The "Expand-Properties" function in PowerShell allows you to display all the properties of an object in a more detailed manner, making it easier to view complex data structures.
Here's a code snippet demonstrating how to expand all properties of a given object:
Get-Process | Select-Object -Property * | Format-List *
Understanding PowerShell Objects
What are PowerShell Objects?
In PowerShell, everything revolves around objects. These are data structures that encapsulate information and associated behaviors, similar to objects in programming languages like C# or Java. Unlike traditional variables that can only hold simple data types (like strings or integers), PowerShell objects can contain multiple properties and methods.
For example, when you retrieve a file using `Get-ChildItem`, you are actually working with a collection of file system objects. Each of these objects has properties like `Name`, `CreationTime`, and `Length`. Understanding how to manipulate these objects will enable you to interact with your system more effectively.
The Importance of Properties
Properties are the attributes of an object that describe its state or characteristics. Each PowerShell object comes equipped with a set of properties, and accessing these is critical for effective scripting and automation.
For instance, a user account object in Active Directory would have properties like `Username`, `EmailAddress`, and `LastLogon`. Knowing how to access and expand these properties can enhance your administrative tasks or troubleshooting effectiveness.
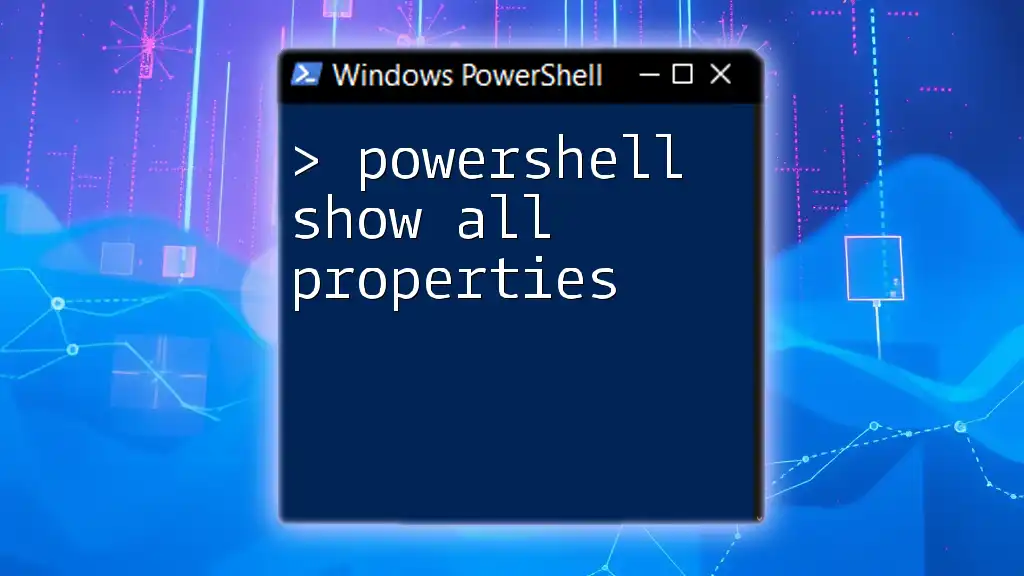
The Need for Expanding Properties
Why Expand Properties?
When working with objects, especially when dealing with large datasets or system configurations, you may encounter objects that contain nested or hidden properties. Expanding properties allows you to view all relevant data, which is crucial for administrative tasks and debugging scripts.
Imagine you're trying to identify issues with a service. By expanding the properties, you can view information such as the service status, dependent services, and configuration settings. This is impossible if you only see limited property outputs.
Common Use Cases
Some typical scenarios where expanding PowerShell properties is essential include:
- Administrative Tasks: Managing users, retrieving system information, and configuring services.
- Data Analysis: Running queries on databases or logs where object structures can be complex.
- Debugging Scripts: Understanding the full structure of objects to troubleshoot effectively.
