To run a PowerShell script with parameters, you can pass the desired arguments directly in the command line, allowing your script to execute with customized inputs.
.\YourScript.ps1 -Parameter1 "Value1" -Parameter2 "Value2"
What Are PowerShell Parameters?
Definition and Importance
In PowerShell, parameters allow you to pass data into scripts and functions, making them more dynamic and flexible. Parameters increase the reusability of scripts, allowing the same logic to be executed with varying inputs.
Types of Parameters
Positional Parameters
Positional parameters are defined by their order in the command. When invoking a script, PowerShell expects arguments to be supplied in the sequence defined in the script.
For example, consider the following script:
param(
[string]$FirstName,
[string]$LastName
)
Write-Host "Hello, $FirstName $LastName!"
You would call the script with:
.\MyScript.ps1 "John" "Doe"
In this scenario, "John" is the first argument (`$FirstName`), and "Doe" is the second (`$LastName`).
Named Parameters
Named parameters allow you to specify which argument corresponds to which parameter, regardless of order. This method improves readability and reduces the possibility of confusion.
Using the previous example, you could execute it like this:
.\MyScript.ps1 -LastName "Doe" -FirstName "John"
Notice how the parameters are invoked with their names, making it clearer what each value represents.
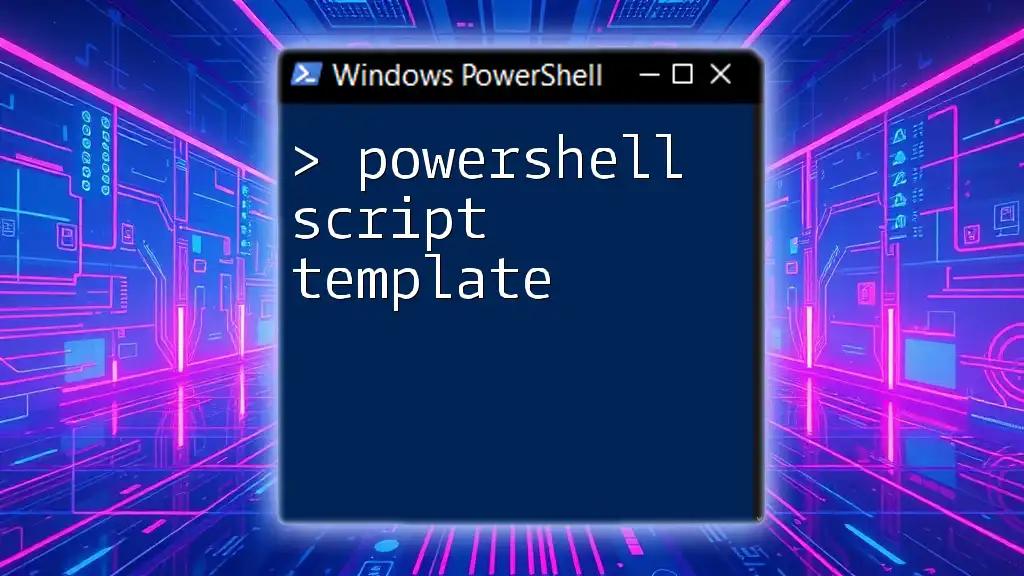
Creating a PowerShell Script with Parameters
Step-by-Step Guide
To create a PowerShell script with parameters, you need to open a text editor and define the parameters using the `param` block at the top of your script.
Using the `param` Block
The `param` block is where you declare the parameters your script will accept. This block should be placed at the beginning of your script for best practices.
param(
[int]$Age,
[string]$City
)
Write-Host "You are $Age years old and live in $City."
