To run a PowerShell script from the command line with parameters, you can use the following syntax, replacing `YourScript.ps1` with your script's name and `param1`, `param2` with your desired parameters:
powershell -File "C:\Path\To\YourScript.ps1" -param1 "Value1" -param2 "Value2"
Understanding PowerShell Scripts
What is a PowerShell Script?
A PowerShell script is a file containing a series of commands and instructions that automate various tasks in the Windows environment. Scripts can simplify repetitive tasks, manage systems, and manipulate data effectively. Common use cases include system administration, data extraction, and network monitoring.
Why Use Parameters?
Parameters allow scripts to be more flexible and reusable. Instead of hardcoding values, parameters enable you to pass different inputs each time the script runs. This can save time and reduce errors, particularly in scenarios where the same operations need to be performed with varying data.
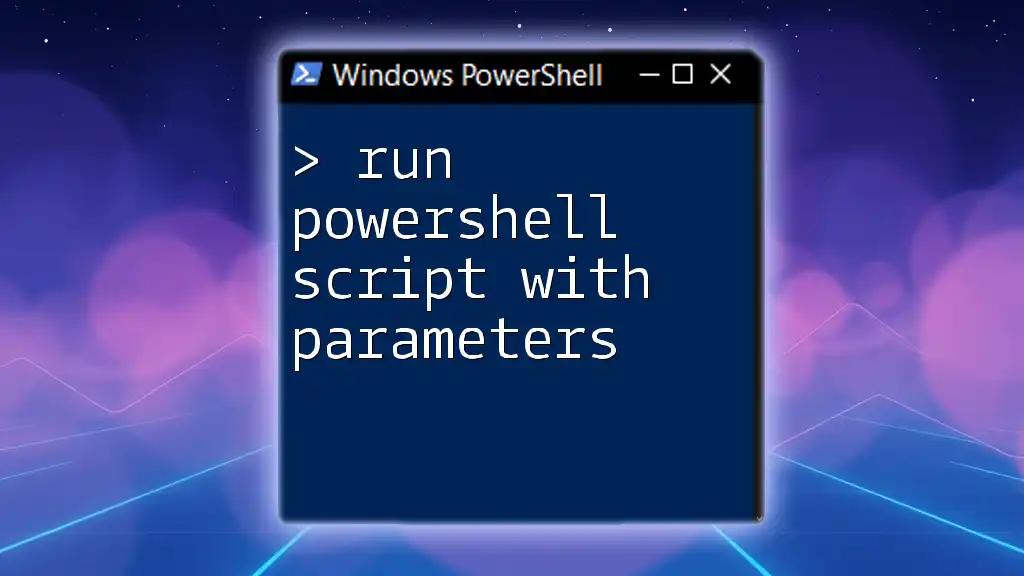
Prerequisites for Running PowerShell Scripts
Installation of PowerShell
Before you can run PowerShell scripts, ensure you have PowerShell installed. Depending on your Windows version, you might have either Windows PowerShell or PowerShell Core, which is cross-platform. You can check your installed version by executing the following command in PowerShell:
$PSVersionTable.PSVersion
Setting Execution Policy
PowerShell includes an execution policy to prevent untrusted scripts from running. To run PowerShell scripts, you may need to adjust this policy. Use the `Set-ExecutionPolicy` cmdlet to modify your execution policy. For most users, `RemoteSigned` is a suitable choice because it allows running local scripts without a signature. To set this, execute:
Set-ExecutionPolicy RemoteSigned

Creating a PowerShell Script with Parameters
Writing Your First Script
Now that your environment is ready, let’s write a PowerShell script that takes parameters. Open any code editor and create a new file named `greet.ps1`. Below is a simple example of what your script could look like:
param (
[string]$Name,
[int]$Age
)
Write-Host "Hello, $Name. You are $Age years old."
In this script:
- The `param` block defines two parameters: `Name` (a string) and `Age` (an integer).
