In PowerShell, you can find a specific string within a variable using the `-like` operator or the `Contains()` method, as shown in the code snippet below.
$myString = "Hello, PowerShell World!"
if ($myString -like "*PowerShell*") { Write-Host "String found!" }
Understanding Strings in PowerShell
What is a String?
A string in programming represents a sequence of characters. In PowerShell, strings can be enclosed in either single quotes (`'`) or double quotes (`"`), with each type having different implications for how variables inside them are treated.
For example:
$singleQuoted = 'This is a single quoted string'
$doubleQuoted = "This string has a variable: $singleQuoted"
Here, `$doubleQuoted` will evaluate to include the content of the `$singleQuoted` variable, while `$singleQuoted` will remain unchanged.
Variables and Strings
In PowerShell, variables are defined with a dollar sign (`$`) followed by the variable name. You can assign strings to these variables as follows:
$message = "Hello, PowerShell!"
Once assigned, you can manipulate or search these strings using various methods.
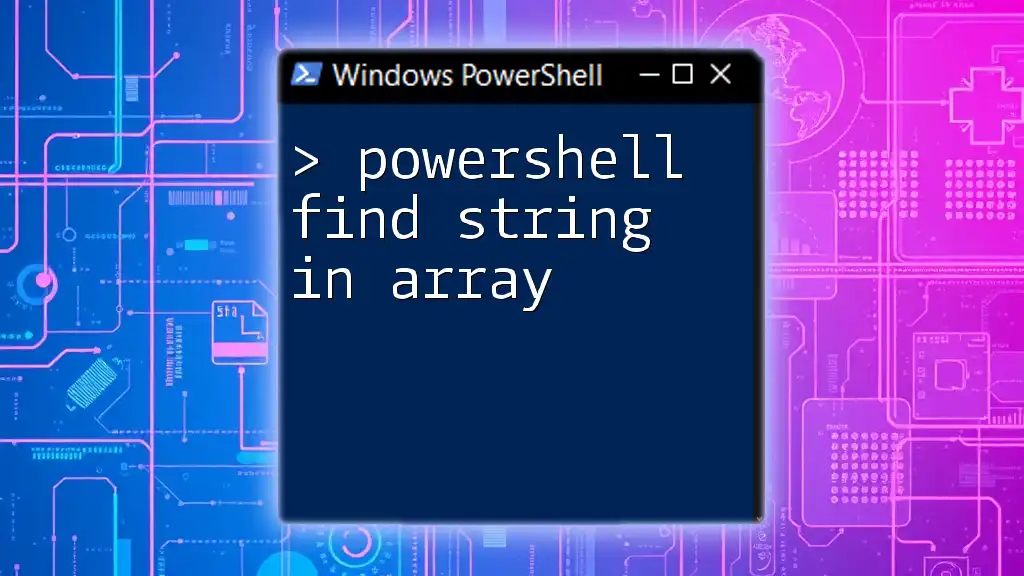
Finding Strings in Variables
Using the `-like` Operator
The `-like` operator allows for pattern matching with the use of wildcard characters (`*` for any sequence of characters and `?` for a single character). This tool is particularly handy when you need to check if a string contains a specific substring.
Example Code Snippet:
$myString = "Hello PowerShell World"
if ($myString -like "*PowerShell*") {
Write-Host "String matched!"
}
In this example, the output will be "String matched!" because “PowerShell” is indeed part of `$myString`.
Using the `-match` Operator
The `-match` operator uses regular expressions, making it a more powerful option for searches that require more complexity, such as partial matches or specific patterns.
Example Code Snippet:
$myString = "Learning PowerShell is fun!"
if ($myString -match "PowerShell") {
Write-Host "Match found!"
}
In this scenario, "Match found!" will be printed as “PowerShell” is part of the string.
Using the `.Contains()` Method
Another effective method to find a substring within a string is the `.Contains()` method, which is straightforward and case-sensitive.
Example Code Snippet:
$myString = "PowerShell is powerful!"
if ($myString.Contains("powerful")) {
Write-Host "The word 'powerful' is in the string."
}
Here, "The word 'powerful' is in the string." appears in the console because the substring exists within `$myString`.
