In PowerShell, you can find a specific string in an array by using the `-contains` operator or the `Where-Object` cmdlet to filter the array effectively.
Here’s a code snippet demonstrating how to find a string in an array:
$array = @('apple', 'banana', 'cherry')
$searchString = 'banana'
if ($array -contains $searchString) {
Write-Host "$searchString found in array."
} else {
Write-Host "$searchString not found in array."
}
Understanding Arrays in PowerShell
What is an Array?
An array in programming is a data structure that can store a collection of items. In PowerShell, an array is particularly flexible and can hold different types of data, including numbers, strings, and even other arrays.
Creating a simple array is straightforward. Below is an example:
$fruits = @("apple", "banana", "cherry")
Write-Host $fruits # Output: apple banana cherry
Array Syntax and Operations
The syntax for creating arrays in PowerShell involves using the `@()` notation. You can include elements separated by commas. Here are some common operations you can perform on arrays:
-
Accessing Array Elements: You can access an array element by its index, which starts at zero.
$firstFruit = $fruits[0] # Output: apple
-
Modifying Array Elements: Arrays in PowerShell can be modified easily.
$fruits[1] = "blueberry" Write-Host $fruits # Output: apple blueberry cherry
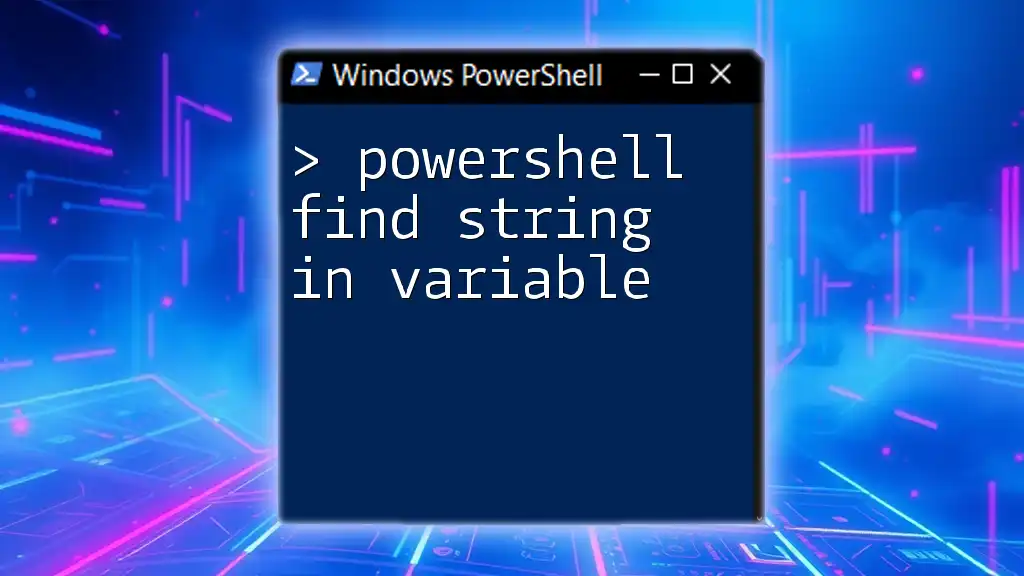
Finding a String in an Array
Why Search for Strings in an Array?
Searching for strings in an array is a common operation in PowerShell scripting. Scenarios where string searches are relevant include:
- Data validation: Ensuring input strings match predefined criteria.
- Configuration checks: Verifying if certain settings exist within a list of options.
- Log analysis: Identifying errors or specific events in log files represented as arrays.
Using the `-contains` Operator
One of the simplest ways to check if an array contains a string is by using the `-contains` operator. This operator returns a Boolean value indicating whether the specified string is present in the array.
Here’s an example:
$fruits = @("apple", "banana", "cherry")
$containsBanana = $fruits -contains "banana" # Returns True
Write-Host $containsBanana # Output: True
Using the `Where-Object` Cmdlet
For more complex searches, the `Where-Object` cmdlet is a powerful tool. It allows you to filter objects based on specific conditions, making it ideal for searching through arrays.
For instance:
$colors = @("red", "blue", "green", "yellow")
$matchingColors = $colors | Where-Object { $_ -like "*e*" }
Write-Host $matchingColors # Output: blue green yellow
In this example, `Where-Object` filters the colors containing the letter "e".
String Comparison with `-match` and `-like`
PowerShell provides different operators to compare strings in arrays, such as `-match` and `-like`.
