The `Import-XML` cmdlet in PowerShell allows you to read XML data from a file and convert it into a usable object format for easy manipulation and querying.
Here's a code snippet demonstrating how to import an XML file:
$xmlData = Import-Clixml -Path "C:\path\to\yourfile.xml"
Understanding XML Basics
What is XML?
XML, or eXtensible Markup Language, is a markup language that is both human-readable and machine-readable. It is designed to store and transport data, providing a format that allows users to create custom tags. This flexibility makes XML exceptionally useful for various applications, particularly in data interchange between systems. Common uses include configuration files, data feeds, and data storage for applications.
Structure of XML Files
The structure of XML files is hierarchical, consisting of nested elements, attributes, and text. The syntax follows a set of rules:
- Elements: The core building blocks, surrounded by opening and closing tags.
- Attributes: Additional information about elements, found within the opening tag.
- Hierarchy: Elements can contain child elements, creating a tree-like structure.
Here’s a simple example of an XML document:
<book>
<title>Learning PowerShell</title>
<author>John Doe</author>
<year>2023</year>
</book>
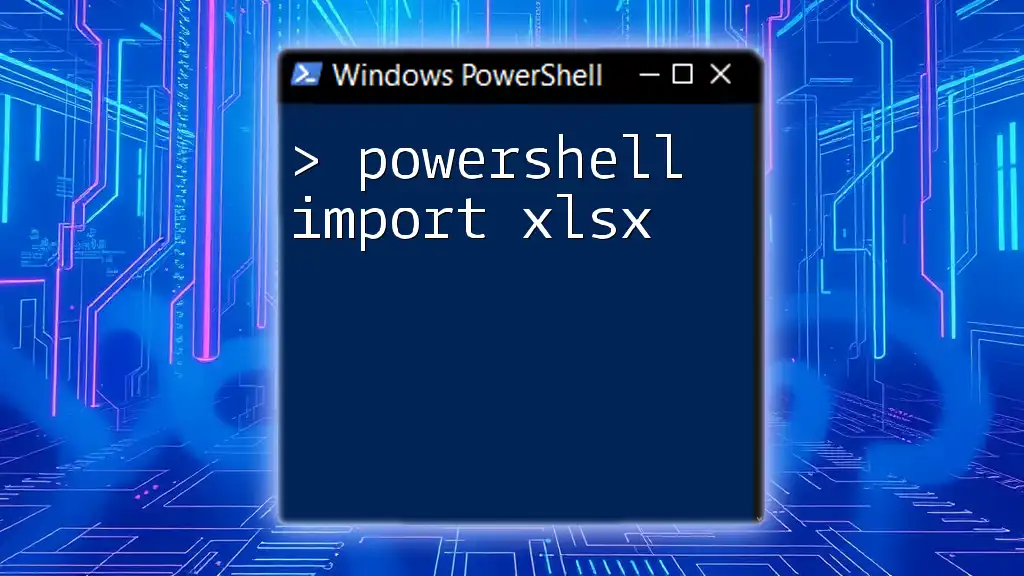
Introduction to PowerShell and XML
Why Use PowerShell for XML?
PowerShell, a powerful scripting language developed by Microsoft, provides robust capabilities to automate system administration tasks. One of its key features is the ability to handle XML data seamlessly. The benefits of using PowerShell for XML manipulation include:
- Automation: Easily automate the import, modification, and export of XML data.
- Integration: Interact with other tools and systems that use XML as a standard format.
- Simplicity: Perform complex operations using concise and readable commands.
How PowerShell Handles XML
PowerShell treats XML data as an object. This means that when you import an XML file, PowerShell creates a representation of the XML structure, allowing for easy navigation and manipulation of its elements.
