To run a PowerShell script from within PowerShell, you can use the dot-sourcing operator or simply call the script by its path.
Here's a code snippet demonstrating both methods:
# Using dot-sourcing
. "C:\Path\To\YourScript.ps1"
# Alternatively, call the script by its path
& "C:\Path\To\YourScript.ps1"
Preparing to Run PowerShell Scripts
Understanding PowerShell Execution Policies
Before you can successfully run PowerShell script from PowerShell, it's crucial to understand Execution Policies. An execution policy is a safety feature that controls the conditions under which PowerShell loads configuration files and runs scripts.
Here are the common levels of Execution Policies:
- Restricted: No scripts can be run. This is the default setting.
- AllSigned: Only scripts signed by a trusted publisher can be executed.
- RemoteSigned: Scripts downloaded from the internet must be signed by a trusted publisher before they can be executed.
- Unrestricted: All scripts can be run but may prompt you for confirmation.
To check your current Execution Policy, use the following command:
Get-ExecutionPolicy
If your policy is set to Restricted, you won’t be able to run scripts.
Setting the Execution Policy
To enable script execution, you may need to change the Execution Policy. Use the following command to set it:
Set-ExecutionPolicy RemoteSigned
When changing the Execution Policy, consider the security implications. For personal or internal scripts, RemoteSigned is usually an appropriate choice.
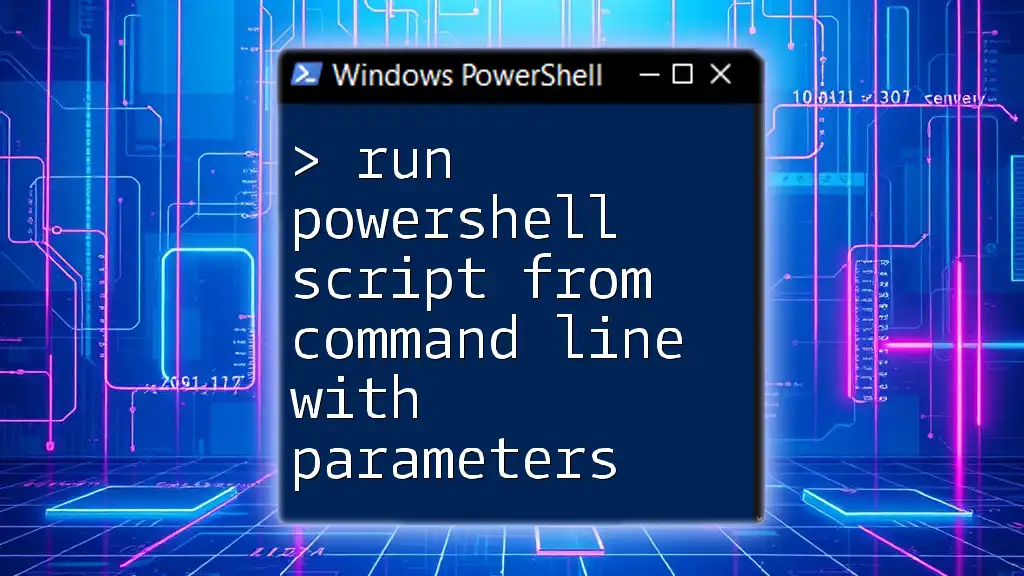
How to Run PowerShell Script from PowerShell
Basic Syntax to Execute a PowerShell Script
Running a PowerShell script involves using the script's file path. The basic structure to call a script looks like this:
.\MyScript.ps1
This command assumes that the script is located in your current directory. PowerShell identifies scripts by their .ps1 file extension.
Understanding the Current Directory in PowerShell
To ensure you are in the correct directory when executing your script, you can check your current location using:
Get-Location
This command helps you avoid common errors related to file paths.
Running Scripts with Full Path
If your script is not in the current directory, you can use the full path to run it. For instance:
C:\Scripts\MyScript.ps1
Using the full path eliminates the ambiguity of the current working directory and ensures the script executes regardless of your location in the file system.
Using the Call Operator (&)
Another powerful method to execute a PowerShell script is by using the Call Operator (&). This operator allows you to invoke a command, script, or script block. Here’s how you would use it:
& "C:\Scripts\MyScript.ps1"
Using the Call Operator is particularly useful when dealing with file paths that contain spaces, as it helps PowerShell correctly interpret the command.
