To import a PFX file with a password in PowerShell, you can use the following command, which specifies the path to the PFX file and the password for its protection.
$certPassword = ConvertTo-SecureString -String "YourPassword" -AsPlainText -Force
Import-PfxCertificate -FilePath "C:\path\to\your\file.pfx" -CertStoreLocation Cert:\LocalMachine\My -Password $certPassword
Understanding PFX Files
What is a PFX File?
A PFX file, short for Personal Exchange Format, is a binary file format used to store a combination of a private key and its associated X.509 certificate chain. It is commonly utilized in SSL certificates and is essential for secure communications within applications and web servers. PFX files are important because they bundle multiple components into a single file, making it easier to manage and transport security credentials.
Why Use PowerShell for PFX Management?
PowerShell provides a dynamic scripting environment for automating numerous administrative tasks, including certificate management. Using PowerShell to handle PFX files allows for:
- Automation: Reduces the manual effort needed to import or manage certificates.
- Scriptability: Facilitates integration into deployment scripts and CI/CD pipelines for seamless security management.
- Centralized Management: Allows administrators to manage certificates across multiple machines through consistent commands.
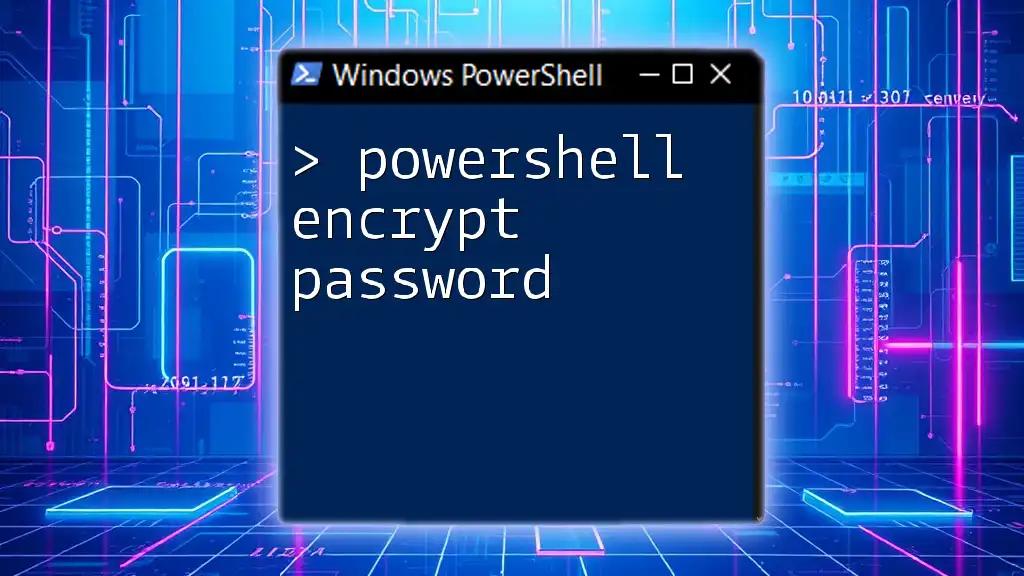
Pre-requisites
PowerShell Version
Before proceeding, it’s crucial to know your PowerShell version. You can easily check this by running the following command in your PowerShell terminal:
$PSVersionTable.PSVersion
Ensure that you are using a version compatible with certificate management, preferably PowerShell 5.1 or later.
Certificates Store
Windows has a built-in Certificate Store that keeps track of all installed certificates. Understanding where to import your PFX file is vital since different stores serve different purposes. Common locations include:
- LocalMachine: Affects all users on the computer.
- CurrentUser: Specific to the current user profile.
