In PowerShell, you can easily extract text from a string using the `-match` operator to match a pattern with regular expressions.
$string = "The quick brown fox jumps over the lazy dog"
if ($string -match 'brown (\w+)') { $extracted = $matches[1]; Write-Host $extracted }
In this example, the code extracts the word that follows "brown" in the given string.
Understanding Strings in PowerShell
What is a String?
A string in PowerShell is a sequence of characters used to represent text. Strings are fundamental in any scripting language, allowing users to manipulate textual data efficiently. They can encompass letters, numbers, symbols, and spaces.
Common Methods of Working with Strings
Strings can be created and manipulated in various ways. In PowerShell, double quotes (`""`) allow variable interpolation, which means any variable contained within the quotes will be resolved:
$name = "World"
$message = "Hello, $name!" # Outputs: Hello, World!
Single quotes (`''`), on the other hand, treat everything literally and will not resolve variables:
$message = 'Hello, $name!' # Outputs: Hello, $name!
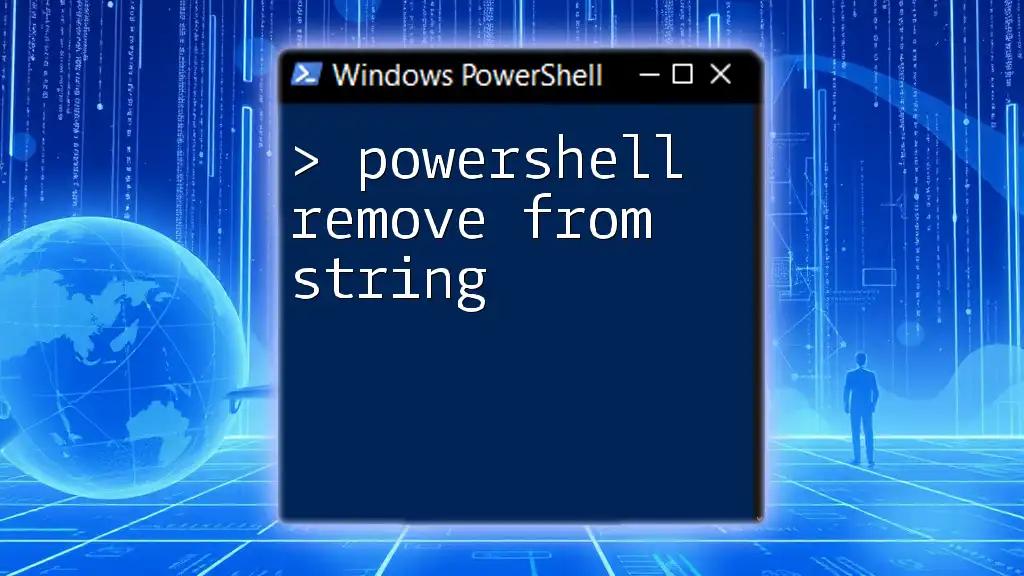
Basic String Manipulation Techniques
Accessing Characters in a String
Strings in PowerShell can be indexed, allowing you to access individual characters. The indexing is zero-based, meaning the first character of a string is at index 0.
For example, to access the character at index 7 in the string "Hello, World!":
$text = "Hello, World!"
$char = $text[7] # Outputs: W
String Length
Finding the length of a string is straightforward in PowerShell. You can use the `.Length` property, which returns the number of characters in the string.
$length = $text.Length # Outputs: 13

Extracting Text from Strings
Using Substring Method
One of the simplest techniques to extract a portion of a string is by using the `Substring` method. This method requires two parameters: the starting index and the length of the substring you wish to extract.
$result = $text.Substring(7, 5) # Outputs: World
Finding Text with IndexOf
When to Use IndexOf
The `IndexOf` method is useful when you need to find the location of a specific substring within a string. If the substring is found, it returns the zero-based index of the first occurrence.
$index = $text.IndexOf("World") # Outputs: 7
Combining IndexOf with Substring
Often, you may need to combine methods to extract text dynamically. By using `IndexOf` to find the starting index and then applying `Substring`, you can extract the desired portion.
$start = $text.IndexOf("World")
$result = $text.Substring($start, 5) # Outputs: World
Regular Expressions for Advanced Extraction
Introduction to Regular Expressions
Regular expressions (regex) provide a powerful way to search and extract patterns from strings. They are particularly useful for complex string extraction tasks.
Using the -match Operator
The `-match` operator allows for pattern matching and can return matched results in the automatic `$matches` variable.
if ($text -match 'W(.*?)!') {
$result = $matches[1] # Outputs: orld
}
The Select-String Cmdlet
For more complex extraction, the `Select-String` cmdlet can be utilized. It's effective for searching text patterns across lines or blocks of text.
$extracted = "Line1: Hello, World!" | Select-String -Pattern "Hello, (.*?)!"
