Sure! The "PowerShell New-Service" command is used to create a new Windows service that can run applications in the background.
Here’s a quick example using a PowerShell code snippet:
New-Service -Name "MyService" -BinaryPathName "C:\Path\To\Your\Application.exe" -DisplayName "My Custom Service" -StartupType Automatic
Understanding Services in Windows
What are Windows Services?
Windows services are specialized applications that run in the background, allowing various tasks to continue without user intervention. They are primarily designed to facilitate system functions, automatically starting at boot time or on demand. Unlike traditional applications, services can operate without a direct user interface, enabling them to run even when no user is logged into the system.
Common examples of Windows services include the Windows Update Service, which manages system updates, and the Print Spooler, responsible for handling print jobs.
Why Use PowerShell for Service Management?
PowerShell provides a powerful framework for managing Windows services efficiently. The use of scripts allows for automation, reducing the manual labor involved with repetitive service management tasks. This increases consistency across operations and minimizes the risk of human error. With PowerShell's robust command structure, IT professionals can automate complex service workflows, leading to more efficient systems management.
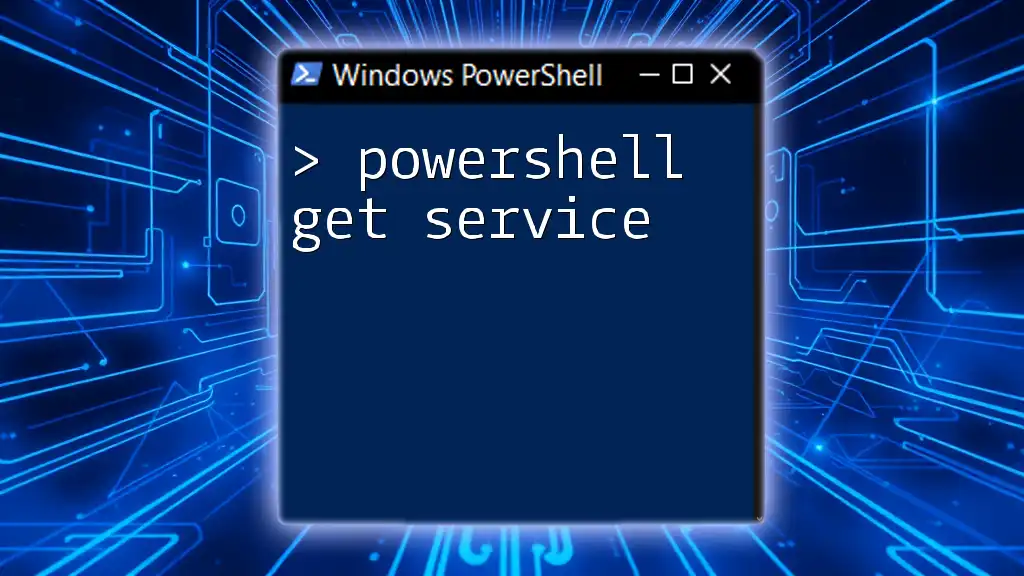
Getting Started with New-Service in PowerShell
Syntax of the New-Service Command
The `New-Service` command in PowerShell enables the creation of a new Windows service directly through the command line. Its basic syntax consists of the command followed by a series of parameters that define the service's characteristics. Understanding how to use these parameters is critical for effective service management.
New-Service -Name "<ServiceName>" -BinaryPathName "<PathToExecutable>" [-DisplayName "<DisplayName>"] [-Description "<Description>"] [-StartupType <StartupType>]
Basic Example of Creating a New Service
Creating a new service involves providing essential parameters that define its identity and behavior. Here’s a straightforward example:
New-Service -Name "MyService" -BinaryPathName "C:\PathToYourExecutable.exe"
In this example:
- -Name specifies the identifier for the service.
- -BinaryPathName indicates where the executable that runs the service is located.
This command sets up a basic service, but adding more characteristics can enhance its utility further.
