To split a string into an array in PowerShell, you can use the `-split` operator followed by the string you want to separate based on a specified delimiter. Here's a code snippet demonstrating this:
$array = "apple,banana,cherry" -split ","
Understanding Strings and Arrays in PowerShell
What is a String?
In PowerShell, a string is a sequence of characters surrounded by single or double quotes. Strings can hold various types of data, including text and numbers. For example:
$name = "John Doe"
$greeting = 'Hello, ' + $name
This creates a string "Hello, John Doe" by concatenating two string variables. Understanding how to manipulate strings is crucial as you often encounter scenarios where data needs to be formatted or parsed.
What is an Array?
An array is a data structure that holds a collection of items, which can be either strings or other types of data. In PowerShell, arrays are created using the `@()` syntax. For example:
$fruits = @("apple", "banana", "cherry")
Here, `$fruits` is an array consisting of three string elements. Arrays allow for efficient management and manipulation of grouped data.
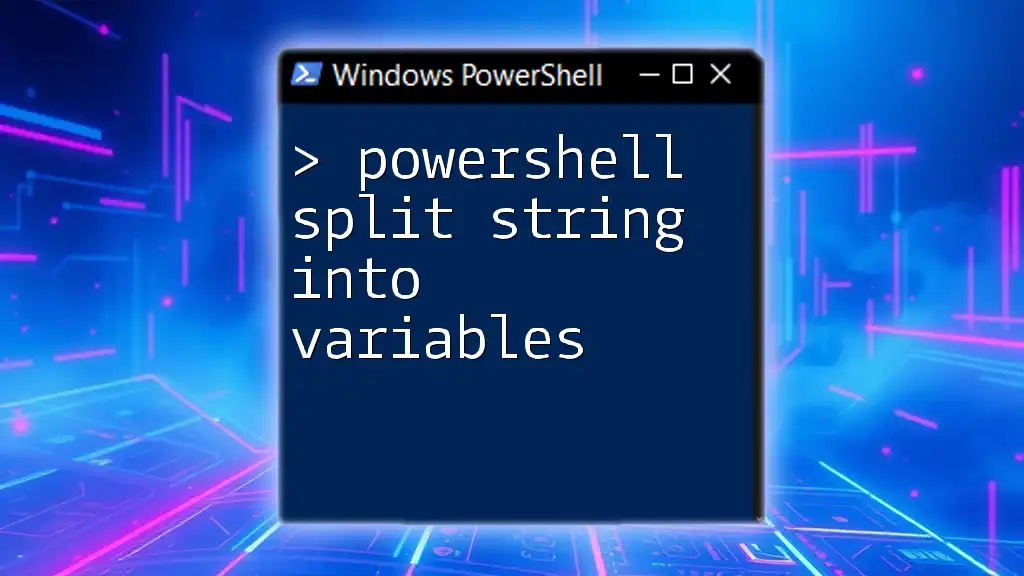
The Need to Split Strings in PowerShell
There are numerous scenarios where the need to split strings arises, particularly when working with structured data formats like CSV, logs, or user inputs. For instance, you may need to retrieve specific pieces of information from a larger text or format data for storage and analysis.
When strings can be divided into meaningful components—such as names, values, or sentences—the ability to split strings is a powerful tool for any PowerShell user.