Using the `IndexOf()` Method
The `IndexOf()` method is useful for not only finding if a substring exists but also for determining its position within the string.
Example Code Snippet:
$myString = "PowerShell commands are great!"
$index = $myString.IndexOf("commands")
if ($index -ge 0) {
Write-Host "Found 'commands' at index $index."
}
This code will output "Found 'commands' at index 15." indicating where the word begins in the main string.
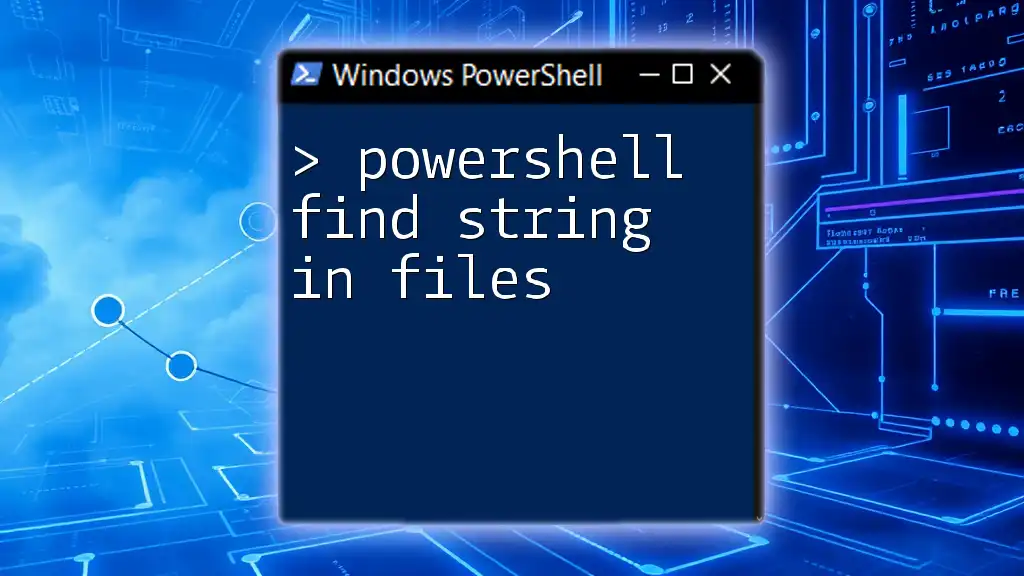
Case Sensitivity Considerations
Understanding Case Sensitivity in PowerShell
PowerShell string searches are case-insensitive by default. This means that searching for "pOwErShElL" would yield the same results as searching for "PowerShell". However, this could lead to unexpected results in some cases where the case matters.
Using the `-cmatch` and `-clike` Operators
To perform case-sensitive matches, PowerShell provides the `-cmatch` and `-clike` operators.
Example Code Snippet:
$myString = "PowerShell"
if ($myString -cmatch "powershell") {
Write-Host "This will not match because of case sensitivity."
}
In this case, no output will be generated as the case does not match; nothing will be printed.
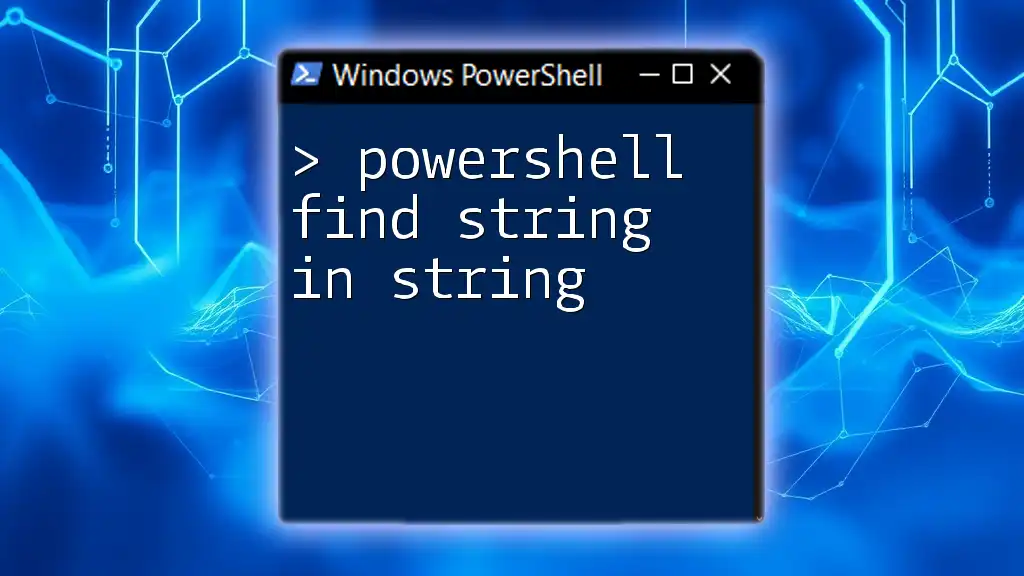
Combining String Searching Techniques
Complex String Searches
In many situations, you might need to combine search techniques. You can create logical conditions that allow for more nuanced searches.