Example Script
Here is a more comprehensive script that showcases multiple parameters:
param(
[string]$UserName,
[string]$Email,
[int]$UserAge
)
Write-Host "User Info: "
Write-Host "Name: $UserName"
Write-Host "Email: $Email"
Write-Host "Age: $UserAge"
This script could be executed by specifying the parameters like so:
.\UserInfo.ps1 -UserName "Alice" -Email "alice@example.com" -UserAge 30
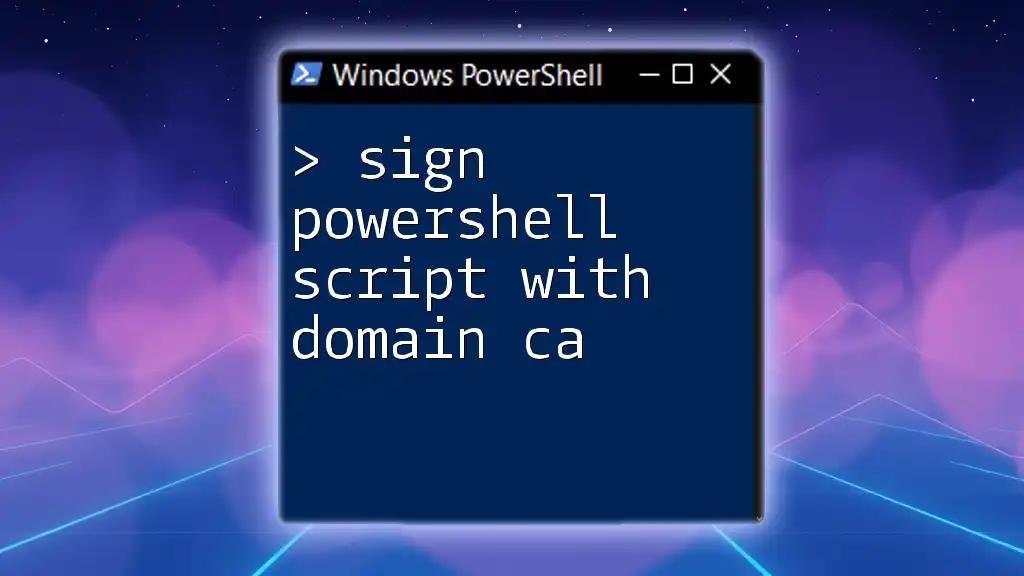
Running PowerShell Scripts with Parameters
Command Syntax
To run a PowerShell script with parameters, the basic command structure is as follows:
.\ScriptName.ps1 -ParameterName ParameterValue
Execute PowerShell Script with Parameters
Let’s break down how to execute a script using both positional and named parameters.
For positional parameters, refer to the earlier example with `UserName`, `Email`, and `UserAge`. This can be run like this:
.\UserInfo.ps1 "Alice" "alice@example.com" 30
For named parameters, the command becomes clearer:
.\UserInfo.ps1 -UserName "Alice" -Email "alice@example.com" -UserAge 30
Troubleshooting Common Issues
When running scripts with parameters, users might encounter common issues such as:
-
Parameter Type Mismatches: Ensure that the data type of the passed argument matches the type defined in the script. For instance, if an integer is expected but a string is provided, PowerShell will throw an error.
-
Handling Empty Parameters: If a parameter is not supplied and hasn't been given a default value, it may cause unintended behavior. Always check for required parameters and handle defaults where applicable.
