To find a specific registry value using PowerShell, you can utilize the `Get-ItemProperty` cmdlet, which efficiently retrieves the properties of a specified registry key.
Here's a code snippet that demonstrates how to find a registry value:
Get-ItemProperty -Path 'HKCU:\Software\YourSoftware' -Name 'YourValueName'
Understanding the Windows Registry
What is the Windows Registry?
The Windows Registry is a hierarchical database used by the operating system to store configuration settings and options for the operating system and installed applications. It contains information, settings, and options for both the operating system and applications installed on Windows. The registry is structured in keys and values, where keys are like folders containing values, which hold the specific data points you want to manage.
Overview of PowerShell and the Registry
PowerShell offers a powerful way to interact with the Windows Registry. By leveraging cmdlets, you can easily automate tasks, query values, and modify registry settings programmatically. This capability makes PowerShell a preferred tool for both system administrators and advanced users.
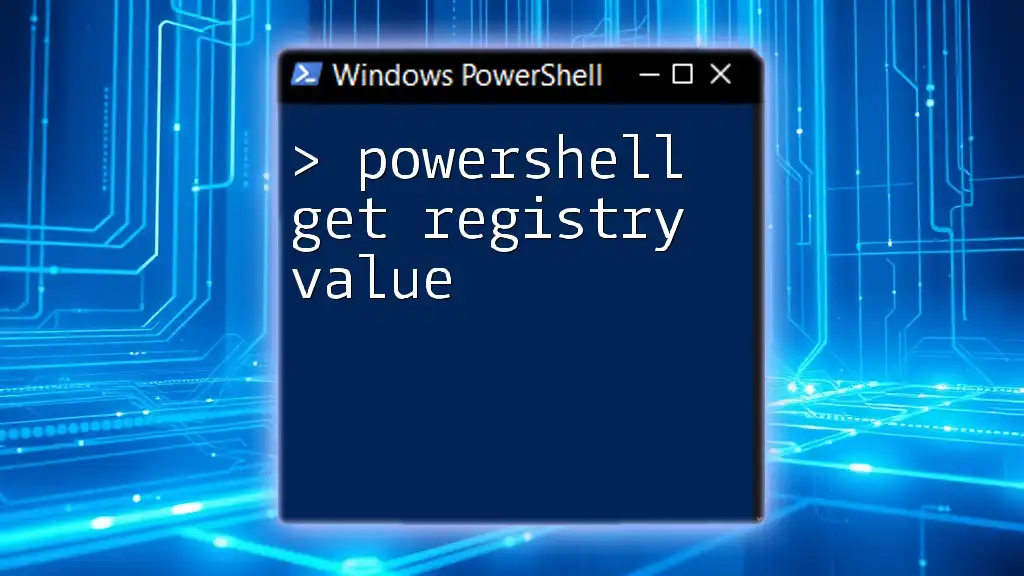
PowerShell Registry Command Basics
Introduction to Cmdlets
PowerShell utilizes cmdlets, which are lightweight commands used in the PowerShell environment. For registry operations, some key cmdlets include:
- Get-Item: Allows you to retrieve a specific registry key.
- Get-ChildItem: Used to list all the subkeys and values in a specified registry path.
- Get-ItemProperty: Fetches the properties associated with a registry key.
Setting Up Your Environment
Before you can effectively work with the registry using PowerShell, ensure you have the necessary permissions. Running PowerShell as an administrator is often required, especially for keys under `HKEY_LOCAL_MACHINE` (HKLM). To do this, right-click the PowerShell icon and select Run as administrator.
