The `Split-Path` cmdlet in PowerShell is used to obtain different components of a file path, such as the directory, file name, or extension, making it easier to manipulate and work with file path strings.
Here’s a code snippet demonstrating its use:
$path = "C:\Users\Example\Documents\file.txt"
$directory = Split-Path $path -Parent
$fileName = Split-Path $path -Leaf
$fileExtension = Split-Path $path -Extension
Write-Host "Directory: $directory"
Write-Host "File Name: $fileName"
Write-Host "File Extension: $fileExtension"
Understanding the Split-Path Cmdlet
PowerShell's `Split-Path` cmdlet is an invaluable tool for manipulating file paths. It allows users to parse and extract different components of a path, making file management tasks more efficient. In this section, we will explore the syntax and parameters that make `Split-Path` a powerful command.
Syntax of Split-Path
The basic syntax of the `Split-Path` cmdlet is as follows:
Split-Path -Path <string> [-Qualifier] [-Parent] [-Leaf] [-NoQualifier] [-PathType <Microsoft.PowerShell.Commands.PathType>]
In this syntax:
- `-Path` is the input path you want to split.
- Optional parameters like `-Qualifier`, `-Parent`, `-Leaf`, and `-NoQualifier` allow you to dictate what portion of the path you need.
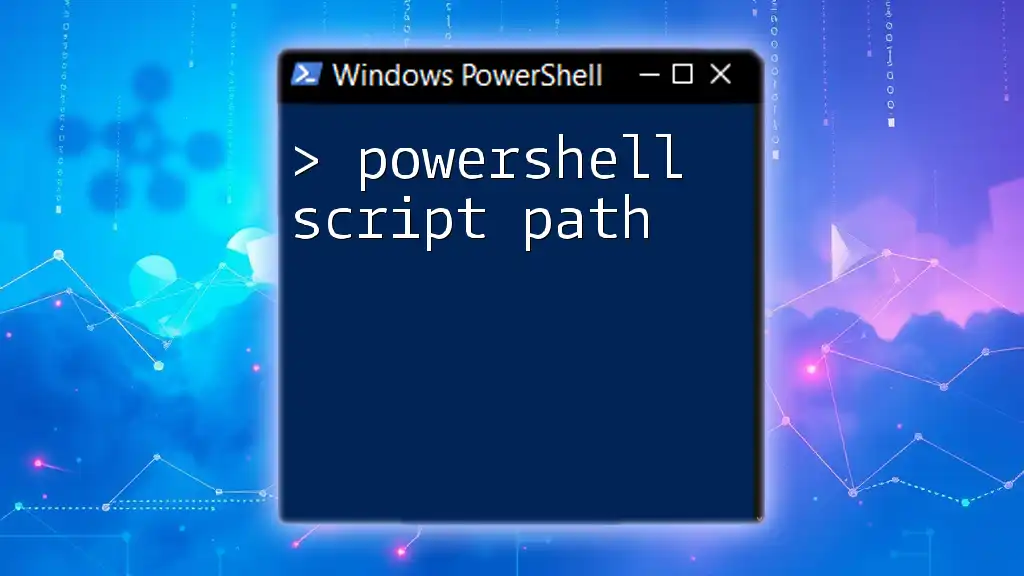
Key Components of Split-Path
The Path Parameter
The `-Path` parameter is the cornerstone of the `Split-Path` cmdlet. It accepts absolute and relative paths as input.
For example:
Split-Path -Path "C:\Folder\Subfolder\File.txt"
This command will return:
C:\Folder\Subfolder
This shows how `Split-Path` retrieves the parent directory from a full file path.
Options and Parameters
Qualifier
The `-Qualifier` parameter allows you to extract just the drive letter or UNC path from a specified path. This is useful when you need to work with multiple paths.
For instance:
Split-Path -Path "C:\Folder\Subfolder\File.txt" -Qualifier
will simply return:
C:\
Parent
When you need to find the parent directory of a file or folder, the `-Parent` parameter is your go-to option.
For example:
Split-Path -Path "C:\Folder\Subfolder\File.txt" -Parent
This command outputs:
C:\Folder\Subfolder
This highlights how `Split-Path` helps you navigate the hierarchy of your file system effortlessly.
Leaf
If you're interested primarily in the name of the file or last element in a path, the `-Leaf` parameter will be invaluable.
