To import a .reg file in PowerShell, you can use the `reg import` command to apply the registry settings contained in the file.
reg import "C:\path\to\your\file.reg"
Understanding the Windows Registry
What is the Windows Registry?
The Windows Registry is a hierarchical database that stores low-level settings for the operating system and for applications that opt to use the registry. The structure consists of keys, which act like folders, and values, which store the actual data. The registry plays a crucial role in the functioning of Windows as it contains configuration settings for both the system and installed software.
When Would You Need to Import a REG File?
You may need to import a REG file in various scenarios, such as:
- Configuration changes: If you need to modify or create new keys and values, importing a pre-made REG file can simplify the process.
- Software setups: Some applications provide REG files to streamline installation by automatically configuring necessary settings.
Understanding these scenarios can enhance your effectiveness in system administration and troubleshooting.
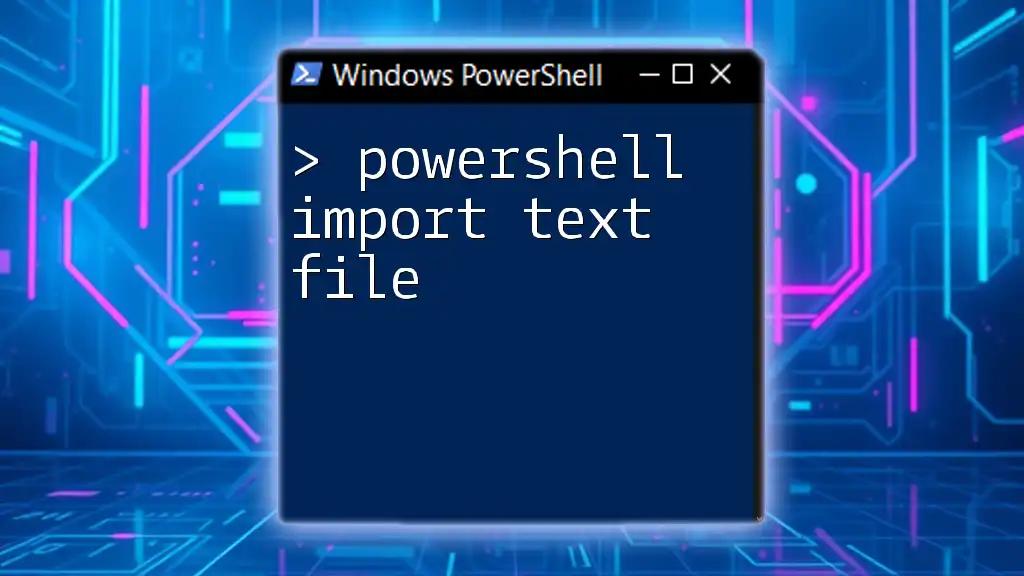
The Basics of PowerShell Commands
Introduction to PowerShell Commands
PowerShell commands, known as cmdlets, allow users to perform administrative tasks on both local and remote systems. The basic syntax of a PowerShell command is:
Verb-Noun -Parameter Value
A foundational understanding of cmdlets will serve you well as you explore the capabilities of PowerShell, including the `powershell import reg file` operation.

Preparing to Import a REG File
Creating a REG File
Creating a REG file is straightforward. A REG file is essentially a text file with a `.reg` extension that contains specific instructions to modify the registry.
Example of a simple REG file content:
Windows Registry Editor Version 5.00
[HKEY_CURRENT_USER\Software\MyApp]
"AppSetting"="Value"
This example adds a key named `MyApp` under `HKEY_CURRENT_USER\Software` and sets a string value named `AppSetting`.
Making Sure You Have the Correct Permissions
Before attempting to import a REG file, it’s vital to ensure you have the necessary permissions. You may need to run PowerShell with elevated privileges. To do this:
- Right-click on the PowerShell icon.
- Select Run as Administrator.
This ensures your commands can modify system settings without facing permission issues.