- The script greets the user by name and age when executed.
Saving the Script
Save the script with the `.ps1` extension. This is crucial, as PowerShell scripts must be saved in this format to execute correctly. Consider saving your script in a designated folder, such as `C:\Scripts`, to keep your projects organized.
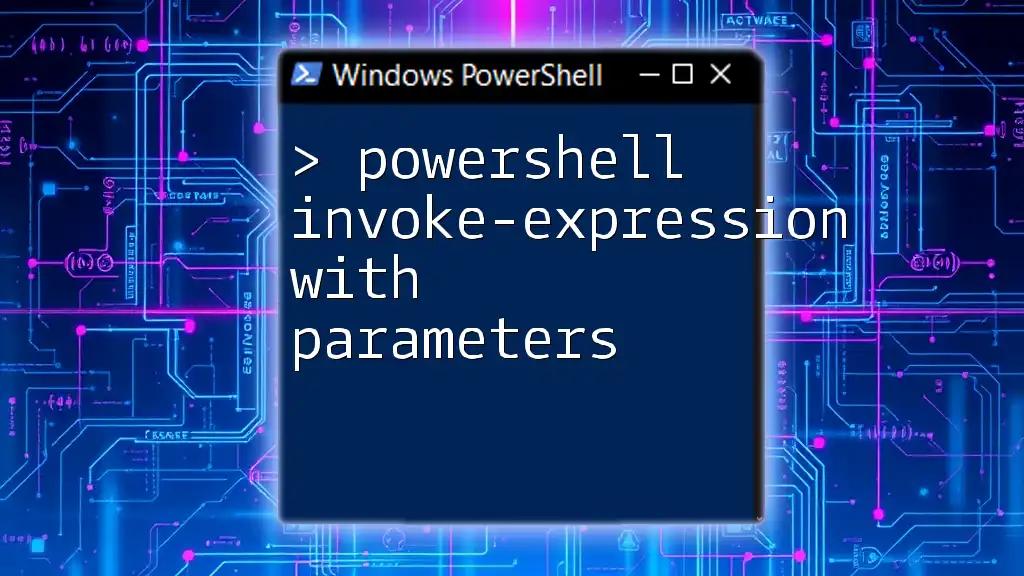
Running the PowerShell Script from Command Line
Accessing the Command Line
To run a PowerShell script from the command line, you first need to open PowerShell. You can do this by pressing `Win + R`, typing `powershell`, and hitting `Enter`.
Basic Syntax for Running Scripts with Parameters
Running a script from the command line requires a specific syntax. Navigate to the directory where the script resides. If your script is in `C:\Scripts`, use the following command:
cd C:\Scripts
After navigating to the directory, execute the script with parameters like so:
.\greet.ps1 -Name "Alice" -Age 30
In this command:
- The `.` indicates that you're executing a command in the current directory.
- `\greet.ps1` specifies the script to run.
- `-Name "Alice"` and `-Age 30` are the parameters passed to the script.
Tips for Handling Paths and Scripts
When executing scripts, be mindful of the file paths. If you prefer, you can run the script from anywhere by using an absolute path. For instance:
C:\Scripts\greet.ps1 -Name "Bob" -Age 25
This method allows you to run the script without changing your current directory.
