In PowerShell, you can add an object to an array using the `+=` operator, which allows you to append the new item seamlessly. Here's how you can do it:
$array = @() # Initialize an empty array
$array += "New Item" # Add an object to the array
Understanding Arrays in PowerShell
What are Arrays?
In PowerShell, an array is a collection of items that can store multiple values under a single variable name. Arrays are crucial because they allow you to manage and manipulate collections of data efficiently. One of the key characteristics of arrays in PowerShell is that they are zero-based, meaning the first item is located at index 0.
Creating Arrays in PowerShell
Creating an array in PowerShell is simple, often achieved with the `@()` syntax. For example, if you wish to create an empty array, you would use:
$myArray = @()
Arrays can also contain different types of data, including strings, integers, and even other objects. Here’s how you might create an array with mixed data types:
$mixedArray = @("String", 123, $true, Get-Date)
Common Uses of Arrays
Arrays allow you to store multiple values efficiently. They are commonly used for:
- Storing lists of items or objects.
- Looping through values to perform actions on each element.
- Collecting output from commands or cmdlets, providing a structured way to handle data.
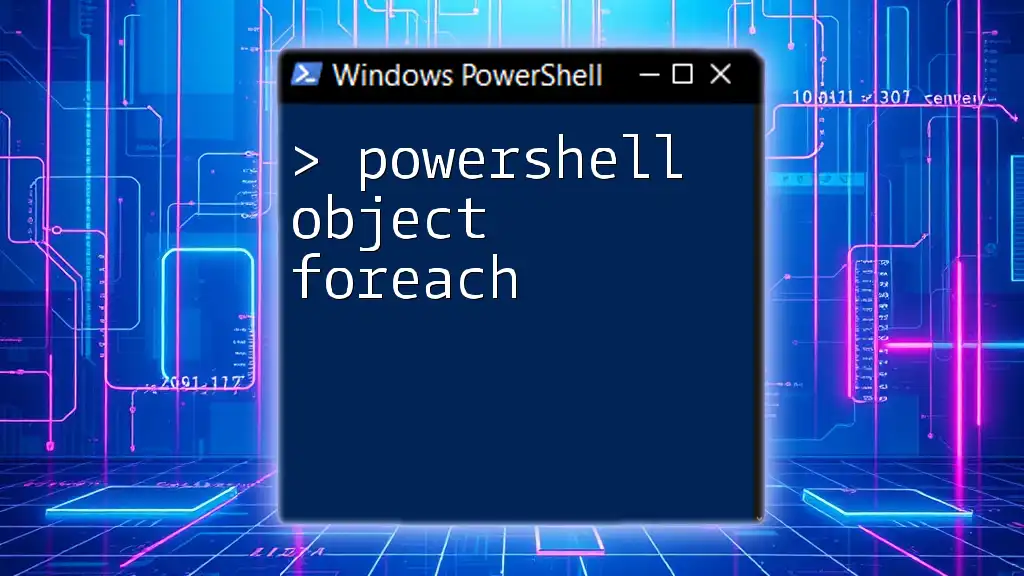
Adding Objects to Arrays in PowerShell
The `+=` Operator
Adding objects to an array can be done using the `+=` operator. This operator appends an item to the end of an array. Here’s how this works in practice:
$myArray = @()
$myArray += "First Object"
$myArray += "Second Object"
While `+=` is straightforward and effective for small arrays, it is important to note that this operator creates a new array each time it is used, which can lead to performance issues when adding elements to large arrays. For extensive data, alternative methods may be more efficient.
Using the `ArrayList` Class
To enhance performance, especially when working with large collections, consider utilizing the `ArrayList` class from the .NET framework. ArrayList can dynamically resize and perform better for frequent additions.
To create an ArrayList, you would use:
$myArrayList = New-Object System.Collections.ArrayList
Adding objects with ArrayList is just as simple:
$myArrayList.Add("First Object")
$myArrayList.Add("Second Object")
By leveraging ArrayList, you can avoid the performance penalties associated with the traditional array for many operations, as it handles resizing dynamically.
Methods for Inserting Objects
Insert Method
ArrayList also features an `Insert` method. This allows you to add an item to a specific index, making it versatile for different use cases.
For example, to insert an item at the beginning of your ArrayList, you would use:
$myArrayList.Insert(0, "New First Object")
Remove Method
Managing items in an array involves not only adding but also removing objects. You can use the `Remove` method to eliminate an item:
$myArrayList.Remove("Second Object")
This flexibility lends itself to effective data management strategies within your scripts.
