PowerShell provides built-in cmdlets for working with XML data, allowing users to easily parse, manipulate, and retrieve information from XML files.
[xml]$xml = Get-Content 'C:\Path\To\File.xml'
$xml.DocumentElement | Select-Object -Property *
Understanding XML
What is XML?
XML, or eXtensible Markup Language, is a versatile markup language that is primarily used for storing and transporting data. It provides a structured way to describe information using self-descriptive tags, making it both machine-readable and human-readable.
Compared to other data formats, such as JSON or CSV, XML’s flexibility allows it to be easily extended, which is particularly useful in many data-driven applications and protocols, such as web services and configuration files.
Structure of an XML Document
An XML document is composed of several key components that define its structure:
- Elements are the building blocks of XML. They are marked by opening and closing tags.
- Attributes provide additional information about elements. They are defined within the opening tag.
- Hierarchy illustrates the nested relationship between different elements.
For example, the structure of a simple XML document is as follows:
<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend!</body>
</note>
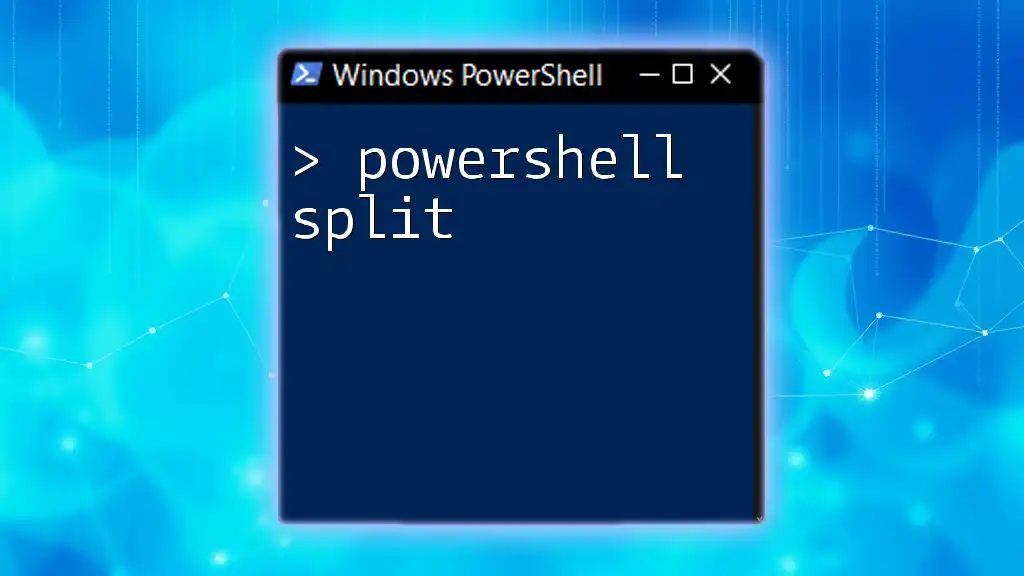
The Role of PowerShell in XML Manipulation
PowerShell's XML Capabilities
PowerShell provides powerful tools and cmdlets that simplify XML manipulation. It is equipped to handle diverse tasks such as reading, modifying, and creating XML files. The ability to automate these processes through scripting significantly enhances productivity, especially in system administration and data management.
Loading XML Data
To begin working with XML data, you first need to load it into your PowerShell session.
Using `Get-Content` to Load XML You can easily load an XML file using the following command:
$xmlData = Get-Content "C:\path\to\your\file.xml"
This reads the content of the specified XML file into the variable `$xmlData`.
Parsing XML with `Get-Xml` For a more structured approach, you can cast the content to XML using:
$xml = [xml](Get-Content "C:\path\to\your\file.xml")
This allows you to work with the XML document as an object, enabling easier access to its elements and attributes.