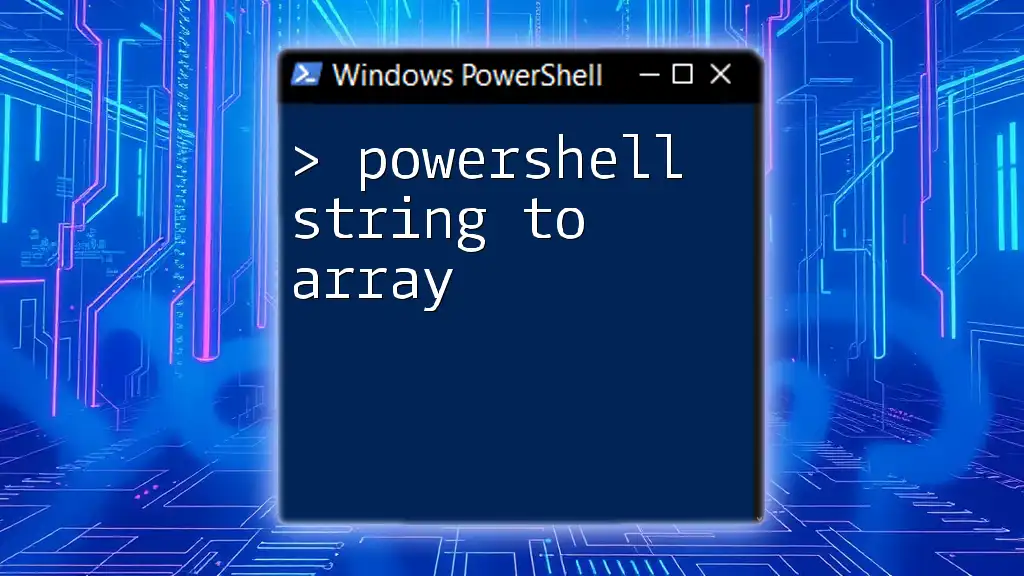
PowerShell Split String into Array
The Split Method
The primary method for splitting strings in PowerShell is the `Split()` method. This method allows you to define a delimiter that separates your strings. Here’s the syntax:
string.Split('Delimiter')
Example:
$string = "apple,banana,cherry"
$array = $string.Split(',')
In this example, the string `apple,banana,cherry` is split into an array `$array` that contains the elements `apple`, `banana`, and `cherry`. It’s worth noting that `Split()` can handle multiple delimiters.
Important to note: If the delimiter is not found within the string, the entire string remains intact as the only element in the resulting array.
Using Regular Expressions with Split
PowerShell also allows you to use regular expressions to split strings, which provides greater flexibility for more complex scenarios. The syntax for using regex is:
string -split 'Pattern'
Example:
$string = "apple;banana,cherry|date"
$array = $string -split '[;,|]'
In this example, various delimiters such as `;`, `,`, and `|` are used to split the string into an array. The resulting `$array` contains `apple`, `banana`, `cherry`, and `date`.
Using regular expressions is advantageous when dealing with unpredictable string patterns or multiple delimiters.
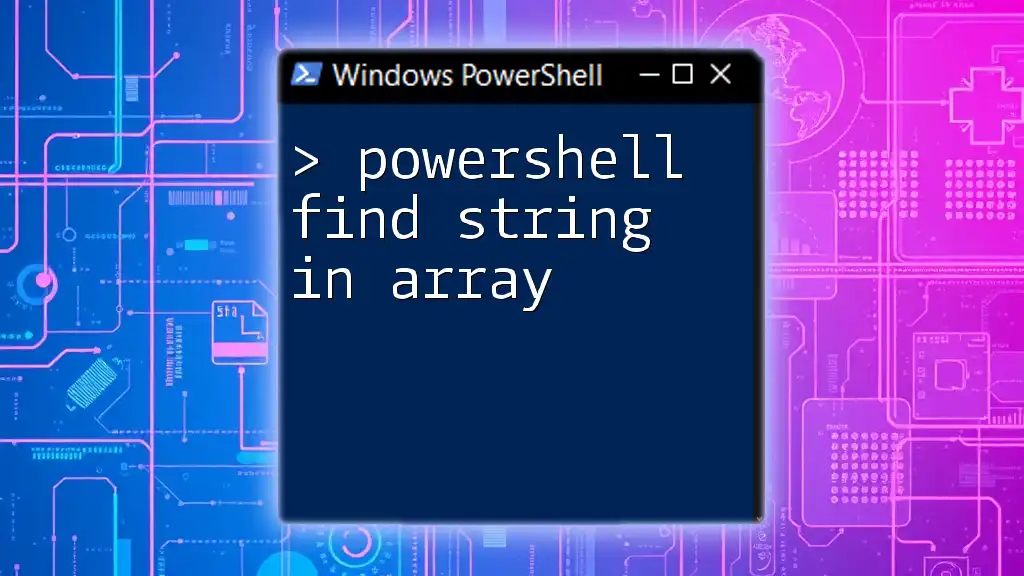
PowerShell Split Line into Array
Reading from Files
You often need to read lines from a file and split them into arrays for processing. For instance, if you have a file containing a list of names:
$lines = Get-Content "C:\path\to\file.txt"
foreach ($line in $lines) {
$array = $line -split ' '
# Process each word in $array as needed
}
Here, each line in the file is read, and every whitespace-separated word in that line is split into an array. This technique is commonly used for data processing in scripts.