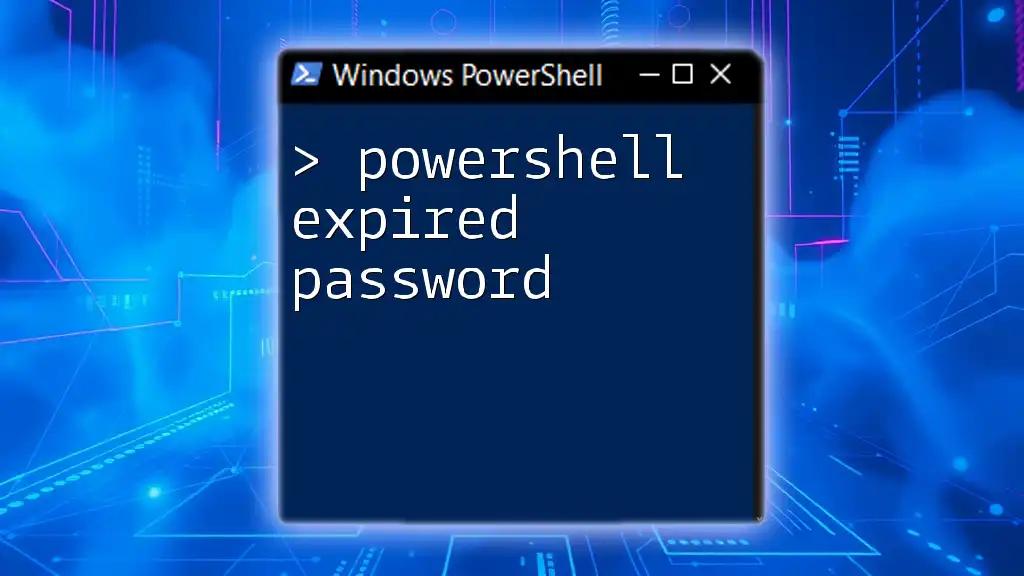
Step-by-Step Guide to Import PFX with Password
Step 1: Locate the PFX File
The first step is identifying the location of the PFX file you wish to import. Ensure your PFX file is stored securely in a known directory. Set your file path using a variable in PowerShell:
$pfxFilePath = "C:\path\to\your\certificate.pfx"
Step 2: Set the Password
Using a secure password for the PFX file is paramount for security. Create a variable for your password, making sure to use a secure string to protect sensitive data.
$password = ConvertTo-SecureString -String "YourPFXPassword" -AsPlainText -Force
This command converts your plaintext password into a secure string, enhancing security during the import process.
Step 3: Import the PFX File
Next, you will use the `Import-PfxCertificate` cmdlet, which provides a straightforward command to import your PFX file. Here’s how the command looks:
Import-PfxCertificate -FilePath $pfxFilePath -CertStoreLocation Cert:\LocalMachine\My -Password $password
Parameters explained:
- `-FilePath`: Specifies the path to your PFX file.
- `-CertStoreLocation`: Indicates where you want to import the certificate. For instance, `Cert:\LocalMachine\My` is a common choice for certificate storage.
- `-Password`: The password you set in the previous step. This ensures that your private key is decrypted correctly during the import process.
Step 4: Verify the Import
After successfully running the import command, it’s essential to ensure that the certificate was imported without issues. You can verify this by querying the certificate store:
Get-ChildItem Cert:\LocalMachine\My | Where-Object { $_.Subject -like "*YourCertificateSubject*" }
Replace `YourCertificateSubject` with a distinguishing characteristic of your imported certificate. If the import was successful, you should see your certificate listed.
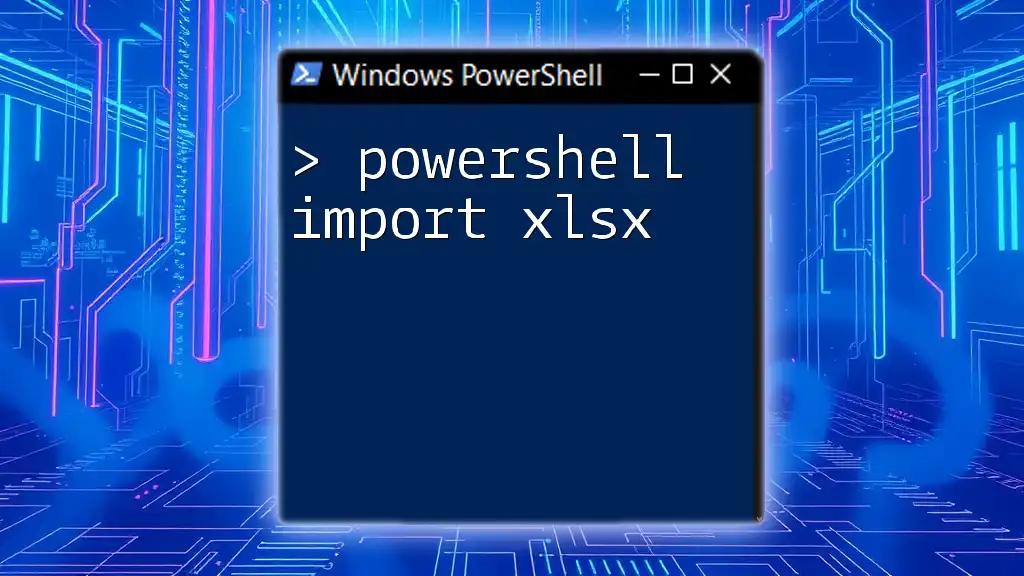
Common Issues and Troubleshooting
Incorrect Password Error
One of the most frequent issues arises from entering the wrong password during the import process. Double-check that the password you converted to a secure string matches the actual password of the PFX file.
