To remove the last character from a string in PowerShell, you can use the `Substring` method, which extracts a portion of the string up to the last character. Here's how you can do it:
$string = "Hello, World!"
$result = $string.Substring(0, $string.Length - 1)
Write-Host $result
Understanding String Manipulation in PowerShell
What is a String?
In programming, a string is a sequence of characters enclosed in quotation marks, representing text. In PowerShell, strings are fundamental data types you will often manipulate. From filenames to user inputs, strings play a pivotal role in scripting.
Why Remove the Last Character from a String?
Removing the last character of a string can be essential for various tasks, such as formatting outputs or cleaning data. Common scenarios include:
- Trimming trailing punctuation from user input.
- Adjusting strings used in file paths.
- Ensuring data integrity before storage or further processing.
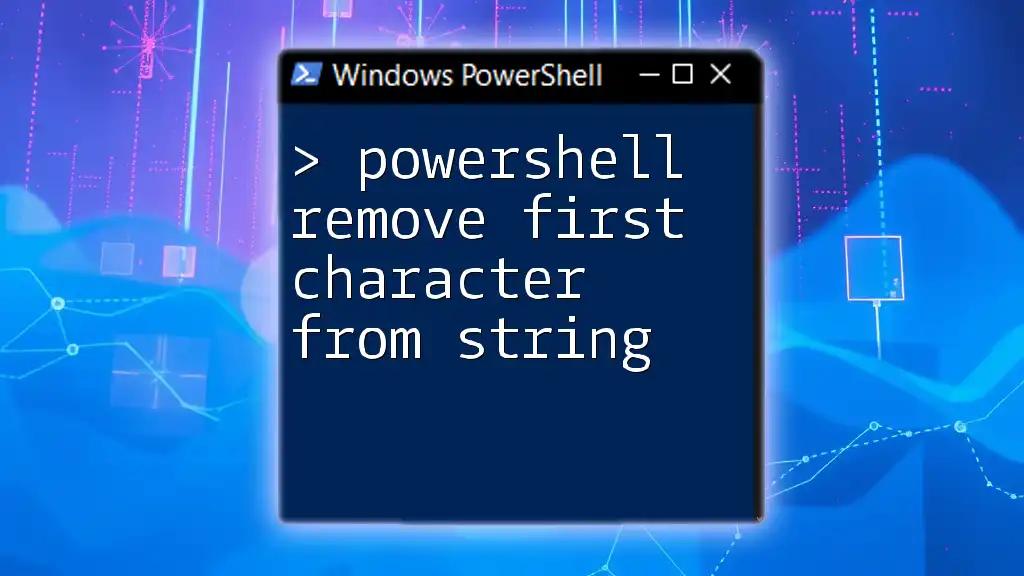
Basic Method to Remove the Last Character
Using the `Substring` Method
The `Substring` method allows you to extract a portion of a string. To remove the last character, we essentially extract everything except the last character.
Here’s how you would do it:
$string = "Hello World!"
$trimmedString = $string.Substring(0, $string.Length - 1)
Write-Output $trimmedString
Output:
Hello World
In this example, `Substring(0, $string.Length - 1)` starts at index 0 and extracts characters up to, but not including, the last character. This method is straightforward and efficient for trimming the last character from a string.
Using the `.Remove()` Method
The `.Remove()` method provides another way to eliminate the last character from a string. Unlike `Substring`, this method removes a specified number of characters starting from a given index.
Consider this example:
$string = "Hello World!"
$trimmedString = $string.Remove($string.Length - 1)
Write-Output $trimmedString
Output:
Hello World
Here, the command `Remove($string.Length - 1)` tells PowerShell to remove one character starting from the index of the last character in the string. This method is clear and operates on the string by directly specifying the position of the character to remove.
