To add quotes to a string in PowerShell, you can use the following method to include quotes by escaping them with a backtick (`` ` ``):
$quotedString = "`"This is a quoted string`""
Write-Host $quotedString
Understanding Strings in PowerShell
What is a String?
A string is a sequence of characters used to represent text in programming. In PowerShell, strings are a fundamental data type, enabling users to handle text data efficiently. Understanding how to manipulate strings is crucial for any scripting or automation task.
Types of Strings in PowerShell
PowerShell supports two primary types of strings:
-
Single-quoted strings: These are used for literal text, meaning the text is treated exactly as it is written, without any variable expansion or special character interpretation. For example:
$string1 = 'This is a single-quoted string.'
-
Double-quoted strings: In contrast, double-quoted strings allow for variable expansion and interpret special characters (like newline `\n`). For instance:
$name = "World" $string2 = "Hello, $name!"
This distinction is crucial when working with strings in PowerShell, especially when you need to add quotes to strings.
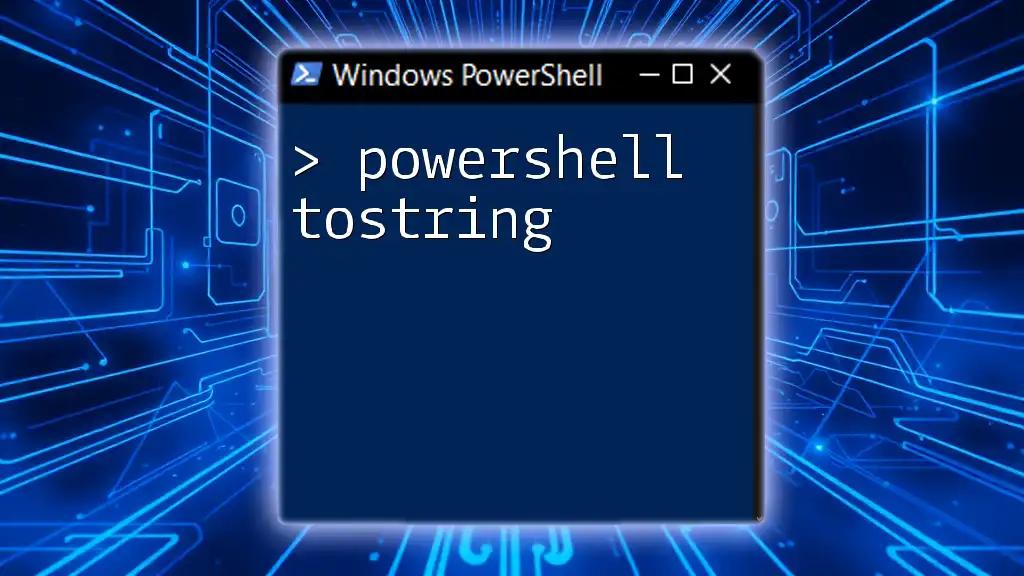
Quoting Mechanisms in PowerShell
Using Single Quotes
Single quotes are ideal when you want the string to remain unchanged. If you want to add quotes within a single-quoted string, you simply escape the internal quotes with a backslash:
$quotedString = 'He said, "Hello!"'
Using Double Quotes
Double quotes are more versatile, allowing for variable expansion. You can insert variables or special characters directly into the string. This is useful when you need dynamic content:
$quotedString = "It's a sunny day!"
Escaping Quotes Within Strings
When dealing with quotes, understanding how to escape them is important. Escaping ensures that PowerShell understands you want to include a quote character in your string rather than terminating the string. Here’s how you do it:
For single quotes:
$escapedString = 'It\'s a beautiful day.'
