In PowerShell, you can split a string into an array by using the `-split` operator with a newline character to separate each line as demonstrated in the following code snippet:
$lines = "Line1`nLine2`nLine3" -split "`n"
Understanding Newlines in PowerShell
What is a Newline?
A newline is a special character in text files that signals the end of a line. In PowerShell, the most common newline characters are:
- LF (Line Feed), represented as `\n` (ASCII 10).
- CRLF (Carriage Return + Line Feed), represented as `\r\n` (ASCII 13 followed by ASCII 10).
Understanding these newline characters is crucial, especially when dealing with text data from various sources like text files or log files. Each operating system may use a different newline character, so knowing how to handle them is key to effective string manipulation in PowerShell.
Importance of Newlines in String Manipulation
Newlines serve as delimiters in many contexts, separating lines of data or output. For example, when reading configuration files, log files, or when parsing multi-line strings, correctly managing newlines becomes essential. Imagine encountering a script that loads a multi-line configuration but mishandles the newlines, leading to errors—this underlines the importance of mastering how to split strings on newlines in PowerShell.
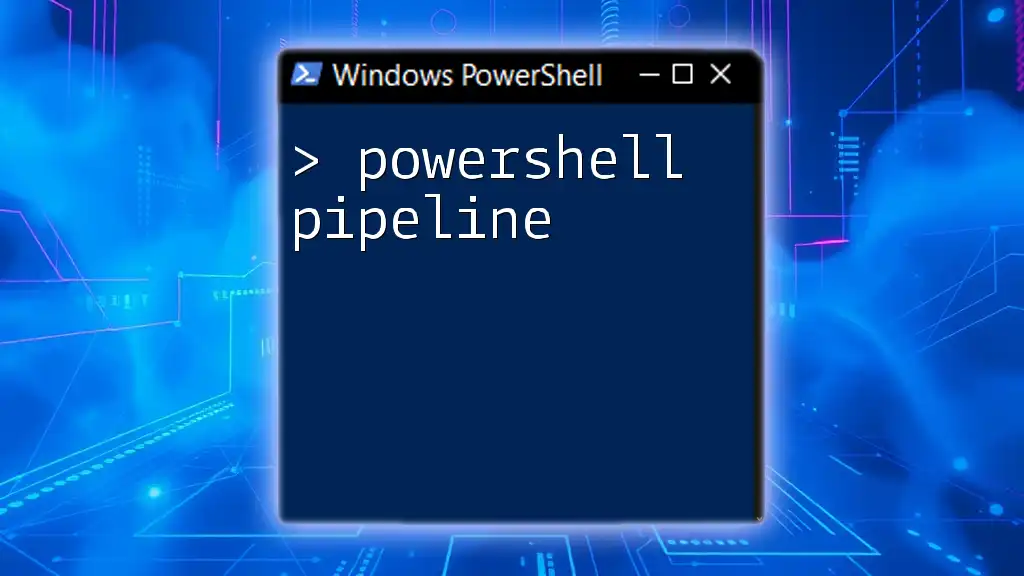
PowerShell String Splitting Basics
The Split Method
PowerShell's `Split()` method is a fundamental way to divide a string into an array using specified delimiters. The basic syntax is as follows:
$string.Split("delimiter")
In the case of splitting on newlines, we can directly input the newline character into the `Split()` method.
Using the Split Method on Newlines
To split a string on newlines, you can do the following:
$text = "Line1`nLine2`nLine3"
$result = $text.Split("`n")
This straightforward code utilizes the newline character (` `n `) to divide the content into an array. After execution, `$result` will hold the following values:
- Line1
- Line2
- Line3
Explanation of the Code
Here, we define a multi-line string stored in the variable `$text`. When we call `Split()`, PowerShell processes each line separated by the newline character, effectively breaking the string into individual components. This is a foundational technique and one of the simplest ways to deal with strings organized in lines.