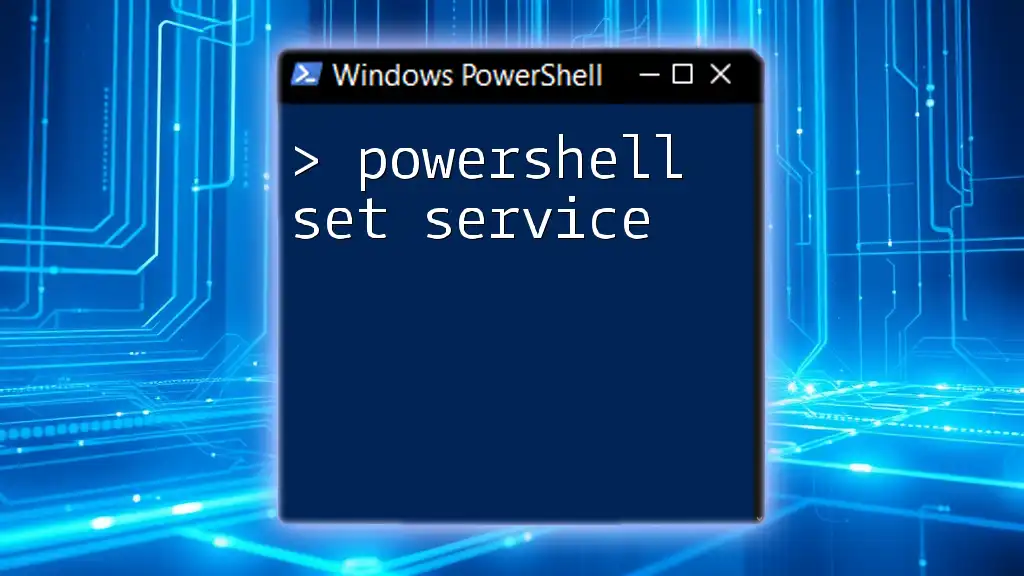
Key Parameters of New-Service
Mandatory Parameters
-
-Name: This parameter is crucial as it defines the service's unique name. It's important to follow Windows naming conventions since choosing an existing service name will lead to errors.
-
-BinaryPathName: It specifies the exact path to the executable that the service will run. This must be accurate; otherwise, the service will fail to start.
Optional Parameters
-
-DisplayName: This is the user-friendly name displayed in service manager interfaces. It can be different from the service name for clarity.
-
-Description: A succinct summary of what the service does. This is helpful for documentation and for those who might manage the service in the future.
-
-StartupType: This parameter dictates when the service should start. Options include:
- Automatic: The service starts when the computer boots.
- Manual: The service only starts when explicitly invoked by a user or application.
- Disabled: The service cannot be started; useful for services that are not needed.
Using `-DependentServices` and Other Advanced Parameters
Setting dependencies can create structured relationships between services. For example, if one service relies on another to function correctly, configuring this can preemptively manage startup sequences:
New-Service -Name "MyDependentService" -BinaryPathName "C:\MyDependentService.exe" -DependentServices "MyService"
Here, "MyDependentService" will not start until "MyService" is up and running.
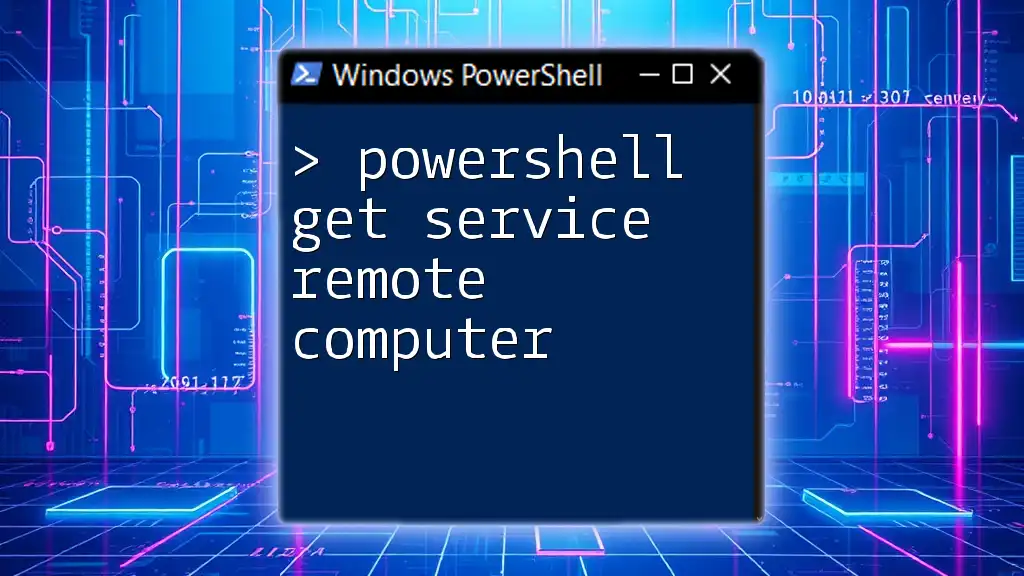
Practical Use Cases for New-Service
Example 2: Running an Application as a Service
Here’s a slightly more advanced example, where an application is configured to run as a service:
New-Service -Name "MyAppService" -BinaryPathName "C:\MyApp\MyApp.exe" -DisplayName "My Application Service" -Description "Service to run My Application" -StartupType Automatic
In this case:
- The service is set to run automatically upon boot, enhancing operational efficiency.