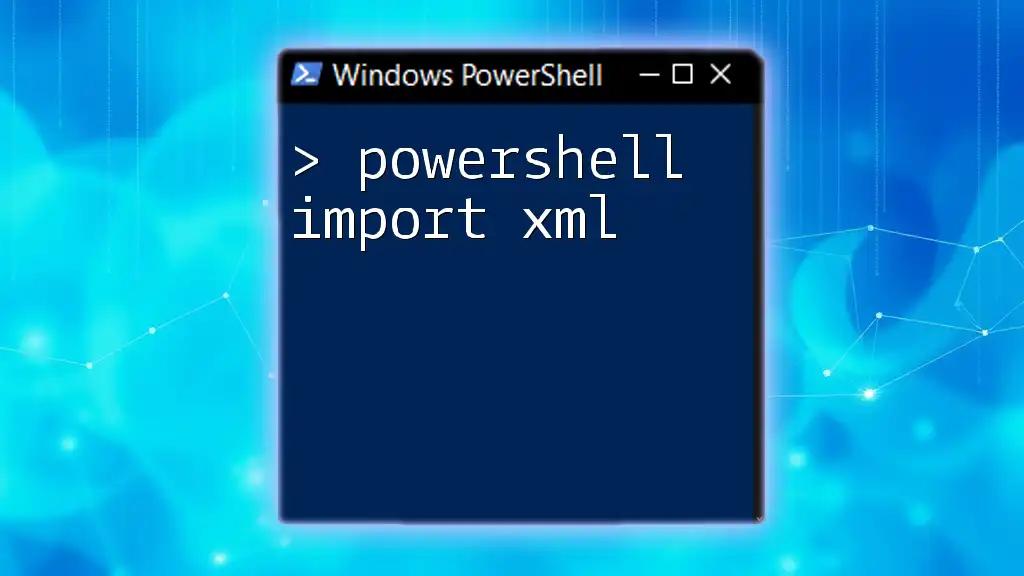
PowerShell Command for Importing a REG File
Using the `reg import` Command
To import a REG file using PowerShell, you can use the `reg import` command. The syntax is straightforward:
reg import "C:\path\to\your\file.reg"
Replace `C:\path\to\your\file.reg` with the actual path to your REG file.
Breakdown of the command syntax:
- reg import: The command used to import a REG file.
- "C:\path\to\your\file.reg": The file path where your REG file is located. Make sure to use quotes if your path has spaces.
Upon executing the command, you should see feedback indicating whether the operation was successful or if it failed due to an issue, such as a file not found or access denied.
What to Expect After Executing the Command
After running the import command, PowerShell will notify you of the import status. Expect a message indicating success or failure. If successful, the changes will take effect immediately, and you should verify that the intended changes were made to the registry.
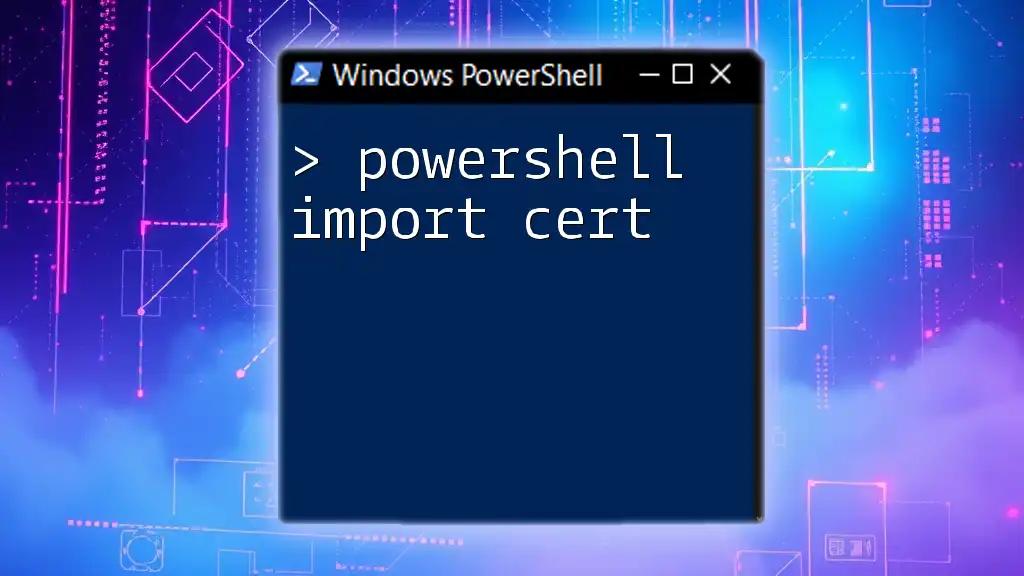
Advanced Techniques for Importing Registry Files
Conditional Importing with PowerShell
In some scenarios, you might want to check if certain keys exist before importing a REG file to prevent overwriting essential data. You can achieve this with a simple conditional statement:
if (-not (Test-Path "HKCU:\Software\YourKey")) {
reg import "C:\path\to\your\file.reg"
}
In this code snippet, if the registry path `HKCU:\Software\YourKey` does not exist, PowerShell will proceed with the import.