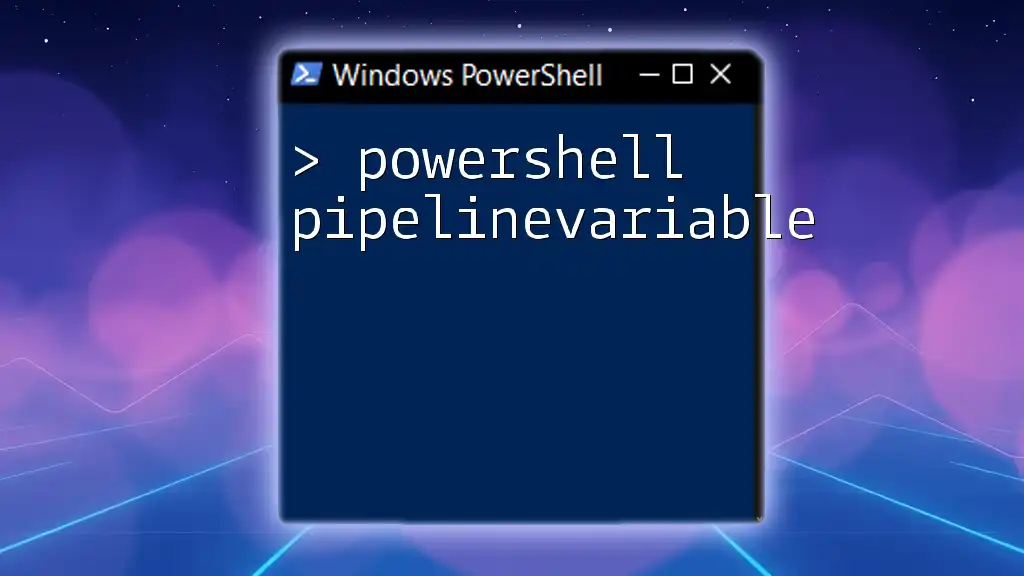
Advanced Techniques for Splitting on Newlines
Using Regular Expressions
For more complex scenarios, such as strings with mixed newline formats, PowerShell allows you to utilize regular expressions (regex) to effectively split strings. Regex provides a powerful way to define string patterns.
To implement regex for newline splitting, you would use:
$multiLineText = "Line1`r`nLine2`r`nLine3"
$result = [regex]::Split($multiLineText, "\r?\n")
Explanation of the Regex Code
In this snippet, the regex pattern `\r?\n` matches both CRLF and LF newline characters. The `?` quantifier signifies that the preceding character (`\r`) may occur zero or one time, enabling the regex to effectively handle both newline scenarios. This flexibility is particularly valuable when working with strings from different operating systems.
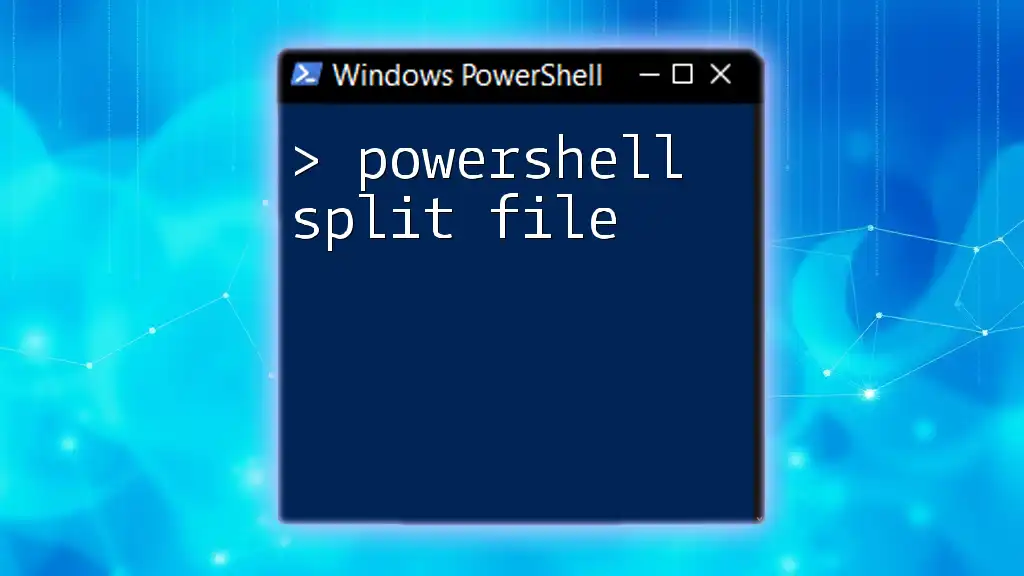
Handling Different Newline Characters
Distinctions between LF and CRLF
It's crucial to differentiate between LF (`\n`) and CRLF (`\r\n`), as using the wrong character may yield unexpected results when trying to split strings. Recognizing and accounting for these differences ensures that your scripts behave as intended, providing accurate output regardless of the source.
Code Snippet for Handling Both Types
To robustly split strings that may contain both types of newlines, you can make use of the `-split` operator:
$text = "Line1`rLine2`nLine3"
$result = $text -split "(`r`n|`n|`r)"
Explanation of the Code Snippet
In this example, we utilize the `-split` operator featuring a regex pattern `"(`r`n|`n|`r)"`, which denotes alternate possibilities for splitting. This means the code can handle combinations of CRLF, LF, and even CR newline characters, which is particularly useful when dealing with text sourced from different environments.