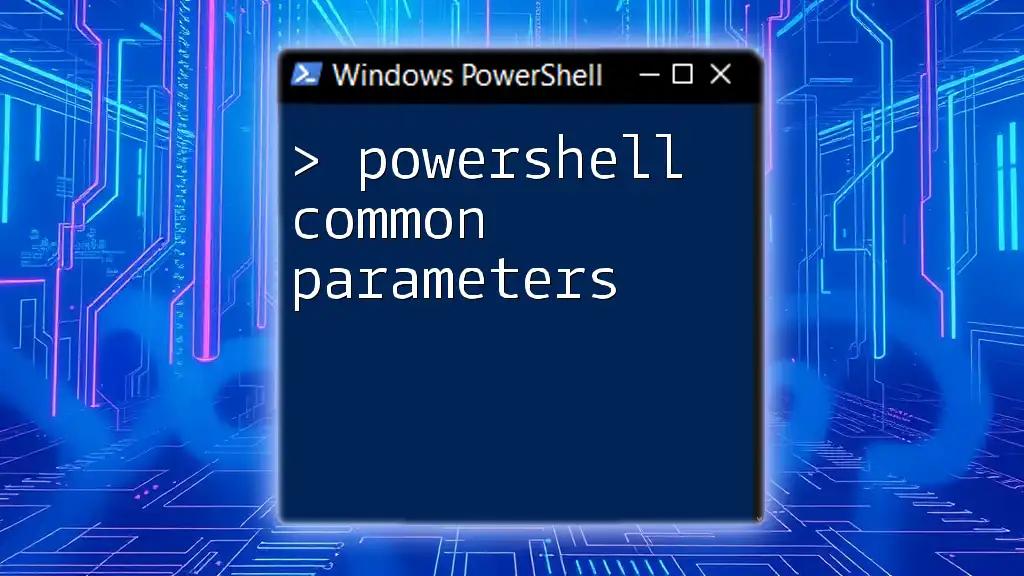
Advanced Techniques for Running PowerShell Scripts
Passing Multiple Parameters
PowerShell allows you to pass as many parameters as necessary, making it versatile for various script functions. You can use the same command structure demonstrated earlier:
.\greet.ps1 -Name "Charlie" -Age 40
Using Named vs. Positional Parameters
PowerShell supports both named and positional parameters. Named parameters explicitly define what value is being passed for which parameter:
.\greet.ps1 -Name "Diana" -Age 30
Positional parameters require you to provide them in the order they are declared without specifying the names:
.\greet.ps1 "Ethan" 35 # Positional
This flexibility simplifies script execution based on user preference or familiarity.
Handling Parameter Validation
Adding validation to your parameters enhances your script’s reliability by ensuring users input valid data. An example of using `ValidateRange` to restrict age input can be seen below:
param (
[string]$Name,
[ValidateRange(1, 120)]
[int]$Age
)
The above snippet ensures that the age value must be between 1 and 120, preventing errors and ensuring data integrity.
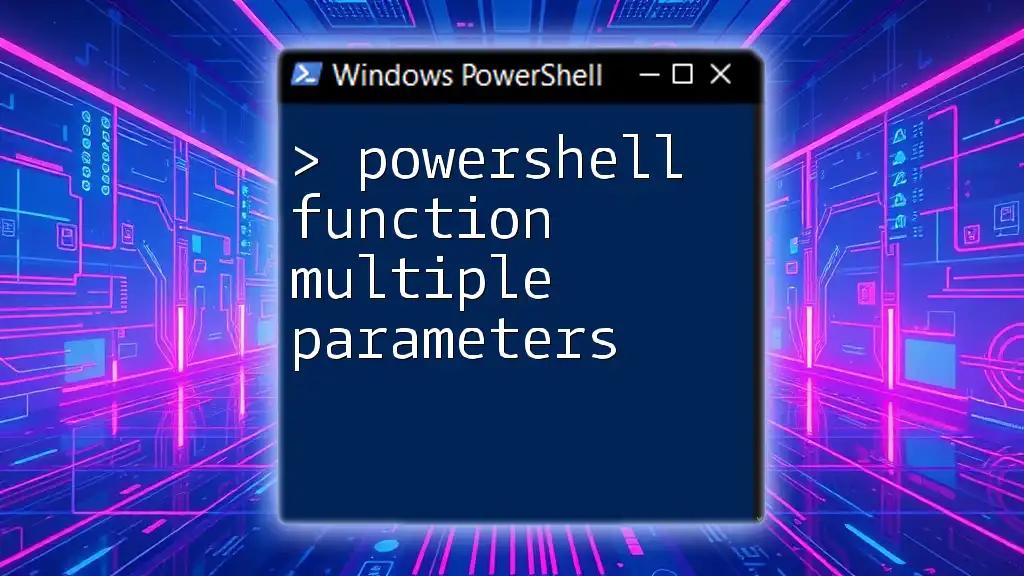
Troubleshooting Common Issues
Script Not Running or Errors
If your script doesn't run or you encounter error messages, check for common issues such as:
- Incorrect path to the script
- Restrictions due to execution policies
- Syntax errors in the script itself
Running PowerShell as an administrator might help in some cases.