- `-match` is used for regular expression matching.
- `-like` is used for wildcard pattern matching.
Here is an illustration of both:
$names = @("Alice", "Bob", "Alice Johnson")
$matchedNames = $names | Where-Object { $_ -match "Alice" } # using -match
$matchedNamesLike = $names | Where-Object { $_ -like "A*" } # using -like
Write-Host $matchedNames # Output: Alice, Alice Johnson
Write-Host $matchedNamesLike # Output: Alice, Alice Johnson
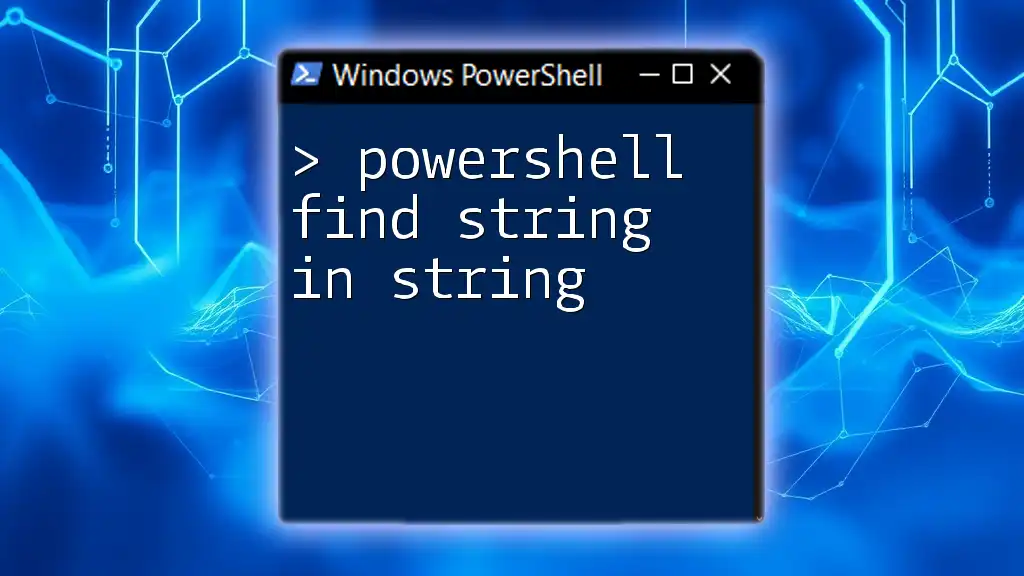
Advanced Methods for String Search
Using `Select-String`
The `Select-String` cmdlet is exceptionally useful for searching string patterns in input data. While it's commonly used to search content within files, it can also be leveraged to search through string arrays.
For example:
$quotes = @("To be, or not to be", "That is the question", "The only thing we have to fear is fear itself")
$foundQuotes = $quotes | Select-String -Pattern "fear"
Write-Host $foundQuotes
In this case, `Select-String` searches for the word "fear" across all the strings in the `$quotes` array.
Searching with Regular Expressions
Using regular expressions with PowerShell can enhance your ability to search arrays effectively. This is particularly useful when you want to apply complex search criteria.
To demonstrate:
$logMessages = @("Error: File not found", "Warning: Disk space low", "Error: Access denied")
$errorMessages = $logMessages | Where-Object { $_ -match "Error" }
Write-Host $errorMessages # Output: Error: File not found, Error: Access denied
Here, any log message containing the word "Error" is filtered successfully.