Access Denied Errors
If you encounter access denied errors, it is often due to insufficient permissions. Always ensure you're running PowerShell as an Administrator to avoid such issues.
Certificate Store Not Found
When specifying the certificate store location, be cautious about the spelling and existence of the store. If you attempt to import into a non-existent store, you will receive an error. Verify that you’re using the correct path.
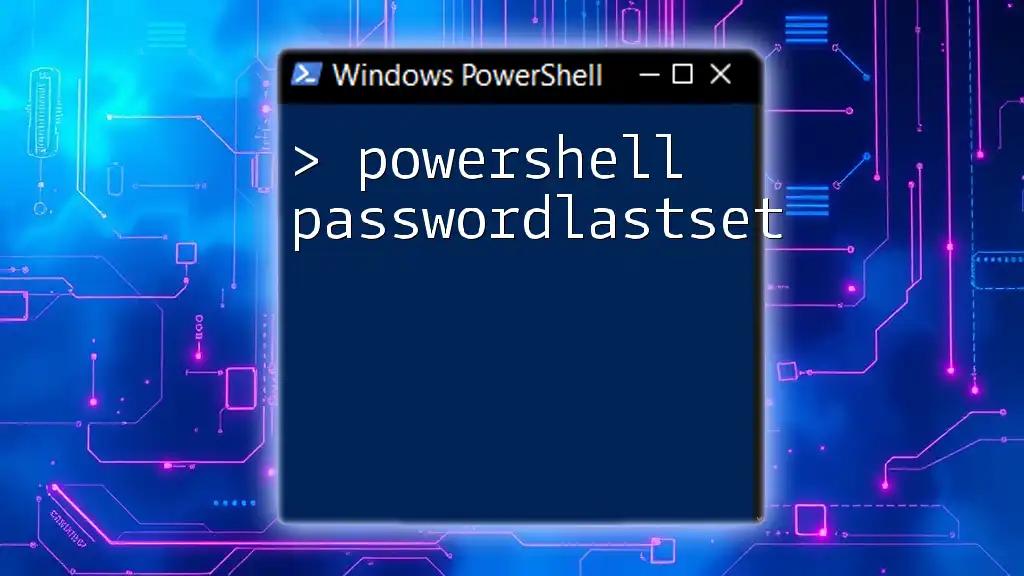
Best Practices for Managing PFX Files
Keep Certificates Secure
Handling PFX files securely is crucial. Ensure you:
- Use strong, complex passwords for your PFX files.
- Store PFX files in secure locations protected by appropriate access controls.
- Regularly audit installed certificates and remove any unnecessary or outdated certificates.
Automating Certificate Imports
Consider automating the import process using scheduled PowerShell scripts. Automating this task not only saves time but also reduces the potential for human error, ensuring that certificates are consistently imported according to organizational policies.
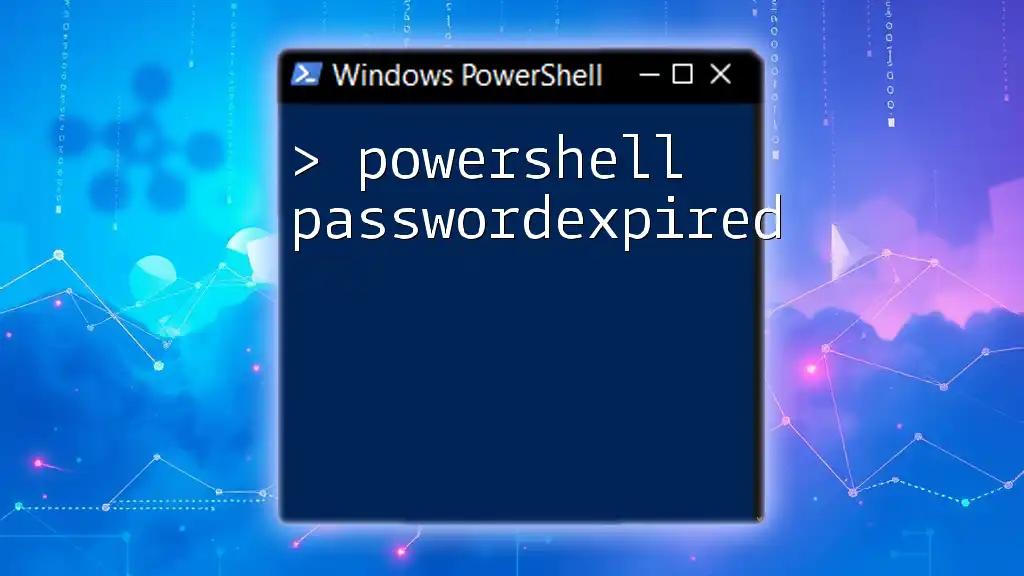
Conclusion
PowerShell is an incredibly powerful tool for managing PFX files and certificates efficiently. By following the straightforward steps outlined above, you can confidently import PFX files with passwords, enhancing your system's security. Embracing automation and best practices not only fosters a secure environment but also streamlines your workflow, making certificate management a breeze.
