In PowerShell, you can remove specific characters or substrings from a string using the `.Replace()` method or the `-replace` operator.
Here's a code snippet illustrating the use of the `-replace` operator to remove occurrences of the substring "World" from the string "Hello, World!":
$originalString = 'Hello, World!'
$modifiedString = $originalString -replace 'World', ''
Write-Host $modifiedString # Output: Hello, !
Understanding Strings in PowerShell
What is a String in PowerShell?
In PowerShell, strings are sequences of characters used primarily to represent text. They are fundamental data types that allow users to manipulate text easily within scripts. Understanding how strings work is crucial for effective scripting, as strings often serve as inputs and outputs of functions and commands.
Basic String Operations
PowerShell supports various operations on strings, including concatenation, comparison, and manipulation. With methods available in .NET, performing operations like checking the length of a string, converting strings to uppercase or lowercase, and replacing characters is simple and efficient.

PowerShell Remove from String
Why Remove Text from Strings?
There are numerous scenarios where removing text from strings is essential. For instance, cleaning user input, formatting output, or preparing data for further processing can all require string manipulation. The ability to customize strings improves the readability and usefulness of any output generated by your scripts.
PowerShell String Remove: Built-In Methods
Using the `-replace` Operator
The `-replace` operator is a powerful feature in PowerShell, allowing you to replace text or patterns within a string. This operator uses regular expressions, making it a versatile tool for string manipulation.
Example:
$originalString = "Hello, World!"
$modifiedString = $originalString -replace "World", "PowerShell"
Write-Output $modifiedString # Output: Hello, PowerShell!
In this example, "World" is replaced with "PowerShell". Using regular expressions, you can manipulate strings with various complexities, such as removing or altering multiple substrings at once.
Using the `Substring()` Method
The `Substring()` method is ideal for removing specific parts of strings by extracting a subset of characters based on starting index and length.
Example:
$originalString = "Remove this part"
$modifiedString = $originalString.Substring(0, 6) # Keeps only "Remove"
Write-Output $modifiedString # Output: Remove
Here, the substring "Remove" is obtained from the original string. The starting index is 0, and the length is 6 characters long.
Using the `Trim()`, `TrimStart()`, and `TrimEnd()` Methods
These methods are handy for removing unwanted whitespace or specific characters from the beginning or the end of strings.
Example:
$originalString = " Trim this text "
$modifiedString = $originalString.Trim() # Removes leading and trailing whitespace
Write-Output $modifiedString # Output: "Trim this text"
In this case, the `Trim()` method cleans up the string by removing spaces surrounding the actual text.
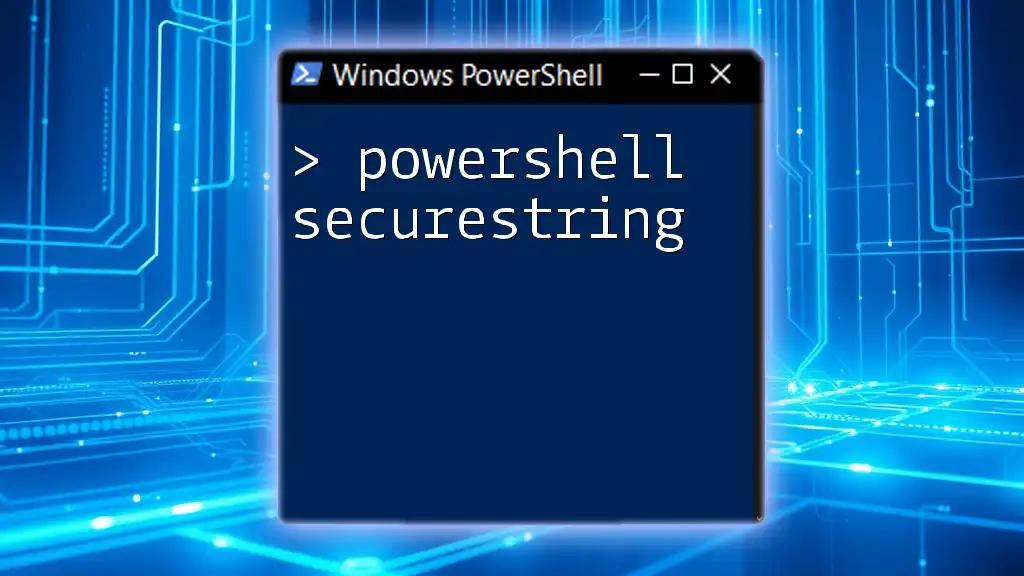
PowerShell Remove String from String: Practical Examples
Removing Substrings by Index
You can remove parts of a string by specifying the index where you want to start removing and how many characters to delete.