Consider this example:
Split-Path -Path "C:\Folder\Subfolder\File.txt" -Leaf
This returns:
File.txt
NoQualifier
The `-NoQualifier` parameter retrieves the path without the qualifier, streamlining the output when you want to focus on the path structure.
For example:
Split-Path -Path "C:\Folder\Subfolder\File.txt" -NoQualifier
This will yield:
Folder\Subfolder\File.txt
PathType
Lastly, the `-PathType` parameter is useful for defining whether the specified path is a container or a leaf. This can help in decision-making processes, ensuring you're operating on the correct type of path.
Here's an example:
Split-Path -Path "C:\Folder" -PathType Container
If the path indeed refers to a container, it will proceed accordingly, aiding in the efficiency of your scripts.
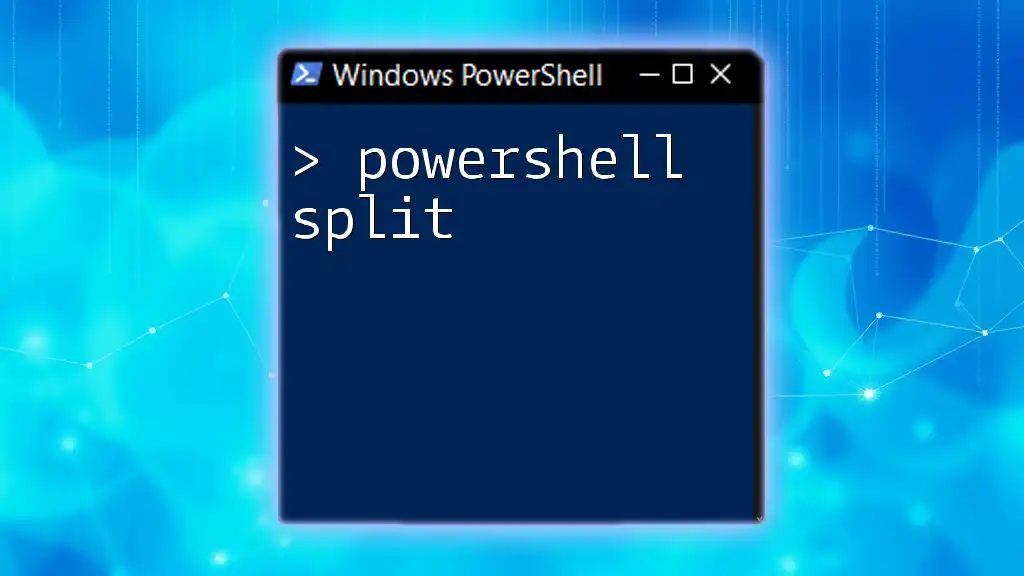
Practical Use Cases for Split-Path
File Management
When managing files through scripts, `Split-Path` can play a central role. For instance, if you’re iterating through files in a directory and need to sort them based on their parent directory, you can implement `Split-Path` seamlessly.
Here’s a sample script that organizes files:
$files = Get-ChildItem -Path "C:\MyFiles"
foreach ($file in $files) {
$parent = Split-Path -Path $file.FullName -Parent
# Further file management logic...
Write-Host "The parent directory of $($file.Name) is $parent"
}
This script lists all files in a designated folder and prints out their respective parent directories, allowing for easy file tracking and management.
Dynamic Path Handling
With `Split-Path`, dynamic path handling becomes an accessible task. If you're writing scripts that need to adapt based on the path structure, `Split-Path` helps create efficiency by allowing manipulation of paths without hardcoding values.
Example:
$basePath = "C:\MyProject\"
$fileName = "Report.docx"
$fullPath = Join-Path -Path $basePath -ChildPath $fileName
$parent = Split-Path -Path $fullPath -Parent
Write-Host "The full path is $fullPath and the parent is $parent"
This demonstrates how `Split-Path` can adapt to generate outputs based on varying inputs.