For double quotes:
$escapedString = "She said, \"Hello!\""
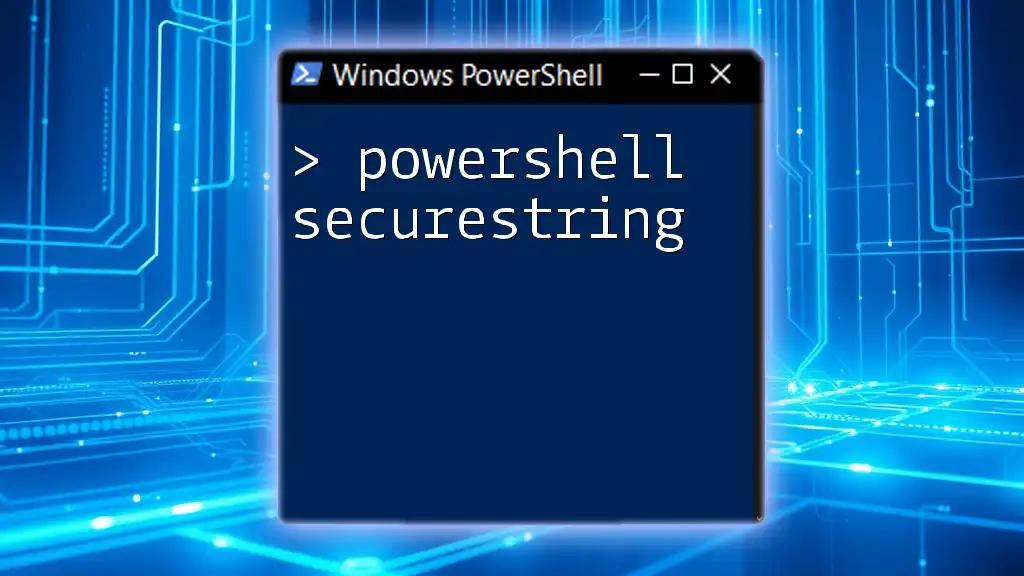
Adding Quotes to Existing Strings
Concatenation
One of the most straightforward ways to add quotes to strings in PowerShell is through concatenation. You can easily append and prepend quotes to your strings. The syntax for this is simple:
$originalString = "Hello World"
$quotedString = '"' + $originalString + '"'
This effectively encloses `Hello World` in quotes.
Using the `-replace` Operator
Another method to add quotes to an existing string is by using the `-replace` operator. This allows you to manipulate strings using regular expressions, which can be a powerful tool in string formatting:
$str = "PowerShell is powerful."
$quotedStr = $str -replace '^(.*)$', '"$1"'
In this example, `-replace` surrounds the string with quotes by capturing everything in it.
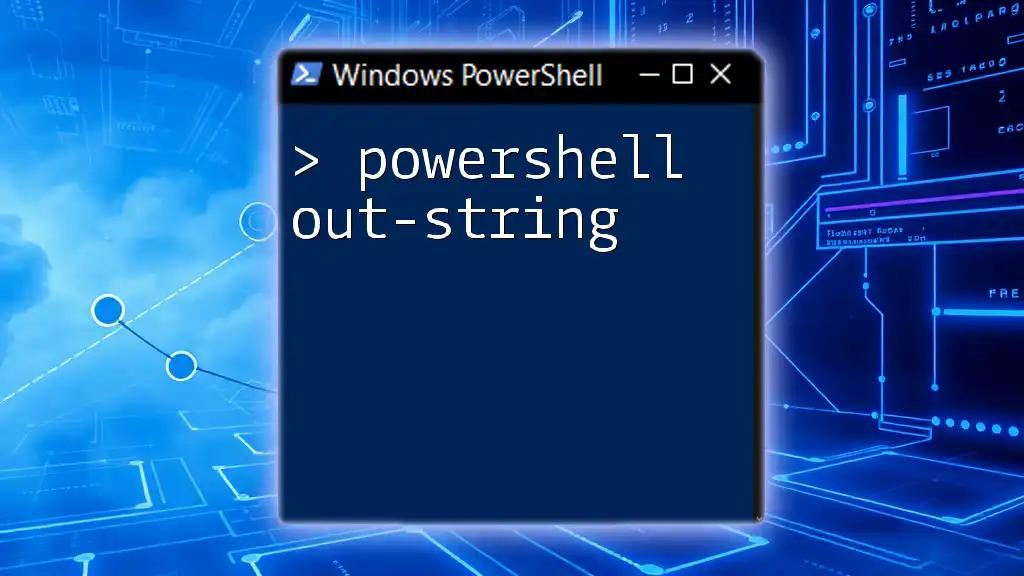
Function to Add Quotes
Creating a Function
Creating a function to add quotes to strings can significantly simplify your workflow in PowerShell. Here’s how to define such a function:
Function Add-Quotes {
param (
[string]$inputString
)
return '"' + $inputString + '"'
}
This reusable function takes an input string and returns it enclosed in quotes, making it a handy tool for various applications.
