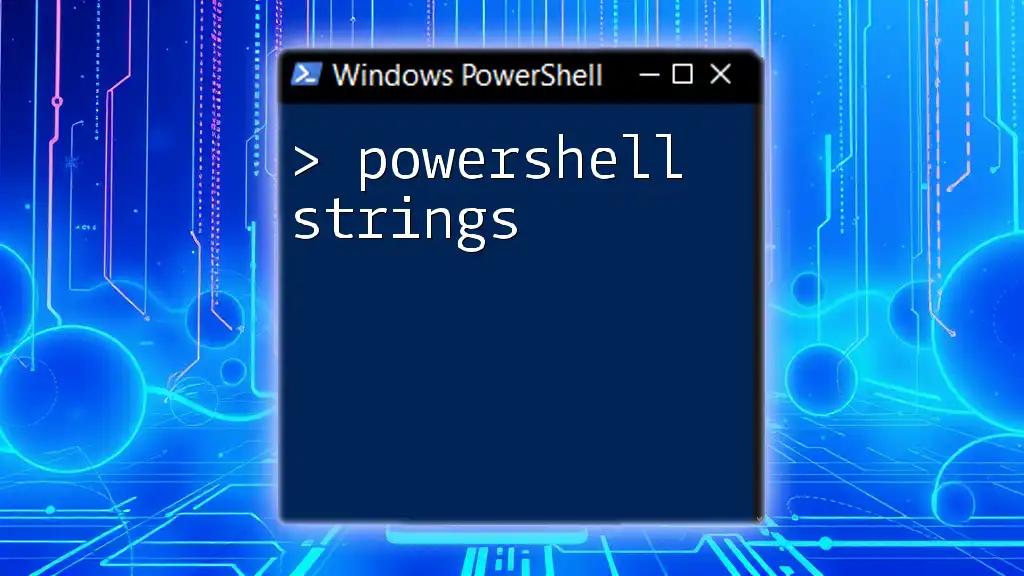
PowerShell Left String in Real-world Applications
Scripting for Data Generation
Using PowerShell to generate random strings often involves utilizing left substrings. When creating user IDs, you might want to take a few characters from a longer generated string for uniqueness.
Text Processing
In situations like parsing logs or processing configuration files, you may find the need to extract left substrings for further analysis. For example, extracting filename prefixes might help categorize files effectively.
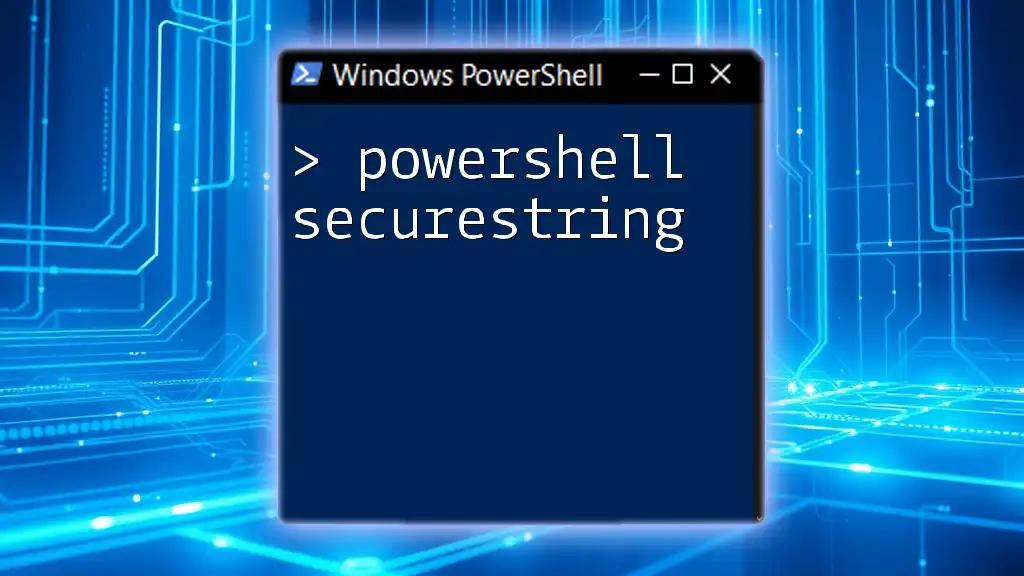
Best Practices
Recommendations for Using `Substring()`
When employing `Substring()`, it’s important to:
- Always validate the start index and length before applying the method to avoid errors.
- Leverage error handling to manage exceptions gracefully.
- Incorporate clear variable naming to enhance code readability and maintainability.

Conclusion
Grasping the concept of PowerShell left strings and mastering the `Substring()` method can significantly enhance your scripting capabilities. This skill set will empower you to handle strings with confidence, making your scripts more efficient and easier to manage.
Moreover, practice is key! Explore real-world applications, experiment with different string operations, and join our community for structured learning opportunities in PowerShell.

Additional Resources
Further Reading
For an in-depth understanding, you can consult the [official PowerShell documentation](https://docs.microsoft.com/powershell/scripting) where you’ll find a wealth of information on string manipulation and other related topics.
Join Our Community
Stay connected and share your learning journey with fellow PowerShell enthusiasts. Join our training programs to break through your learning barriers and master PowerShell scripting!

FAQs
Common Questions About PowerShell Left String Commands
If you have questions about using left strings in PowerShell, don't hesitate to explore community forums and tutorials that provide insights and solutions to common queries.