Example:
$originalString = "Hello, World!"
$modifiedString = $originalString.Remove(7, 6) # Removes "World"
Write-Output $modifiedString # Output: Hello, !
This removes the substring "World" from the original string, demonstrating how precise index targeting can result in tailored text output.
Removing Multiple Occurrences
The `-replace` operator doesn't only replace text but can also remove multiple occurrences of a substring. By replacing it with an empty string, you effectively remove it.
Example:
$originalString = "Remove and remove again"
$modifiedString = $originalString -replace "remove", ""
Write-Output $modifiedString # Output: " and again"
This effectively removes both instances of "remove", leaving behind just the surrounding text.
Filtering Out Unwanted Characters
Removing unwanted characters such as punctuation is another common requirement. Using regular expressions with the `-replace` operator allows for efficient filtering.
Example:
$originalString = "Hello, World!"
$modifiedString = $originalString -replace '[,!.]', ''
Write-Output $modifiedString # Output: Hello World
In the above code, commas, exclamation marks, and periods are removed, showcasing how you can customize strings for cleaner output.
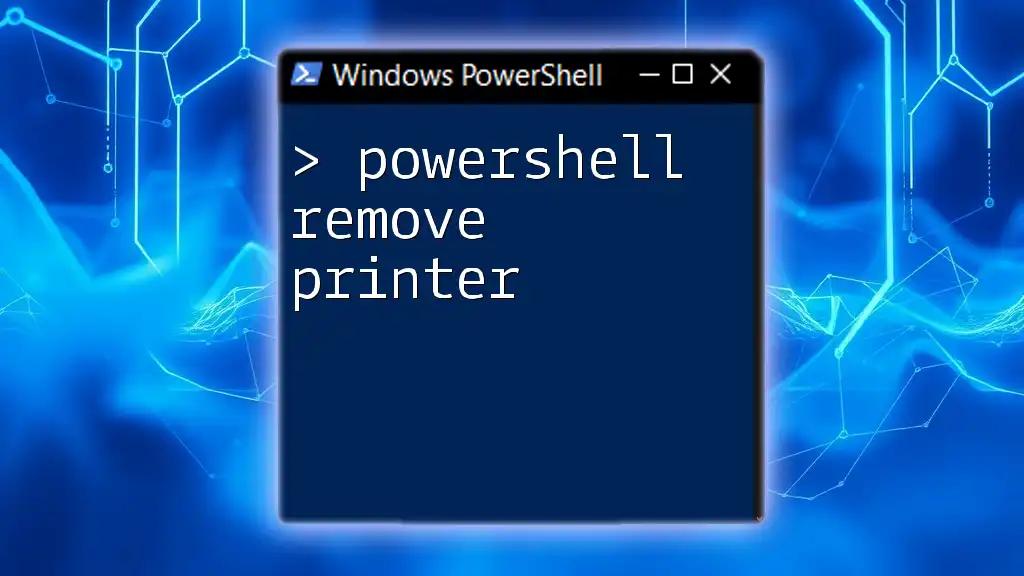
Combining Techniques for Complex Scenarios
Creating a Custom Function to Cleanse Strings
To streamline text processing, creating a custom function can simplify your scripts and enhance reusability. A function can integrate various techniques described earlier to remove unwanted characters.
Example:
function Remove-UnwantedChars {
param ([string]$inputString)
return $inputString -replace '[^a-zA-Z0-9]', '' # Removes anything that's not alphanumeric
}
$result = Remove-UnwantedChars "Hello, World 2023!"
Write-Output $result # Output: HelloWorld2023
In this case, the function `Remove-UnwantedChars` systematically strips the input of anything that is not an alphanumeric character, producing a cleaned string.
Working with Arrays of Strings
String manipulation isn't limited to single strings; you can also apply similar techniques to arrays of strings. Filtering can be done with conditions to remove specific strings.
Example:
$stringArray = @("apple", "banana", "remove", "cherry")
$filteredArray = $stringArray | Where-Object { $_ -ne "remove" }
Write-Output $filteredArray # Output: apple, banana, cherry
This filters out the string "remove", showcasing how PowerShell handles collections.