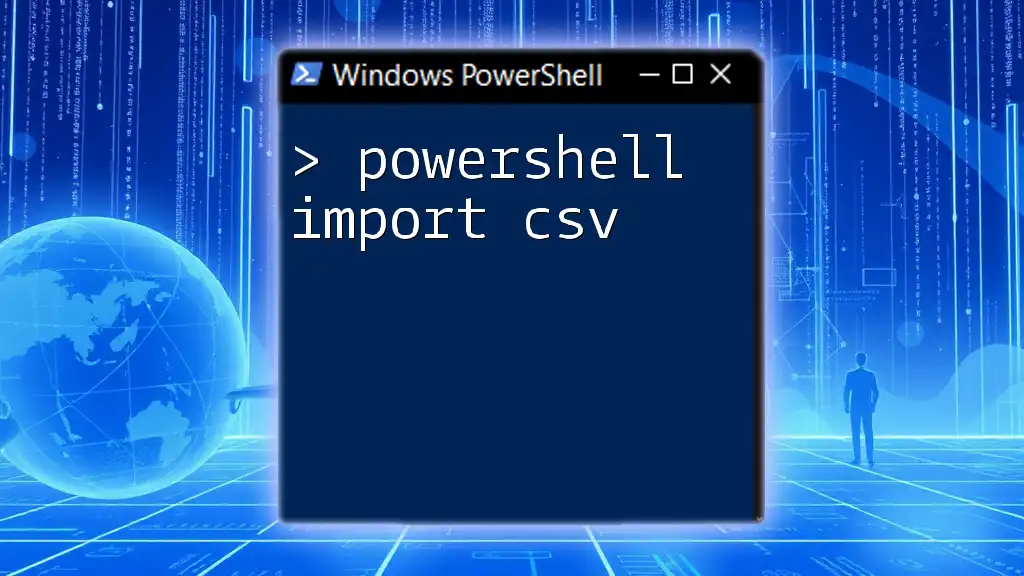
The Import-Xml Cmdlet
What is Import-Xml?
The `Import-Xml` cmdlet is a built-in PowerShell command that imports XML data from a specified file. It converts the XML into a PowerShell object, making it easier to work with the data programmatically.
Basic Usage of Import-Xml
To import an XML file, you can use the following command:
$xmlData = Import-Xml -Path "C:\path\to\your\file.xml"
In this example, replace `"C:\path\to\your\file.xml"` with the actual path to your XML file. The variable `$xmlData` now holds the XML content as a PowerShell object, enabling further manipulation.
Understanding the Output
Once the XML is imported, its structure can be accessed programmatically. For instance, if your XML has a root element called `<book>`, you can access its child elements easily:
$xmlData.book.title
This command retrieves the title of the book contained in the XML file. By following this method, you can extract specific pieces of information from the XML structure efficiently.
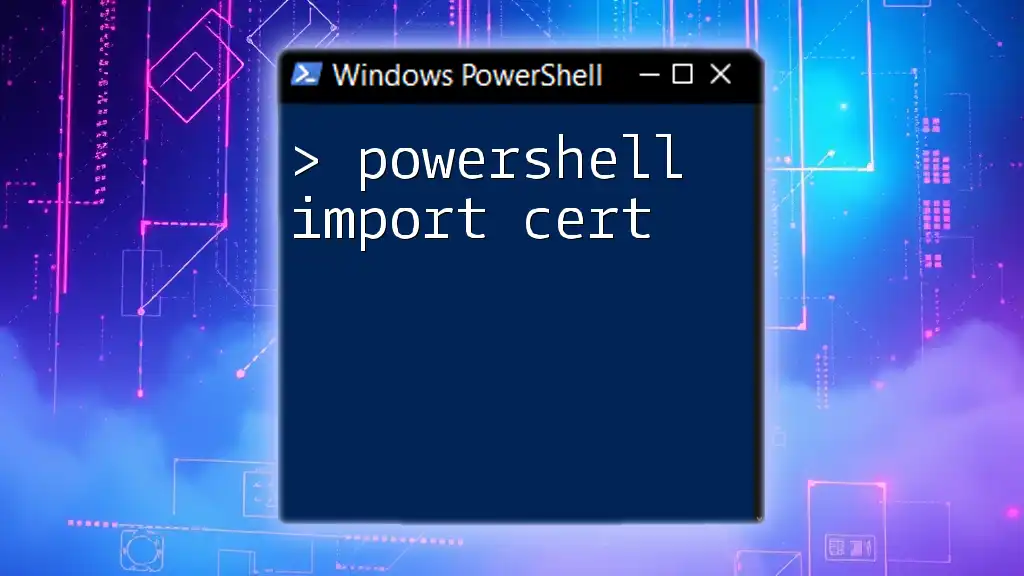
Manipulating XML Data
Navigating Through XML Nodes
To navigate through the nodes in an imported XML object, you can use dot notation. This allows you to reference elements and their properties directly. Consider iterating through child nodes with the following example:
foreach ($node in $xmlData.book) {
Write-Host "Title: $($node.title)"
}
In this code, the `foreach` loop iterates over the book elements in the XML, outputting their titles.