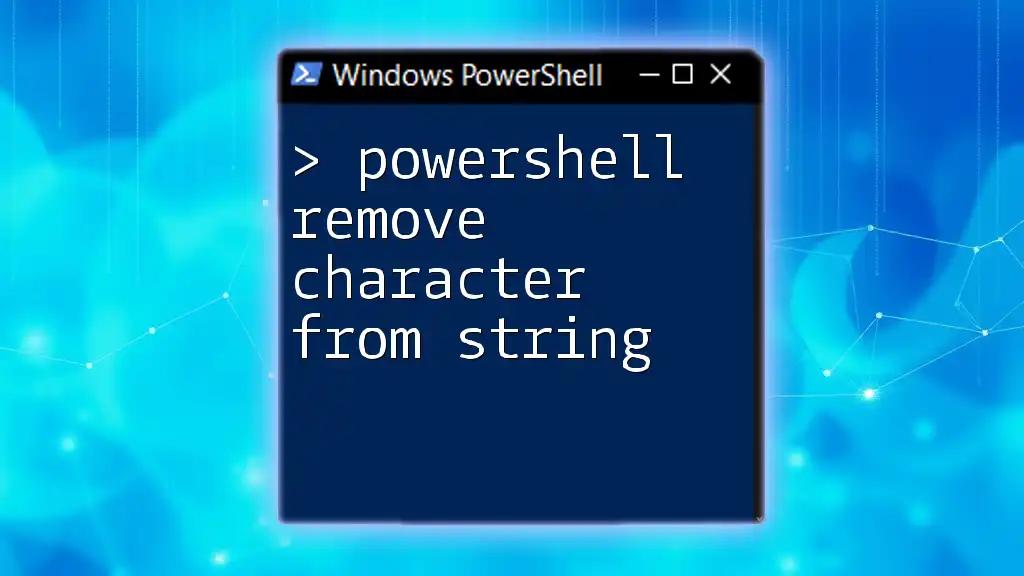
Advanced Techniques for Removing Last Character
Utilizing Regular Expressions
Regular expressions (regex) offer powerful capabilities for text manipulation, including trimming operations. With regex, we can effectively match and remove the final character from a string.
Here’s how to utilize regex for this task:
$string = "Hello World!"
$trimmedString = [regex]::Replace($string, '.$', '')
Write-Output $trimmedString
Output:
Hello World
In this snippet, the regex pattern `.$` matches the last character (indicated by the `.`) at the end of the string (indicated by `$`). The `Replace` method substitutes it with an empty string, thereby removing it. Regular expressions can handle more complex patterns, making them highly versatile.
PowerShell Trim Last Character with Conditional Logic
In some cases, you may want to ensure your script is robust enough to handle various string conditions, such as empty strings or strings with length 1.
Here’s an example that incorporates conditional logic:
$string = "H"
$trimmedString = if ($string.Length -gt 1) { $string.Substring(0, $string.Length - 1) } else { "" }
Write-Output $trimmedString
Output:
(empty string)
In this code snippet, we check if the length of the string is greater than 1. If so, we proceed to remove the last character; if not, we return an empty string. This handling helps prevent errors and unintended behavior when dealing with short or empty strings.
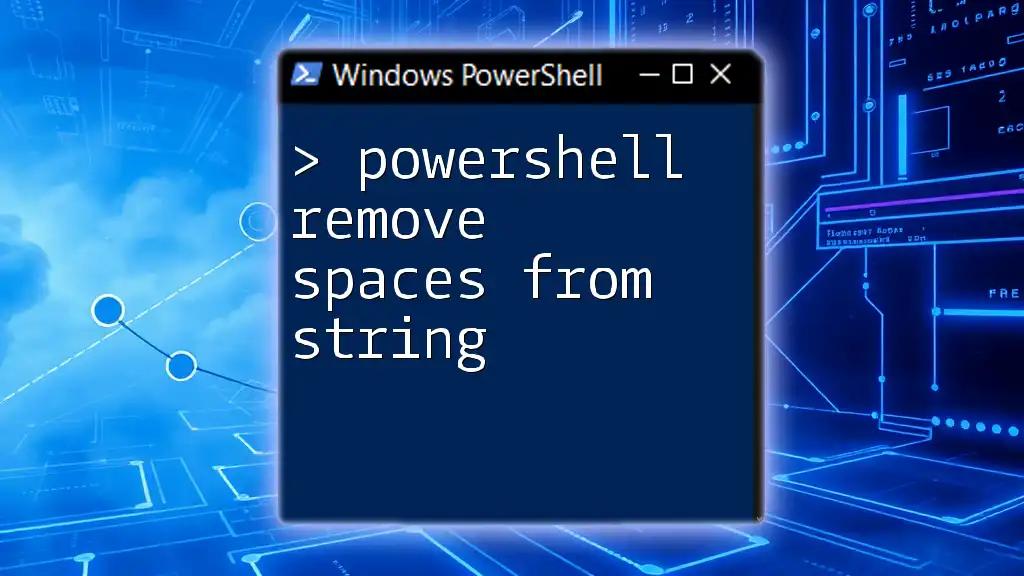
Performance Considerations
Which Method is the Fastest?