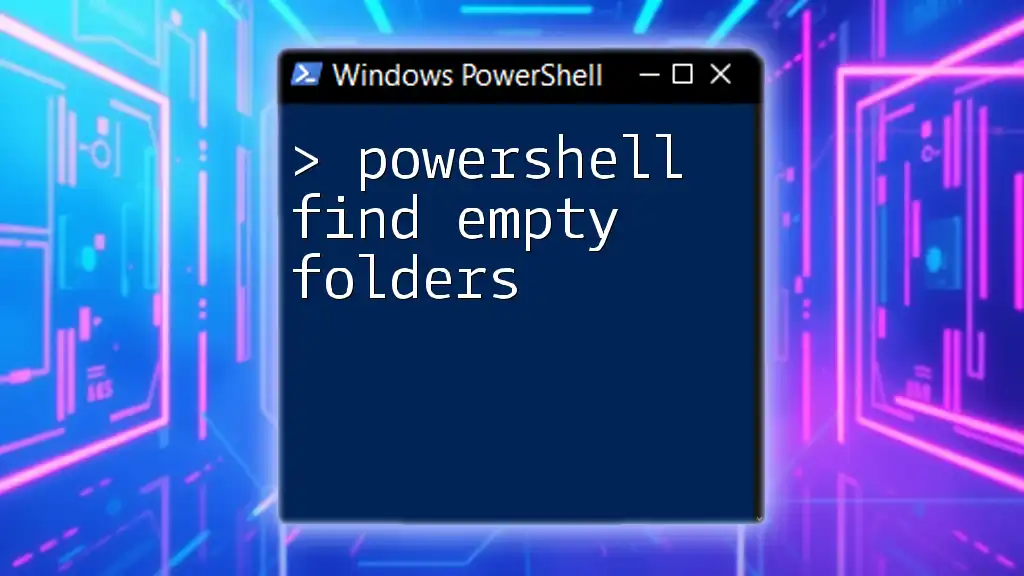
Performing a PowerShell Registry Search
PowerShell Search Registry for Value
Searching for a specific value in the registry can be done quickly using PowerShell. A common approach is to use the `Get-ItemProperty` cmdlet, which directly queries a specified registry key for a particular value.
For example, to search for the installation path of a commonly used application, you might use the following command:
$keyPath = "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion"
$valueName = "ProgramFilesDir"
$value = Get-ItemProperty -Path $keyPath -Name $valueName -ErrorAction SilentlyContinue
if ($value) {
Write-Output "Value found: $($value.$valueName)"
} else {
Write-Output "Key or value doesn't exist."
}
In this code, we define a key path and a value name, then check if the value exists. The `-ErrorAction SilentlyContinue` parameter ensures that no error messages are shown if the value doesn't exist, allowing a smooth user experience.
PowerShell Check Registry Key Value
To check if a registry key exists and retrieve its value, the above method works well. Checking registry values can be crucial for configuration management or troubleshooting tasks. Understanding whether a key or value exists allows you to take appropriate actions, such as creating new settings or restoring defaults.
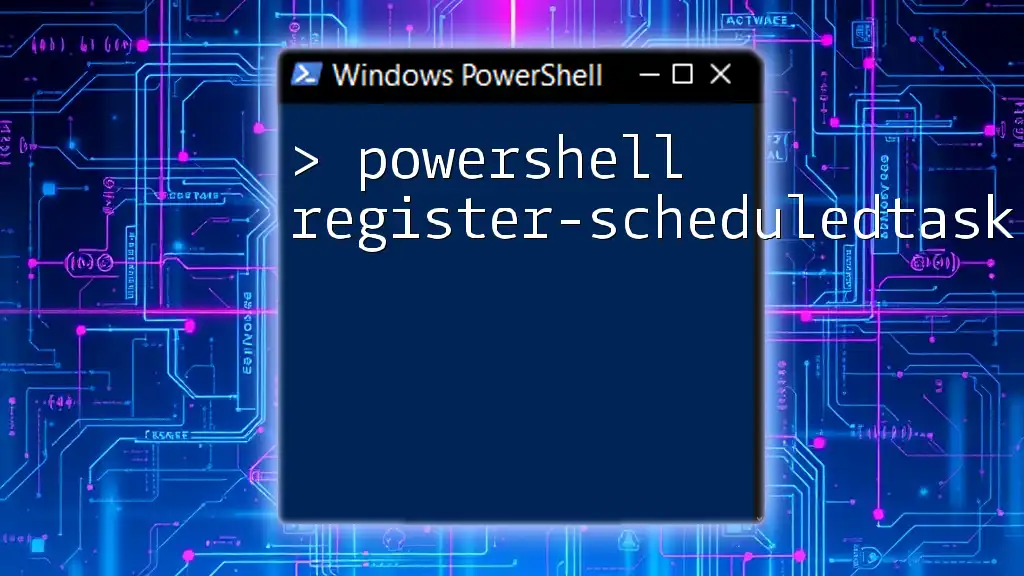
Advanced Registry Searches with PowerShell
PowerShell Registry Search Techniques
For more extensive searches, particularly when the exact location of a value is unknown, PowerShell’s capability to traverse subkeys becomes invaluable. The `Get-ChildItem` cmdlet can be leveraged to perform recursive searches across the entire registry.