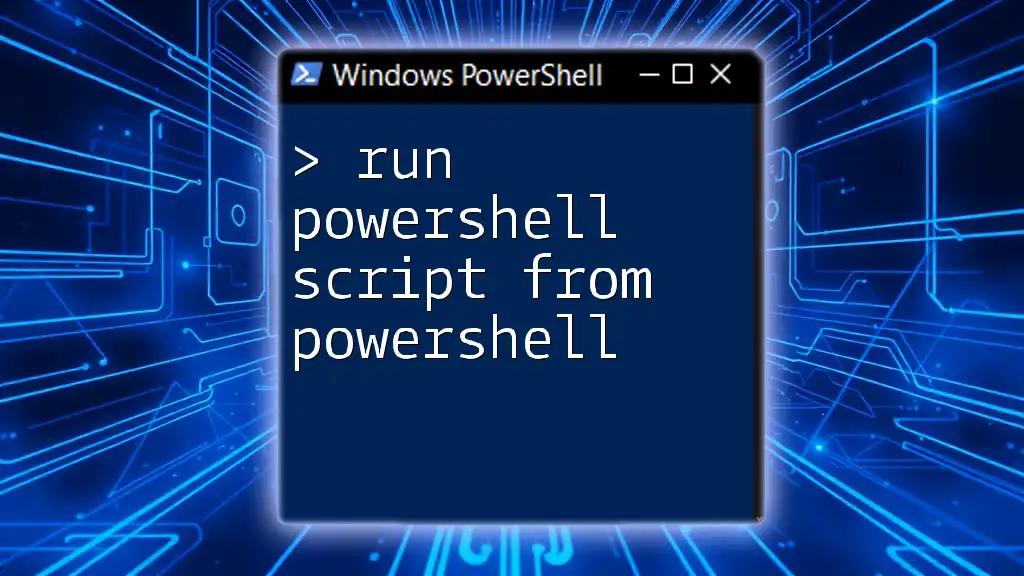
Best Practices for Using Parameters in PowerShell
Clear Naming Conventions
Using clear and descriptive names for parameters enhances the readability of your scripts. For instance, prefer `$UserAge` over `$a`. Clear names help others (and your future self) understand what each parameter signifies.
Type Validation
Incorporate type validation to prevent errors at runtime. This ensures that your script receives the data type it expects, which can significantly enhance reliability.
param(
[string]$UserName,
[int][ValidateRange(1, 120)]$UserAge
)
Here, if a user attempts to pass an integer outside the range of 1 to 120, PowerShell will raise an error.
Default Values
Setting default values for parameters can make your scripts more user-friendly. This allows users to omit parameters without disrupting the script.
Here’s how to set defaults:
param(
[string]$UserName = "Guest",
[int]$UserAge = 25
)
Write-Host "User: $UserName, Age: $UserAge"
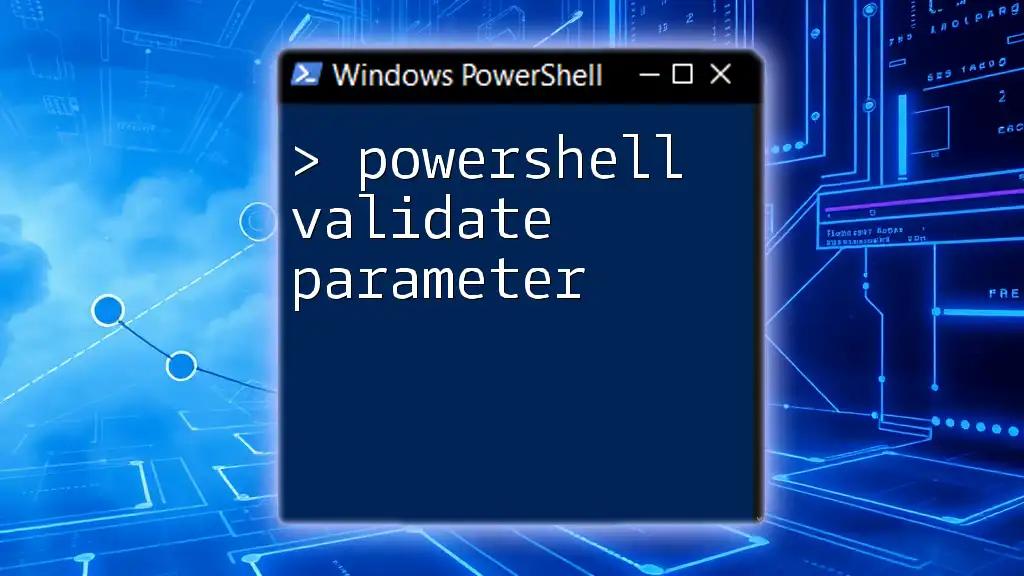
Advanced Techniques
Passing Arrays and Collections
You can also pass arrays or collections as parameters for more complex scenarios. Here's an example script that accepts an array of numbers:
param(
[int[]]$Numbers
)
foreach ($number in $Numbers) {
Write-Host "Processing number: $number"
}
Invoke it with:
.\ProcessNumbers.ps1 -Numbers 1, 2, 3, 4, 5
Using Parameter Sets
Parameter sets are beneficial when you want to create scripts that behave differently based on the combination of parameters provided.