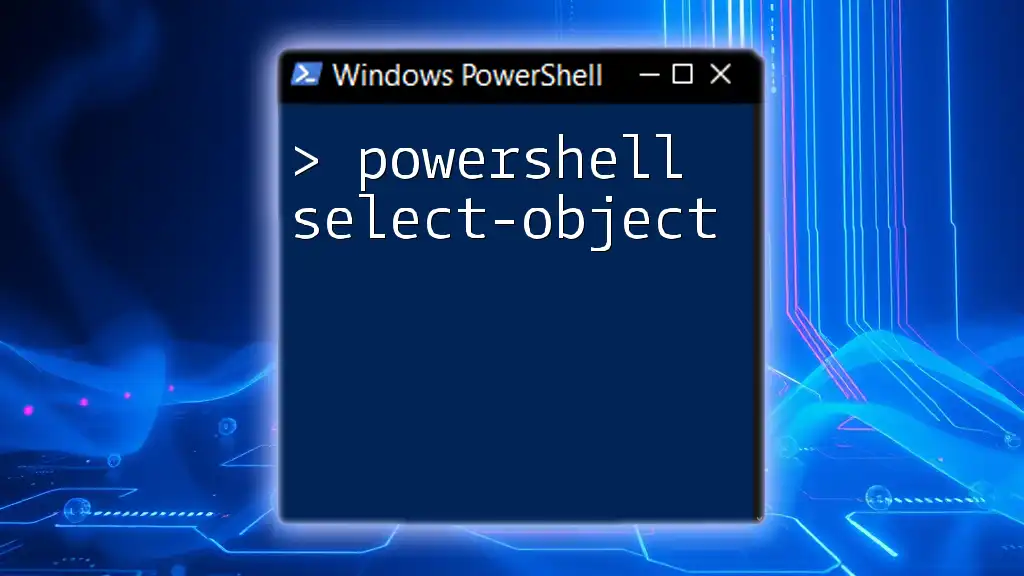
Working with XML in PowerShell
Reading XML Content
Once you have loaded your XML file, you can access its contents.
To read specific nodes, you can target elements directly:
$xml = [xml](Get-Content "C:\path\to\your\file.xml")
$xml.note.to
This command accesses the `<to>` element within the `<note>` structure, allowing you to utilize the data as needed.
Modifying XML Content
Creating or modifying elements within the XML structure is straightforward.
For example, you can add a new element like this:
$newElement = $xml.CreateElement("newElement")
$newElement.InnerText = "This is a new element."
$xml.note.AppendChild($newElement)
This creates an element named `<newElement>` and appends it to the existing `<note>` element.
Saving Changes to XML
After making modifications to the XML document, it’s essential to save your changes. You can do this with:
$xml.Save("C:\path\to\your\modifiedfile.xml")
This command rewrites the file, reflecting any updates made during your session.
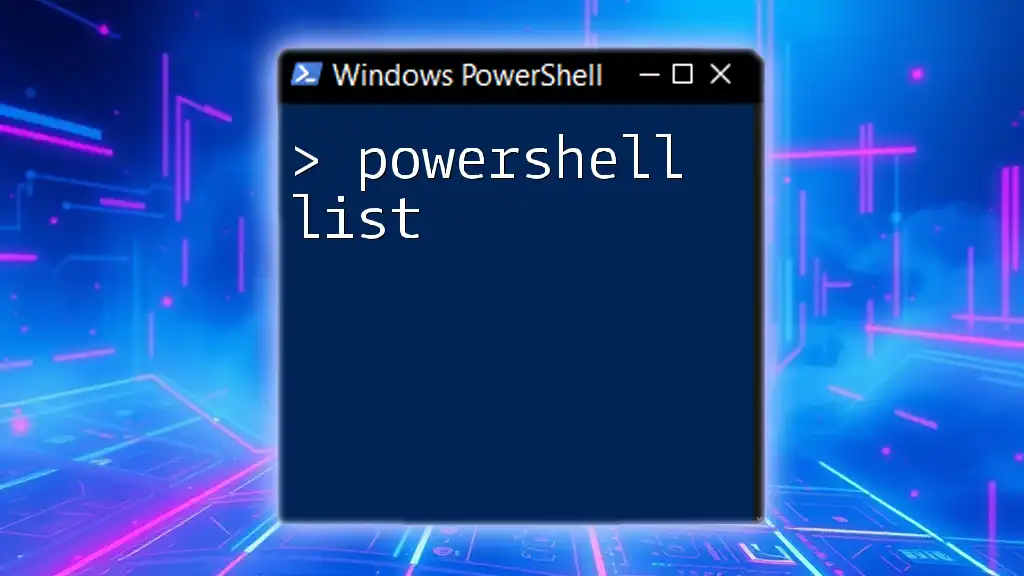
Advanced XML Manipulation Techniques
Querying XML Data with XPath
XPath (XML Path Language) is a powerful way to navigate through elements and attributes in an XML document.
