In PowerShell, you can extract a specific value from an object using the dot notation to access its properties, like this:
# Example: Extracting the 'Name' property from an object
$person = New-Object PSObject -Property @{ Name = 'John'; Age = 30 }
$person.Name
Understanding PowerShell Objects
What is a PowerShell Object?
In PowerShell, everything is an object. This means that when you use cmdlets, filters, and procedures, you're working with structured data known as objects. Objects are instances of types, and they can have properties (attributes) and methods (functions) that operate on those properties.
Types of Objects in PowerShell
PowerShell works with various object types, including:
- Cmdlets: Built-in PowerShell commands that return object types.
- Custom Objects: User-defined objects that can encapsulate data.
- .NET Objects: Objects from the .NET framework that leverage the extensive libraries that .NET provides.

Accessing Object Properties
Dot Notation
One of the most straightforward ways to extract values from objects in PowerShell is through dot notation. This involves using a period (dot) to navigate to the desired property of an object.
For example, to access the `DisplayName` property of a service object, you can execute:
$object = Get-Service | Select-Object -First 1
$object.DisplayName # Accessing DisplayName property
This command retrieves the first service object and extracts its `DisplayName`.
Using Select-Object
The `Select-Object` cmdlet enhances your ability to extract specific properties from an object.
Selecting Specific Properties
This approach is beneficial when you want to see or work with only certain attributes.
For instance:
Get-Service | Select-Object -Property Name, Status
This command fetches the names and statuses of all services, filtering only the required properties.
Creating Custom Objects
Defining custom objects allows you to encapsulate data in a structured format. You can define custom properties and access them similarly to standard object properties.
Here's an example of creating a custom object:
$customObject = New-Object PSObject -Property @{ Name = "Test"; Value = 123 }
$customObject.Name # Accessing custom property
This will give you the output:
Test
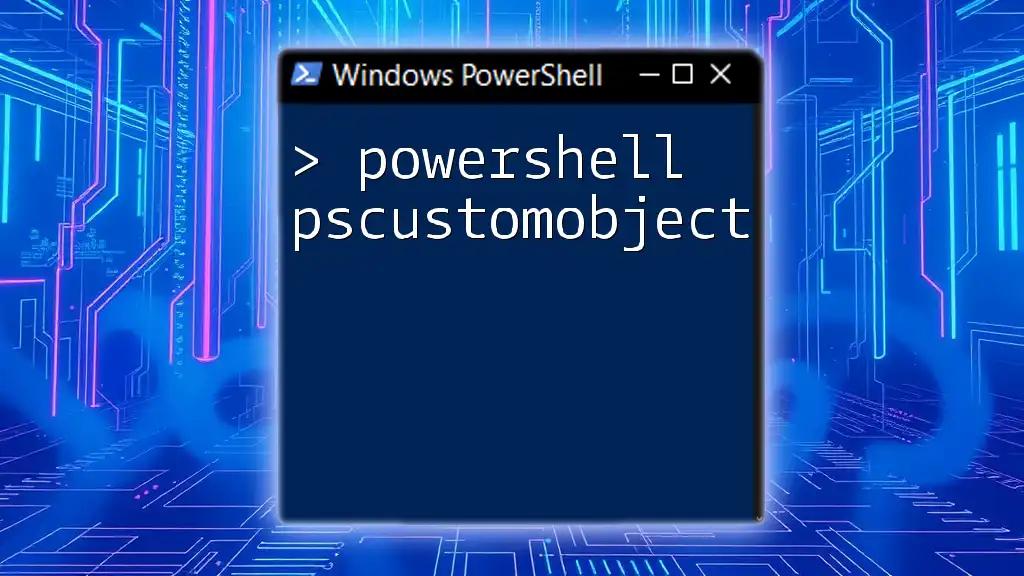
Extracting Values from Nested Objects
Understanding Nested Objects
Nested objects occur when properties of an object themselves contain objects. Understanding this concept is crucial for extracting nested properties efficiently.
Accessing Nested Properties
You can still use dot notation to access properties of nested objects. For example, if you retrieve a process using `Get-Process`, you can extract the `MainWindowTitle` property directly:
$process = Get-Process | Select-Object -First 1
$process.MainWindowTitle # Accessing nested property
This extracts the title of the main window of the first process returned.
Using ForEach-Object for Collections
When dealing with collections of objects, the `ForEach-Object` cmdlet is invaluable. This cmdlet allows you to apply an operation to each item in a collection.
For instance, if you want to extract and display the names of all running services, you would use:
Get-Service | ForEach-Object { $_.Name }
The `$_` variable represents the current object in the pipeline.