Debugging Your Script
Debugging is crucial when scripts don’t behave as expected. Use the `Write-Debug` command within your script for troubleshooting:
Write-Debug "Current Name: $Name"
This will print messages only if debugging is enabled during script execution.
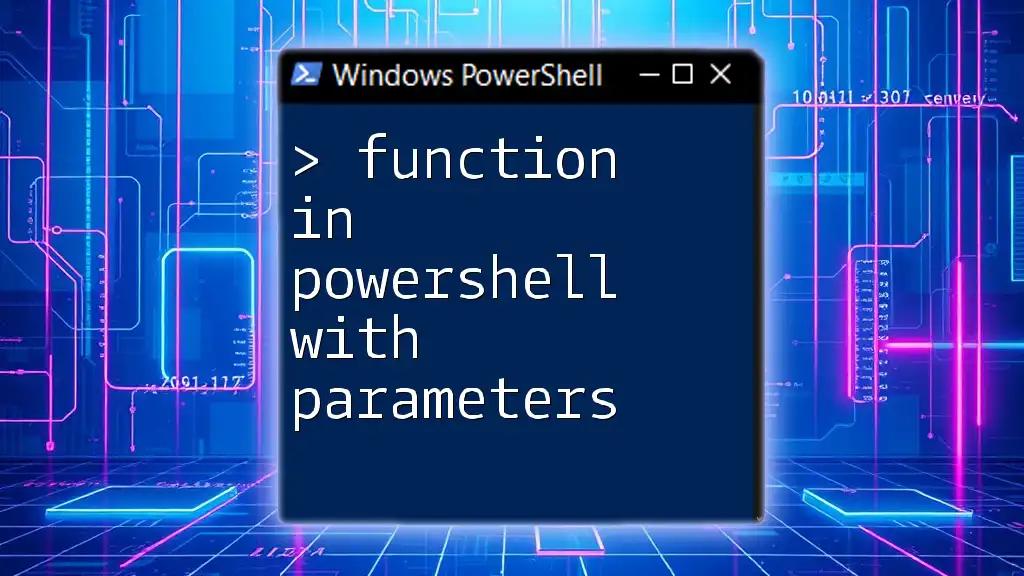
Conclusion
In this guide, we explored how to run PowerShell scripts from the command line with parameters effectively. By using parameters, you can make your scripts adaptable and user-friendly. Now that you’re equipped with this knowledge, practice writing and executing your own scripts. Don’t hesitate to reach out for feedback or questions; the world of PowerShell is vast and exciting!