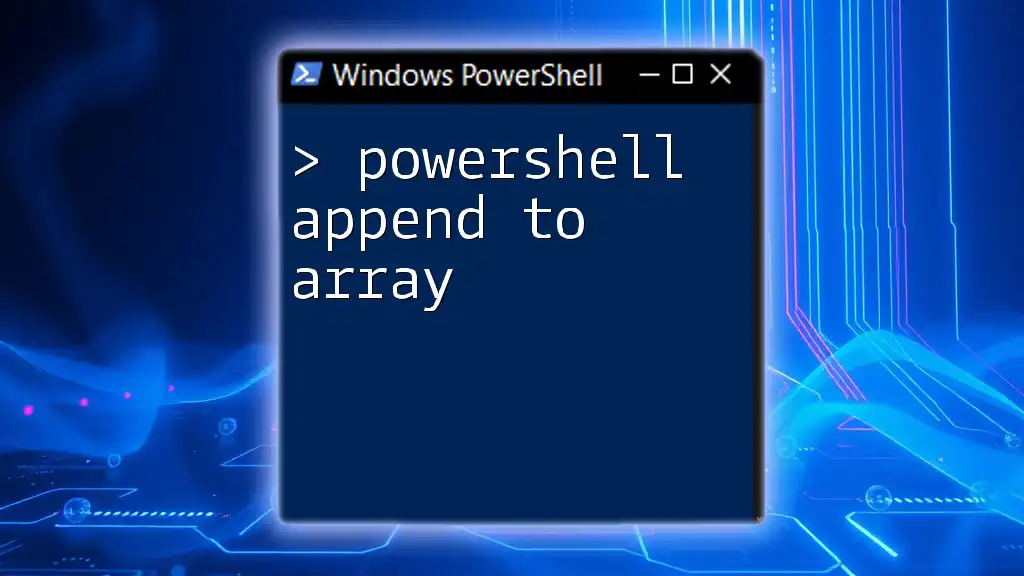
Best Practices for Managing Arrays in PowerShell
Pre-defining Array Sizes
For performance reasons, it's advantageous to pre-define array sizes when the number of items is known. This avoids the continuous resizing that occurs with `+=`, ensuring efficient memory usage. For example:
$myArray = New-Object string[] 5 # Creates an array of 5 elements
Performance Considerations
Understanding the operational distinctions between arrays and ArrayList can significantly impact your script's performance. Traditional arrays are less performant when frequently altered. In contrast, ArrayLists provide the versatility needed for dynamic data handling. Always choose the structure that aligns with your scripting needs.
Using Functions for Array Operations
Encapsulating logic into functions enhances code readability and maintainability. Here’s an example function that adds an item to an array using a reference parameter:
function Add-ToArray {
param (
[Parameter(Mandatory=$true)]
[string]$item,
[ref]$array
)
$array.Value += $item
}
This approach streamlines array operations and makes groups of commands easier to manage.
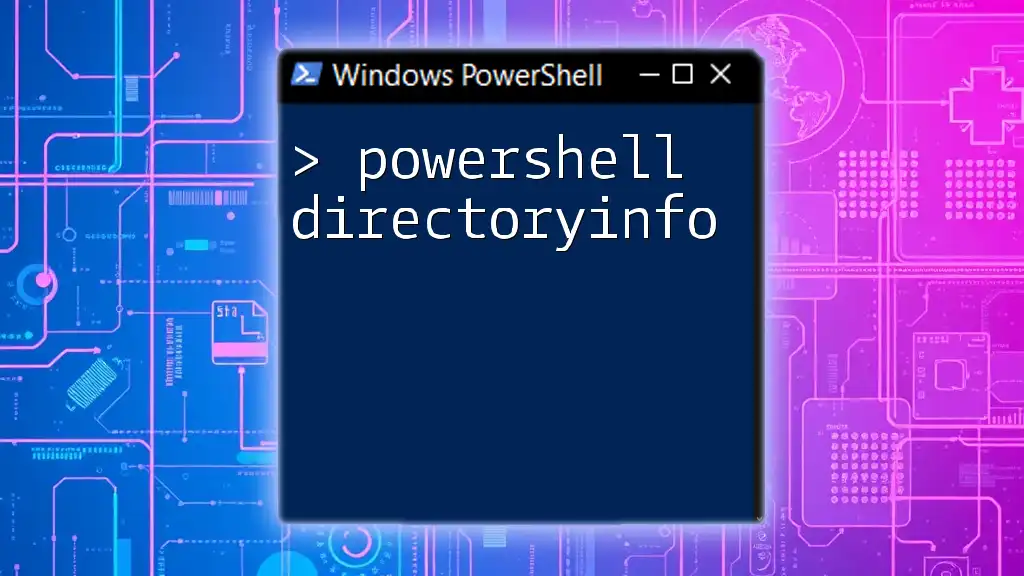
Workflows Involving Arrays
Looping Through Arrays
Looping through an array is a common task, typically accomplished using a `foreach` loop. Here’s a snippet to illustrate this:
foreach ($item in $myArray) {
Write-Host $item
}
This method enables you to effortlessly execute commands on each element stored within the array.
Conditional Operations
In certain situations, you may want to include conditional logic when working with arrays—like filtering or modifying elements based on specific criteria. Utilizing the `Where-Object` cmdlet is beneficial in these cases.