Example 3: Creating a Service with Dependencies
Building on the earlier concepts, here's how to configure a service with dependencies:
New-Service -Name "MyDependentService" -BinaryPathName "C:\MyDependentService.exe" -DependentServices "MyService"
This constructs a dependency, ensuring that your services operate in the correct order, which can be vital for applications that need to maintain data consistency or resource availability.
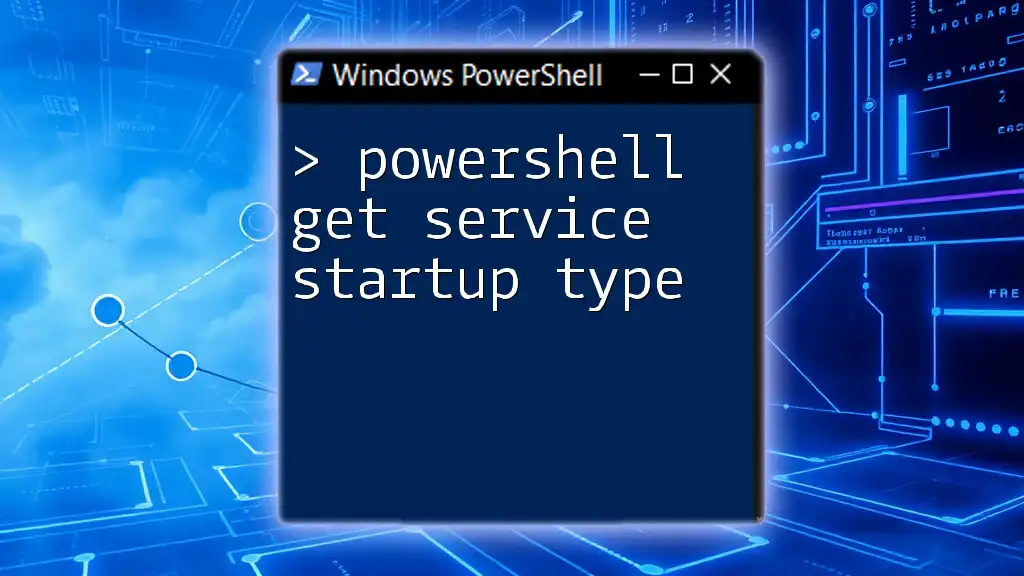
Managing New Services with PowerShell
Starting and Stopping Services
Once created, managing services is straightforward with PowerShell. Use `Start-Service` to initiate a service and `Stop-Service` to halt it:
Start-Service -Name "MyService"
Stop-Service -Name "MyService"
This allows for real-time control over your service operations, crucial for troubleshooting or managing system resources effectively.
Modifying Existing Services
If adjustments are necessary after a service has been created, `Set-Service` can be employed to modify various service properties:
Set-Service -Name "MyService" -DisplayName "Updated Service Name"
This command demonstrates how to change the display name of an existing service, aiding in clarity for administrative tasks.