Importing with Error Handling
Error handling is essential to ensure that your scripts are robust and user-friendly. You can wrap your import command in a try-catch block to handle potential errors gracefully:
try {
reg import "C:\path\to\your\file.reg"
} catch {
Write-Host "An error occurred: $_"
}
This approach allows you to catch any errors that occur during the import and provide meaningful feedback instead of a generic failure message.
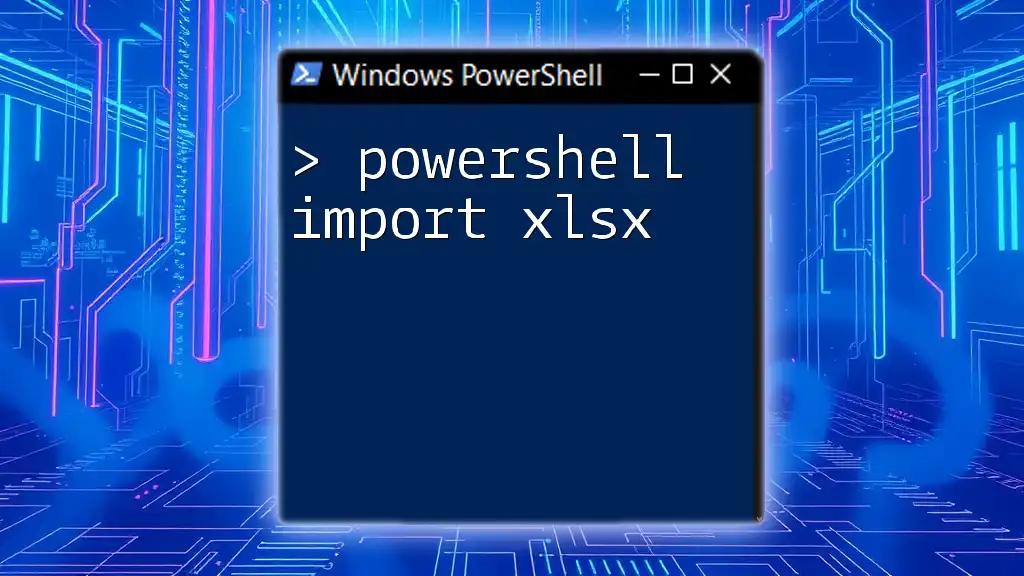
Troubleshooting Common Issues
Common Errors During Import
While performing a `powershell import reg file`, you might encounter certain common errors, such as:
- File not found: This happens if the path is incorrect.
- Permission denied: You might not have the necessary permissions to modify the registry.
- Invalid data format: Ensure your REG file's content is formatted correctly.
How to Verify Successful Imports
After running your import command, it's crucial to determine whether the changes were applied successfully. To check if a specific registry key was added, you can run:
Get-ItemProperty "HKCU:\Software\YourKey"
This command retrieves properties for the specified key, allowing you to confirm that the expected settings are in place.