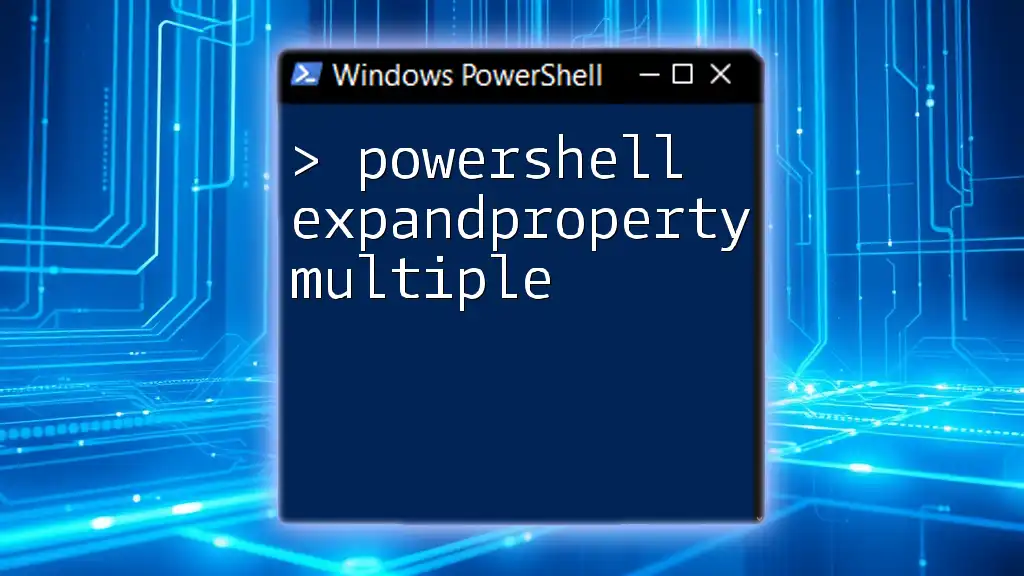
Using `Select-Object` to Expand Properties
Introduction to `Select-Object`
The `Select-Object` cmdlet is one of PowerShell's most powerful tools, allowing you to select specific properties from an object. The syntax is straightforward, making it an excellent choice for data manipulation.
Example: Using `Select-Object` to Show All Properties
To retrieve all properties of a service, you can use the following command:
Get-Service | Select-Object *
This command outputs a list of all attributes associated with each service, giving you a comprehensive view of their states and attributes. Accessing all properties can surface details that are critical for effective system management.
Filtering Specific Properties
If you only need select properties while expanding others for further insight, `Select-Object` can suit this need perfectly. For instance, consider the following command:
Get-Process | Select-Object Name, Id, @{Name='MemoryUsage'; Expression={$_.WorkingSet/1MB}}
In this example, we retrieve the process names and IDs while calculating the memory usage in megabytes. This flexibility allows you to customize your output according to your analytics or administrative requirements.
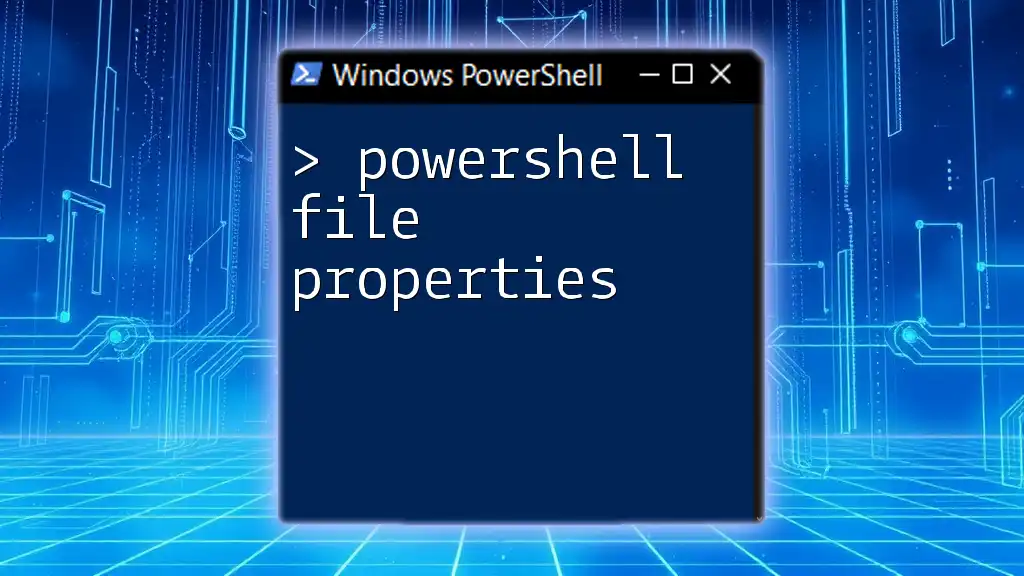
Using `Format-List` to Expand All Properties
Overview of `Format-List`
The `Format-List` cmdlet is fundamentally different from `Select-Object`. While `Select-Object` is concerned with selecting properties, `Format-List` formats the output as a list where all properties are expanded.