Consider an example where we need to search through user-specific registry settings:
$searchPath = "HKCU:\"
$searchValue = "UserName"
Get-ChildItem -Path $searchPath -Recurse |
Where-Object { $_.GetValue($searchValue) -ne $null } |
ForEach-Object { Write-Output "Found in: $($_.PSPath), Value: $($_.GetValue($searchValue))" }
This command searches the current user registry (`HKCU:\`) for all subkeys and filters out those that contain a specified value (`UserName`). For every match, it outputs the path of the key along with its value. This technique can save time when dealing with a vast registry.
Filtering Search Results
To streamline the search process, you can employ `Where-Object` to apply additional filtering. For instance, if you're only interested in values of a particular data type or keys that contain specific text in their names, filtering can help you pinpoint the necessary entries quickly.
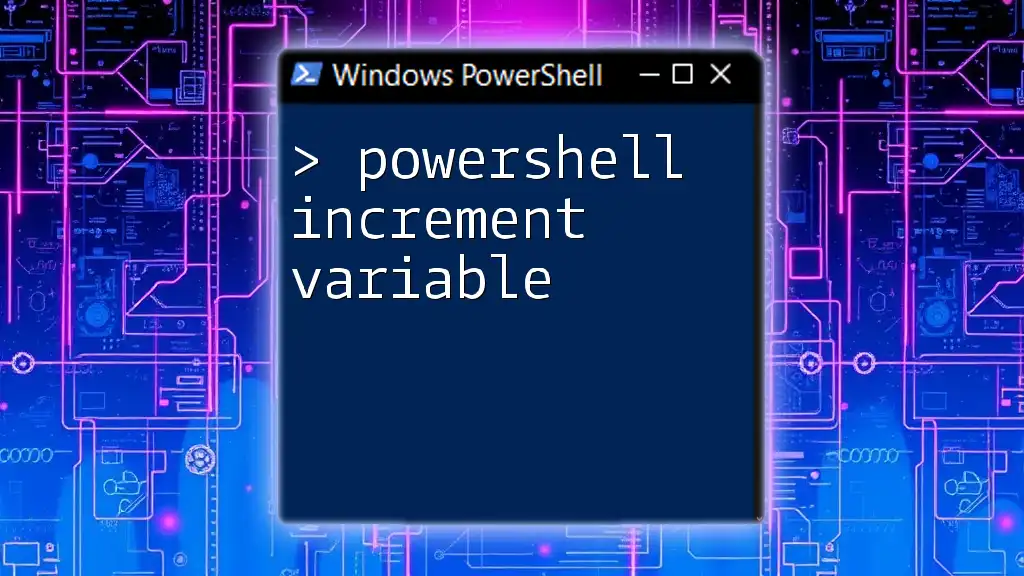
Common Use Cases for Finding Registry Values
Troubleshooting Applications
Registry searches can be particularly useful for troubleshooting applications. Many applications store critical configuration settings in the registry, such as paths to installation directories, settings for plugins, or activation keys.
For example, if an application is not starting correctly, you can check registry values to ensure that critical keys are present and correctly formatted.
System Settings and Configurations
Aside from applications, many system-level settings are stored in the registry. This includes configurations impacting system performance or user experience features. Searching for specific configurations can provide insights into potential issues or verify settings that are supposed to be applied.