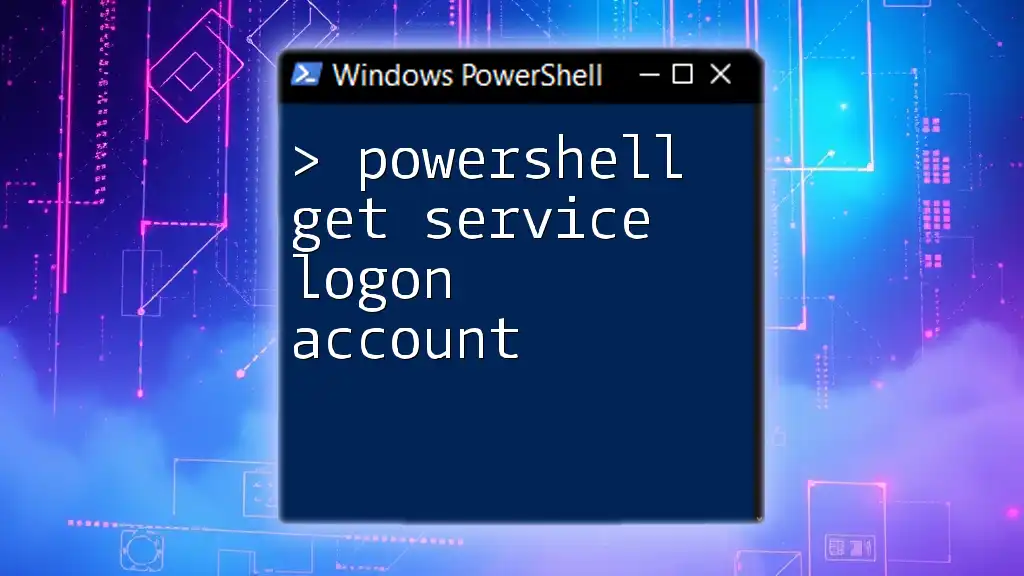
Troubleshooting Common Issues
Common Errors When Using New-Service
Working with `New-Service` can sometimes lead to common pitfalls. For example, attempting to create a service with a name that already exists will trigger an error. Before creating a new service, it's often wise to check for existing services with:
Get-Service -Name "MyService"
Logging and Error Handling in Scripts
When automating service management, incorporating logging can be invaluable. PowerShell allows for error handling using Try-Catch blocks, aiding in the resolution of issues during execution:
try {
New-Service -Name "MyService" -BinaryPathName "C:\PathToYourExecutable.exe"
} catch {
Write-Host "An error occurred: $_"
}
This ensures that potential issues are captured, allowing for smoother operations.
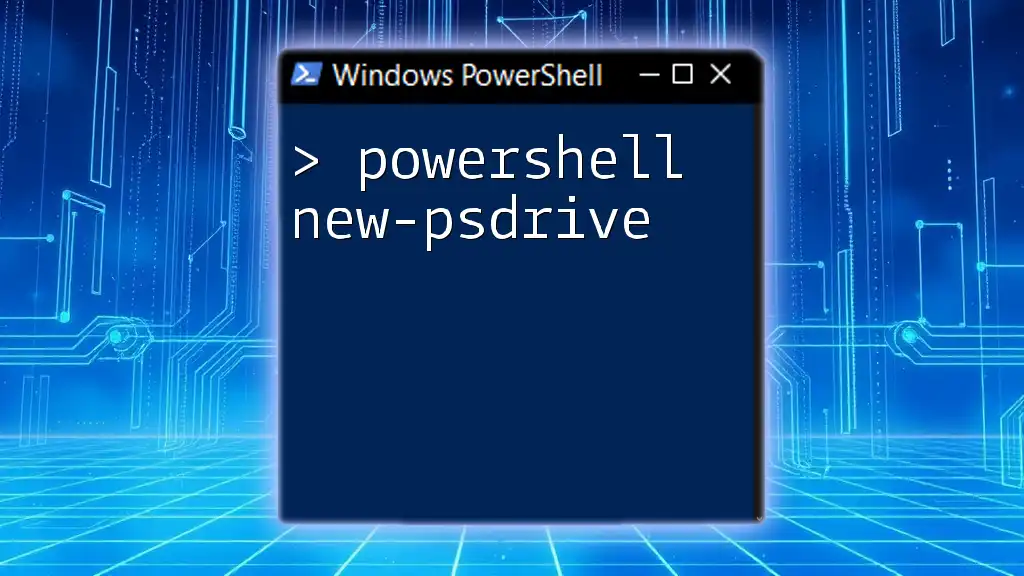
Best Practices for Service Management with PowerShell
Variables and Script Reusability
For recurring tasks, employing variables is a smart approach. This allows for easy adjustments and enhances readability:
$ServicePath = "C:\MyApp\MyApp.exe"
$ServiceName = "MyAppService"
New-Service -Name $ServiceName -BinaryPathName $ServicePath -StartupType Automatic
Documentation and Service Management Guidelines
Thorough documentation is essential. Keeping track of services, their names, purposes, and configurations can help future administrators avoid confusion and maintain efficient service management.