Example: Displaying All Properties with `Format-List`
To display all properties of processes as a formatted list, use:
Get-Process | Format-List *
The output will display each property on a separate line, allowing you to read complex information quickly. This is particularly useful when working with objects that contain many properties.
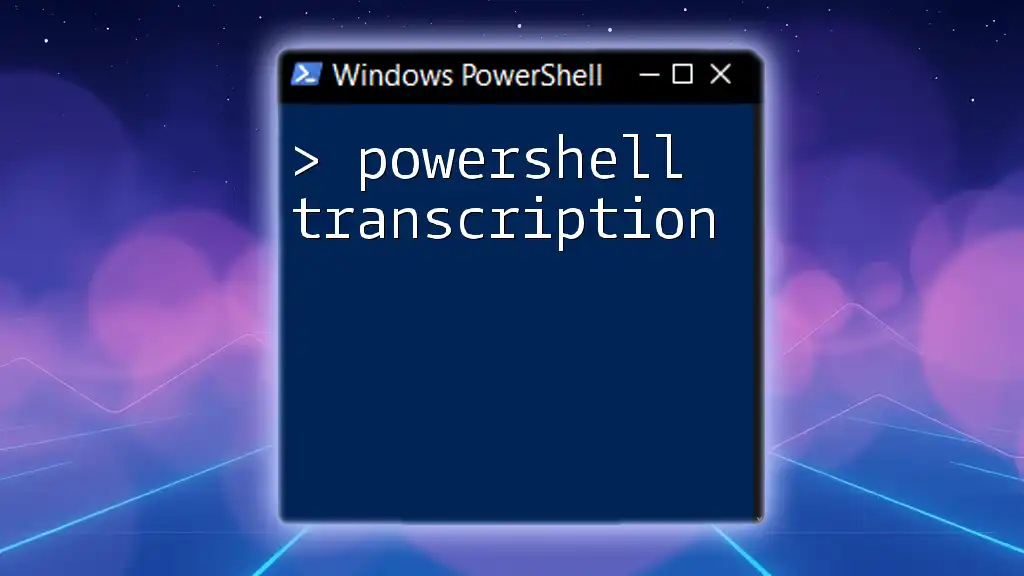
Exploring Nested Properties
What are Nested Properties?
Nested properties are properties within properties. These can commonly be found in objects that contain collections or structures, such as user profiles or organizational units in Active Directory. Understanding how to navigate these nested structures is key for advanced PowerShell work.
How to Expand Nested Properties
You can retrieve and expand nested properties using the `Select-Object` cmdlet and leveraging calculated properties via scripting. For instance:
Get-ADUser -Identity 'username' | Select-Object *, @{Name='Phone'; Expression={$_.TelephoneNumber}}
This example expands the user account properties, featuring a calculated property to show the user’s phone number, demonstrating how you can selectively extract useful information.
Handling Complex Data Structures
To navigate complex objects effectively, scripts can include loops and custom functions. For example, if you have multiple nested properties and want to output them in a more user-friendly format, you might iterate over these properties using a `ForEach-Object` loop:
Get-Item "C:\Logs\*" | ForEach-Object {
$_.PSObject.Properties | ForEach-Object {
Write-Host "$($_.Name): $($_.Value)"
}
}
This snippet allows you to expose all properties regardless of how deeply nested they are.
