In PowerShell, you can read the contents of a file into an array by using the `Get-Content` cmdlet, which allows you to easily manipulate and access each line of the file as an individual element.
Here’s a code snippet demonstrating this:
$array = Get-Content 'C:\path\to\your\file.txt'
Understanding Arrays in PowerShell
What is an Array?
An array in PowerShell serves as a collection of items stored in a single variable. Arrays can hold multiple values at once, which makes them incredibly powerful for data handling and manipulation. By using arrays, you can easily manage lists of data without the need to declare each item separately.
Types of Arrays in PowerShell
PowerShell supports various types of arrays, each with unique use cases:
-
Single-dimensional arrays are the most straightforward type, allowing you to store a list of items sequentially. For example:
$singleArray = @("Apple", "Banana", "Cherry")
-
Multi-dimensional arrays enable the storage of data in a grid-like structure, perfect for scenarios that require rows and columns:
$multiArray = @(@("John", 25), @("Jane", 30))
-
ArrayLists offer greater flexibility, allowing dynamic resizing and more complex manipulations of collection elements. For instance:
$arrayList = New-Object System.Collections.ArrayList $arrayList.Add("Apple") $arrayList.Add("Banana")
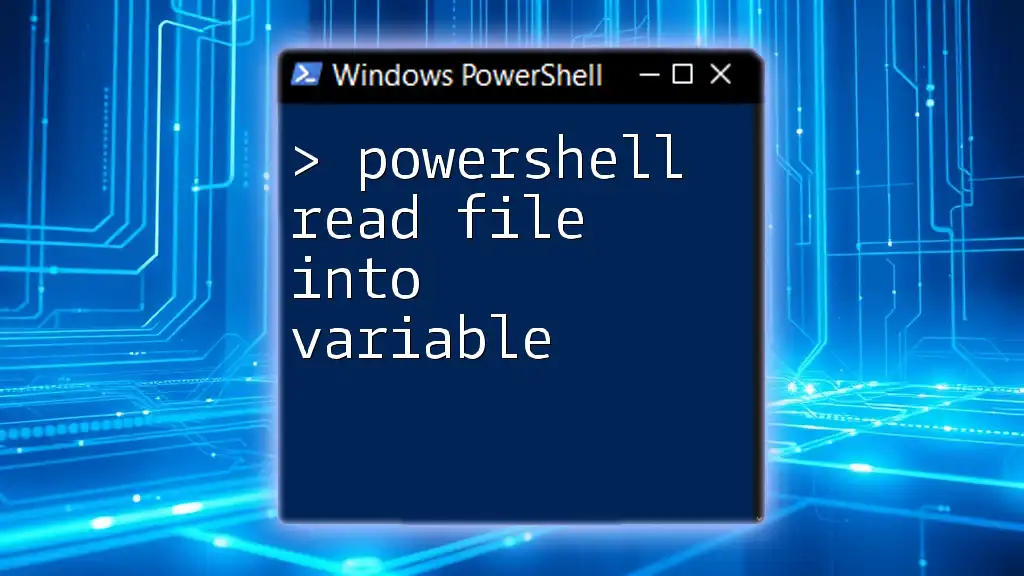
Reading a File into an Array
The Basics of File Handling in PowerShell
In PowerShell, the ability to read files is essential for data retrieval and automation tasks. Files can come in various formats, including plain text, CSV, and JSON. Understanding how to read these files into arrays simplifies data processing and makes your scripts more efficient.
Using `Get-Content` to Read Files
The `Get-Content` cmdlet is a primary tool for reading text files in PowerShell. Its straightforward syntax allows you to quickly load the contents of a file into an array.
For example, to read the contents of a text file:
$array = Get-Content -Path "C:\path\to\your\file.txt"
In this example, all the lines in `file.txt` will be stored as individual elements in the `$array` variable. Each line will represent a separate index.
Working with Different File Formats
Reading CSV Files
CSV files are a common format for data exchange, especially in analytical tasks. PowerShell's `Import-Csv` cmdlet simplifies reading CSV files and automatically structures the data into an array of objects for easy access.
For example:
$array = Import-Csv -Path "C:\path\to\your\file.csv"
This command imports the CSV file and treats the header line as property names for each object in the array, making it easy to refer to individual columns by name.