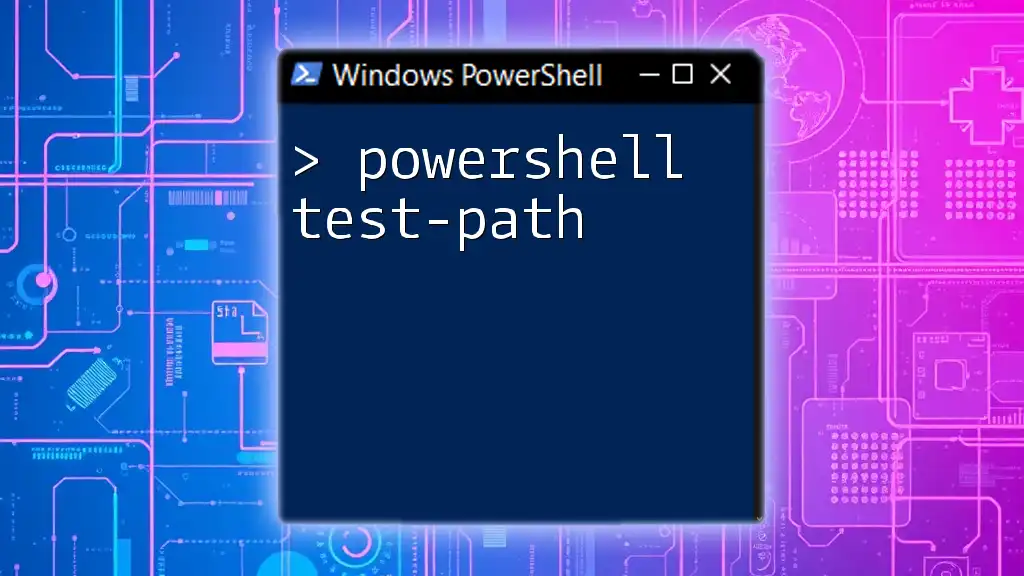
Best Practices for Using Split-Path
One fundamental best practice is to maintain clarity and consistency in your paths. This makes it easier to debug and enhances readability. Always test paths before executing operations, employing the `Test-Path` cmdlet to ensure that you’re working with valid paths.
Example:
if (Test-Path -Path $fullPath) {
Write-Host "The path is valid."
} else {
Write-Host "The path does not exist!"
}
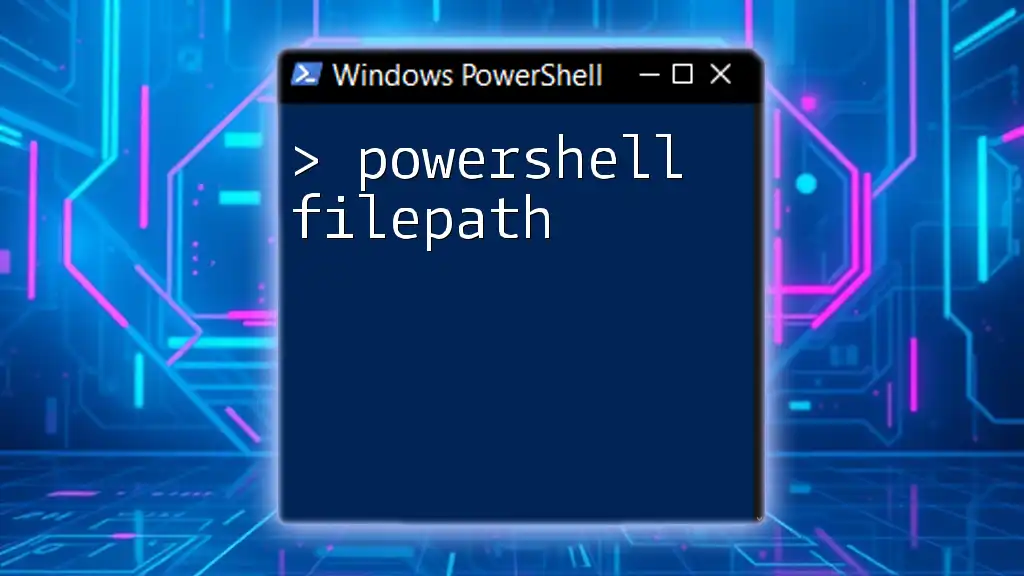
Troubleshooting Common Issues
Incorrect Path Format
One common pitfall when using `Split-Path` is entering an incorrect path format, which can lead to unexpected outputs or errors. Always ensure that the path exists and is well-formed.
Understanding Output Types
Understanding the types of data `Split-Path` returns is crucial. For instance, `Split-Path` can yield strings that represent different path components, so be prepared to handle these returns properly in your scripts.