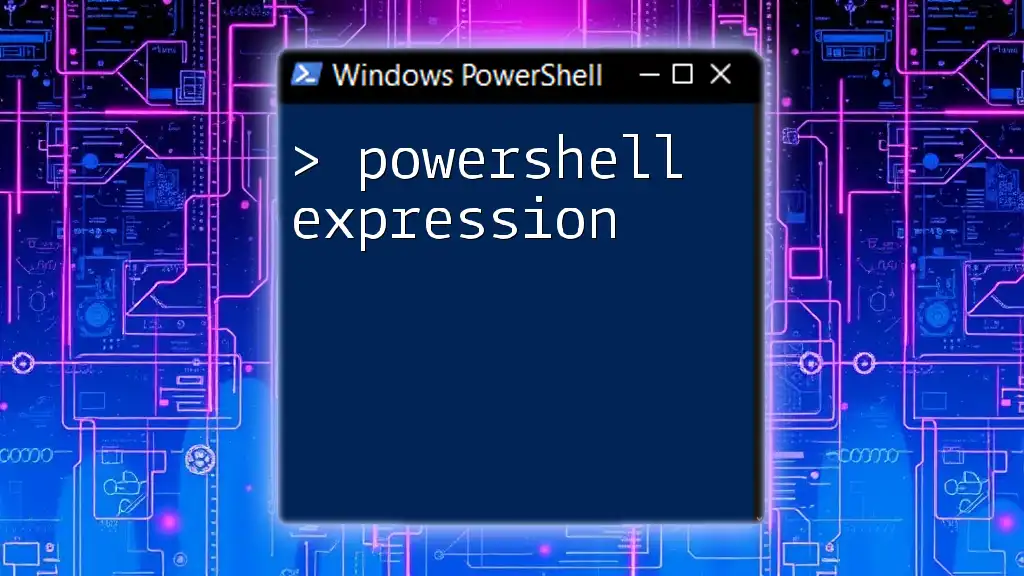
Working with Custom Objects
Creating Custom Objects with PSObject
Through PowerShell, you can create your own custom objects using `PSObject`. This capability provides flexibility in structuring the data as per your requirements:
$myObject = New-Object PSObject -Property @{
Name = "Test"
Age = 30
}
This simple command creates a new object with defined properties. When you urgently need specific data to visualize or process, creating custom objects can save time.
Adding Nested Properties
To enrich a custom object with even more intricate structures, you can add nested properties using the `Add-Member` cmdlet:
$myObject | Add-Member -MemberType NoteProperty -Name 'Address' -Value @{Street='123 Main St'; City='Anytown'}
Now, `$myObject` has an address property that stores a hashtable, effectively showing how flexible PowerShell can be when organizing data.
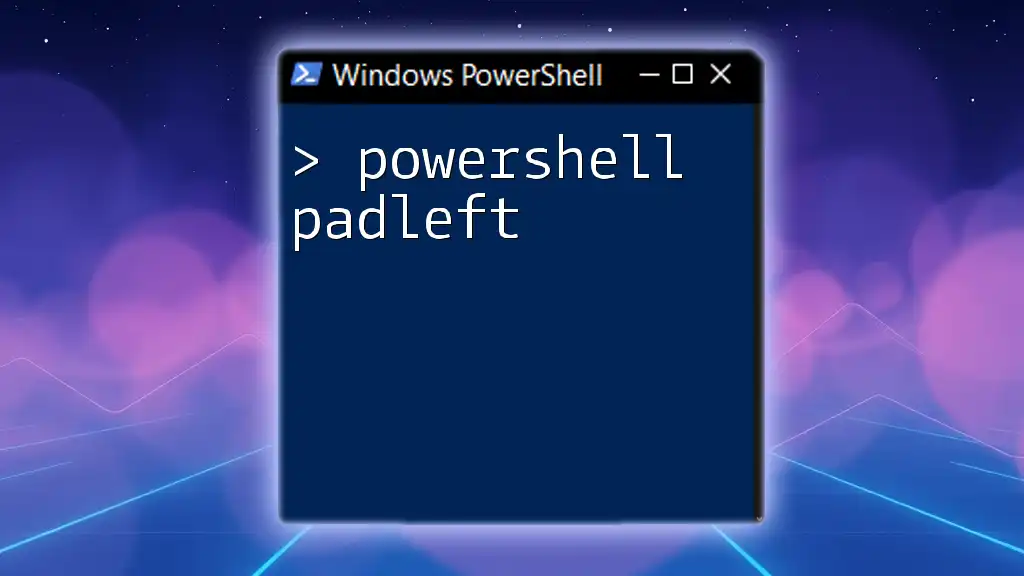
Practical Examples and Use Cases
Script Example: Managing User Accounts
Imagine a scenario where you need to manage Active Directory accounts efficiently. A PowerShell script that expands user properties could look like this:
Get-ADUser -Filter * | Select-Object Name, EmailAddress, LastLogon
This command provides a direct insight into multiple user properties, allowing administrators to audit user accounts quickly.