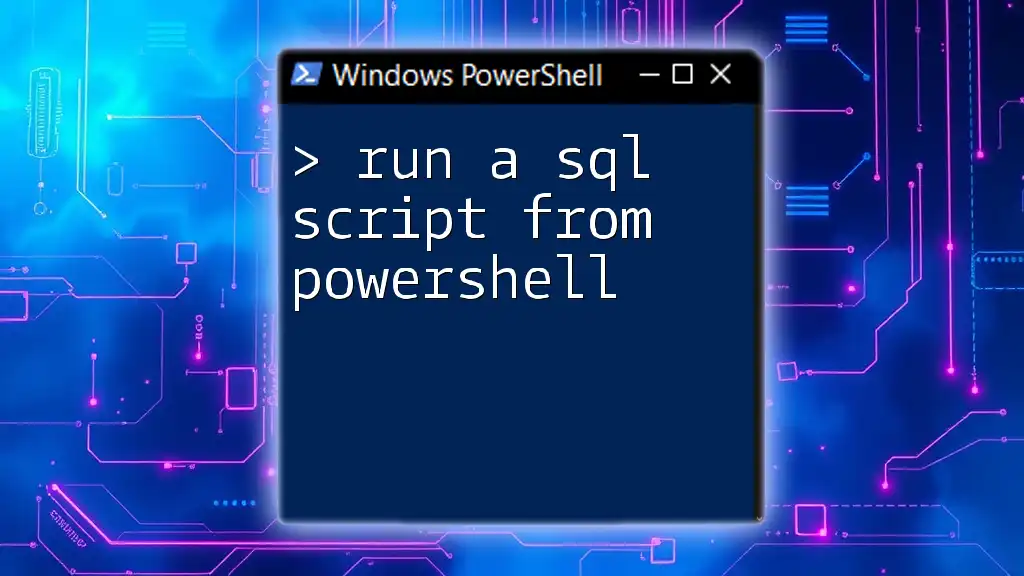
Passing Parameters to PowerShell Scripts
Definition and Importance of Parameters
Parameters are used to provide inputs to your scripts, making them flexible and reusable. For example, a script designed to send out a welcome message can accept a name as a parameter.
How to Define Parameters in Your Script
To set up parameters in your script, use the param keyword. Here's an example of a simple script:
param (
[string]$Name,
[int]$Age
)
Write-Host "Welcome $Name, you are $Age years old!"
Calling Scripts with Parameters
When running a script with defined parameters, you can pass the values directly:
.\MyScript.ps1 -Name "John" -Age 30
This command invokes the script while providing it with specific inputs. As a result, you'll receive a tailored output based on the parameters supplied.

Handling Errors When Running Scripts
Common Errors and Troubleshooting Tips
Running scripts can lead to errors, especially if the environment isn't set up correctly. Some common errors include path not found, file not found, or permission errors.
To handle these gracefully, you can implement error handling techniques using `Try`, `Catch`, and `Finally`. Here's an example script demonstrating this:
Try {
.\MyScript.ps1
} Catch {
Write-Host "An error occurred:" $_.Exception.Message
}
The above code tries to run your script and catches any exceptions that occur, providing informative error messages.
Viewing Script Output
To capture and review the output generated by your script, you can redirect it to a file. For example:
.\MyScript.ps1 | Out-File -FilePath "output.txt"
This command sends the output to a file named output.txt, allowing you to inspect the results later.