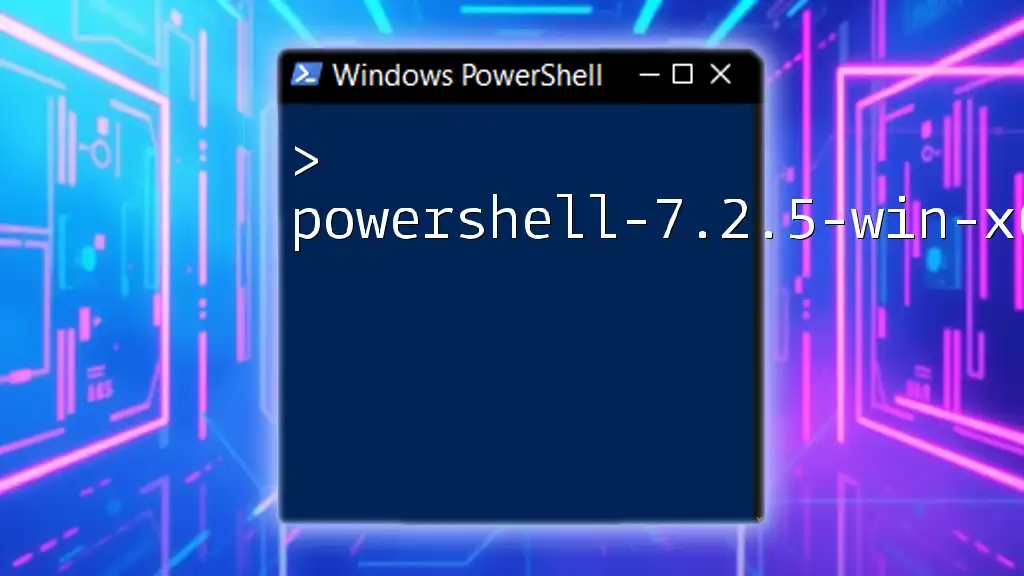
Practical Applications
Splitting Files into Arrays
Consider a scenario where you need to read a text file and process its content line by line. PowerShell provides a seamless way to achieve this:
$fileContent = Get-Content "C:\path\to\file.txt"
$lines = $fileContent -split "`n"
This code reads the content of a text file and splits it into an array `$lines`, where each element corresponds to a line in the file.
Explanation of File Handling
When working with large files, properly splitting text can facilitate efficient processing, enabling scripts to iterate over each line, extract data, or make modifications without overwhelming memory resources.
Common Use Cases in PowerShell Scripts
Splitting strings is prevalent in many PowerShell scripting scenarios, such as:
- Parsing Logs: When analyzing log files, being able to split entries by lines allows for easier searching and categorization.
- Formatting Output: Creating readable output by breaking up long strings into manageable segments helps users visually parse data.
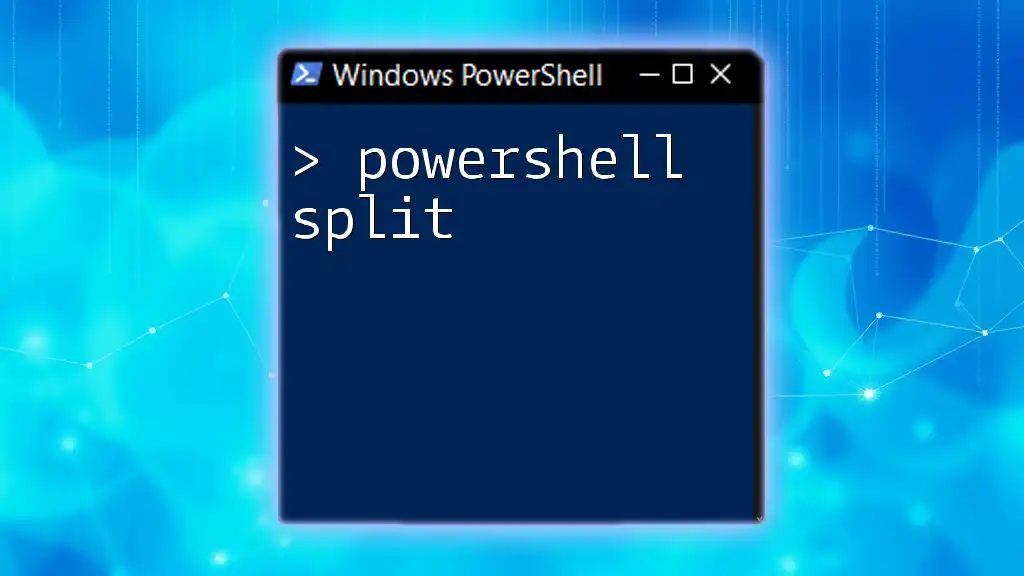
Troubleshooting Common Issues
Common Mistakes to Avoid
While working with string splitting, it's common to overlook newline characteristics. A frequent mistake is assuming all text files follow the same newline structure. Not considering this can lead to incomplete data processing.
