In PowerShell, you can convert an integer to a string by using the `ToString()` method or by simply concatenating it with an empty string.
# Using ToString() method
$integer = 123
$string = $integer.ToString()
# Concatenating with an empty string
$string2 = $integer + ''
Understanding Data Types in PowerShell
What is an Integer?
An integer is a whole number that can be positive, negative, or zero, and does not have a fractional part. In programming, integers are often used for counting, indexing, and performing mathematical calculations. They are one of the fundamental data types in PowerShell.
For example, an integer in PowerShell can be defined as follows:
$integer = 42
Here, `$integer` holds the value `42`, which is clearly a whole number.
What is a String?
A string, on the other hand, is a sequence of characters that can include letters, numbers, and symbols. Strings are often used to represent text or any information that is not purely numerical. In PowerShell, strings are enclosed in either single or double quotes.
A simple string example is:
$string = "Hello, World!"
In this case, `$string` contains the text `"Hello, World!"`.
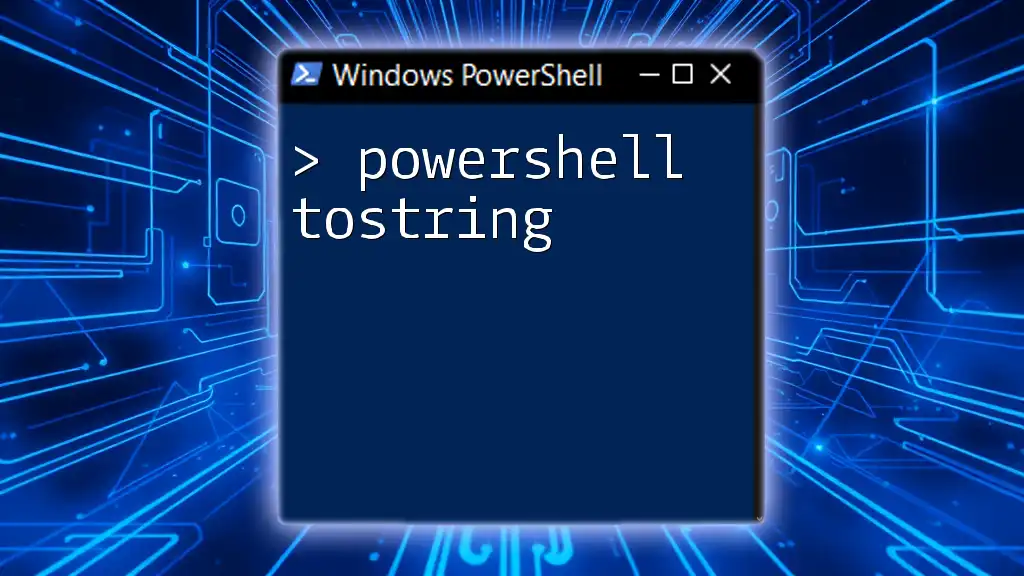
Converting Integer to String in PowerShell
Why Convert Integer to String?
Understanding how to convert an integer to a string in PowerShell is crucial because there are various scenarios in which this conversion is necessary. For instance, when you want to display messages to users, log data for processing, or concatenate integers with other strings for better readability, you must convert integers into strings.
Methods for Conversion
Method 1: Using .ToString() Method
One of the simplest methods to convert an integer to a string is by using the `.ToString()` method. This approach is direct and easy to understand.
Here's how you can use the `.ToString()` method:
$integer = 100
$string = $integer.ToString()
Write-Output $string # Output: "100"
In this example, we first define a variable `$integer` with the value of `100`. By invoking the `ToString()` method on `$integer`, we convert it into a string, which is then stored in `$string`.
Method 2: String Interpolation
Another straightforward approach is to leverage string interpolation in PowerShell. This method allows seamless inclusion of variables within strings.
Here is how it works:
$integer = 200
$string = "The value is $integer"
Write-Output $string # Output: "The value is 200"
In the example above, the variable `$integer` is directly included in the string using `$integer`. PowerShell automatically handles the conversion, resulting in the final string being `"The value is 200"`.
