A PowerShell custom object array allows you to create an array of objects with defined properties, enabling easy data manipulation and retrieval.
Here's a simple example of how to create a custom object array in PowerShell:
$customObjects = @(
New-Object PSObject -Property @{ Name = "Alice"; Age = 30 },
New-Object PSObject -Property @{ Name = "Bob"; Age = 25 },
New-Object PSObject -Property @{ Name = "Charlie"; Age = 35 }
)
What is a Custom Object in PowerShell?
Custom objects are a powerful feature in PowerShell that provide a way to create data structures in a flexible manner. They allow users to define their own properties and methods, unlike traditional data types. This adaptability makes custom objects particularly useful when dealing with complex data scenarios.
Why Use Custom Objects?
Using custom objects offers several advantages:
- Flexibility: Custom objects can represent intricate data more effectively than built-in types, which can often be limiting.
- Clarity: By defining specific properties, the code becomes easier to read and understand.
- Grouping of Related Data: They allow you to encapsulate related pieces of information together, making your scripts more organized.

Creating Custom Objects
Using PSObject Class
One way to create custom objects is using the `PSObject` class. This method leverages PowerShell's ability to construct a personalized object by specifying properties dynamically.
For example:
$customObject = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
Occupation = "Software Developer"
}
This command creates a custom object with three properties: Name, Age, and Occupation.
Using [PSCustomObject] Type Accelerator
A more streamlined approach to create custom objects is using the `[PSCustomObject]` type accelerator. This method is often favored because it enhances performance and readability.
Here’s how to create a custom object with the `[PSCustomObject]` type accelerator:
$customObject = [PSCustomObject]@{
Name = "Jane Doe"
Age = 28
Occupation = "Data Analyst"
}
This creates a similar custom object, but with clearer and more concise syntax.
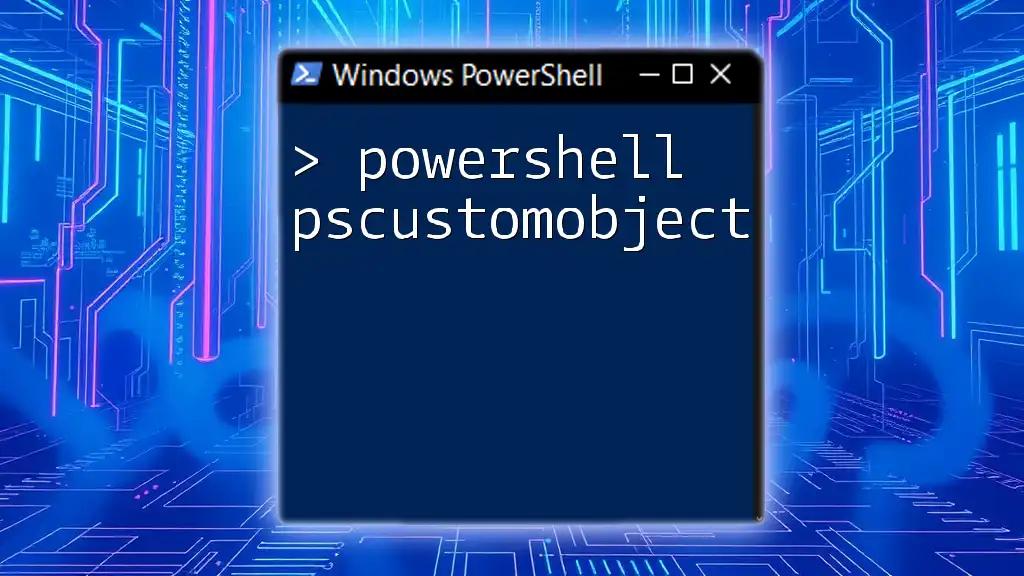
Understanding Arrays in PowerShell
What is an Array?
An array in PowerShell is a data structure that can hold multiple items of data. Arrays are versatile and can include strings, integers, or even custom objects.
Creating Arrays in PowerShell
You can create a simple array easily. Here’s an example:
$simpleArray = @("Apple", "Banana", "Cherry")
This command initializes an array containing three strings.