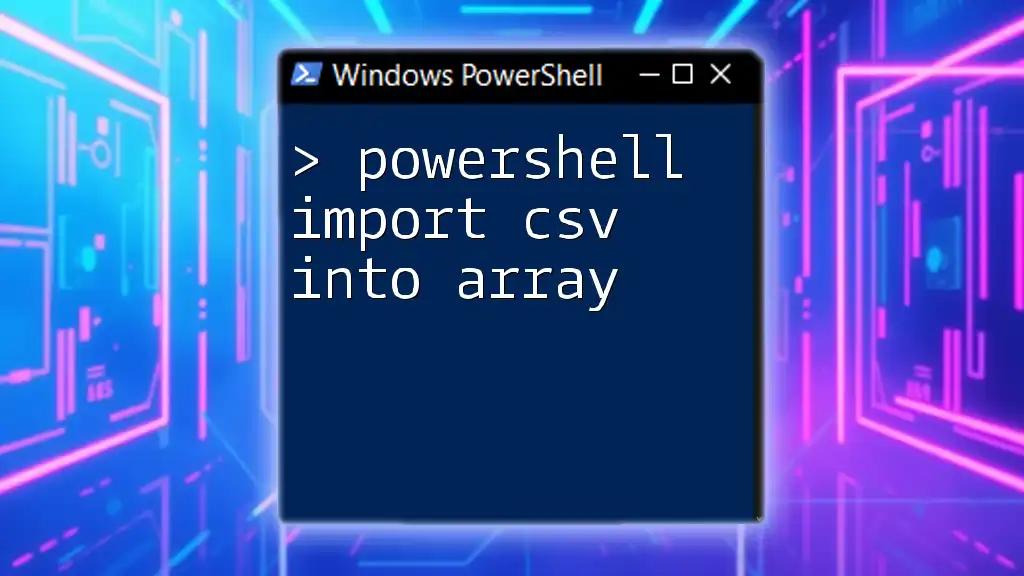
Additional Resources
For further reading, you can explore the official Microsoft documentation on PowerShell cmdlets and certificate management. These resources provide deeper insights and updates on best practices, ensuring you stay informed.
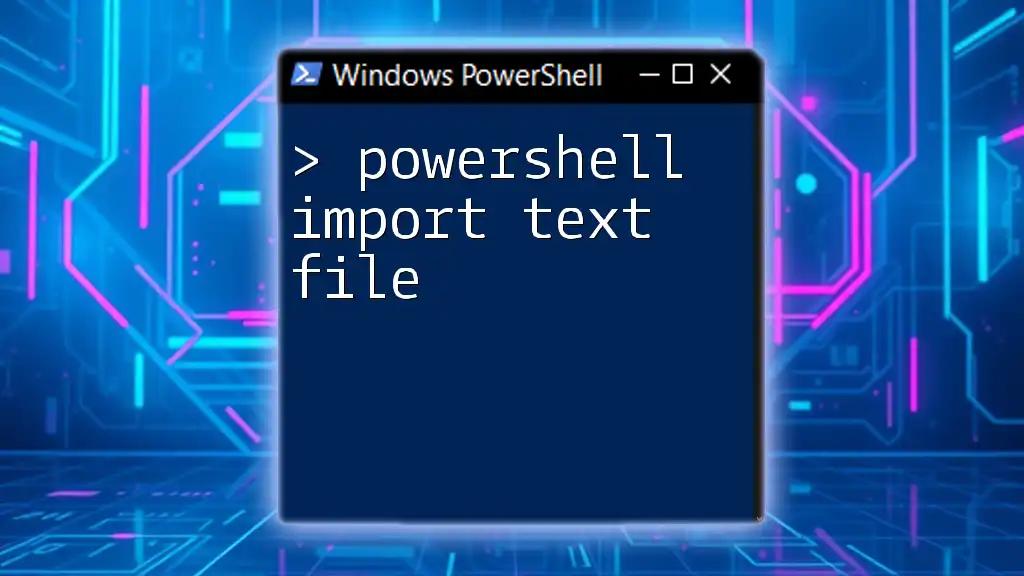
Call to Action
If you found this guide helpful, be sure to subscribe for more tips and tricks on leveraging PowerShell for your automation needs. We encourage you to share your experiences or any questions you may have in the comments section below!