Example Code Snippet:
$myString = "PowerShell is a robust shell!"
if ($myString -like "*Power*Shell*" -and $myString -match "robust") {
Write-Host "Both conditions met!"
}
Here, "Both conditions met!" will be displayed since both conditions evaluate to true based on the content of `$myString`.
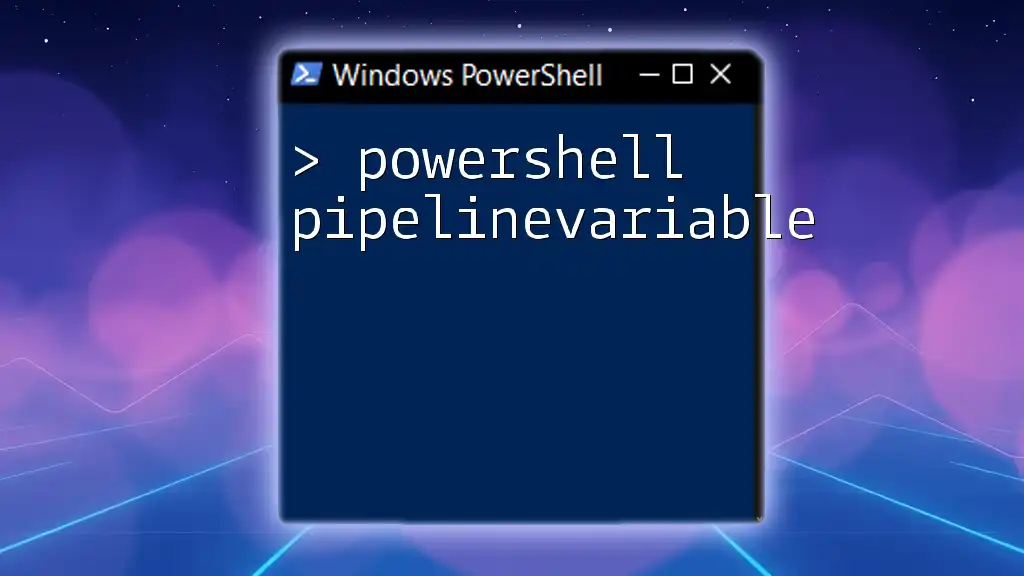
Performance Considerations
When to Use Which Method
Understanding which method to employ depends on your needs. For simple checks, `-like` is effective. If you require regular expressions, use `-match`. Methods like `.Contains()` and `IndexOf()` offer clear advantages for performance when you want straightforward substring checks.
Common Mistakes to Avoid
Be cautious of common pitfalls, such as case sensitivity and assuming all operators behave similarly. Testing your searches in different contexts can save you from frustration later in your scripting efforts.
