To import a CSV file into an array using PowerShell, you can utilize the `Import-Csv` cmdlet to read the file and store its content in a variable.
Here’s a code snippet to demonstrate this:
$array = Import-Csv -Path 'C:\path\to\yourfile.csv'
Understanding CSV Files
What is a CSV File?
A CSV (Comma Separated Values) file is a simple text file that is commonly used for storing tabular data. Each line in the file corresponds to a row in the table, and each field in that row is separated by a specific delimiter, usually a comma. CSV files are widely used because they are easy to read and write, often serving as a standard for data exchange between different applications.
Structure of a CSV File
A typical CSV file consists of headers that define the fields followed by the data entries. For example, a CSV might look like this:
Name,Age,Occupation
John Doe,30,Software Engineer
Jane Smith,25,Data Analyst
This basic structure outlines three fields: Name, Age, and Occupation. Understanding how to work with this data is crucial for many scripting tasks.
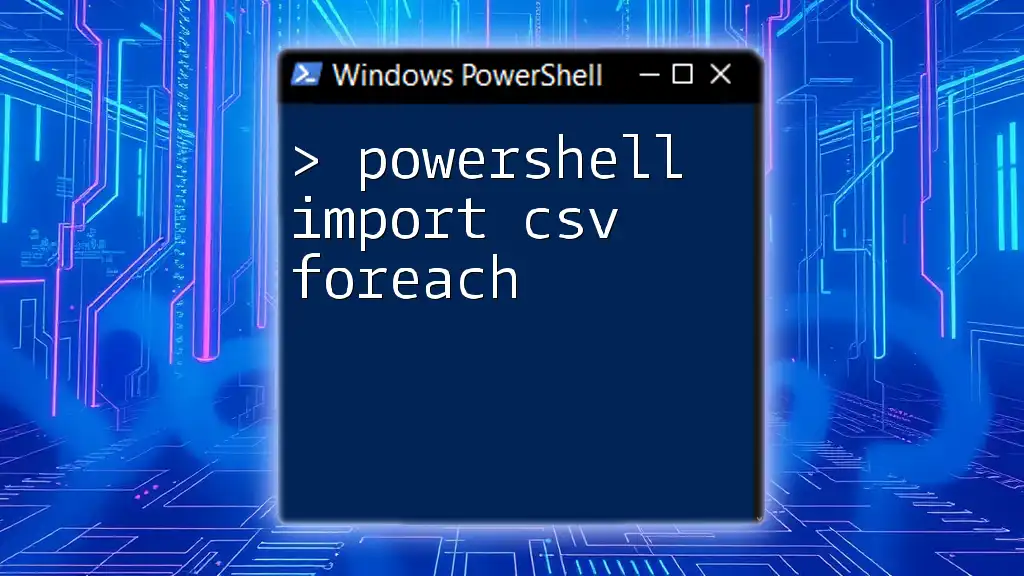
Getting Started with PowerShell
What is PowerShell?
PowerShell is a task automation framework from Microsoft, consisting of a command-line shell and associated scripting language. It allows administrators to automate system tasks, manage configurations, and control system resources with ease. PowerShell is particularly powerful because it enables users to interact with .NET objects, making it a favorite among system admins and automation enthusiasts alike.
Setting Up Your PowerShell Environment
To get started with PowerShell, simply open it on your Windows machine. You can launch it by searching “PowerShell” in the Start menu. Ensure that your version is up-to-date, as newer features might not be available in older versions.