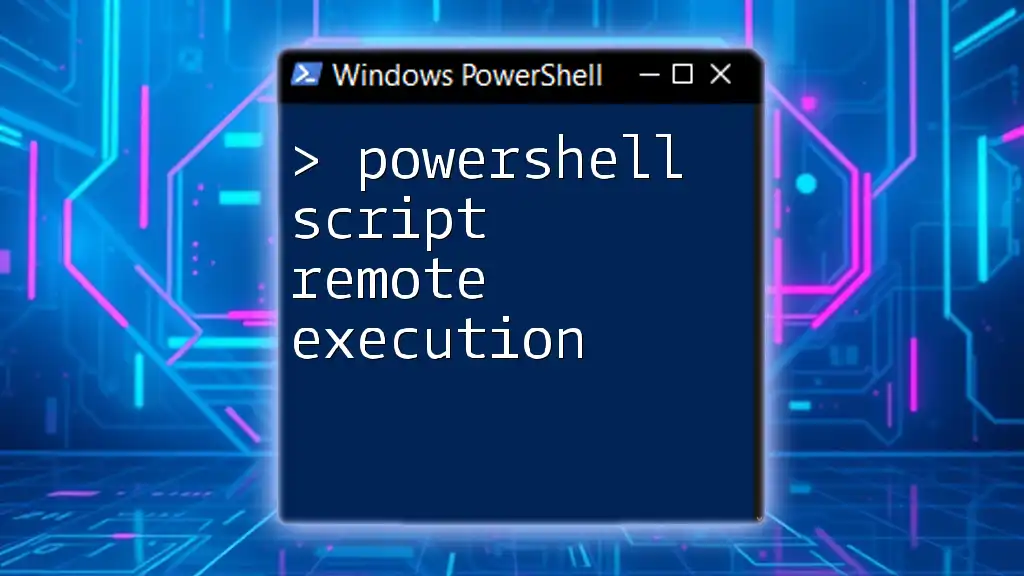
Executing Scripts in Different Environments
Running PowerShell Scripts in Windows vs. PowerShell Core
When running scripts, remember that there can be differences between Windows PowerShell and PowerShell Core (cross-platform). Some cmdlets or features in Windows PowerShell might not be available in PowerShell Core, so it’s essential to ensure compatibility based on your environment.
Executing Scripts Remotely
PowerShell also allows for remote execution of scripts, which can be extremely useful in networked environments. To run a script on a remote computer, you can use the `Invoke-Command` cmdlet. For example:
Invoke-Command -ComputerName Server01 -FilePath "C:\Scripts\MyScript.ps1"
This command sends the script to the specified remote computer (`Server01`) for execution, making it essential for managing multiple systems efficiently.
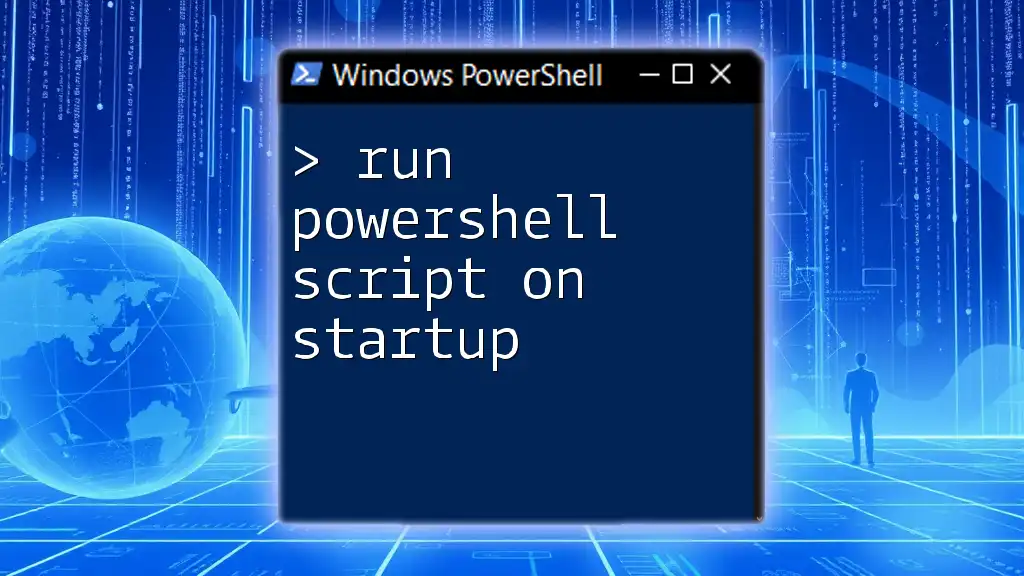
Conclusion
By following the steps outlined, you can easily run PowerShell script from PowerShell. Whether you're handling parameters, managing execution policies, or troubleshooting errors, mastering these techniques will empower you to take full advantage of PowerShell's scripting capabilities. Practice running your scripts, and don't hesitate to explore advanced features to enhance your skills further.
As you become more comfortable, consider diving deeper into the world of PowerShell with more advanced tutorials and resources to elevate your capabilities in scripting and automation.