Reading JSON Files
JSON is another widely used format, especially in web applications. PowerShell allows you to read JSON files and convert them into PowerShell objects.
For instance:
$jsonData = Get-Content -Path "C:\path\to\your\file.json" | ConvertFrom-Json
$array = @($jsonData)
In this example, the file contents are first read and then converted from JSON format into PowerShell objects, which you can easily manipulate.
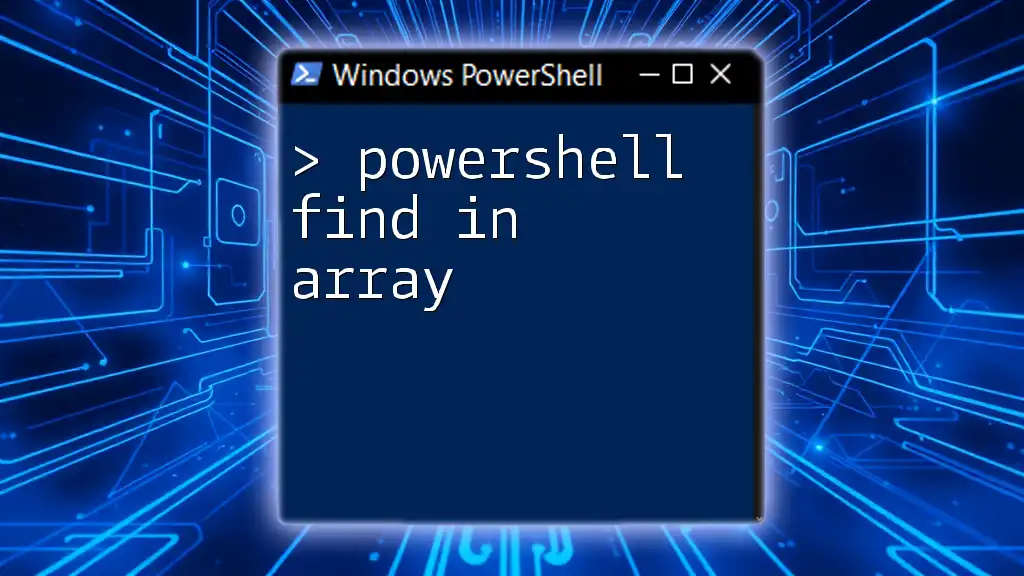
Manipulating Arrays After Reading
Accessing Array Elements
Once you have read a file into an array, accessing array elements becomes crucial. Each element is indexed, starting from zero. This allows you to retrieve specific data points efficiently.
For example:
$firstElement = $array[0]
This retrieves the first element from the array stored in `$firstElement`.
Looping Through an Array
Looping through an array is often necessary for processing each item iteratively. PowerShell makes this easy through the `foreach` loop.
Example:
foreach ($item in $array) {
Write-Output $item
}
This will print each item from the array one by one, allowing for further actions on each element if necessary.
Modifying Array Elements
Sometimes, you may need to change an array element based on new information or operations. This can be done directly by accessing the specific index.
Example:
$array[0] = "New Value"
This replaces the first element of the array with "New Value".
Adding and Removing Elements
Adding an Element
To add an element to an array, you can use the `+=` operator:
$array += "New Element"
This appends "New Element" to the end of the array.
Removing an Element
To remove an element, you might want to filter it out using the `Where-Object` cmdlet:
$array = $array | Where-Object { $_ -ne "Element to Remove" }
This command removes all elements matching "Element to Remove" from the array.