Example: Pulling System Information
Another useful example is gathering comprehensive system information from a WMI class. Run the following command:
Get-WmiObject -Class Win32_OperatingSystem | Select-Object *
This command will output all properties of the operating system, allowing for detailed insight into your system configuration, which is invaluable during troubleshooting.
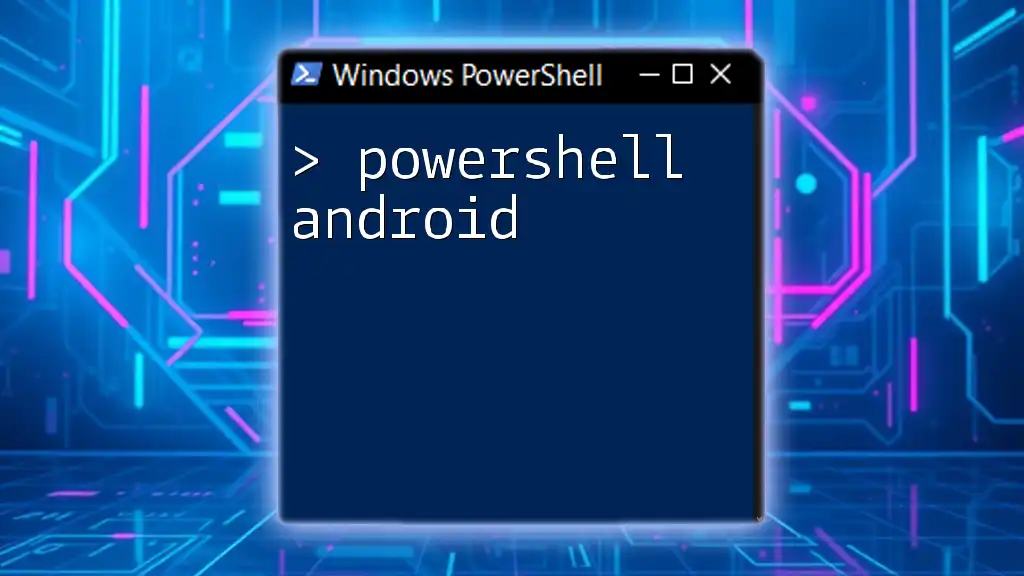
Conclusion
Throughout this guide, we’ve explored how to expand all properties of PowerShell objects, an essential skill for any admin or scripter. By utilizing cmdlets like `Select-Object`, `Format-List`, and techniques for accessing nested properties, you can enhance your PowerShell scripting arsenal significantly.
Understanding these principles will enable you to interact more effectively with objects, ultimately streamlining your administrative tasks and enhancing your overall productivity in PowerShell.