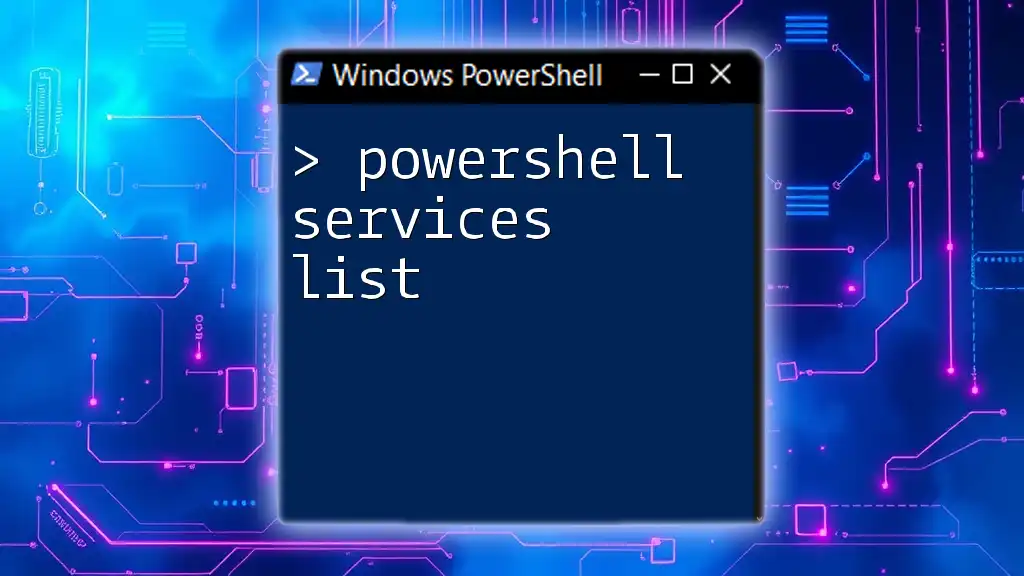
Conclusion
The `New-Service` command is a powerful tool for automating the management of Windows services through PowerShell. With the ability to create, modify, and manage services programmatically, IT professionals can enhance their productivity and improve system reliability. As you explore more commands and techniques, remember that automation is key to elevating your workflow and maximizing efficiencies in your administrative tasks.
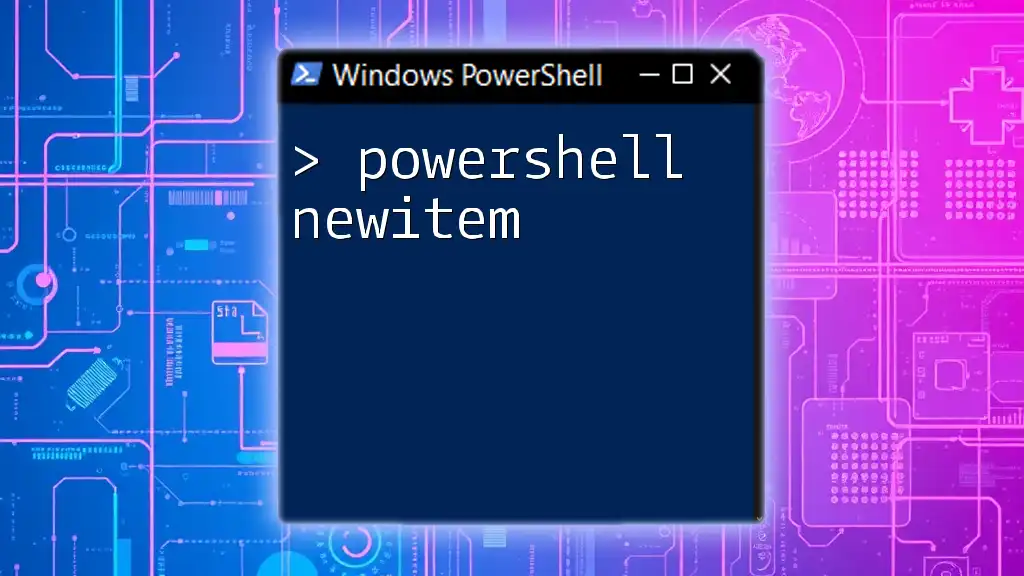
Additional Resources
For further learning, consider checking out the official PowerShell documentation, diving into recommended books, and participating in community forums to connect with fellow PowerShell enthusiasts.