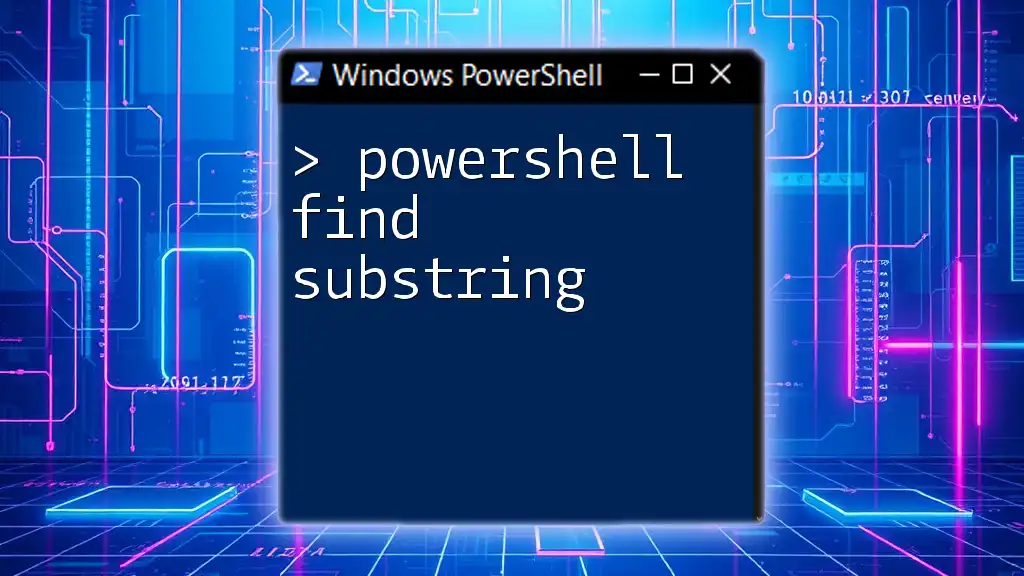
Tips for Efficient Registry Management
Best Practices
When working with the Windows Registry, safety should always be a priority. Always back up your registry before making any modifications. You can do this using the built-in regedit tool or by exporting specific keys. Use PowerShell to automate the backup process by creating script files that export necessary keys.
Troubleshooting Common Issues
While working in the registry, you may encounter various common issues, such as permissions-related errors or missing keys. Always ensure that PowerShell is running in a context that has the necessary permissions to access and modify the registry. For missing keys, consider whether the application is correctly installed or if it needs reinstallation.
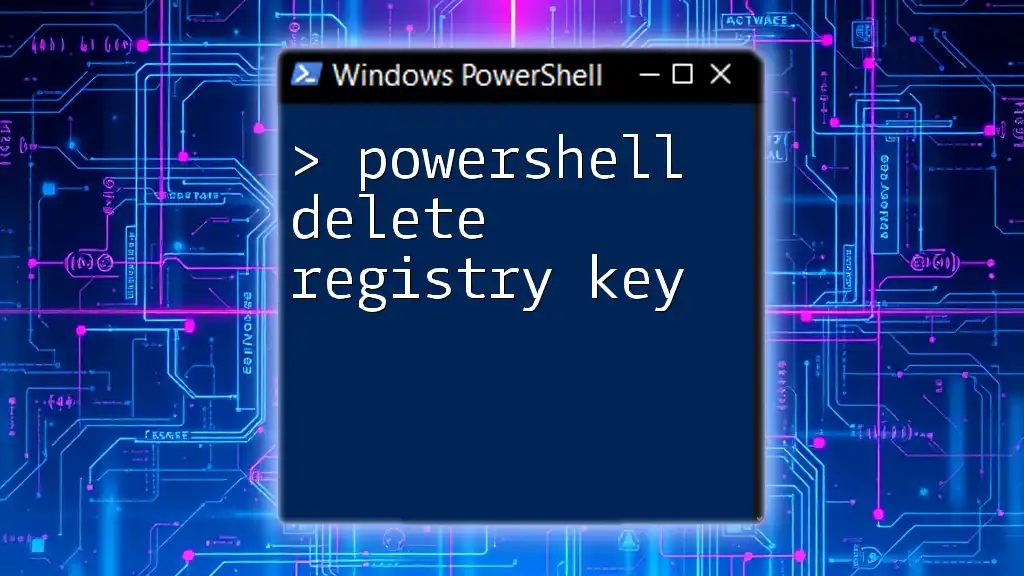
Conclusion
Understanding how to efficiently find registry values using PowerShell can significantly enhance your ability to manage system settings and troubleshoot application issues. By applying the techniques discussed, you can confidently navigate the registry, ensuring that you access and modify values safely.