Method 3: Using String Format
PowerShell also supports string formatting, which is useful when you need more control over how strings are built. Utilizing the `-f` operator allows for formatted strings.
For instance:
$integer = 300
$string = "{0}" -f $integer
Write-Output $string # Output: "300"
In this code snippet, the string format operator `-f` is used to define how the integer should be converted into its string representation. Here, `{0}` serves as a placeholder for the value of `$integer`.
Method 4: Using String Concatenation
String concatenation is another common way to combine strings and integers. This method involves adding strings together, where the integer is automatically converted to a string in the process.
Here's an example of string concatenation:
$integer = 400
$string = "Value: " + $integer
Write-Output $string # Output: "Value: 400"
In this case, the string `"Value: "` is combined with the `$integer` variable using the `+` operator, leading to the output `"Value: 400"`.
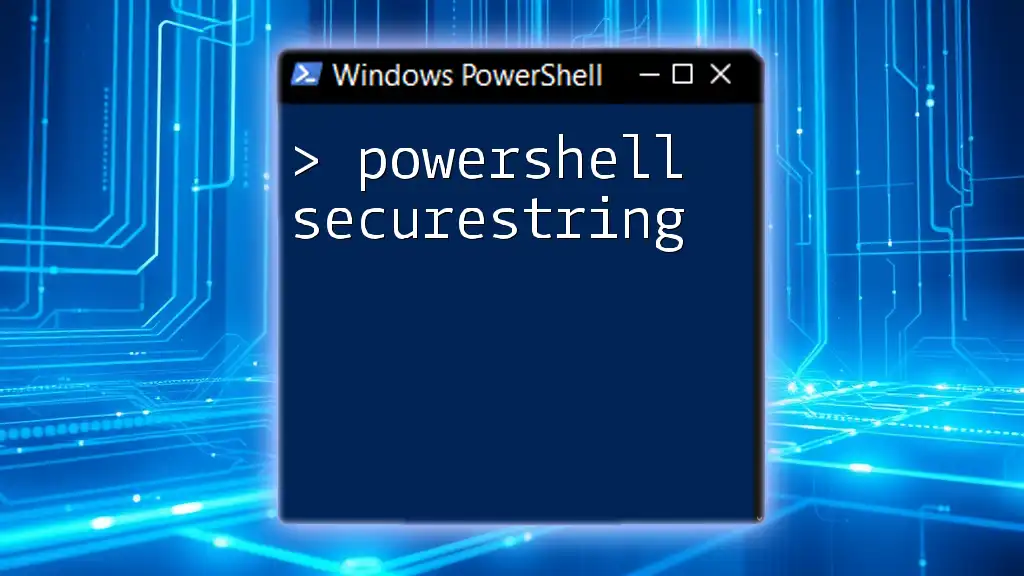
Best Practices for Conversion
When to Use Which Method?
Choosing the right method for converting integers to strings often depends on the context. The `.ToString()` method is most straightforward for simple conversions. For creating more context-driven messages, string interpolation is both readable and efficient. If formatting is necessary, consider using the string format method. In cases where combining multiple data types, concatenation can be used effectively.
Error Handling During Conversion
It’s important to anticipate possible errors during conversion, especially if the source variable may not consistently hold an integer. Common pitfalls include attempting to call `.ToString()` on null or invalid data types.
You can implement error handling to catch and manage such issues gracefully:
try {
$integer = "NotAnInteger"
$string = $integer.ToString()
}
catch {
Write-Host "Conversion failed: $_"
}
In this example, a `try-catch` block is used to handle exceptions. If a conversion error occurs, the catch block will produce a message detailing what went wrong, which aids in troubleshooting.