Returning Arrays from Functions
When designing your scripts, returning arrays from functions can help keep your code modular. This improves clarity and reusability. Here’s a simple example of a function that constructs and returns an array:
function Get-Numbers {
$numbers = @()
for ($i=1; $i -le 5; $i++) {
$numbers += $i
}
return $numbers
}
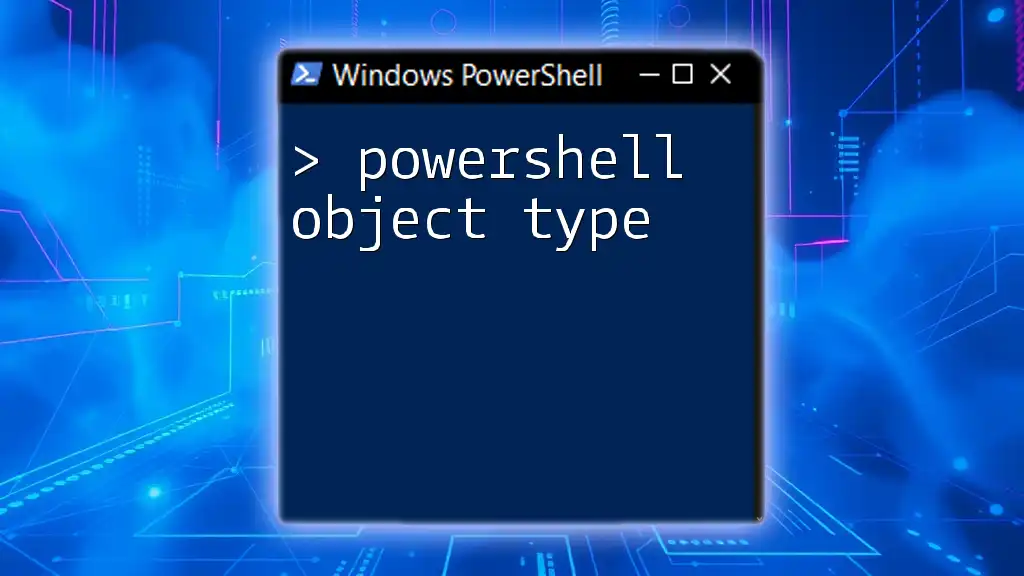
Troubleshooting Common Issues
While working with arrays, you may encounter pitfalls like attempting to modify immutable arrays or misusing indexing. To mitigate these issues, ensure that your approach to array handling is well structured. Debugging techniques can also assist in identifying problems that surface during script execution.
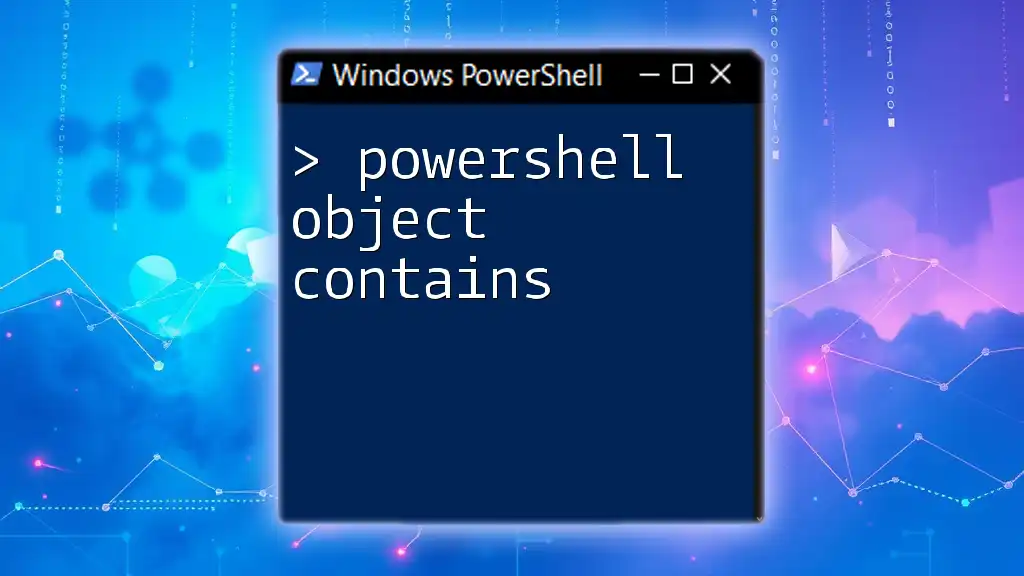
Conclusion
In this guide, we have delved into the critical aspects of adding objects to arrays in PowerShell. From understanding the basic structure to leveraging advanced techniques like ArrayLists and function encapsulation, these concepts empower you to manage data effectively in your scripts. As you practice these techniques, you will discover more functions and features that enhance your PowerShell skill set. Don’t hesitate to explore further; the PowerShell community offers a wealth of information and resources at your disposal.