Filtering and Parsing Object Values
Using Where-Object for Filtering
The `Where-Object` cmdlet is essential for filtering objects based on specific conditions. It allows you to focus on relevant data more effectively.
As an example, if you want to find processes using excessive CPU, you could execute:
Get-Process | Where-Object { $_.CPU -gt 100 }
This command will return only the processes where CPU usage exceeds 100.
Parsing Values from Strings
Using -split and Regular Expressions
Sometimes, data is stored in strings, necessitating parsing to extract useful values. You can use the `-split` operator and regular expressions to achieve this.
For example, if you have a string like the following:
$string = "Name:Test;Value:123"
$parsed = $string -split ';'
This operation splits the string at the semicolon, allowing you to manipulate or examine individual components.
Transforming Data with Select-Object
The `Select-Object` cmdlet allows for more than just simple extraction. It can also transform data using calculated properties.
For instance:
Get-Service | Select-Object Name, @{Name='ServiceStatus';Expression={if($_.Status -eq 'Running'){'Active'}else{'Inactive'}}}
In this example, a new property called `ServiceStatus` evaluates whether the service is Active or Inactive based on its status.
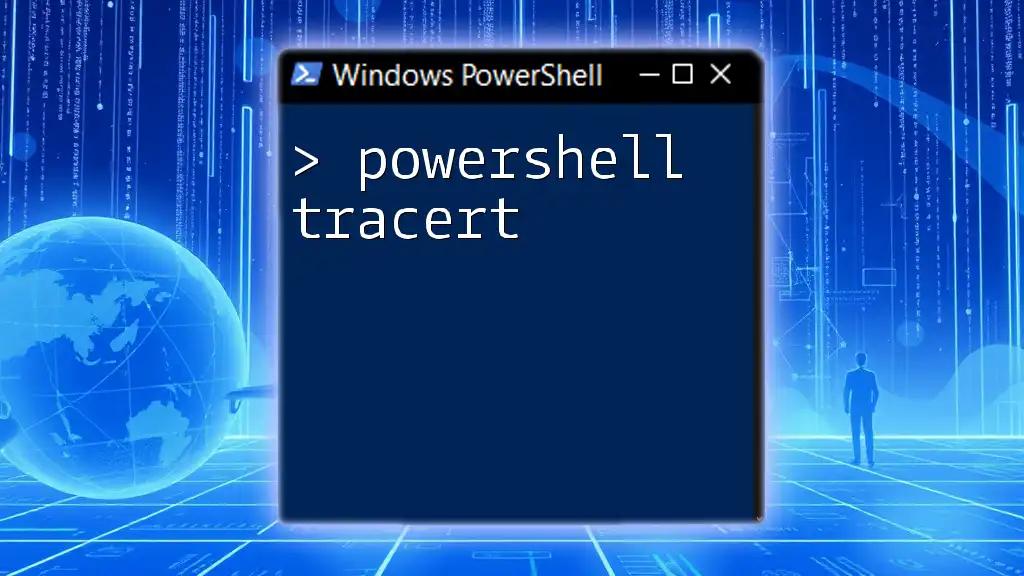
Common Use Cases
Retrieving System Information
PowerShell is often used to gather essential system information. A practical example of this would be to get the operating system name and version:
Get-ComputerInfo | Select-Object OsName, WindowsVersion
This illustrates how easy it is to extract values from objects when running system diagnostics.
Working with Active Directory Objects
If you manage an Active Directory environment, PowerShell can streamline retrieving user information.
For example, you can extract names and email addresses using:
Get-ADUser -Filter * | Select-Object Name, EmailAddress
This command will output the name and email address of all users in the directory.

Best Practices
Ensuring Code Readability
When writing PowerShell scripts, ensure that your code is readable. Use meaningful variable names and consider breaking lengthy scripts into smaller functions or segments.
Avoiding Common Pitfalls
Common pitfalls include not validating data before processing or misunderstanding object properties. Always remember to verify the data types and check for null values to avoid unexpected behaviors in your scripts.
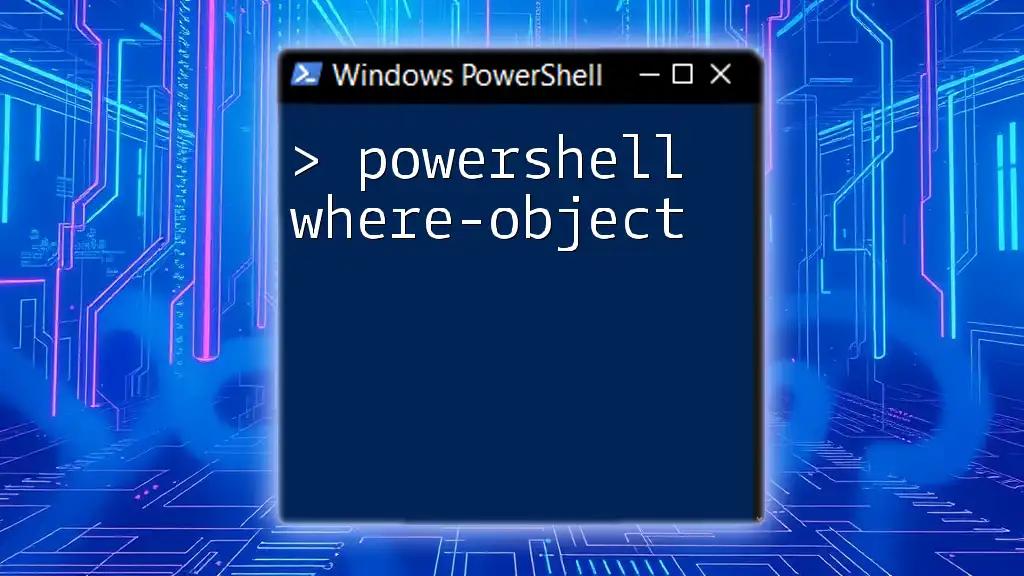
Conclusion
Throughout this guide, we've explored various techniques and methodologies regarding how to effectively extract values from objects in PowerShell. By leveraging dot notation, cmdlets like `Select-Object` and `Where-Object`, and understanding the structure of nested objects, you're well-equipped to manipulate and retrieve data efficiently. Empower yourself through practice and continual learning to harness the full potential of PowerShell in your workflows.