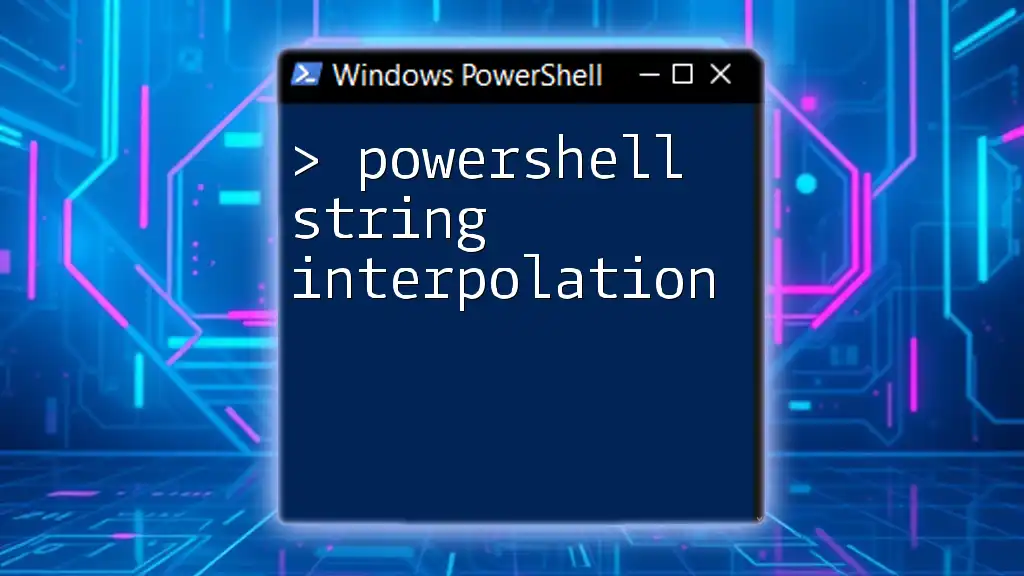
Handling Split Arrays
Common Issues When Splitting Strings
While splitting strings can be straightforward, you may encounter some challenges, such as:
- Dealing with Empty Entries: If your string has consecutive delimiters, you may end up with empty entries in your resulting array. You can use the `-replace` operator beforehand to clean up your string.
- Managing Escape Characters: Special characters can interfere with the split process. Ensure your regex patterns account for these special cases.
- Capturing Leading or Trailing Whitespace: Use the `.Trim()` method on your elements to remove any leading or trailing spaces after splitting.
Joining Arrays Back into Strings
Sometimes, after processing an array, you may want to reconstruct a string from it. The `Join()` method serves this purpose effectively.
Example:
$array = @("apple", "banana", "cherry")
$string = -join $array
This code will produce the string `applebananacherry`. If you want to include spaces or commas, you can specify a delimiter in the `Join()` method:
$string = -join ($array -replace '(.+)', '$1, ')
This technique is useful when you need to combine processed data back into a single string format.