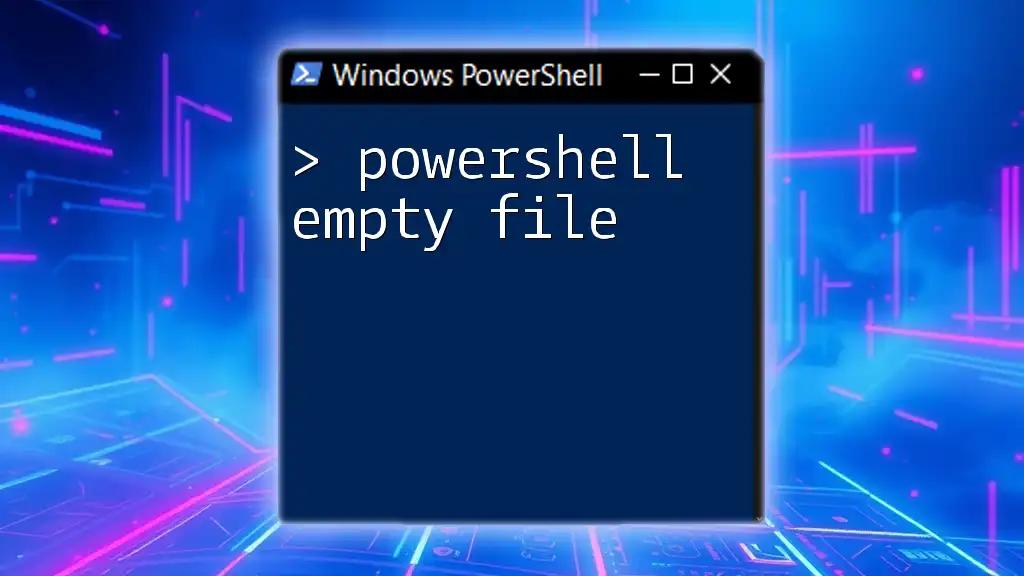
Best Practices for Working with REG Files in PowerShell
Backup Your Registry Before Changes
Always create a backup of the registry before making any changes. This precaution allows you to restore your settings if something goes wrong. You can back up the registry through PowerShell by exporting the current settings:
reg export "HKCU" "C:\path\to\backup.reg"
Documenting Changes Made by REG Imports
It is beneficial to maintain a change log for all registry modifications. Documenting what changes were made, when, and why can significantly aid troubleshooting and system administration in the future.
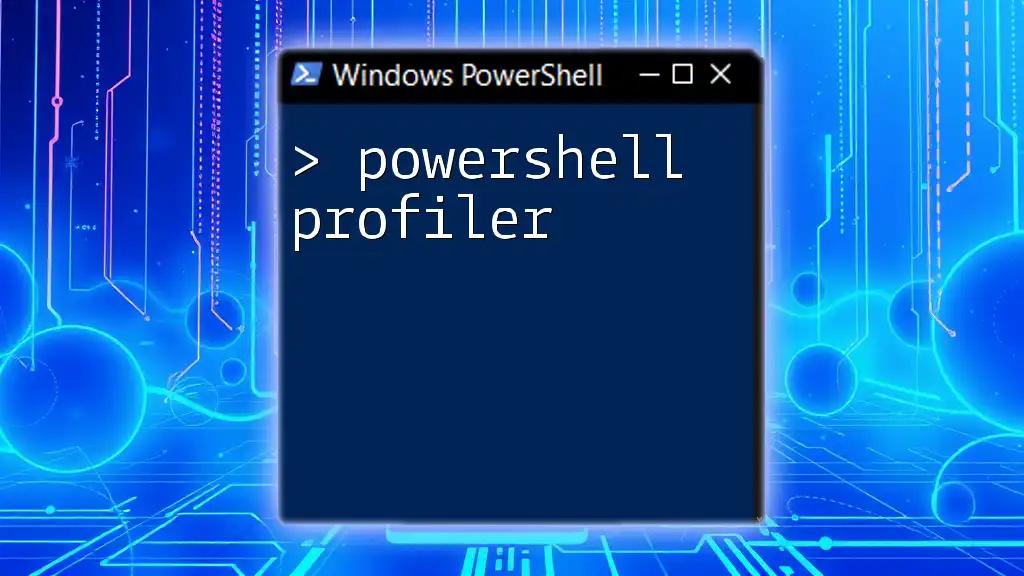
Conclusion
In this guide, we’ve explored the process of using PowerShell to import REG files, delving into command usage, best practices, and common pitfalls. By understanding these concepts, you can effectively manage registry settings and enhance your PowerShell skills.