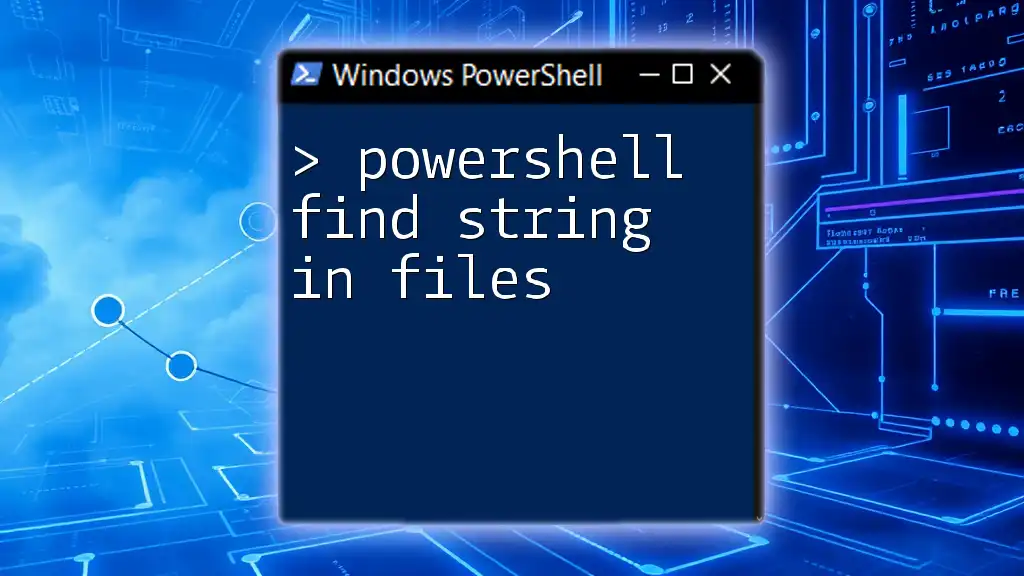
Tips and Best Practices
Performance Considerations
When working with larger arrays, it's essential to consider performance implications. Each method of searching strings can have different performance characteristics. For instance, using `-contains` is generally more efficient for existence checks, whereas `Where-Object` is more flexible for conditions.
Error Handling
PowerShell scripts can encounter various errors, especially when searching arrays. Error handling should be part of your script. Using `try-catch` blocks can help manage exceptions effectively.
Example of error handling in action:
$fruits = @("apple", "banana", "cherry")
try {
$result = $fruits -contains "mango"
} catch {
Write-Host "An error occurred: $_"
}
In this snippet, if an error arises during the check, a descriptive message is displayed.
Debugging and Testing Searches
Debugging is crucial for any scripting process. To ensure your string searches work correctly, you can use `Write-Host` to output relevant information during execution.
Example debugging output while iterating through an array:
foreach ($fruit in $fruits) {
Write-Host "Checking: $fruit"
}
This approach helps you trace the flow and identify any potential issues.
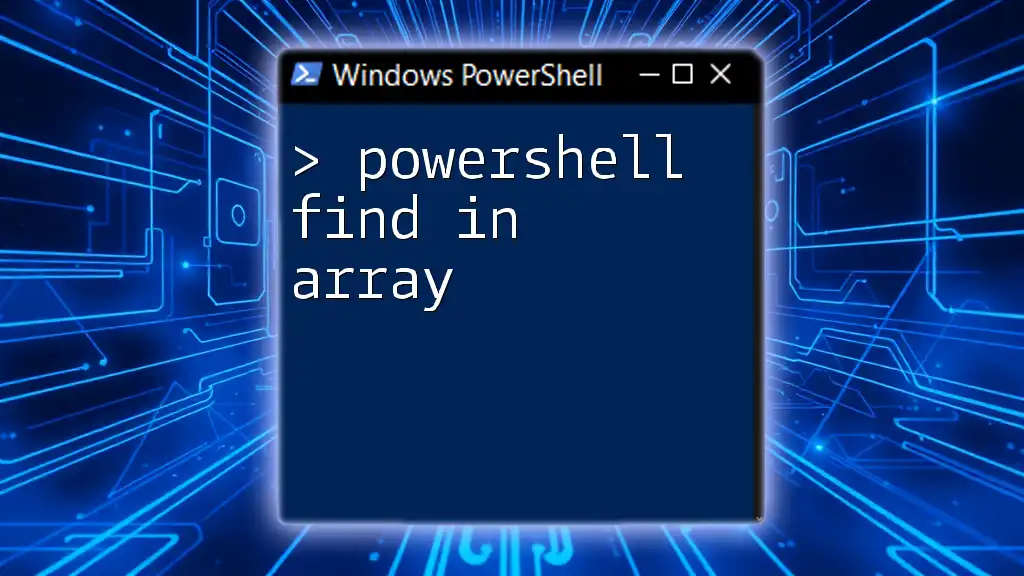
Conclusion
In summary, finding a string in an array using PowerShell can be accomplished through various methods, each suited for different scenarios. The techniques range from simple existence checks with `-contains` to more complex searches utilizing `Where-Object` and regular expressions.
Experimenting with the examples provided will enhance your understanding and proficiency in using PowerShell for string searches in arrays. Continuous exploration and practice are the keys to mastering these commands.