Here’s an example:
param(
[Parameter(Mandatory=$true, ParameterSetName="Set1")]
[string]$Name,
[Parameter(Mandatory=$true, ParameterSetName="Set2")]
[int]$ID
)
if ($PSCmdlet.ParameterSetName -eq "Set1") {
Write-Host "Name: $Name"
} elseif ($PSCmdlet.ParameterSetName -eq "Set2") {
Write-Host "ID: $ID"
}
Parameter Attributes
Various PowerShell attributes, such as `[Alias()]` and `[Parameter()]`, can enhance functionality.
For example:
param(
[Alias("First")]
[string]$UserName,
[Parameter(Mandatory=$true)]
[int]$UserAge
)
The alias allows you to call the parameter either by `$UserName` or `$First`.
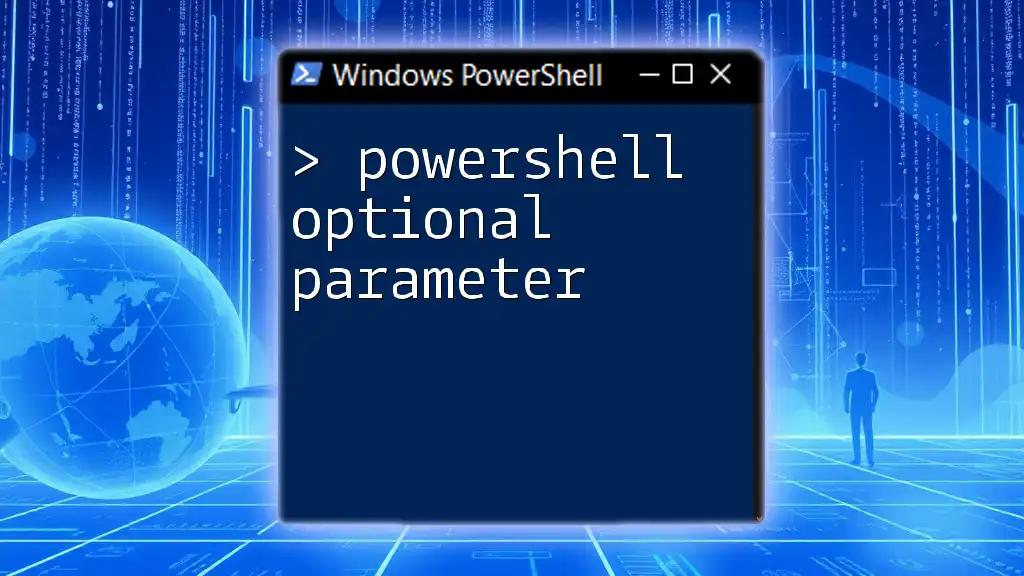
Conclusion
Incorporating parameters into your PowerShell scripts significantly increases their flexibility and usability. By understanding how to utilize different types of parameters, script creators can ensure a more dynamic scripting experience. Experimenting and practicing will develop your skills even further.