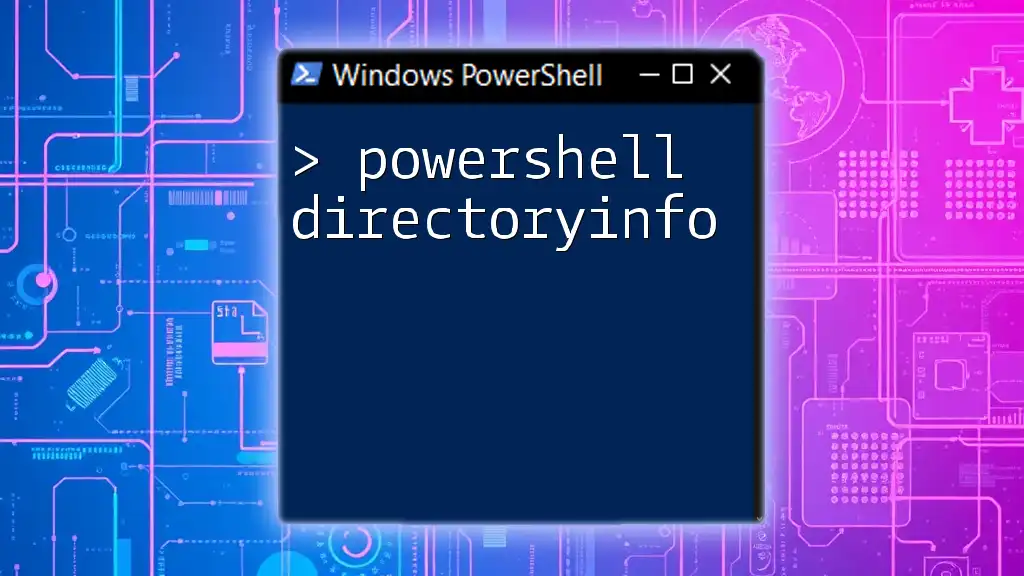
Best Practices for Reading Files into Arrays
Error Handling
Proper error handling is critical when reading files to ensure your script does not break unexpectedly. Using try-catch blocks around file reading operations is a good practice.
Example:
try {
$array = Get-Content -Path "C:\path\to\your\file.txt"
} catch {
Write-Error "Could not read the file: $_"
}
This catches errors while attempting to read the file and outputs a descriptive message.
Performance Considerations
While arrays are efficient, for very large datasets, consider performance implications. PowerShell provides options like `ArrayLists` for dynamic resizing or using `DataTables` for complex data manipulations. Optimize your scripts according to the specific needs of your tasks to maintain performance.
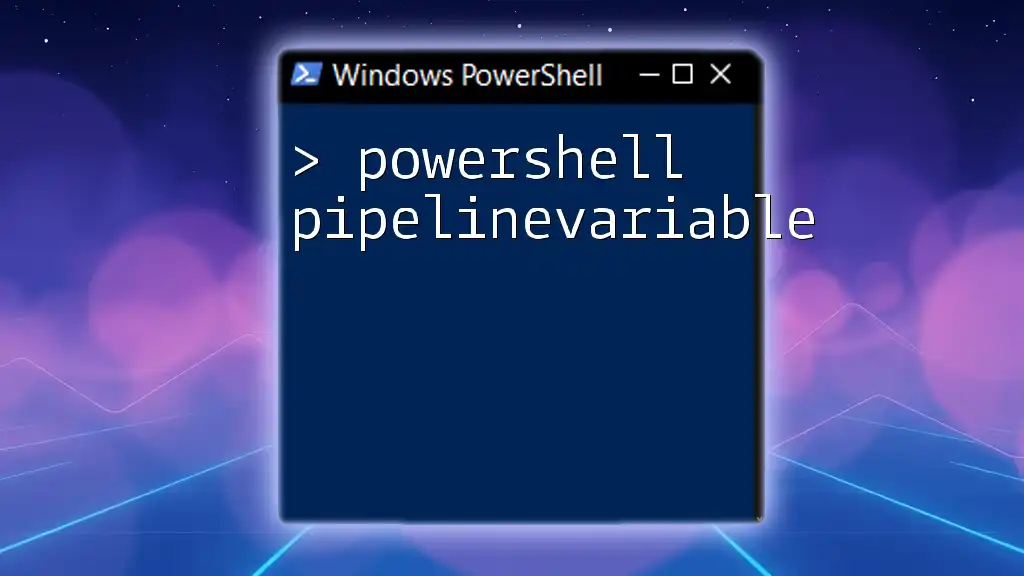
Common Use Cases
Data Processing and Transformation
Reading files into arrays is particularly useful for data processing, where you can manipulate the data easily. For example, sorting or filtering data from a CSV file can be efficiently accomplished after loading it into an array.
Scripting and Automation
Integrating file reading into your scripts enhances automation capabilities. For instance, reading a list of server names from a text file and executing commands on those servers as an array expands your scripting power and effectiveness in a task.