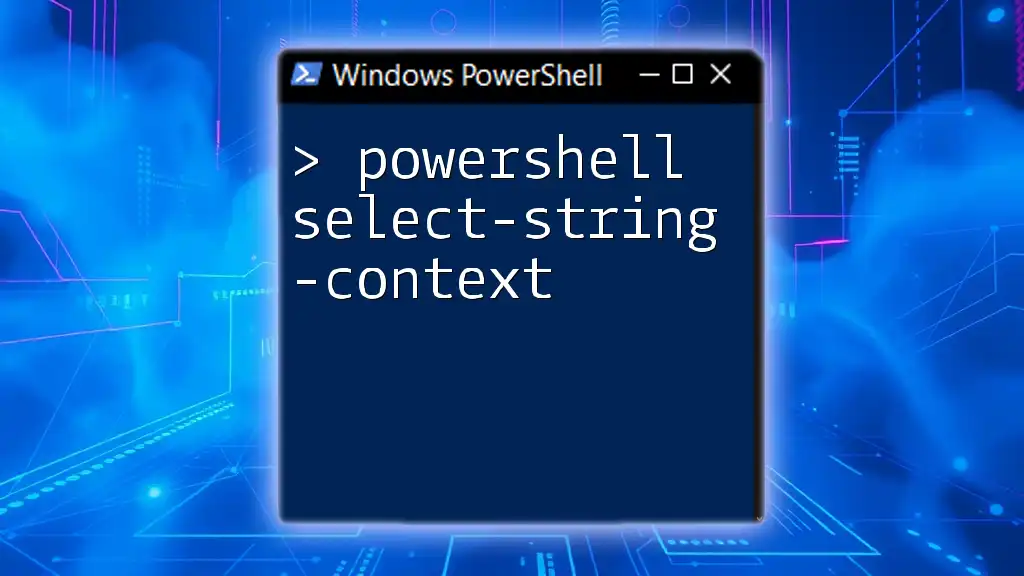
Practical Examples of Split Arrays
Example 1: CSV Data Processing
Often, data comes in the form of CSV strings that require splitting. Here’s how to parse a CSV string:
Example:
$csvString = "name,age,location"
$fields = $csvString -split ','
# Process fields as needed
In this scenario, `$fields` will contain an array of three elements: `name`, `age`, and `location`. This makes it easy to manipulate or extract distinct values from your data.
Example 2: Dynamic String Manipulation
PowerShell allows dynamic user input, making string manipulation essential in interactive scripts.
Example:
$input = Read-Host "Enter your colors separated by commas"
$colorArray = $input -split ','
Here, users can input a list of colors, which will then be converted into an array called `$colorArray`. This feature is immensely useful for applications that require user interaction and dynamic data handling.
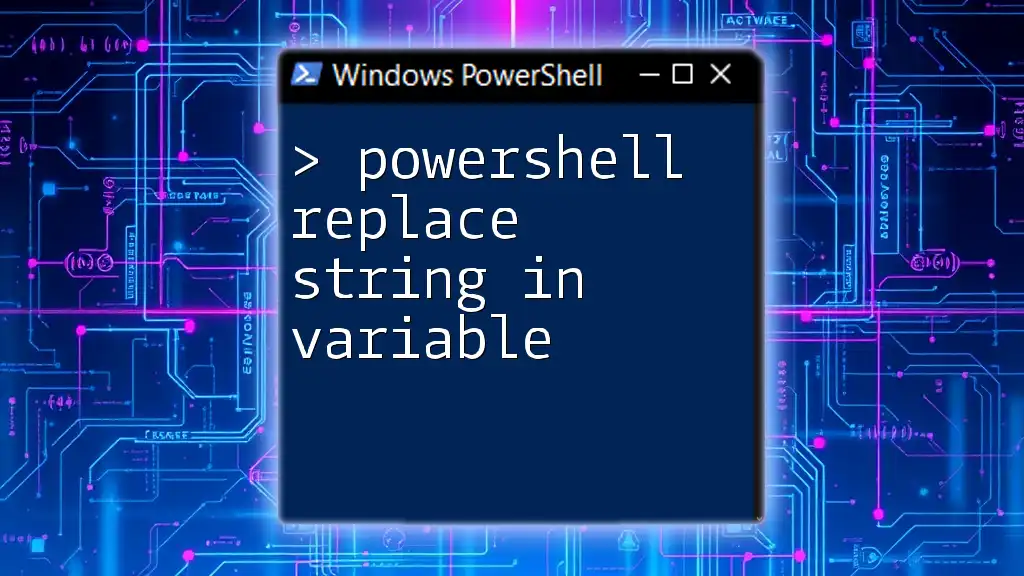
Conclusion
By mastering the use of PowerShell to split strings into arrays, you significantly enhance your scripting capabilities. This skill is practical across numerous real-world applications, enabling efficient methods for data handling, logging, and user input processing. Remember to practice these techniques regularly to become more proficient in PowerShell.
For further learning, consider joining online communities focused on PowerShell or exploring advanced resources that dive deeper into string manipulation and data processing techniques.