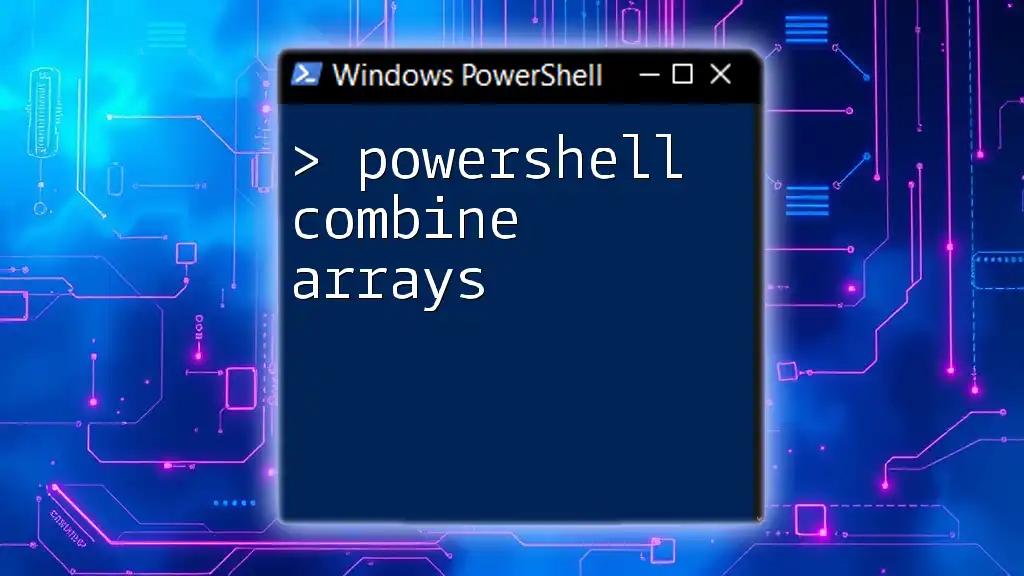
Combining Custom Objects and Arrays
Concept of Object Arrays
An object array is essentially an array where each element is a custom object. This allows you to manage collections of data that are structured and meaningful.
Creating an Array of Custom Objects
To create an array of custom objects, you simply encapsulate multiple custom objects in an array notation. Here's an example:
$employees = @(
[PSCustomObject]@{ Name = "Alice"; Age = 25; Occupation = "Designer" },
[PSCustomObject]@{ Name = "Bob"; Age = 35; Occupation = "Manager" }
)
In this case, we have an array called $employees containing two custom objects, each representing an employee with specific properties.

Accessing and Manipulating Custom Object Arrays
Accessing Elements in Custom Object Arrays
You can access elements in a custom object array by using their index. For instance, to retrieve the name of the first employee, use the following command:
$employees[0].Name # Outputs: Alice
This command accesses the Name property of the first custom object in the array.
Filtering Custom Object Arrays
Filtering is an essential aspect of managing object arrays. You can use the `Where-Object` cmdlet to filter objects based on certain criteria. For example, if you want to find all employees who are managers, you can run:
$managers = $employees | Where-Object { $_.Occupation -eq "Manager" }
This snippet effectively filters the $employees array down to only those with the occupation of Manager.

Modifying Custom Objects in Arrays
Editing Properties
To change properties of an object within an array, you can directly assign a new value to the property. For instance, if you want to change Bob’s age:
$employees[1].Age = 36 # Change Bob's age to 36
This updates the Age property of the Bob custom object.
Removing Custom Objects from an Array
Removing custom objects from an array can be accomplished by filtering out the ones you want to eliminate. For example, if you want to remove Alice from the employee list:
$updatedEmployees = $employees | Where-Object { $_.Name -ne "Alice" }
This filters the $employees array to create a new array, $updatedEmployees, that excludes Alice.

Real-World Use Cases for Custom Object Arrays
Reporting
Custom object arrays are incredibly useful for generating reports. By encapsulating data into structured objects, you can easily format and present information. For instance, you may extract employee information and print a formatted report listing each employee's name and occupation.
Scripting for Automation
Another practical application of custom object arrays is in automation scripts. For example, you could manage and automate the deployment of user accounts based on the data stored in a custom object array.