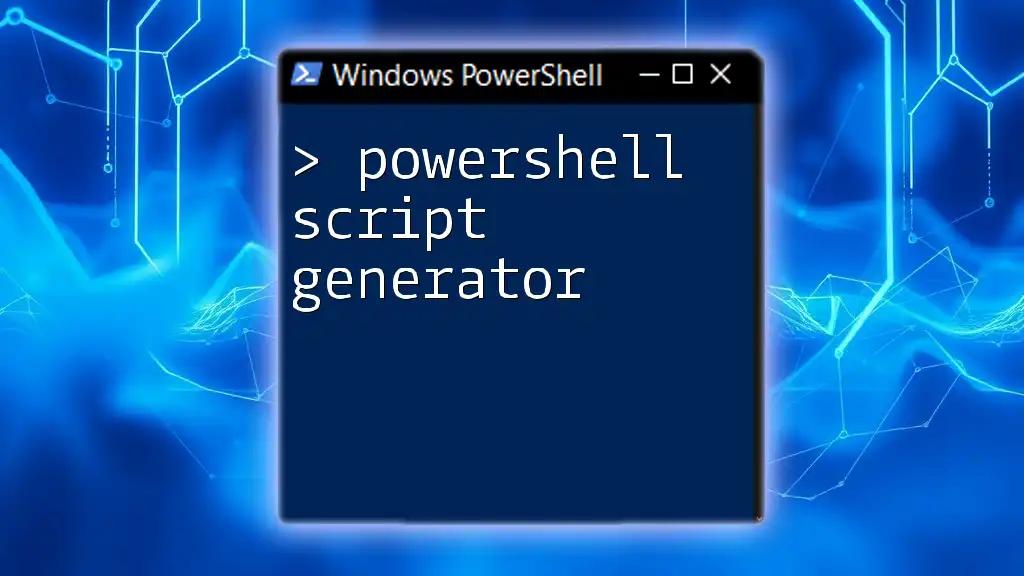
Additional Resources
For those looking to deepen their knowledge of PowerShell, consider visiting the [official Microsoft documentation](https://docs.microsoft.com/en-us/powershell/) to explore scripting topics in greater detail.
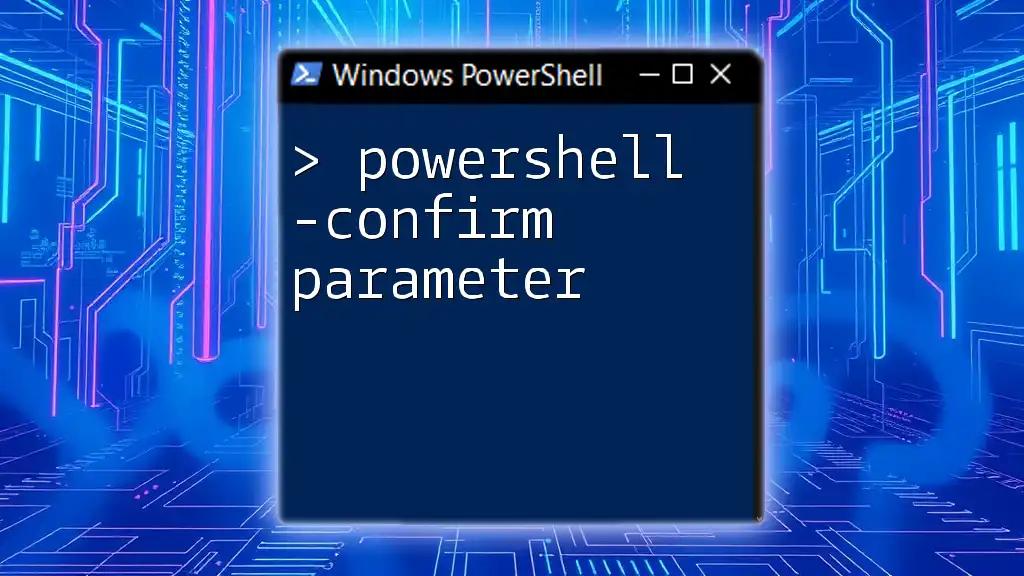
Call to Action
If you’re interested in mastering PowerShell scripting, subscribe to our content for more tutorials and updates!