To remove the first character from a string in PowerShell, you can use the `Substring` method to extract the remaining part of the string starting from the second character.
Here’s the code snippet to achieve this:
$string = "Hello, World!"
$result = $string.Substring(1)
Write-Host $result # Outputs: "ello, World!"
Understanding Strings in PowerShell
What is a String?
In PowerShell, a string is a sequence of characters, which includes letters, numbers, symbols, and even whitespace. Strings are integral to scripting as they hold data and can be manipulated to achieve desired outcomes.
For example, consider the string:
$greeting = "Hello, World!"
This string is composed of characters that can be used, modified, and formatted for various purposes in scripts.
String Indexing
PowerShell strings use a zero-based indexing system, meaning that the first character of a string is accessed using the index `0`. This indexing enables you to retrieve or manipulate specific characters within the string easily. For instance:
$example = "PowerShell"
$firstCharacter = $example[0] # Retrieves 'P'
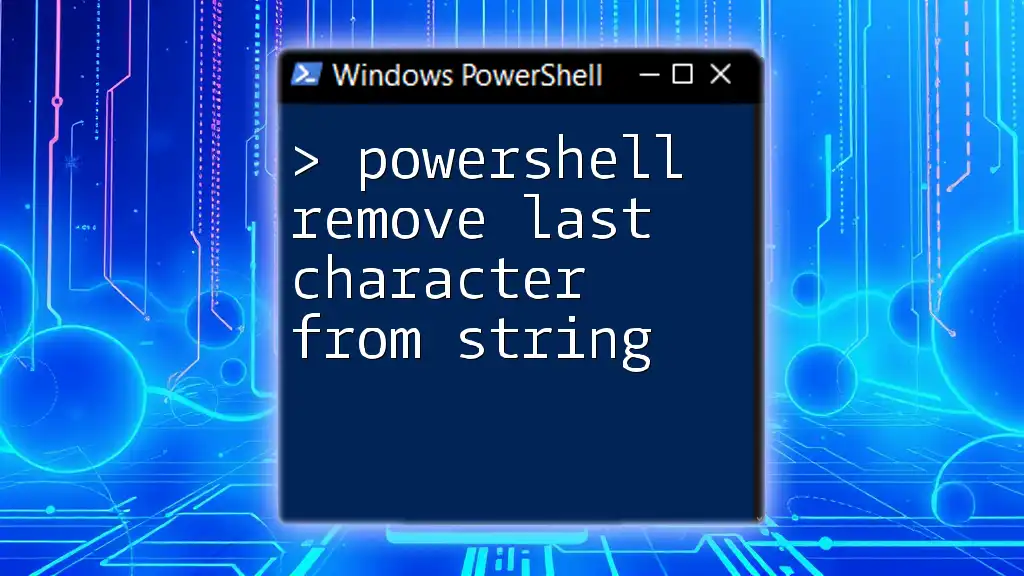
Removing the First Character from a String
Simple Method to Remove the First Character
One of the most straightforward ways to remove the first character from a string is by using the `Substring()` method. This method extracts a portion of a string starting from a specified index.
For example, consider the following PowerShell code:
$originalString = "Hello, World!"
$modifiedString = $originalString.Substring(1)
Write-Output $modifiedString # Outputs "ello, World!"
In this code, `Substring(1)` is invoked, which tells PowerShell to start from the character at index `1` and return the rest of the string. The result is the original string with the first character removed.
Using PowerShell String Array Method
Another approach involves using the `-replace` operator, which facilitates a regex-based string manipulation technique. Here’s how you can do it:
$originalString = "PowerShell"
$modifiedString = $originalString -replace "^.", ""
Write-Output $modifiedString # Outputs "owerShell"
In this case, the regex pattern `^.` matches the first character of the string, and `-replace` effectively removes it, resulting in the desired output.