When determining performance between these methods, `Substring` and `.Remove()` are generally comparable, but performance may vary based on context. For most typical scenarios, either will suffice. However, regex can be slower due to its complexity in pattern matching, especially with larger strings. For simple tasks like removing the last character, stick to using `Substring` or `.Remove()` for optimal performance and readability.
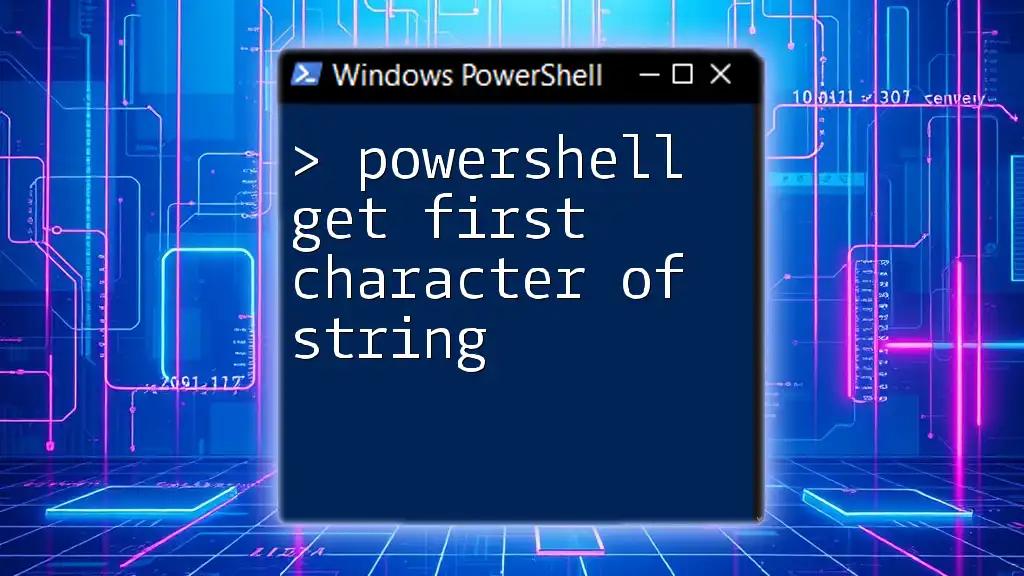
Practical Applications
Integrating into PowerShell Scripts
When implementing the ability to remove the last character from a string in real-world situations, you might find this capability useful in a variety of scripts. For instance, if you are processing files, you could use these methods to format filenames properly.
$filename = "report.txt!"
$formattedFilename = $filename.Remove($filename.Length - 1)
Write-Output $formattedFilename # Outputs "report.txt"
In this scenario, we're using `.Remove()` to ensure that an unintended character at the end of a filename does not prevent the proper utilization in file operations.
User Input Scenarios
User input often contains extra characters due to mistakes or formatting. By applying the techniques highlighted, you can sanitize input effectively.
$userInput = "UserEntry!"
$cleanInput = [regex]::Replace($userInput, '.$', '')
Write-Output $cleanInput # Outputs "UserEntry"
In this example, we handle potential extraneous characters that may confuse further processing. Utilizing regex enhances flexibility and control over string cleansing.