Example Usage of the Function
To use the `Add-Quotes` function, simply call it with your desired string:
$result = Add-Quotes "This will be quoted"
The output will be:
"This will be quoted"

Common Use Cases for Adding Quotes
Formatting Output
Adding quotes can help improve readability in scripts, particularly when outputting data to the console. For example, when displaying results in a table format, using quotes can clearly delineate each entry:
Write-Host "Result: '$quotedString'"
Handling CSV Files
When working with CSV files in PowerShell, quotes are essential for enclosing values, especially if those values contain commas. For example, when creating a CSV string from a list of values, you can format each entry with quotes:
$data = @("Name1,Location1", "Name2,Location2")
$csvString = $data -replace '(\w+)', '"$1"'
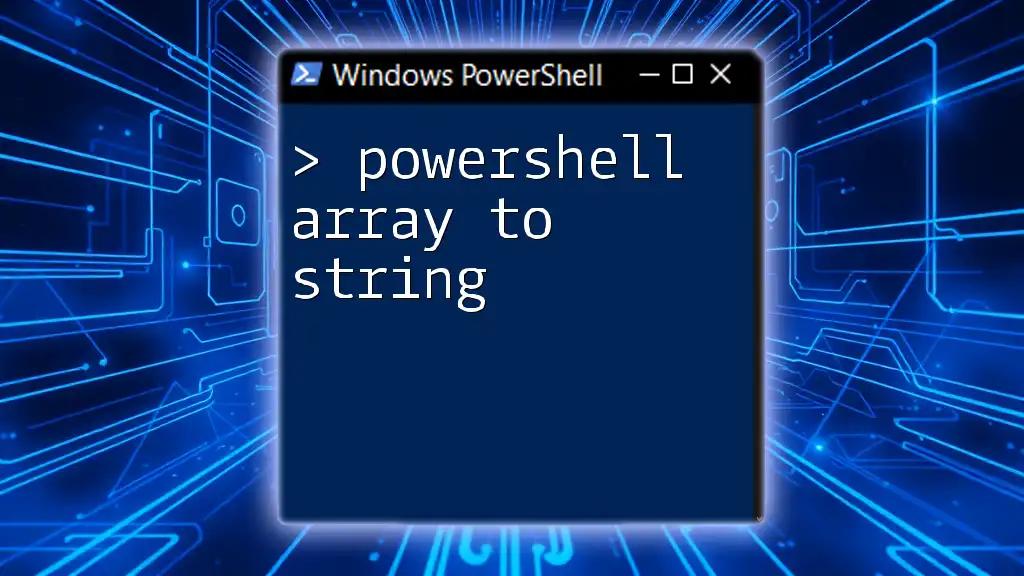
Best Practices
Consistency in Quote Usage
When writing scripts, it's vital to maintain consistency in your quote usage. Decide whether to use single or double quotes based on your needs and stick to that decision throughout your code. This improves readability and reduces errors.
Performance Considerations
Keep in mind that excessive string manipulation, particularly in large loops or extensive scripts, can affect performance. While adding quotes is straightforward, use efficient methods and combine operations to reduce performance overhead.

Conclusion
Manipulating strings to add quotes is a vital skill for anyone using PowerShell. By understanding the different types of strings, quoting mechanisms, and techniques for adding quotes, you can enhance your scripting capabilities. These skills will not only improve your automation scripts but also allow for better data handling and output formatting.
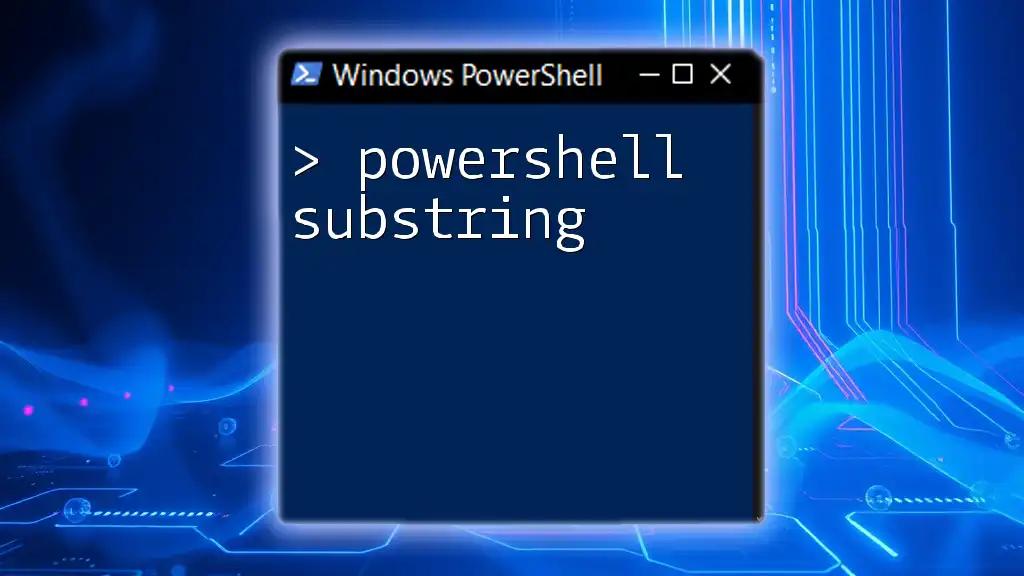
Call to Action
Now that you have a comprehensive understanding of how to PowerShell add quotes to string, it's time to put your knowledge into practice! Experiment with the examples provided and follow us for more tips and tricks on mastering PowerShell.