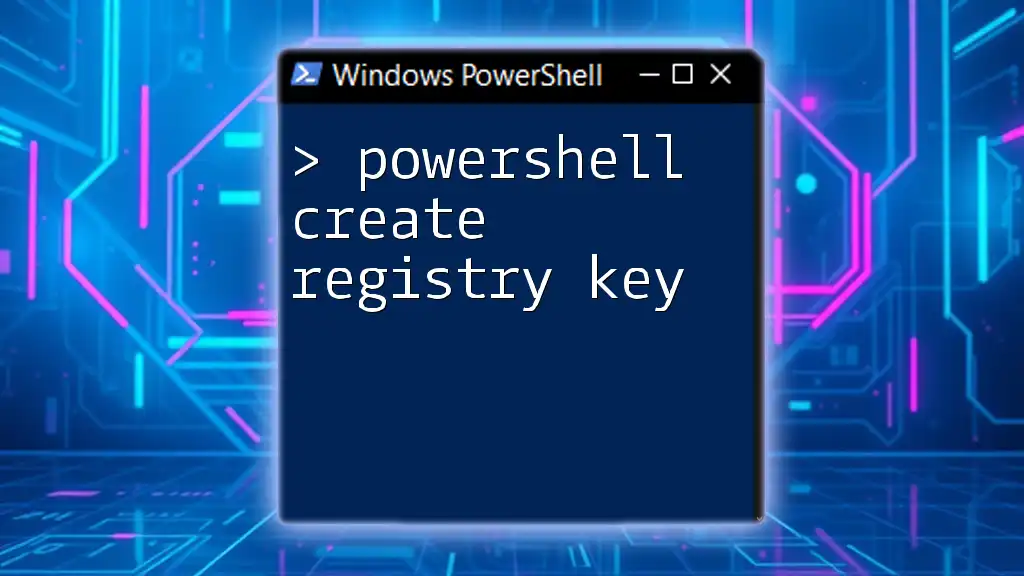
Additional Resources
Useful Cmdlets Reference
- Get-Item: Retrieve specific registry keys.
- Get-ChildItem: List subkeys and values.
- Get-ItemProperty: Fetch values and properties associated with keys.
Further Reading and Tutorials
Explore more advanced topics and tutorials to deepen your understanding of PowerShell, including scripting techniques, command automation, and further registry management.
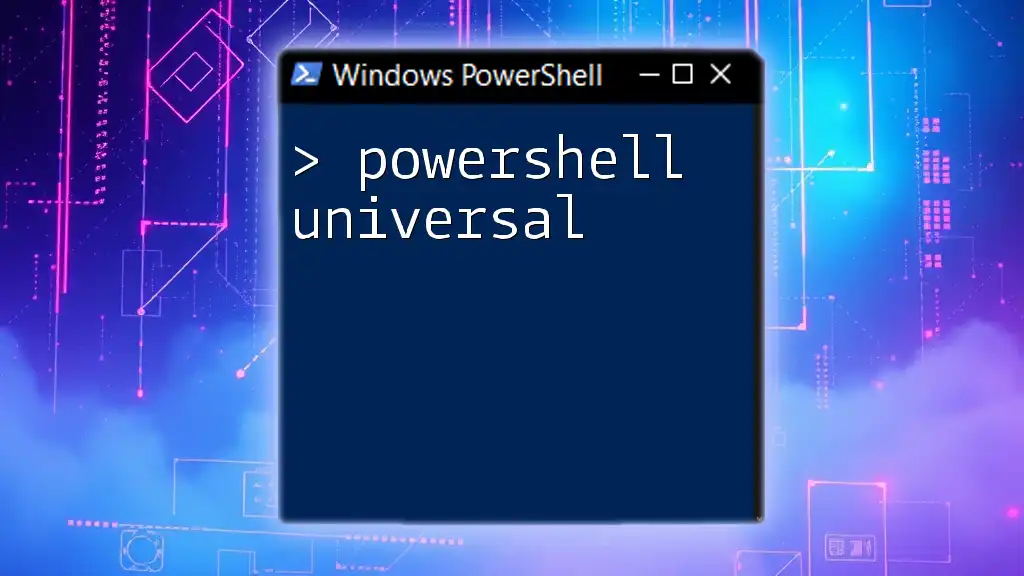
Call to Action
Consider participating in workshops or online courses to expand your knowledge of PowerShell and improve your automation skills. Join a community of enthusiasts, and start debugging and managing your settings like a pro!