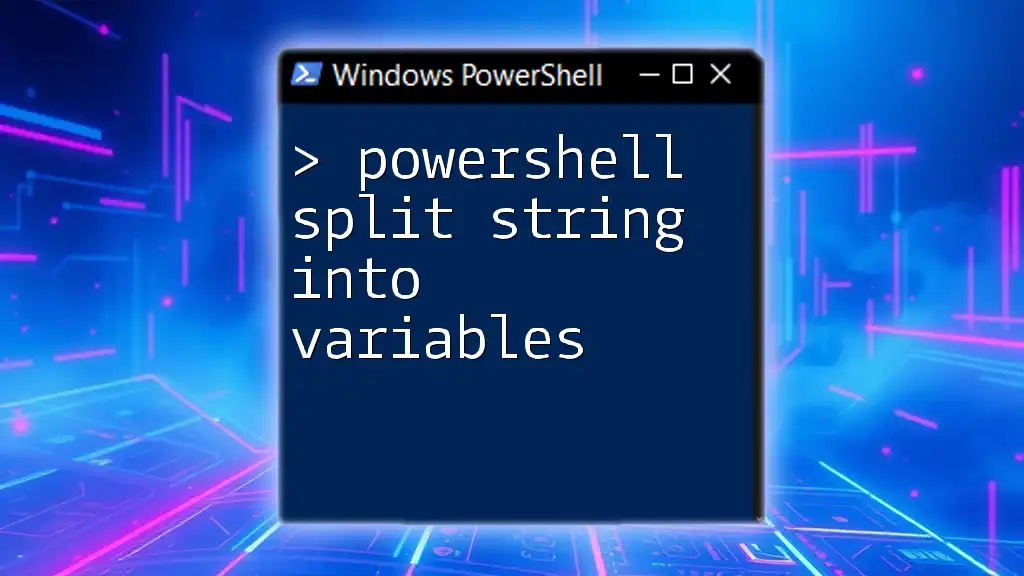
Conclusion
In summary, knowing how to find strings in variables using PowerShell is essential for any user looking to manipulate data effectively. With various methods available—from `-like` to `.Contains()`—you can confidently handle string searches in your PowerShell scripts. Happy scripting!
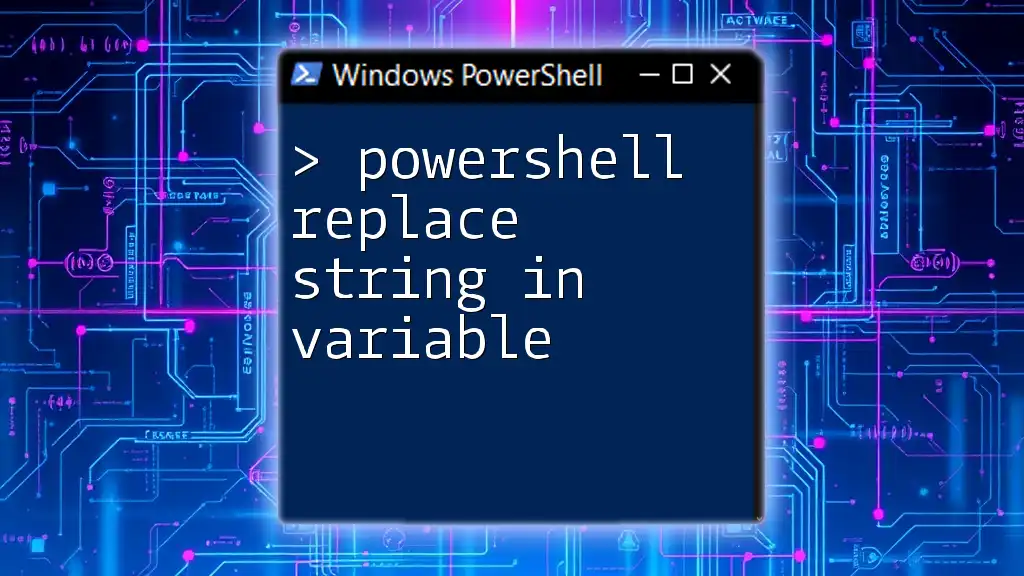
FAQs
Frequently Asked Questions about Finding Strings in PowerShell
- What happens if a substring isn't found? Using methods like `IndexOf()` will return -1 whereas `Contains()` will return false.
- Are PowerShell string searches case-sensitive? By default, they are not; use `-cmatch` or `-clike` for case-sensitive searches.
- How can I search for multiple substrings? You can combine logical operators with the aforementioned methods to check for multiple conditions.