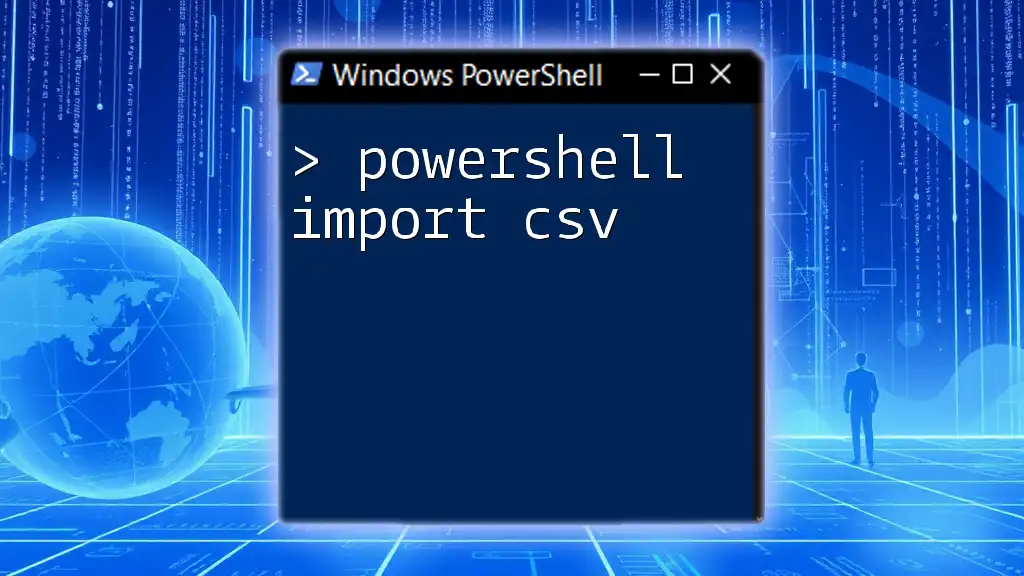
PowerShell Import CSV to Array
Why Use Arrays?
Arrays in programming are data structures that allow the storage of multiple items in a single variable. In this context, they are particularly useful when working with CSV files as they enable you to manage and manipulate data efficiently. Using arrays can dramatically simplify workflows, especially when handling larger datasets.
Basic Command to Import a CSV File into an Array
To begin importing your CSV data into a PowerShell array, you can use the following command:
$array = Import-Csv -Path "C:\path\to\yourfile.csv"
In this command:
- `Import-Csv` is the command that tells PowerShell to load CSV data.
- `-Path` is the parameter that specifies the location of the CSV file.
With this command, your CSV data is now stored in the `$array` variable, allowing for easy access and manipulation.
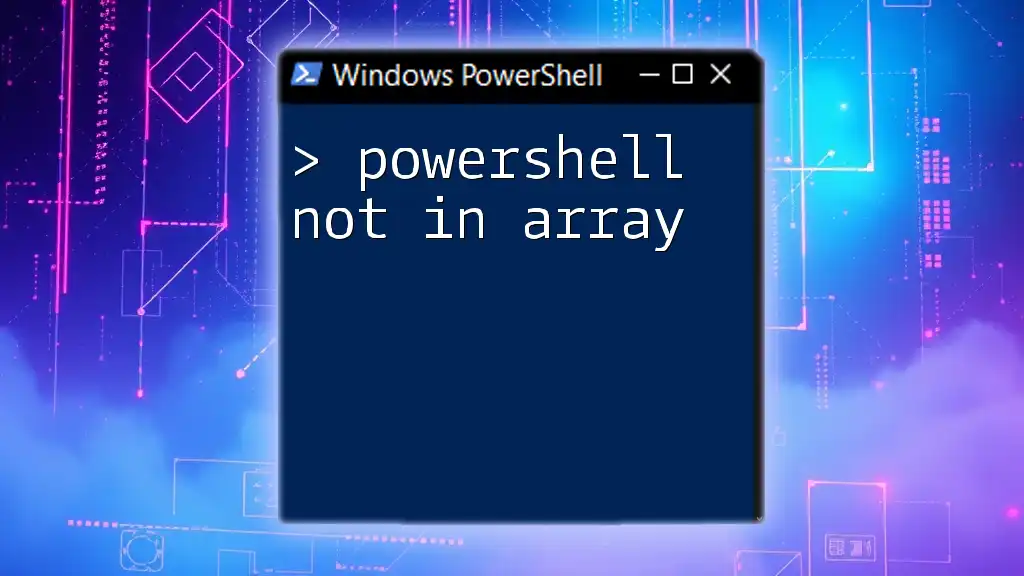
PowerShell Read CSV into Array
Reading Data from a CSV File
Once you have your CSV data imported into an array, accessing specific elements can be straightforward. Here’s how you can read and display a specific column's content:
$array | ForEach-Object { $_.ColumnName }
In this snippet, `ForEach-Object` iterates over each object (or row) in the array, allowing you to specify which column to display. Replace `ColumnName` with the actual name of the column you want to retrieve.