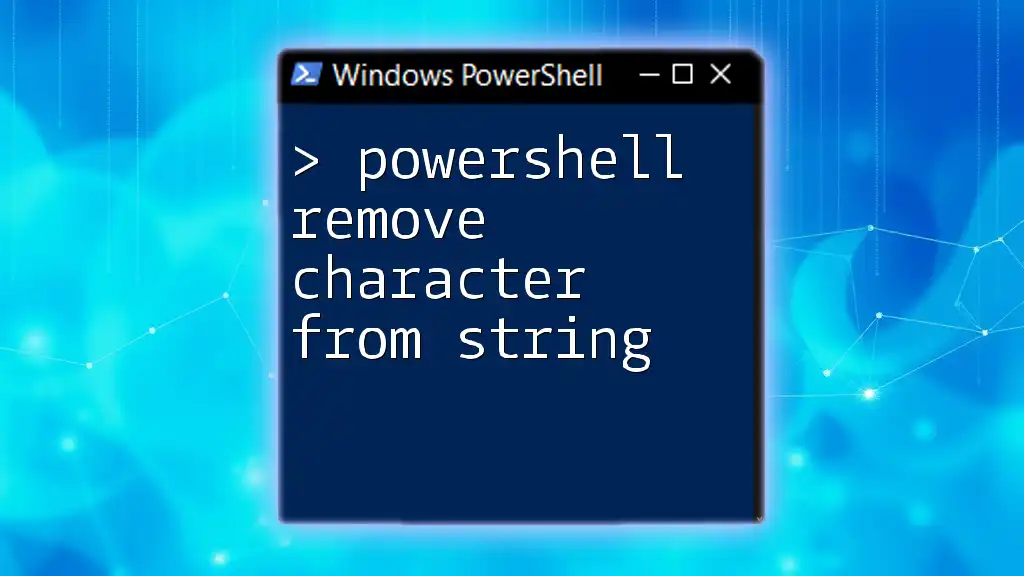
Trimming the First Four Characters from a String
Why Trim Multiple Characters?
At times, you may need to remove more than just the first character from a string, such as leading identifiers or prefixes. Trimming multiple characters demands thoughtful use of the methods available in PowerShell.
Using Substring to Trim First Four Characters
To remove the first four characters from a string, you can seamlessly adjust the `Substring()` method:
$originalString = "PowerShell Academy"
$modifiedString = $originalString.Substring(4)
Write-Output $modifiedString # Outputs "Shell Academy"
Here, invoking `Substring(4)` starts from the fifth character of the string, eliminating the first four characters entirely.
Trimming with Regular Expressions
You can also use regex to trim multiple characters effectively. Reference the code below:
$originalString = "1234HelloWorld"
$modifiedString = $originalString -replace "^\d{4}", ""
Write-Output $modifiedString # Outputs "HelloWorld"
The regex pattern `^\d{4}` identifies the first four numeric characters at the start of the string, and by using `-replace`, we can drop them and return the remaining string.
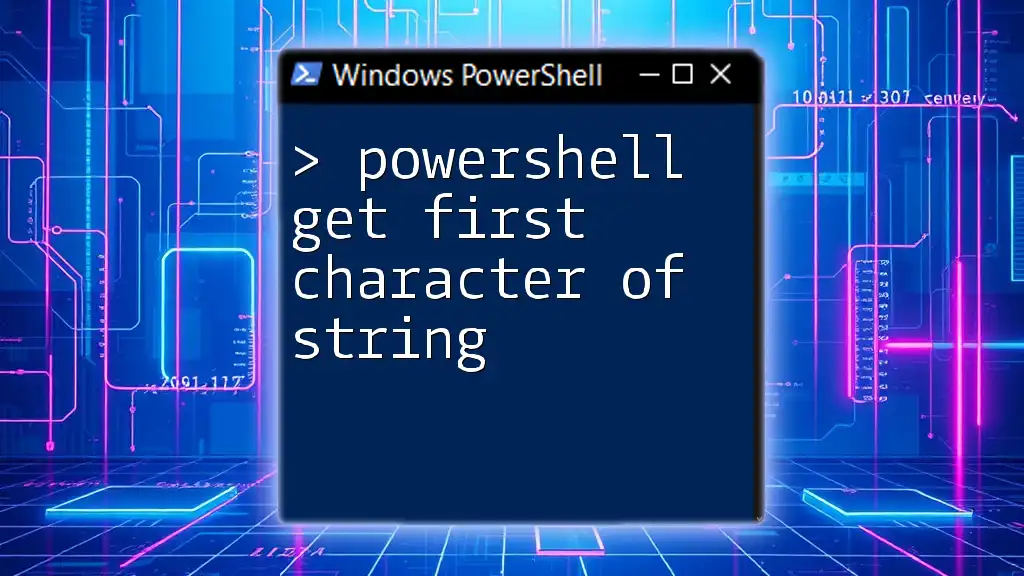
Handling Edge Cases in String Manipulation
What Happens if the String is Too Short?