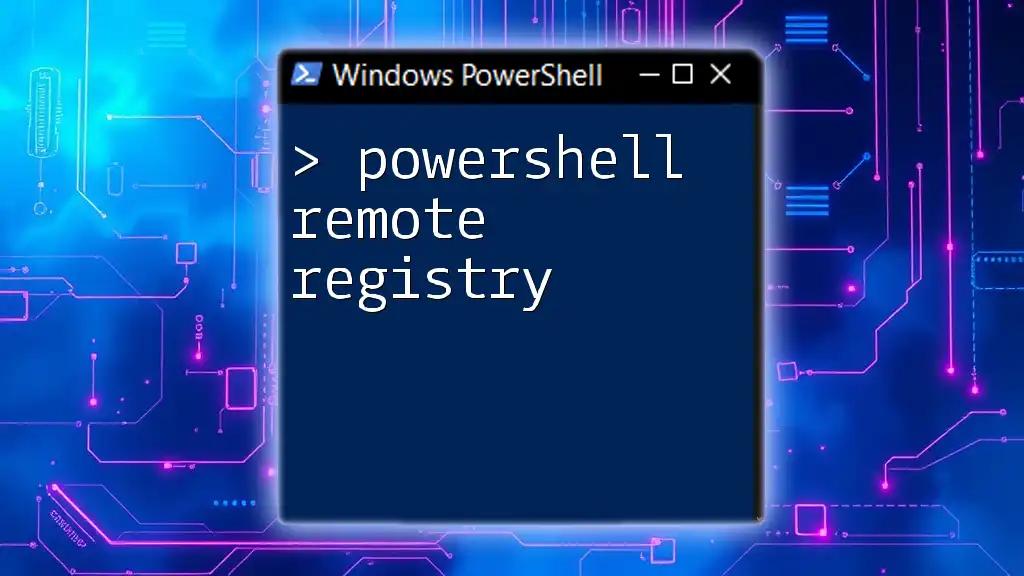
Conclusion
Mastering string manipulation is an essential skill in PowerShell scripting. With the ability to remove text from strings, customize outputs, and create reusable functions, you can significantly enhance the efficiency of your scripts. Practice these techniques routinely to become confident in your string-handling abilities.
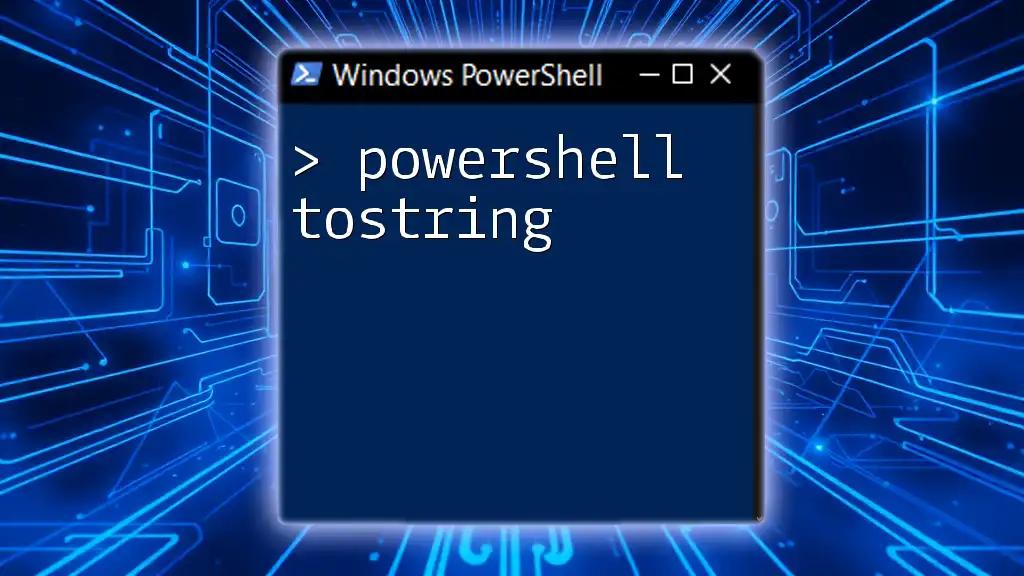
Call to Action
Feel free to share your own challenges or solutions regarding string manipulation in the comments below! Explore more resources and courses provided by our company to further your PowerShell knowledge and skills.