Accessing Array Elements
Each item stored in an array can be accessed through its index. For instance, if you want to access the first element of your array:
$firstElement = $array[0]
PowerShell arrays are zero-based, meaning the first element is at index 0. It’s essential to remember this as you navigate through your data.
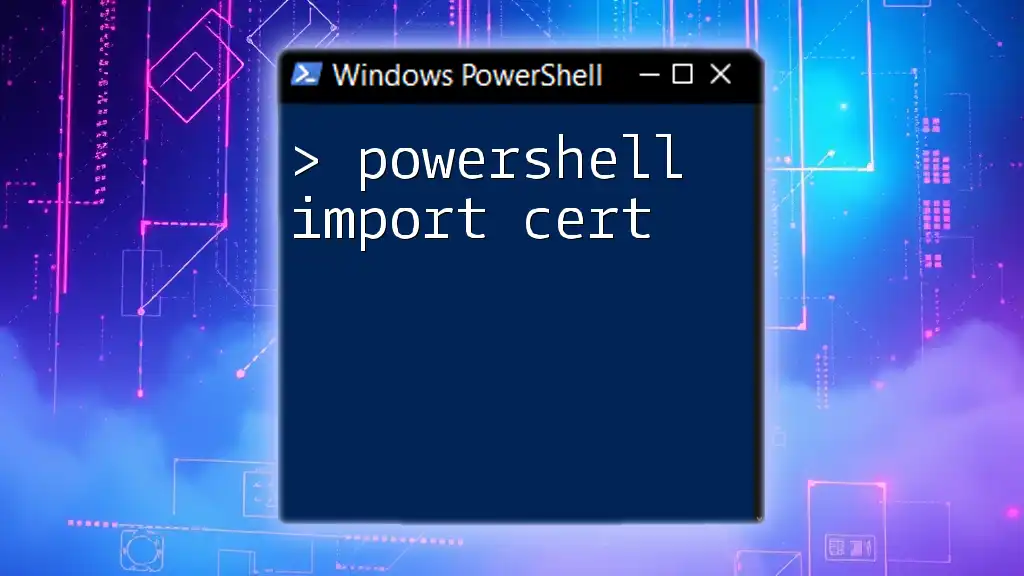
Advanced Techniques for Importing CSV as Array
Filtering Data During Import
Often, you may want to only work with a subset of your data right from the import stage. PowerShell allows for filtering data using the `Where-Object` cmdlet. For example:
$filteredData = $array | Where-Object { $_.ColumnName -eq "Value" }
This command filters the entries where the value of `ColumnName` matches `"Value"`. This technique can streamline your workflows by reducing the amount of data to process.
Transforming Data During Import
PowerShell also provides ways to transform data as it’s being imported. For example, using calculated properties can reshape your data:
$modifiedData = $array | Select-Object @{Name="NewName"; Expression={ $_.OldName }}
In this command, you create a new property called `NewName` that derives its value from `OldName`. This is handy for renaming fields or deriving new metrics on the fly.
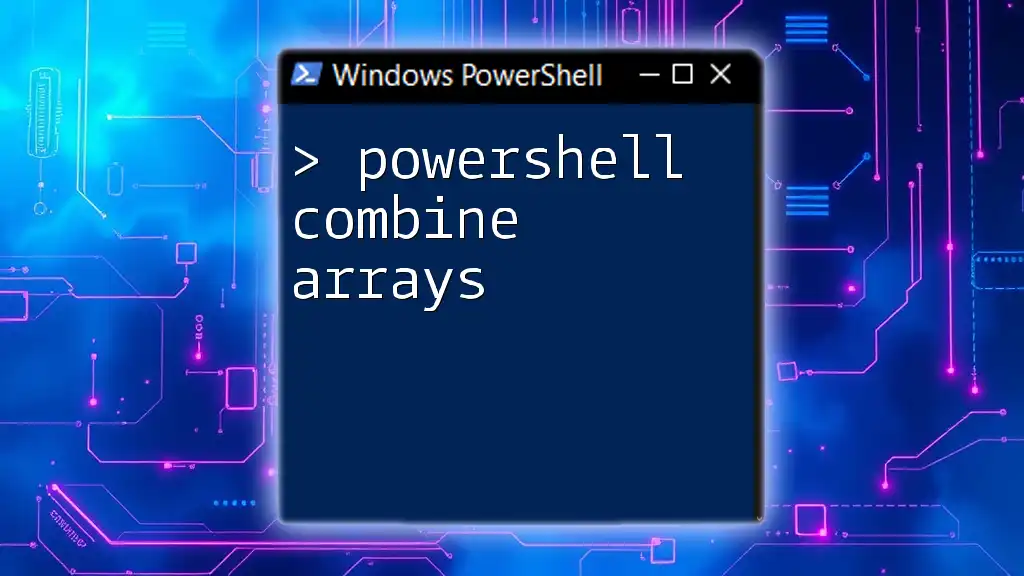
Common Use Cases for Importing CSV to Array
Data Analysis Tasks
Importing CSV data into arrays can assist significantly with performing data analysis. Once your data is neatly organized, you can apply statistical functions or generate summaries that aid in decision-making. PowerShell, combined with CSV, becomes an effective tool for reports and insights.