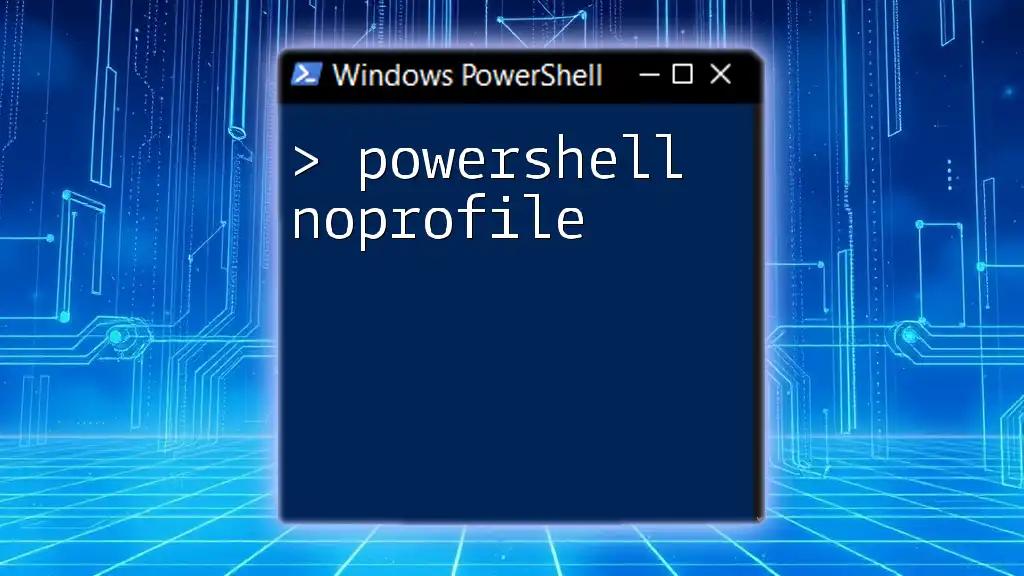
Call to Action
Now that you have the knowledge, try executing your first import with the provided examples. Don’t hesitate to explore other PowerShell commands for comprehensive system management. For more concise and targeted training sessions on PowerShell, consider signing up for our upcoming courses!
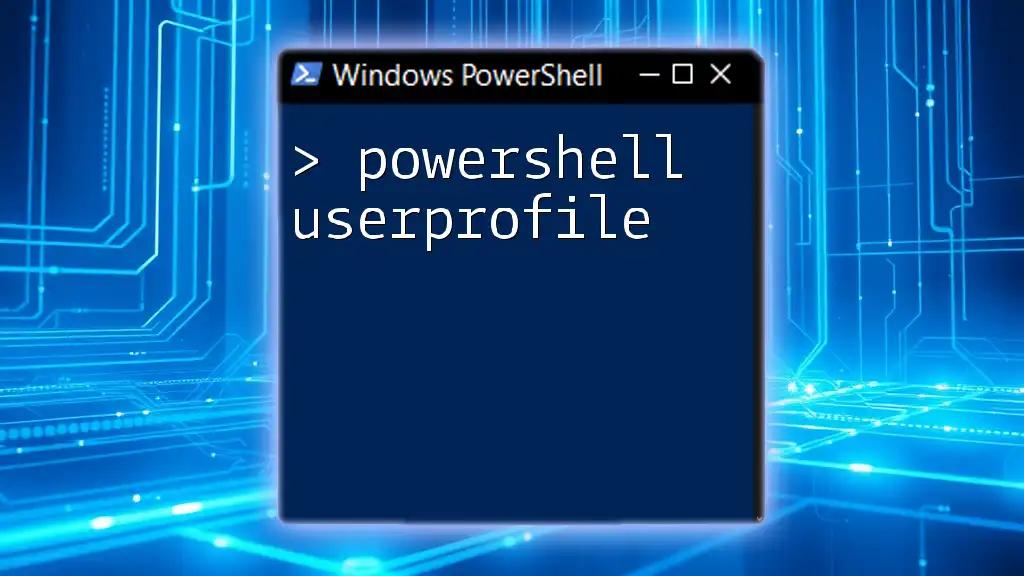
Additional Resources
Links to PowerShell Documentation
For further reading and to deepen your understanding of PowerShell’s capabilities, visit Microsoft's official documentation on PowerShell.
Recommended Tools and Scripts
Explore third-party tools to aid in managing REG files and enhancing your PowerShell scripting experience. These can serve as valuable resources as you expand your skills.