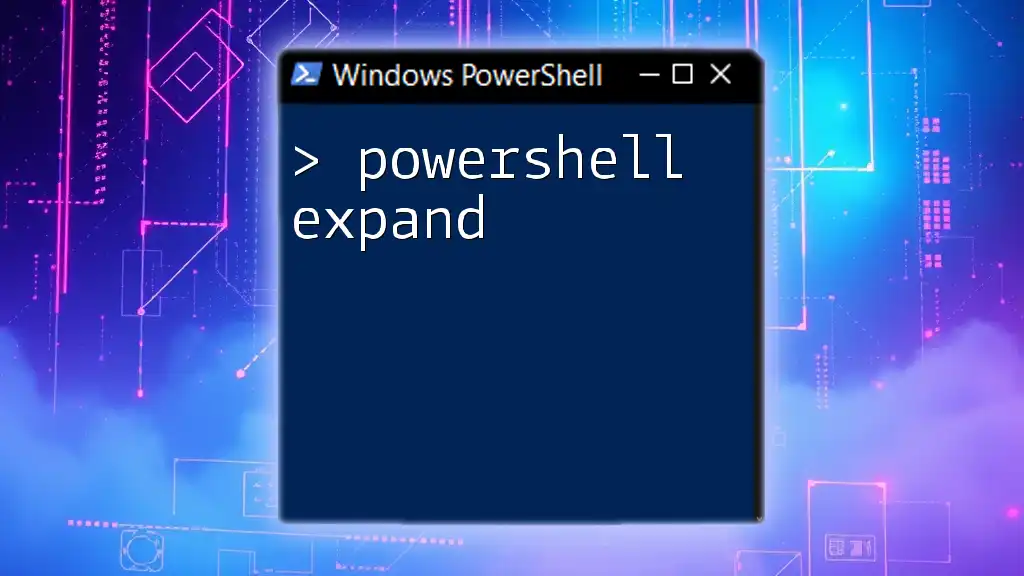
Additional Resources
For those wishing to delve deeper into PowerShell, consider checking out the official Microsoft PowerShell documentation or resources such as community forums, online courses, and recommended books to master this incredibly powerful tool.
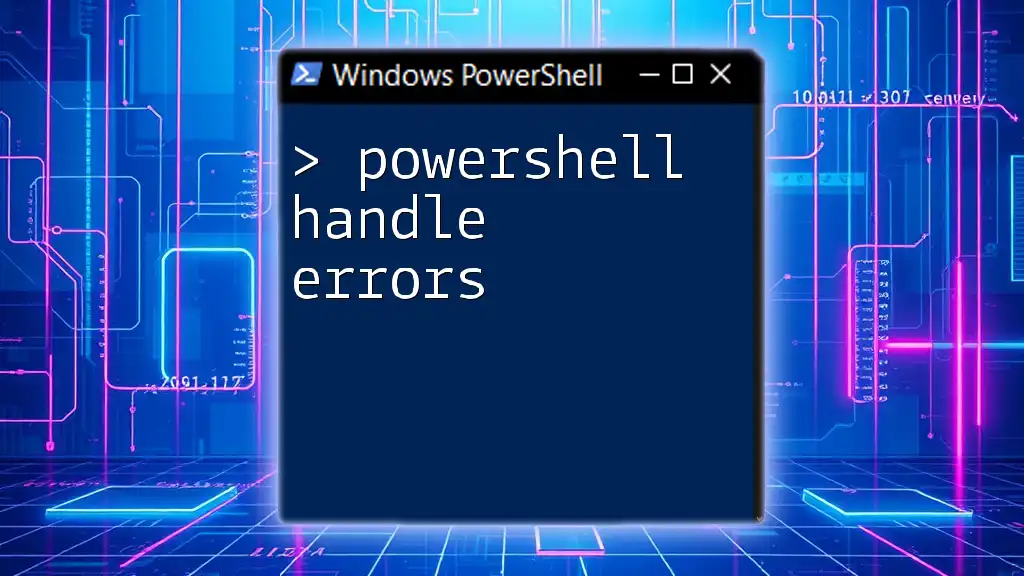
Call to Action
We invite you to subscribe to our blog for even more PowerShell tips, commands, and practical guidance. Feel free to share your experiences or ask questions in the comments – your journey in mastering PowerShell starts here!