Tips for Debugging Split Operations
To effectively troubleshoot, the use of `Write-Host` or `Write-Output` to confirm the contents of your array after a split operation is advisable:
$lines | ForEach-Object { Write-Host $_ }
This enables you to visualize each line and confirm that the string has been correctly split as intended.
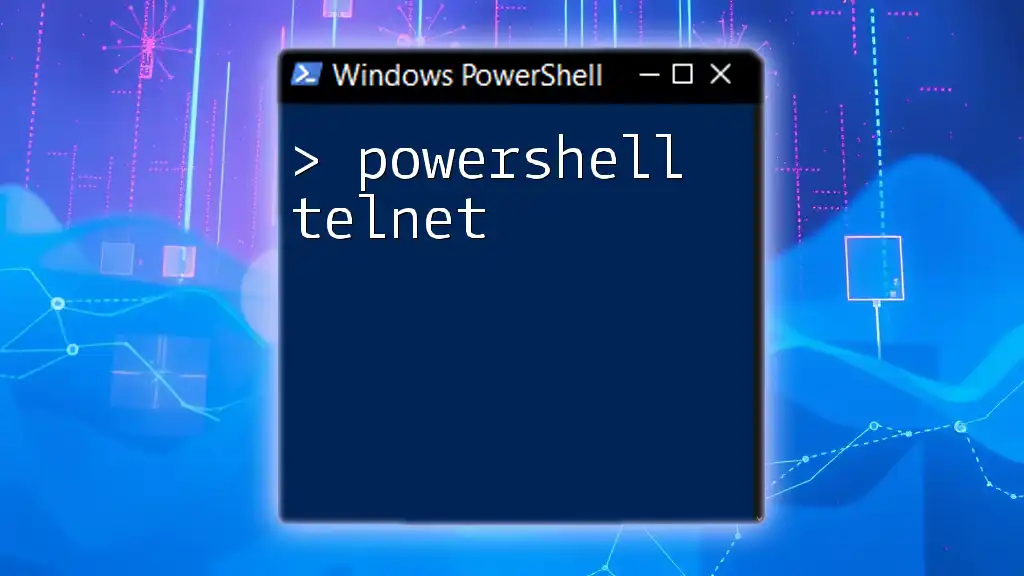
Conclusion
Mastering how to perform a PowerShell split on newline is an essential skill that enhances your scripting capabilities. By understanding how to manipulate strings effectively, you can streamline data processing and ensure your scripts handle text inputs robustly. Engaging with these techniques will empower you to wield PowerShell with greater confidence and efficiency.
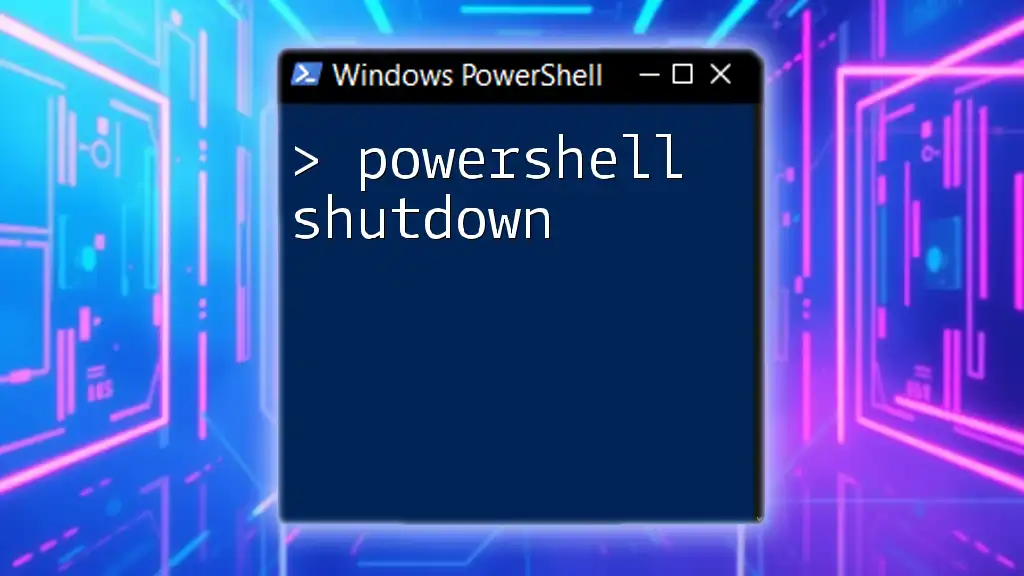
Additional Resources
To further enhance your PowerShell string manipulation skills, consider exploring the official PowerShell documentation on string handling and regex, as well as recommended books and online courses that dive deeper into this critical scripting language.