Automation of Administrative Tasks
Imagine a scenario where you’re responsible for managing user accounts in a system. By importing a CSV file detailing user information into an array, you can quickly create new accounts, modify user details, or even remove users in bulk:
$array | ForEach-Object {
New-ADUser -Name $_.Name -GivenName $_.FirstName -Surname $_.LastName -UserPrincipalName $_.Email
}
In this example, you leverage the array to automate user creation in Active Directory, showcasing how powerful this combination can be.
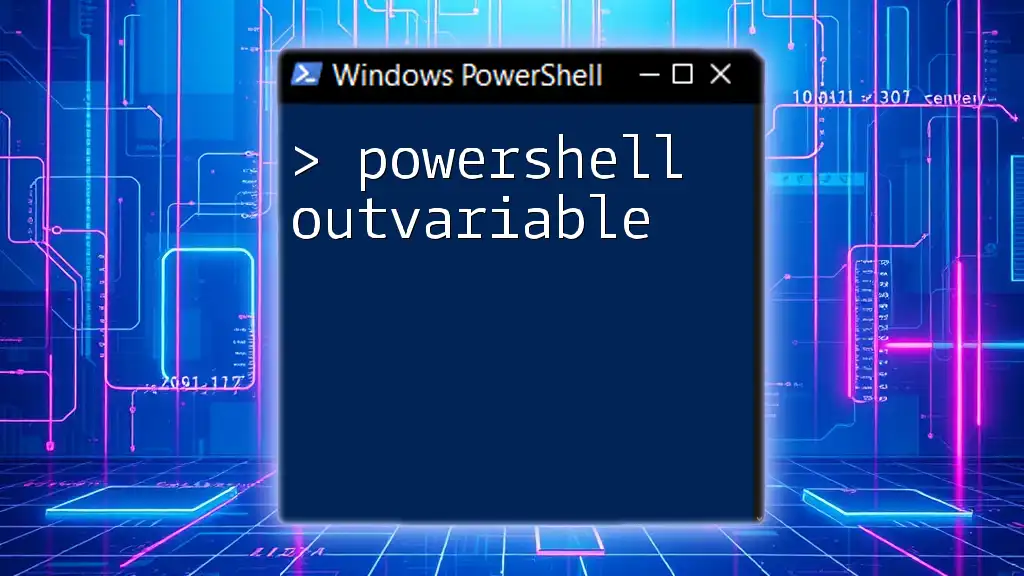
Troubleshooting Common Issues
Common Errors When Importing CSV
While working with CSV files in PowerShell, you may encounter a few common errors:
- File not found: Ensure the path you provided is correct; check for typos or invalid paths.
- Unexpected characters: Special characters in CSV files may cause import issues. Ensure your CSV is formatted correctly.
Best Practices for Working with CSV Files in PowerShell
To maintain data integrity and efficiency:
- Always validate your CSV files before importing them.
- Use quotes for paths containing spaces.
- Make use of error handling in your scripts to catch and address issues gracefully.