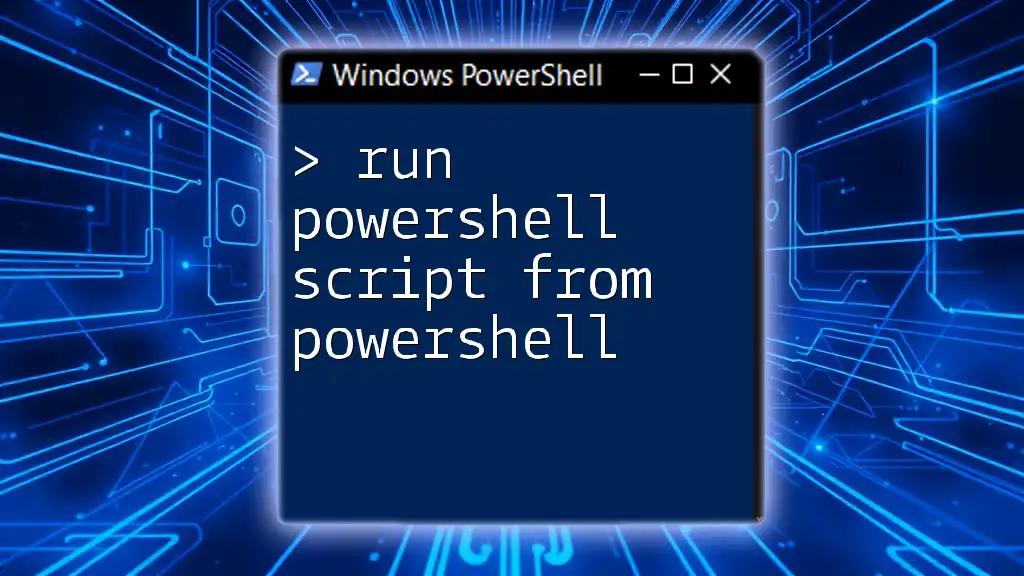
Additional Resources
For further learning, consider exploring online forums, documentation, and communities dedicated to PowerShell. Books and comprehensive online courses can also provide deeper insights into mastering PowerShell techniques.
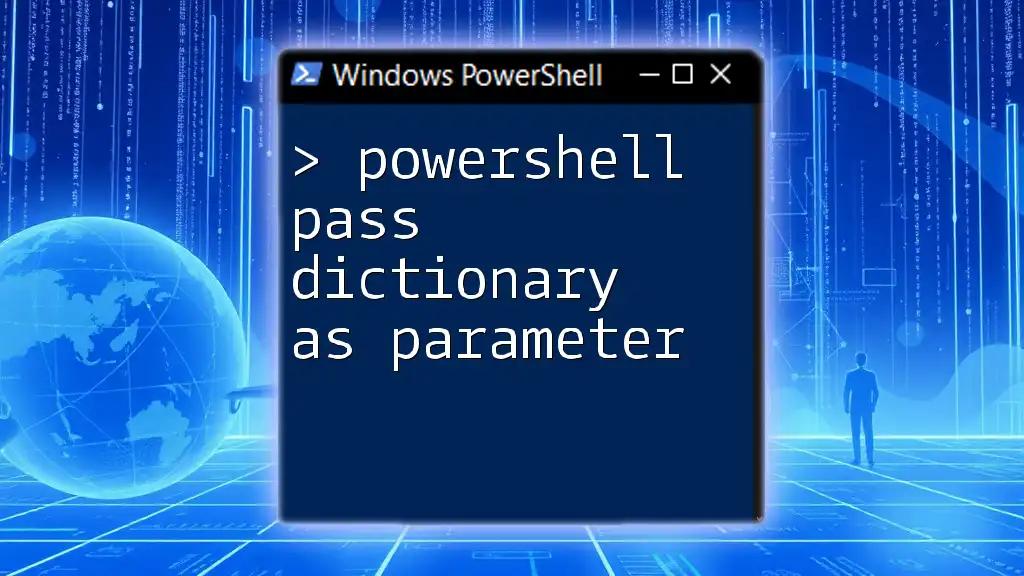
FAQ Section
What is the .ps1 file extension?
The `.ps1` file extension is used for PowerShell scripts, signifying that the file contains PowerShell commands to be executed by the PowerShell engine.
Can I run PowerShell scripts on Linux or macOS?
Yes, PowerShell Core is cross-platform and can be run on Linux and macOS, allowing you to execute scripts on different operating systems.
How can I schedule a PowerShell script to run automatically?
You can use the Task Scheduler in Windows to schedule a PowerShell script, allowing for automation at specified times or events.