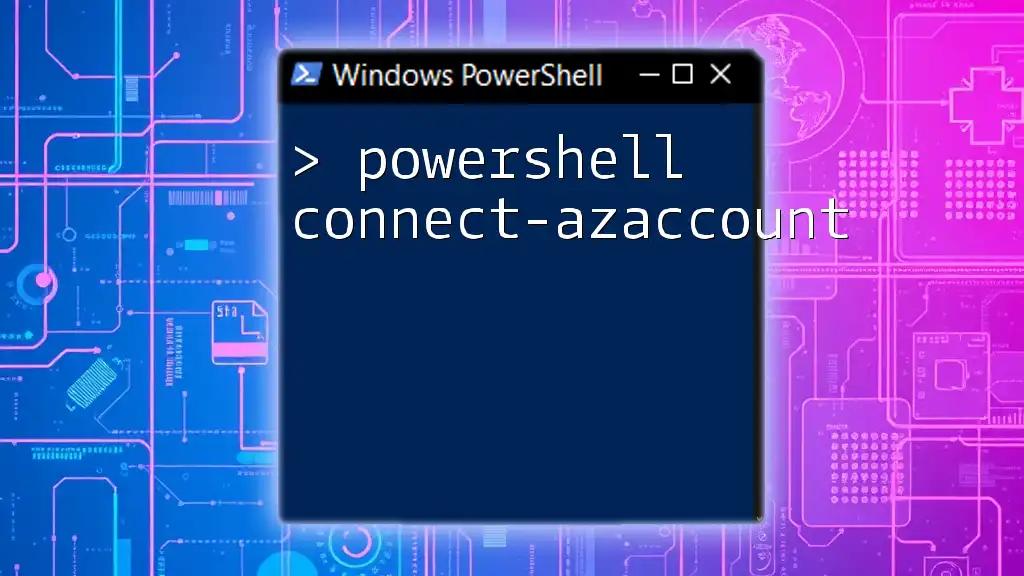
Best Practices for Using Custom Object Arrays
Clarity and Readability
When working with custom object arrays, prioritize clarity. Use descriptive property names and maintain an organized structure throughout your objects to enhance readability for you and others who may use your scripts.
Performance Considerations
For larger datasets, be mindful of performance. Custom objects allow extensive flexibility, but they can consume more resources than basic types. Optimize your scripts by using arrays judiciously and consider potential performance impacts.
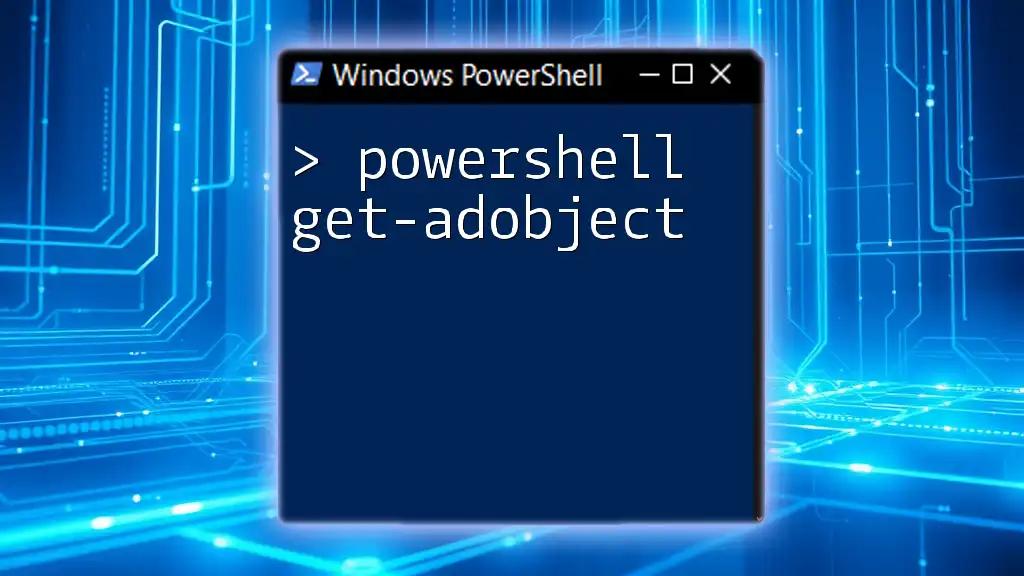
Conclusion
In summary, PowerShell custom object arrays are invaluable tools for structuring and managing data efficiently. They allow for clear representation of complex information and streamline various scripting tasks. By understanding how to create, access, and manipulate these arrays, you can enhance your PowerShell scripting capabilities significantly.