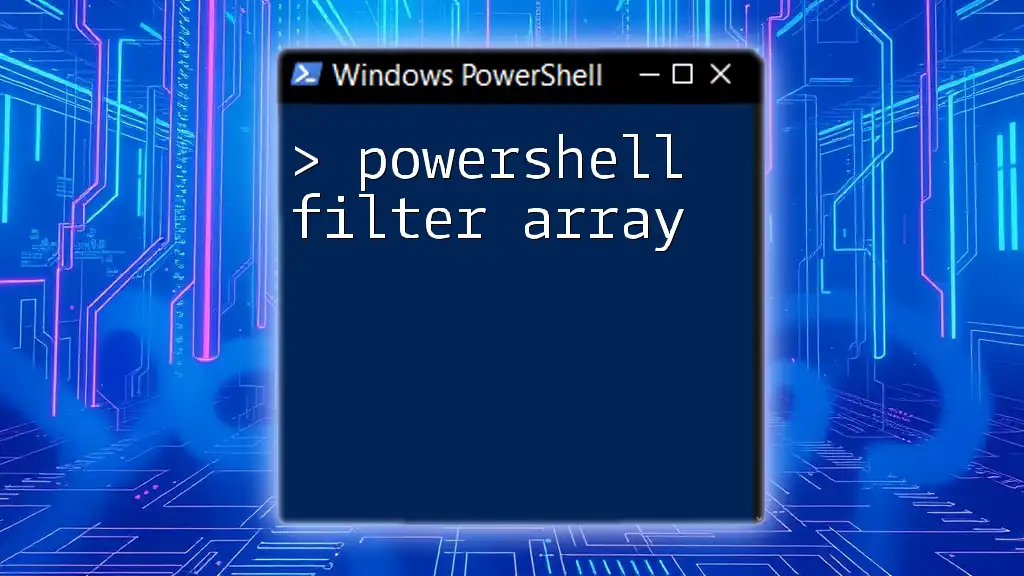
Conclusion
Understanding how to PowerShell read file into array is foundational for effective scripting. By leveraging the capabilities of the `Get-Content`, `Import-Csv`, and `ConvertFrom-Json` cmdlets, you can efficiently handle a variety of data formats. Remember to practice different techniques and adapt them to your specific needs, enhancing your PowerShell skills and productivity.
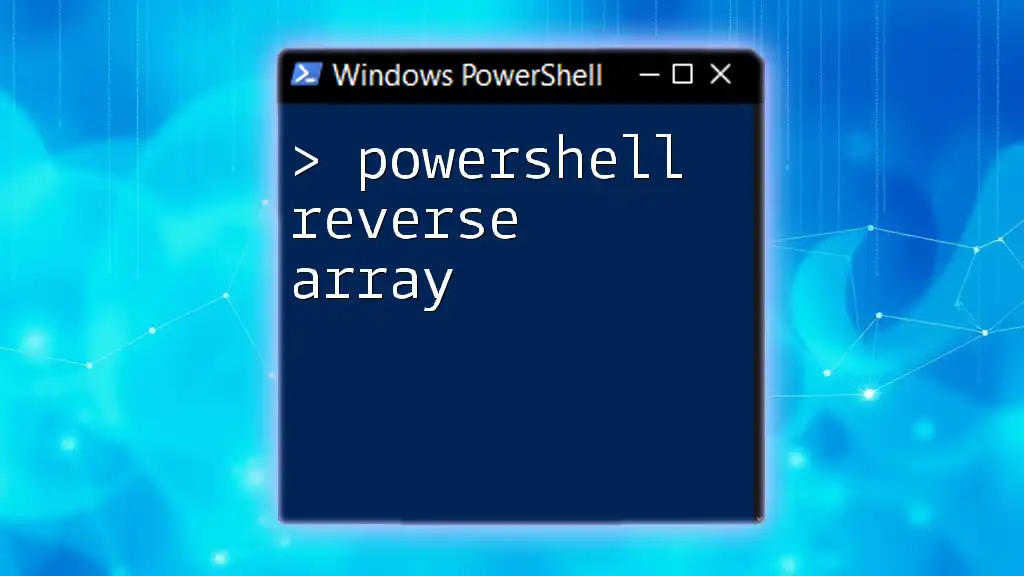
Additional Resources
For further reading, consider exploring the official PowerShell documentation and joining online forums to connect with other PowerShell enthusiasts. This community can provide support, advanced techniques, and additional use case examples.