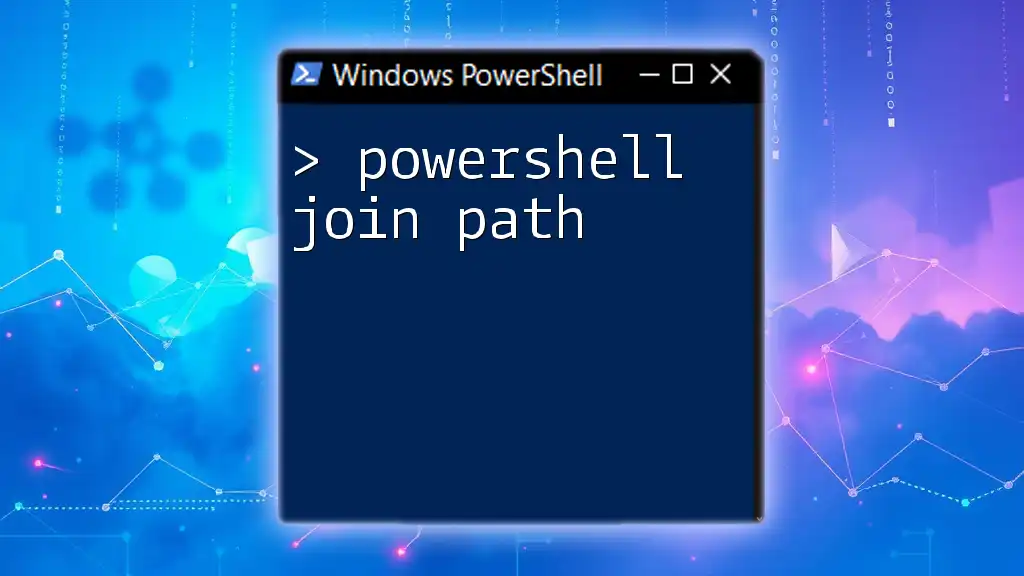
Conclusion
In conclusion, mastering PowerShell Split Path is vital for efficient file management and path manipulation. With the ability to extract and manipulate path components easily, you can streamline your scripts and improve your workflows. The practical examples provided will not only aid your understanding but also encourage you to explore the `Split-Path` cmdlet further for all your file handling needs. Be sure to practice and apply this knowledge for enhanced PowerShell scripting skills.
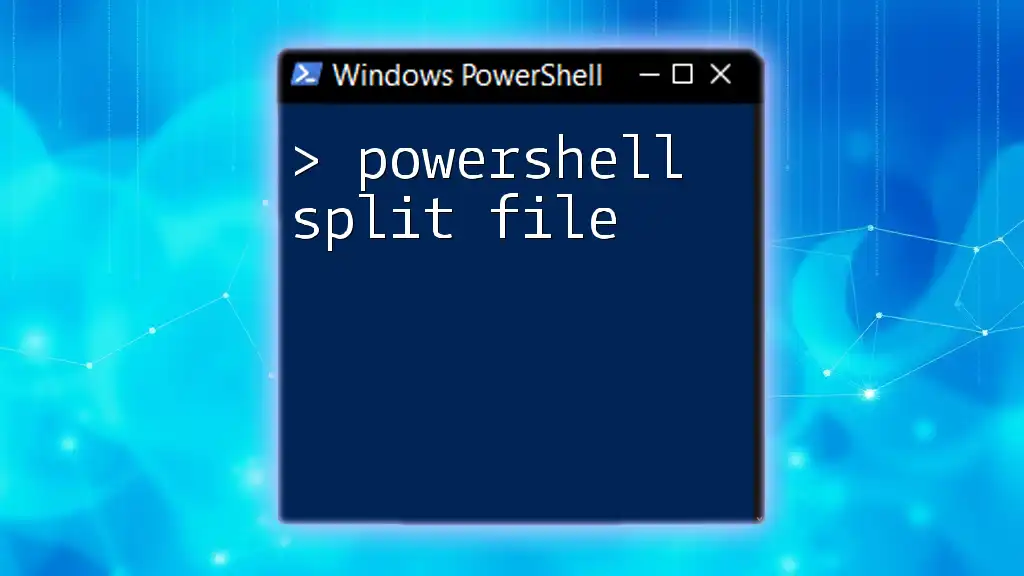
Additional Resources
For those looking to delve deeper into `Split-Path`, consider checking out the official Microsoft documentation that provides extensive insight and advanced usage patterns. Exploring related PowerShell cmdlets can also broaden your scripting toolbox, making you a more proficient user overall.