You can use XPath expressions in PowerShell to query XML data efficiently. For example:
$nodes = $xml.SelectNodes("//to")
This command selects all `<to>` nodes from the XML document, enabling targeted data retrieval.
Transforming XML with XSLT
XSLT (eXtensible Stylesheet Language Transformations) allows you to transform XML documents into other formats, such as HTML. This is useful for display purposes or converting data for different applications.
In PowerShell, you can apply XSLT as follows:
$xslt = New-Object System.Xml.Xsl.XslCompiledTransfomer
$xslt.Load("C:\path\to\your\stylesheet.xsl")
$xslt.Transform($xml, $null, "C:\path\to\output.html")
This code snippet loads a specified XSLT stylesheet and transforms the XML document into an HTML file, showcasing the versatility of PowerShell in managing and converting XML data.
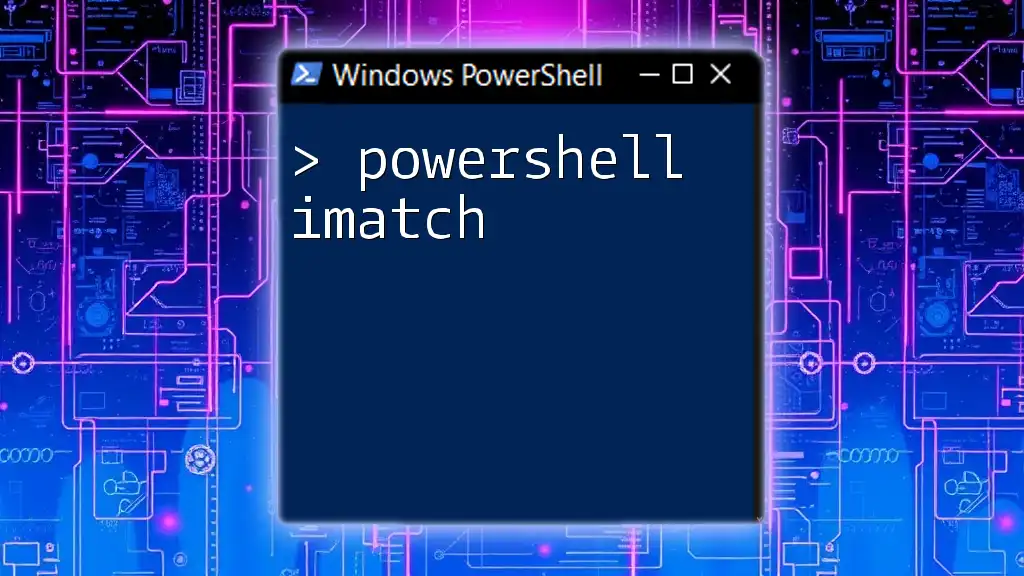
Error Handling and Best Practices
Common Errors with XML in PowerShell
When working with XML in PowerShell, you may encounter various issues. Common errors can arise from:
- Malformed XML: Ensure your XML files are well-formed with proper opening and closing tags.
- Data types: Be cautious with data types when manipulating elements or attributes, as mismatches can lead to errors.
- Path issues: Verify the file paths to avoid file not found errors.
Best Practices for XML Management
To maximize efficiency and maintain XML readability, consider the following best practices:
- Consistent formatting: Use indentation and spacing to enhance the visibility of the XML structure.
- Validating XML structure: Regularly validate your XML files to catch errors early in the process.
- Backup original files: Before making modifications, ensure you back up your original XML files to avoid data loss.