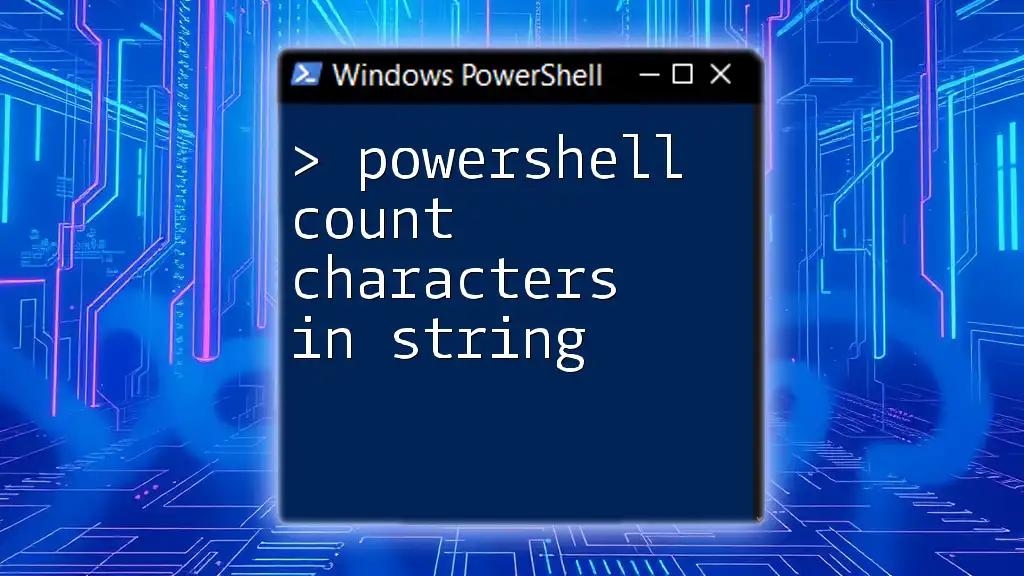
Troubleshooting Common Errors
Common Mistakes and How to Avoid Them
When working with string manipulation, it's easy to run into common issues such as:
- Invalid operations on empty strings: Always verify the string length before attempting to manipulate it.
- Off-by-one errors: Ensure that your indices align correctly, particularly when working with string lengths.
To prevent these, incorporate checks to validate string conditions before performing operations, as showcased in previous examples.
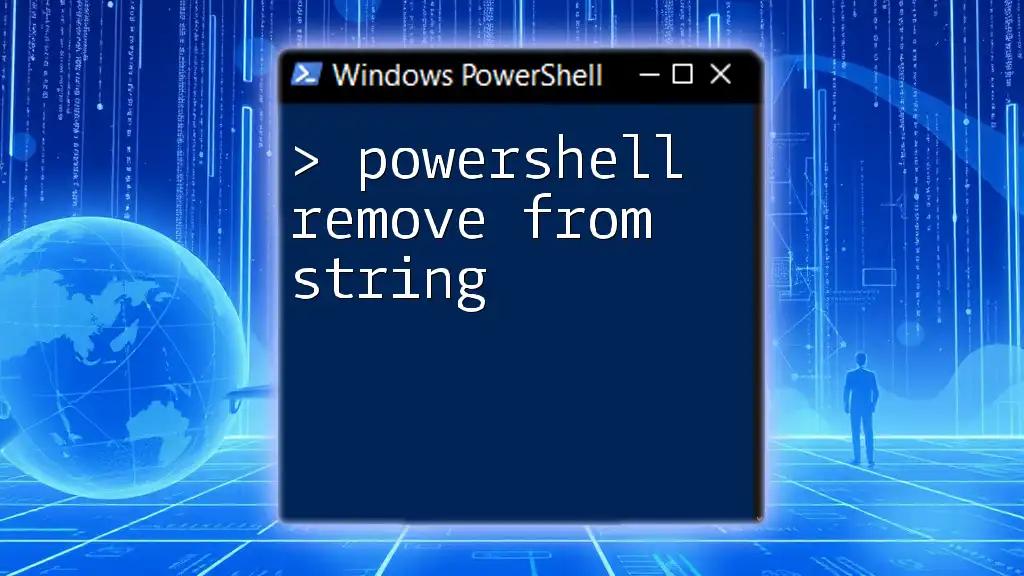
Conclusion
Throughout the article, we explored several methods to remove the last character from a string using PowerShell. Each method—whether using `Substring`, `.Remove()`, or regex—is powerful in its own right and can serve different needs. By getting comfortable with these techniques, you'll enhance your scripting capabilities, allowing for cleaner input handling and more efficient string management.