Modifying XML Data
PowerShell allows you to modify existing XML data easily. If you want to update an element's value, you can use the following command:
$xmlData.book.title = "Advanced PowerShell Techniques"
This command changes the title element's value to "Advanced PowerShell Techniques". This flexibility makes it straightforward to keep your XML data up to date.
Adding and Removing XML Nodes
To enhance your XML structure, you can add new child elements. Here’s how you can create a new element and append it:
$newElement = $xmlData.CreateElement("publisher")
$newElement.InnerText = "Tech Books Publishing"
$xmlData.book.AppendChild($newElement)
Conversely, if you need to remove an existing child element, you can use:
$xmlData.book.RemoveChild($xmlData.book.publisher)
This command removes the `publisher` element from the `book` node.
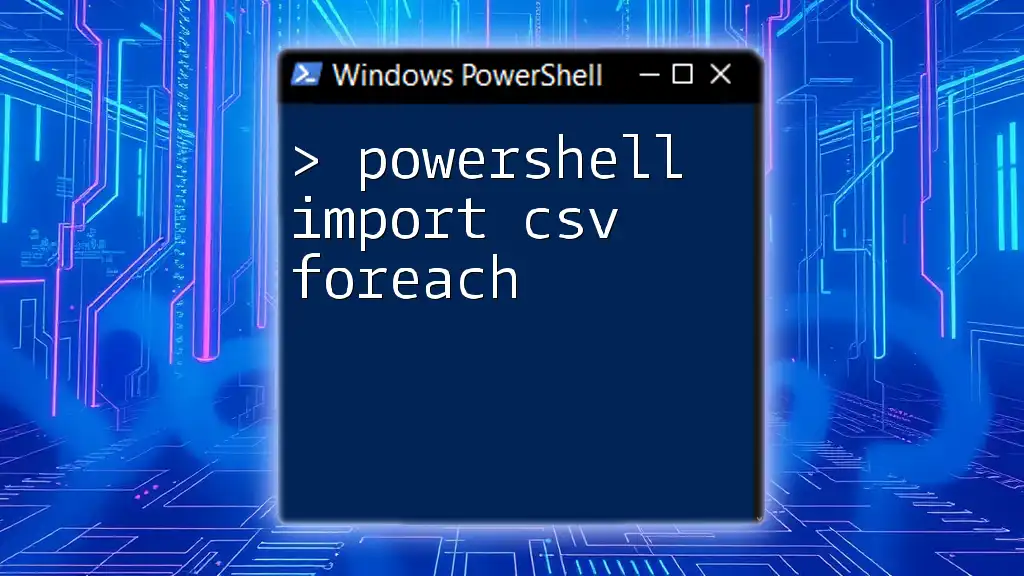
Saving XML Data Back to File
Understanding the Export-CliXml Cmdlet
The `Export-CliXml` cmdlet is the counterpart to `Import-Xml` that allows users to export PowerShell objects to an XML file. By converting objects back into XML format, you can create structured data files.