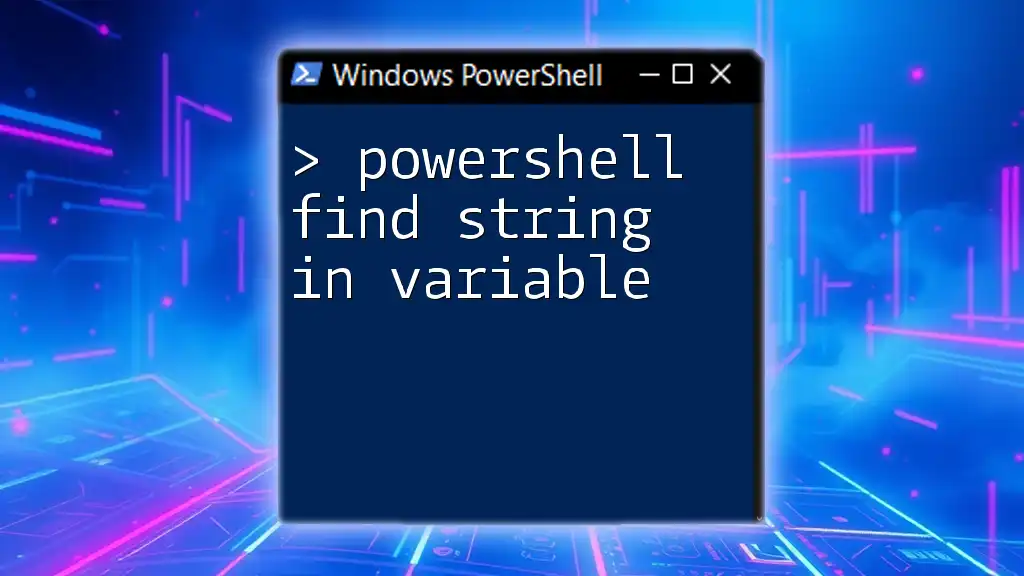
Additional Resources
To enhance your understanding and skills, refer to the official PowerShell documentation, which provides extensive resources and examples for every command and method discussed in this article. Recommended books and online courses can also supplement your learning experience, giving you an edge in mastering PowerShell scripting.
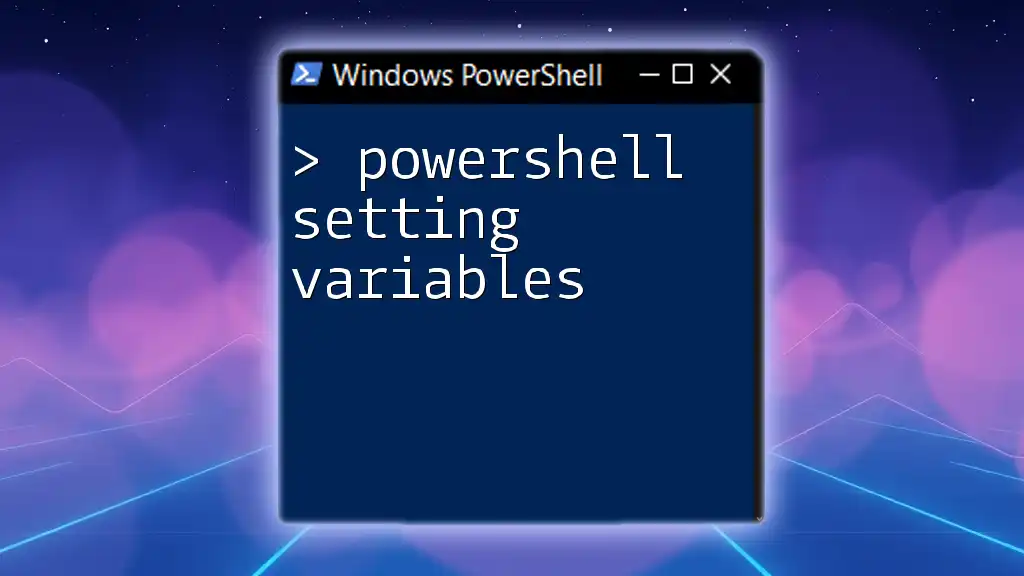
FAQs
If you have questions or encounter common issues related to the use of the `Split()` method or string manipulation, don't hesitate to seek out community forums or documentation for troubleshooting tips. Practice makes perfect!