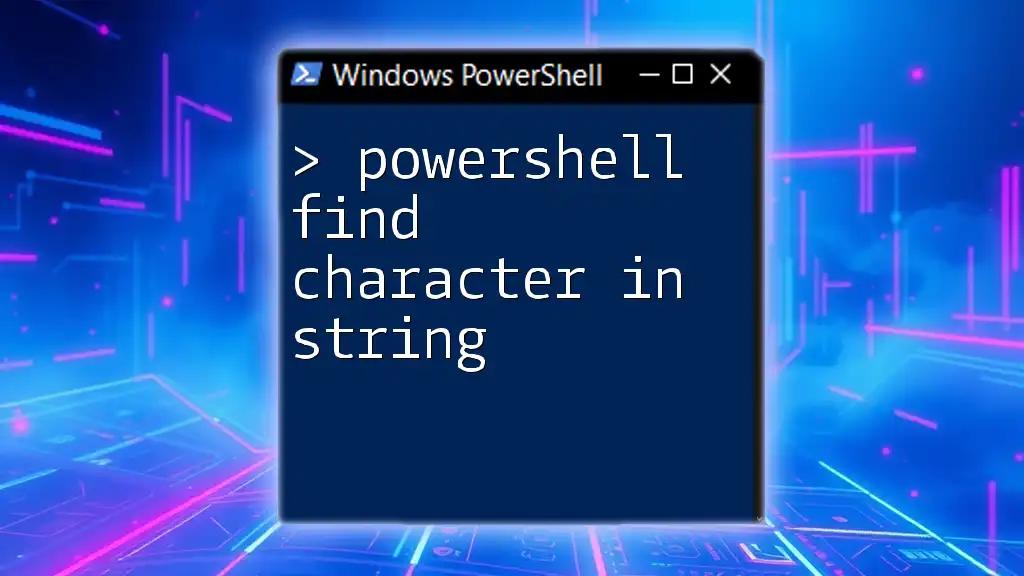
Further Reading and Resources
For those interested in diving deeper into PowerShell string manipulation, consider exploring the official Microsoft documentation, which provides extensive detail on string methods, regex, and best practices.
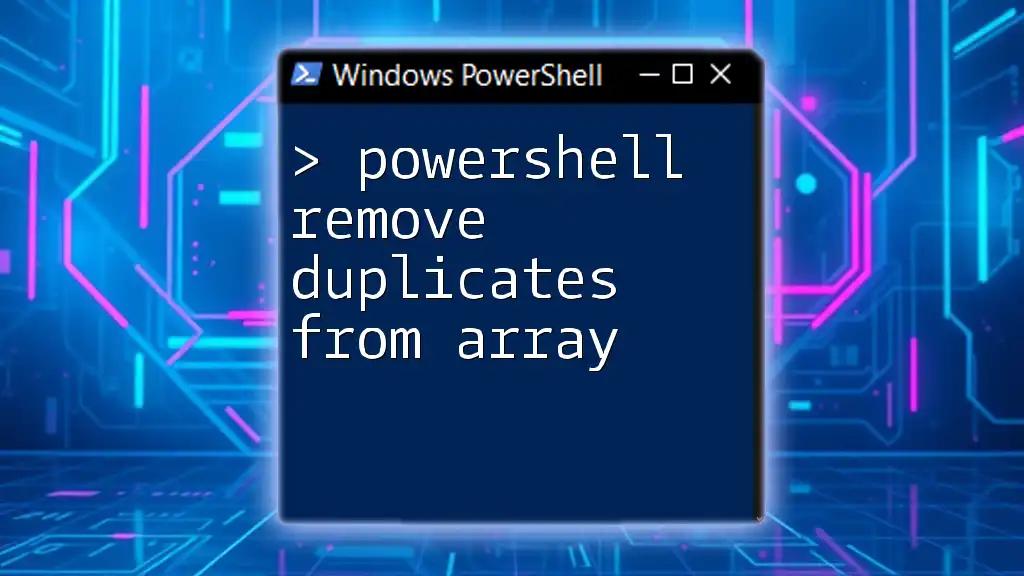
FAQ
Can I remove multiple characters at once?
Yes! For removing multiple characters, you would adjust the length parameter in either `Substring` or `.Remove()` methods. You can also utilize regex patterns for more complex removals.
How to trim whitespace from the end of a string?
To trim whitespace specifically, you can use the `TrimEnd()` method. It allows you to remove specific characters from the end of a string without needing to specify indices.
Are there built-in functions for string manipulation?
Absolutely! PowerShell has various built-in string functions such as `Join-String`, `Split-String`, and others that simplify text manipulation tasks. Use these functions to streamline your scripts and enhance functionality.