How to Save Modified XML
To save any modifications made to the XML back to a file, you will execute:
$xmlData.Save("C:\path\to\your\modified_file.xml")
This command writes the modified XML content from the `$xmlData` object to a new XML file. It’s crucial to specify the correct path, ensuring any changes made are retained in the output file.
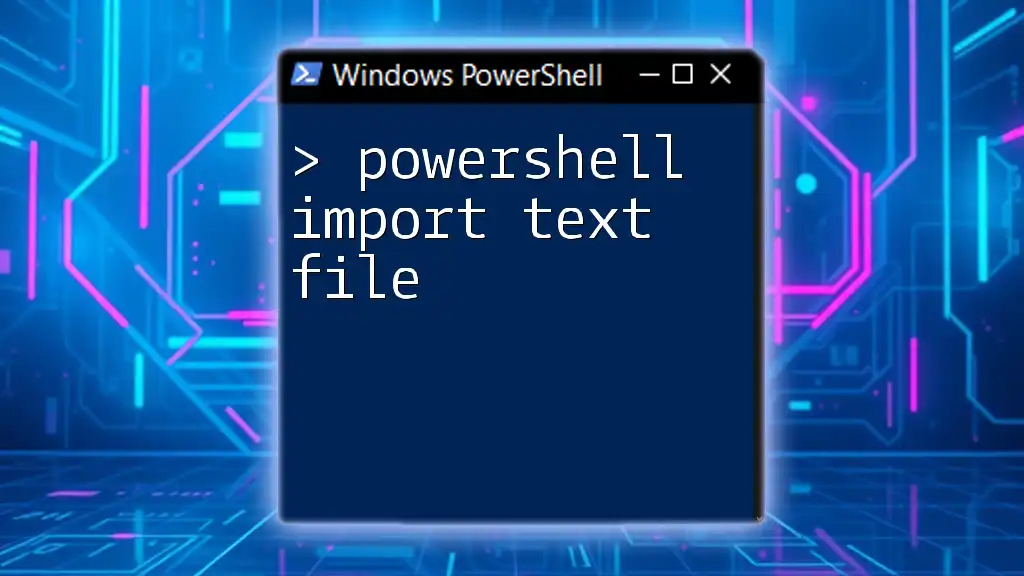
Common Use Cases for Importing XML in PowerShell
Configuration Files
Using XML for configuration files is a prevalent practice. PowerShell can easily read and update settings stored in XML format. For instance, you might have an XML configuration file that specifies application parameters. Importing this file allows you to adjust settings dynamically without editing text files directly.
Data Exchange
XML serves as a universal data format for application integration. Organizations often use PowerShell scripts to extract, transform, and load (ETL) data between disparate systems. PowerShell's ability to handle XML data makes it an excellent tool for these types of tasks.
Reporting
Generating XML reports is another powerful use case. For example, you can create PowerShell scripts that retrieve data from various sources and compile this information into an organized XML report. This approach not only automates reporting but also ensures consistency across reports.
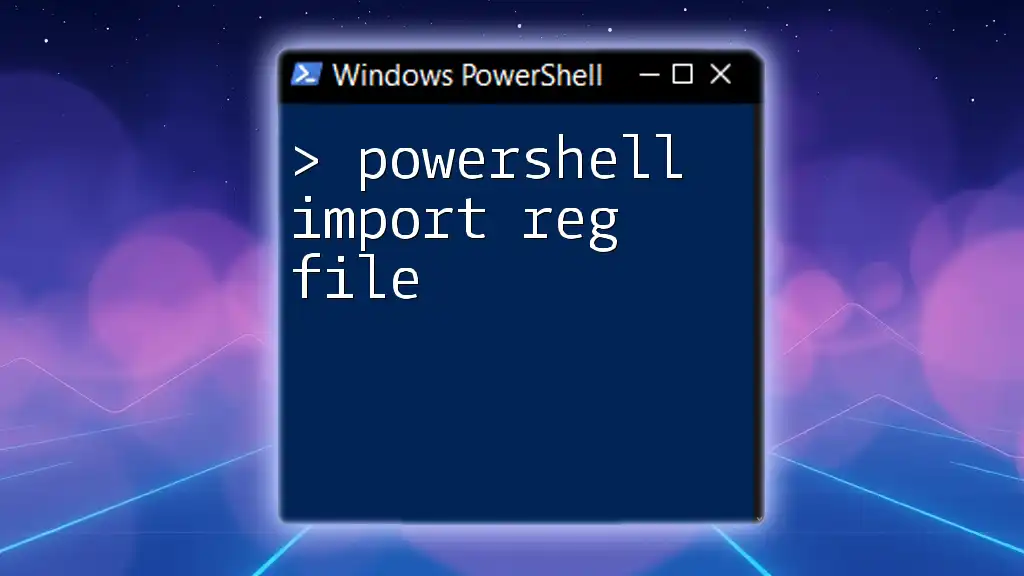
Troubleshooting Common Issues
Common Errors in Import-Xml
During the use of `Import-Xml`, users may encounter issues such as file not found errors. This problem typically arises from an incorrect file path. Always verify that the file exists at the specified location.