An important aspect to consider while manipulating strings is their length. If the string is shorter than expected, handling it gracefully prevents errors. For example:
$originalString = "Hi"
if ($originalString.Length -gt 1) {
$modifiedString = $originalString.Substring(1)
} else {
$modifiedString = $originalString
}
Write-Output $modifiedString # Outputs "Hi"
In this code, a conditional statement checks the string length. If it’s more than one character, it proceeds to remove the first character; otherwise, it retains the original string.
Trimming Special Characters
When strings contain special characters or non-alphanumeric symbols, you may need to consider those in your trimming logic. For instance:
$originalString = "!@#HelloWorld"
$modifiedString = $originalString -replace "^[^a-zA-Z0-9]{3}", ""
Write-Output $modifiedString # Outputs "HelloWorld"
In the above code, the regex pattern `^[^a-zA-Z0-9]{3}` identifies the first three non-alphanumeric characters and removes them, efficiently resulting in a clean string.
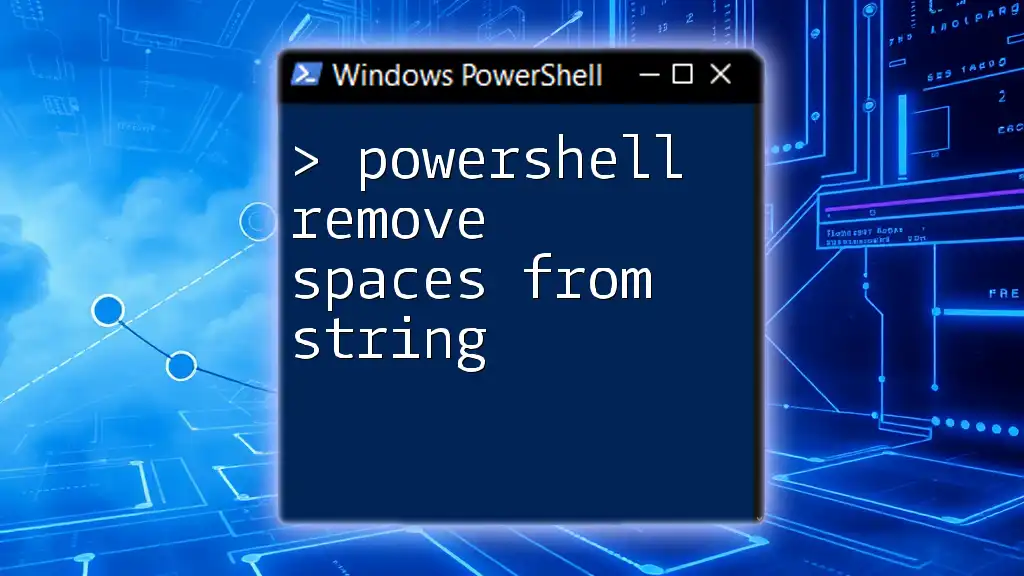
Conclusion
To successfully remove the first character from a string in PowerShell, you can utilize various methods like `Substring()`, `-replace`, or regex. Each technique serves its purpose depending on the situation at hand, including trimming multiple characters. Understanding these methods opens up a realm of possibilities for efficient string manipulation in your scripts. Practice these commands to gain confidence and improve your PowerShell skills.