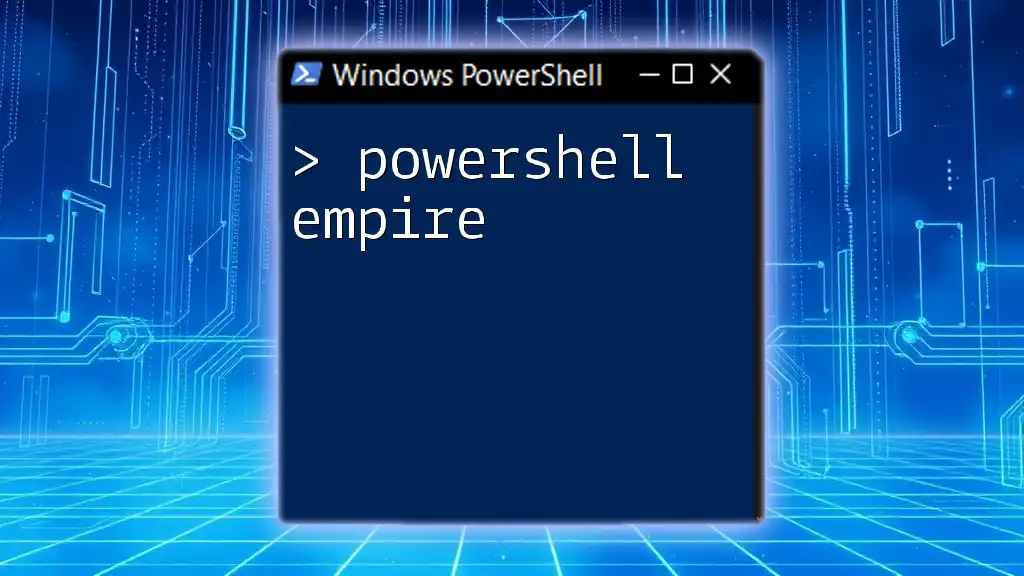
Conclusion
Mastering PowerShell XML manipulation can greatly enhance your ability to handle data in various applications—whether in automation scripts, web services, or configuration management. By implementing the skills outlined in this guide, you can navigate XML documents more effectively and expand your automation toolkit.
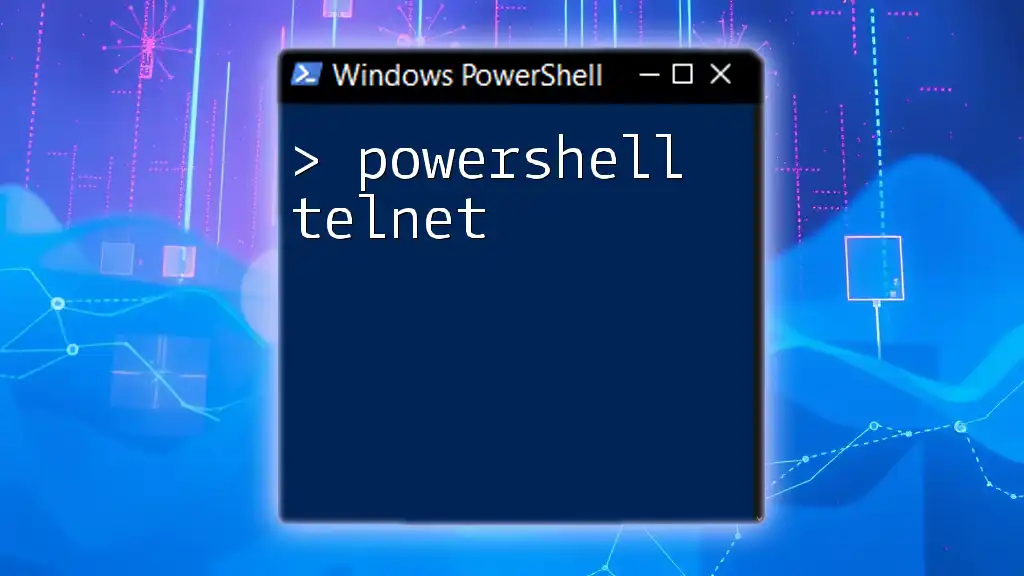
Additional Resources
For further learning, consider exploring recommended books on PowerShell scripting, enrolling in online courses, and participating in community forums focused on PowerShell and XML. The official PowerShell documentation is also a helpful resource for commands and detailed explanations of working with XML.