Another common issue is related to XML formatting. If the XML file is not well-formed (e.g., missing closing tags), PowerShell will throw an error. It’s advisable to validate your XML before importing it, ensuring that it adheres to standard XML rules.
Tips for Ensuring XML Compatibility
To avoid challenges with XML compatibility, always validate the XML before importing. Tools like XML validators can help ensure that the file structure is correct. Additionally, employing schema definitions (XSD) can ensure that your XML adheres to necessary structural guidelines.
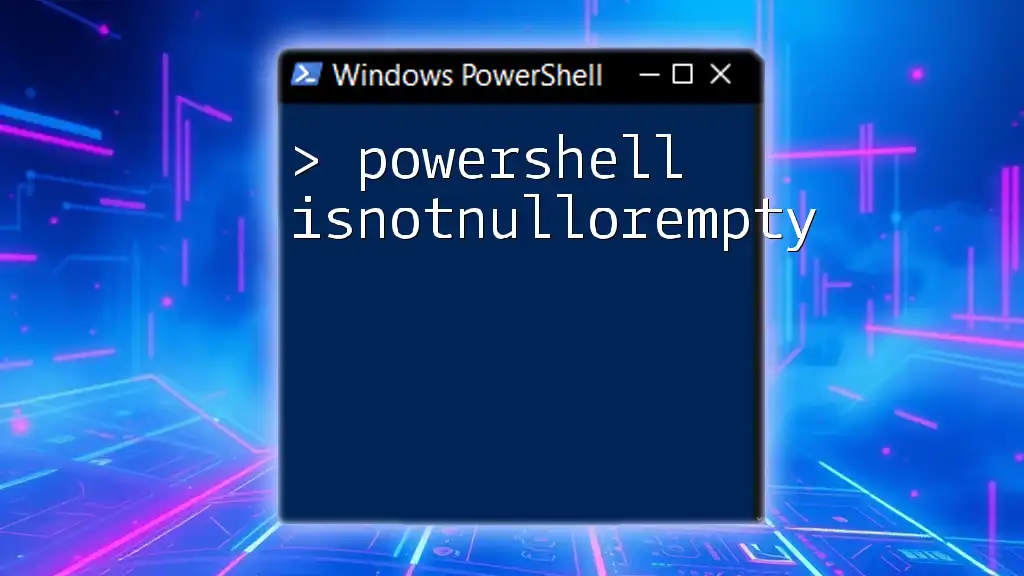
Conclusion
In summary, the PowerShell import XML capabilities offer a powerful toolset for handling XML data efficiently. From importing and modifying XML files to exporting them back after changes, PowerShell simplifies numerous tasks. By understanding how to import XML and manipulate it through PowerShell, users can leverage this knowledge to enhance their automation capabilities and streamline workflows.
As you dive deeper into PowerShell scripting and XML manipulation, consider exploring additional resources and tutorials that will help you master these tools even further. Happy scripting!