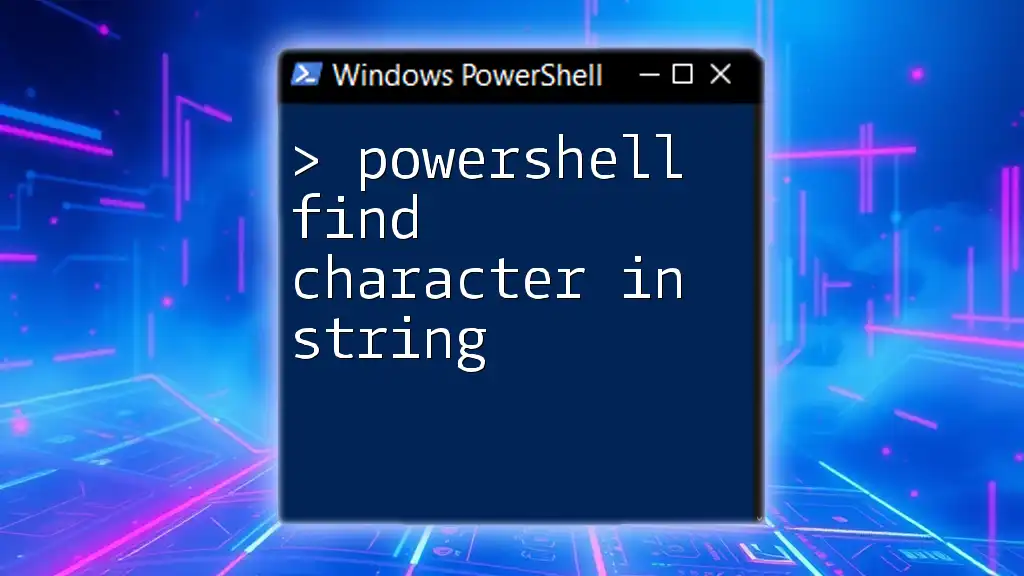
FAQs on PowerShell String Manipulation
What if my string has leading spaces?
You can handle leading spaces by employing the `.TrimStart()` method. This method allows you to remove unwanted whitespace characters from the beginning of your string, making your scripts cleaner.
Can I remove characters from the end of a string?
Yes! For trimming from the end, you can also use the `Substring()` method, adjusted to maintain the desired length of the string.
Is string manipulation different in PowerShell version 7?
In general, string manipulation remains consistent across PowerShell versions, but it's always good to check the latest documentation for any updates or new features that might optimize your scripting experience.
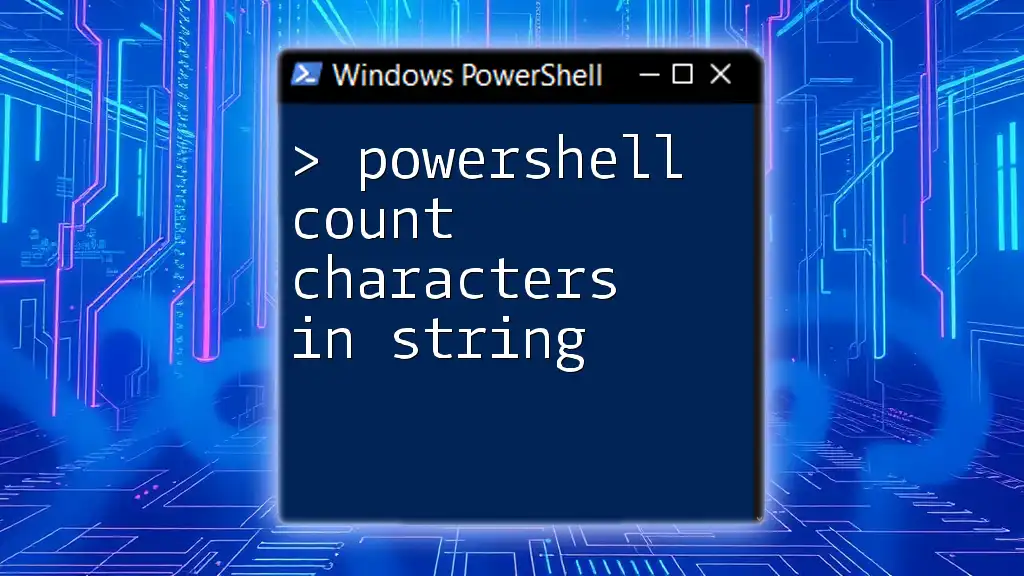
Call to Action
Feel free to share your questions or experiences with string manipulation in PowerShell in the comments below! Don't forget to share this article with anyone eager to learn more about using PowerShell effectively.