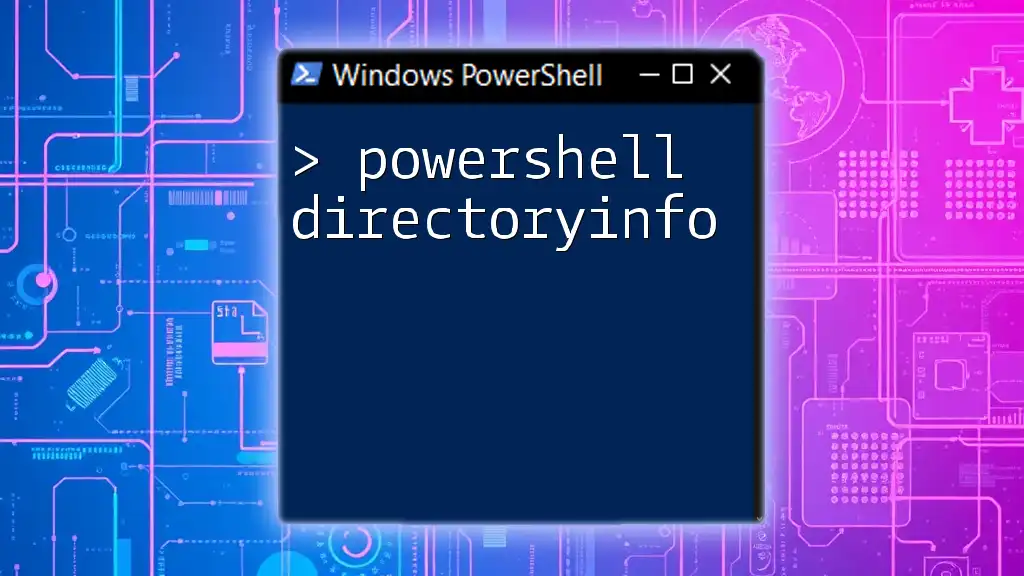
Conclusion
Understanding how to use PowerShell to import CSV into an array is invaluable for anyone looking to streamline data management and manipulation tasks. With the commands and techniques outlined in this article, you can harness the full potential of PowerShell and CSV files. Practice with various datasets to grow comfortable with these commands, and continue exploring the robust capabilities that PowerShell offers in automation and data analysis.
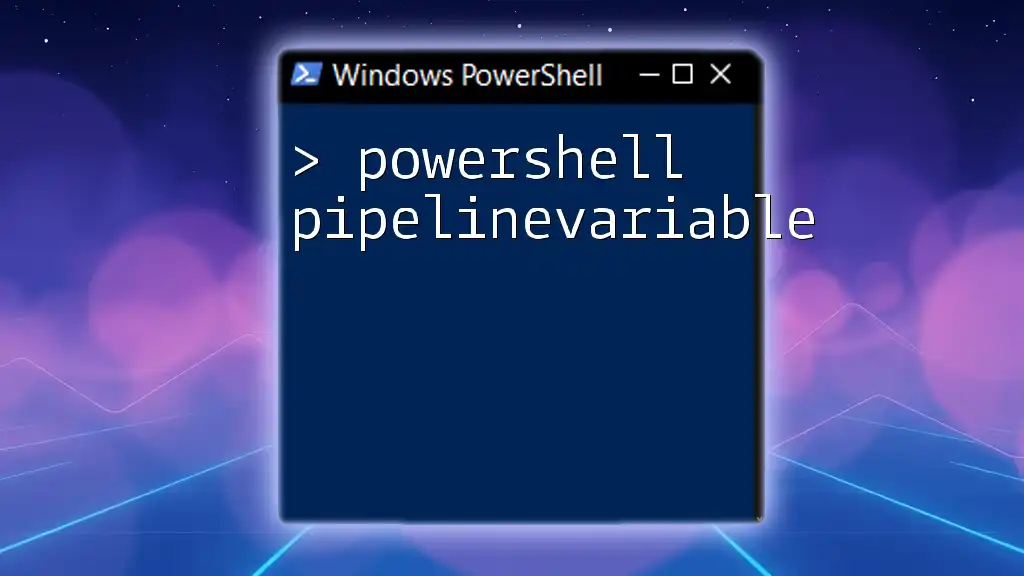
Additional Resources
For further exploration, consider reading the official PowerShell documentation or engaging with community forums where experienced users frequently share their knowledge and techniques. Practice scripts with sample CSV files to become proficient in these commands and broaden your PowerShell toolkit.