# Outputs: Hello, World!

Practical Examples
Extracting Email Addresses from a String
A common use case involves extracting email addresses from a block of text. By leveraging regex, this becomes straightforward:
$emailText = "Contact us at info@example.com or support@example.org"
$emails = [regex]::Matches($emailText, '[\w\.-]+@[\w\.-]+') | ForEach-Object { $_.Value }
# Outputs: info@example.com, support@example.org
Extracting Specific Data from CSV File
PowerShell's ability to handle structured data like CSV makes it easy to extract specific fields. Assume you have a CSV file containing user data, and you want to extract email addresses:
$csvData = Import-Csv -Path "data.csv"
$extractedData = $csvData | ForEach-Object { $_.Email }
# Outputs: List of email addresses from the CSV file
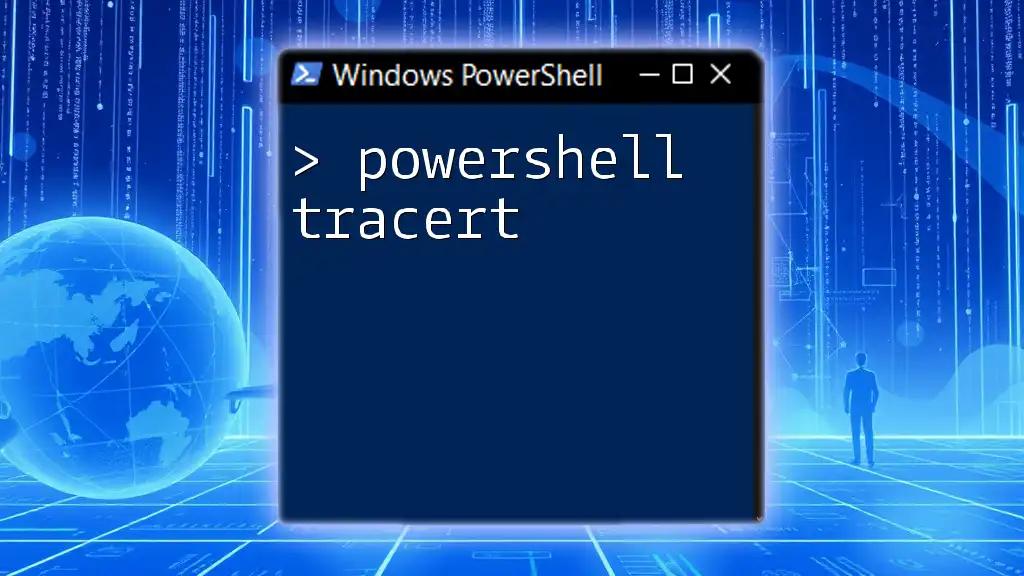
Best Practices for String Extraction
Performance Considerations
When it comes to performance, choosing the right method can have a significant impact. For straightforward substring extraction, using the `Substring` and `IndexOf` methods is typically faster. For complex pattern extraction, regex is more robust but can be slower due to its complexity.
Readability and Maintainability
Clean code is critical in any scripting or programming task. Make sure to use descriptive variable names and comment on your code where appropriate. This will aid both your future self and collaborators in understanding the intentions behind your scripts.
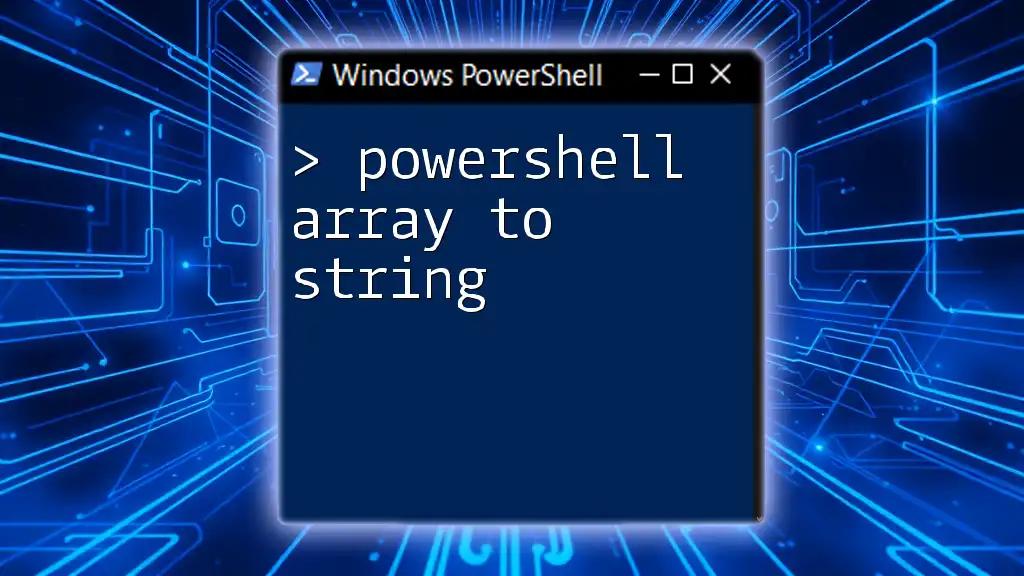
Conclusion
In this guide, we explored various ways to extract text from strings in PowerShell, from simple methods like `Substring` to the more complex use of regular expressions. By leveraging these techniques, you can enhance your automation scripts, making them more efficient and easier to maintain.
Additional Resources
For those interested in diving deeper, consider exploring the official PowerShell documentation for more advanced topics and best practices regarding string manipulation and regex.