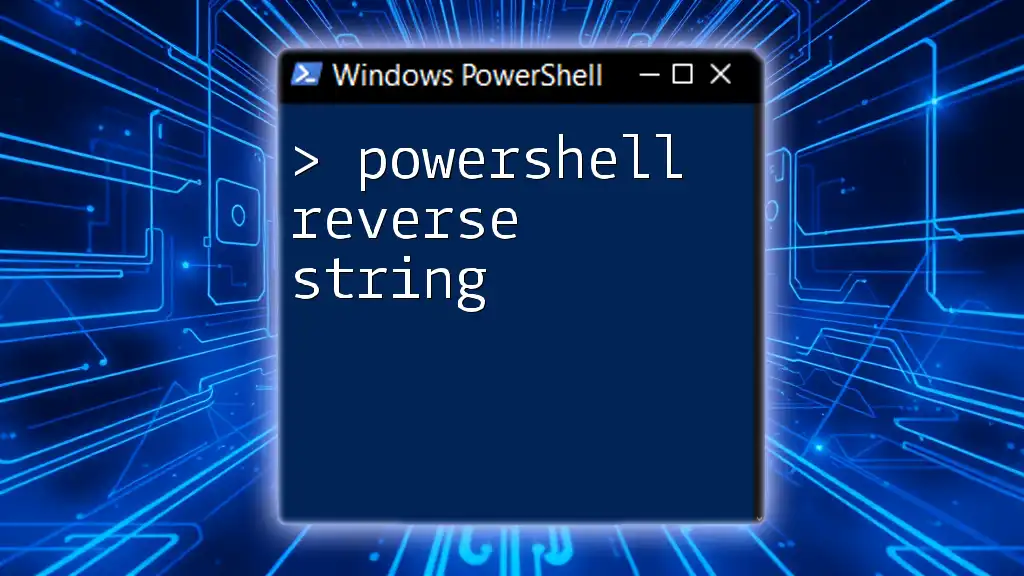
Conclusion
In conclusion, converting an integer to a string in PowerShell is a fundamental skill that facilitates effective data handling and presentation. Armed with methods such as `.ToString()`, string interpolation, string formatting, and concatenation, you can easily manage your data types. Practicing these techniques will enhance your PowerShell skills and empower you to handle various tasks efficiently.
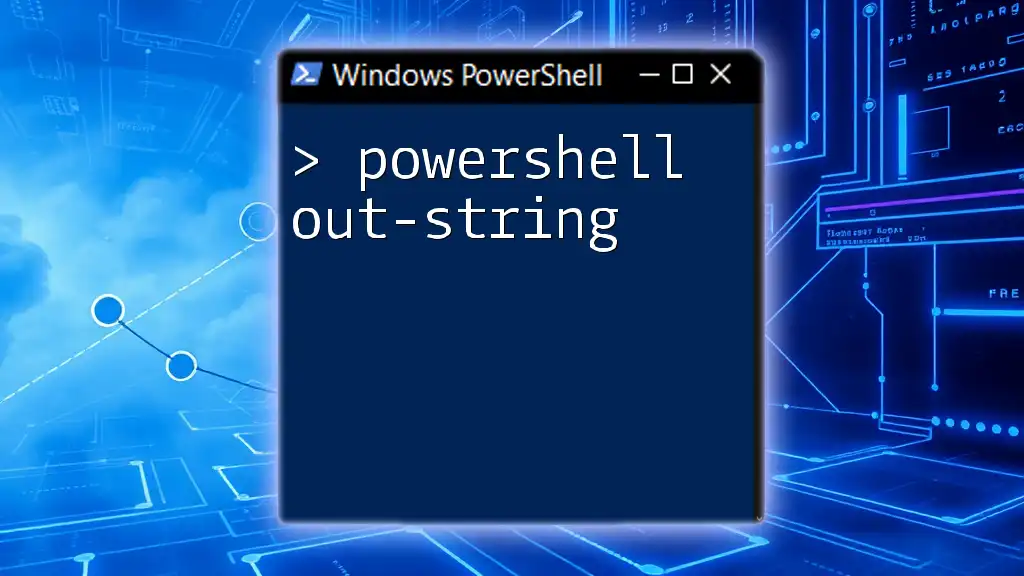
Additional Resources
For further exploration of conversion practices and other PowerShell commands, consider referring to the official documentation and engaging with community forums that focus on PowerShell. These resources can provide additional insights and support as you continue your PowerShell journey.