In PowerShell, a function with parameters allows you to create reusable scripts that can accept input values, making your code more flexible and efficient.
function Greet-User {
param (
[string]$Name
)
Write-Host "Hello, $Name!"
}
Understanding PowerShell Functions
What is a Function?
In PowerShell, a function is a reusable block of code that can perform specific tasks. Functions help organize your scripts by creating modular pieces of code that can be executed multiple times without rewriting the same code. This modular approach enhances readability and maintainability, making your scripts more efficient.
Structure of a PowerShell Function
The basic structure of a PowerShell function consists of two primary components: the function name and the script block. Below is the basic syntax:
function FunctionName {
# Code goes here
}
You define a function using the `function` keyword followed by the name of the function and encapsulate the logic within curly braces.

Creating a Function with Parameters
PowerShell Create Function with Parameters
Adding parameters to a PowerShell function allows you to pass information into that function, making it more versatile. Parameters are defined using the `param` keyword, which allows you to specify the type and name of the parameters.
Here’s an example:
function Greet-User {
param (
[string]$Name,
[int]$Age
)
"Hello, $Name! You are $Age years old."
}
In this example, `Greet-User` accepts two parameters: `Name` and `Age`. When called, the function will utilize these parameters to produce a customized greeting.
Types of Parameters
Understanding the differences between mandatory and optional parameters is crucial when working with functions.
- Mandatory Parameters: These must be provided when calling the function. Omitting them will lead to an error.
Here’s an example of a function with mandatory parameters:
function Subtract-Numbers {
param (
[int]$FirstNumber,
[int]$SecondNumber
)
return $FirstNumber - $SecondNumber
}
- Optional Parameters: These can have default values and are not required when calling the function.
For example:
function Set-DefaultGreeting {
param (
[string]$Greeting = "Hello, World!"
)
$Greeting
}
In this case, if no argument is passed for the `Greeting`, it will default to `"Hello, World!"`.
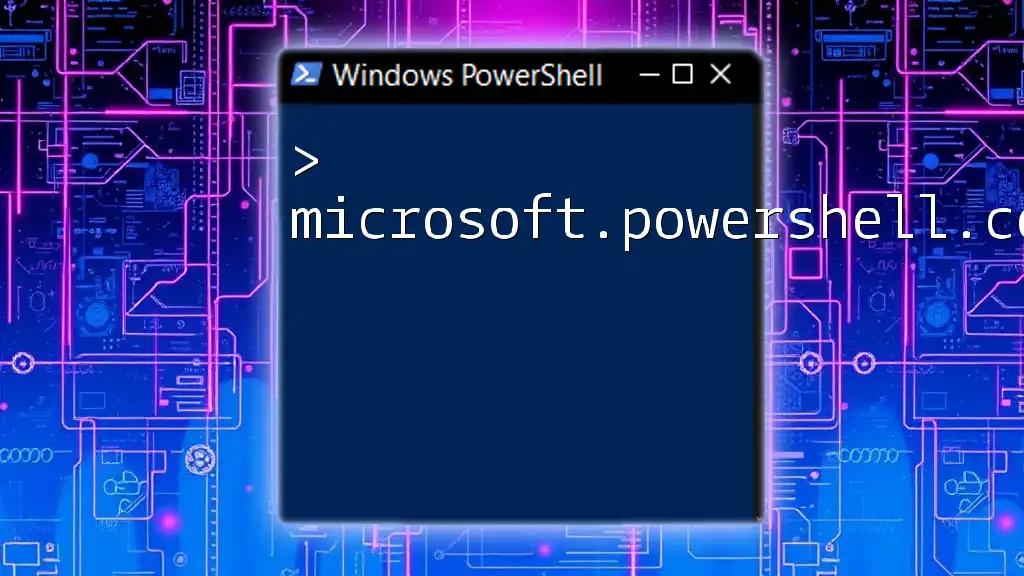
Calling PowerShell Functions with Parameters
Powershell Call Function with Parameters
When you want to execute your function, simply call its name and provide the required parameters. There are two common ways to do this: directly and by using named parameters.
Here’s an example of calling a function with named parameters:
Greet-User -Name "John" -Age 30
This format is often preferred because it improves readability, especially for functions with multiple parameters.
Passing Parameters to Function
Parameters can also be sent in the order they are defined, which is a more straightforward approach. For instance:
Subtract-Numbers 10 5
Although this is valid, using named parameters can help avoid confusion, particularly in functions with a larger number of parameters.
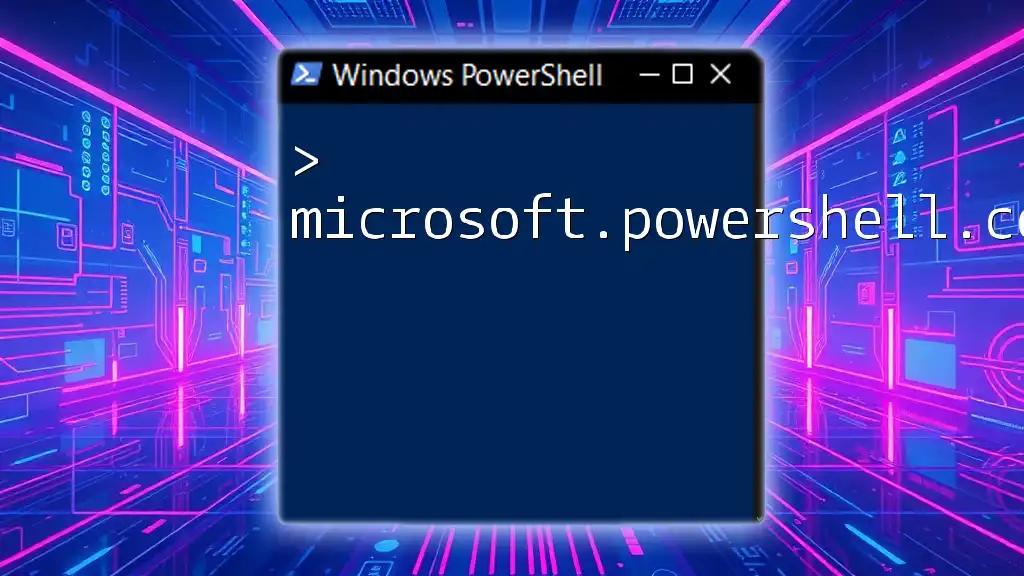
Advanced Parameter Techniques
Validation of Parameters
PowerShell allows you to enforce rules on input parameters using validation attributes. This is particularly useful for ensuring that the function only receives valid data types or formats.
Consider the following example that uses a validation attribute:
function Validate-User {
param (
[ValidateNotNullOrEmpty()]
[string]$Username
)
"Username: $Username is valid."
}
Here, the function will check if the `Username` parameter is not null or empty before proceeding, thus improving robustness.
Arrays and Hash Tables in Parameters
If you need to pass multiple values, consider using arrays. Arrays allow you to handle collections of items conveniently.
Here’s how to create a function that accepts an array:
function List-Numbers {
param (
[int[]]$Numbers
)
foreach ($number in $Numbers) {
"Number: $number"
}
}
For more complex data structures, hash tables can be employed to pass key-value pairs to your function, enabling more structured input.
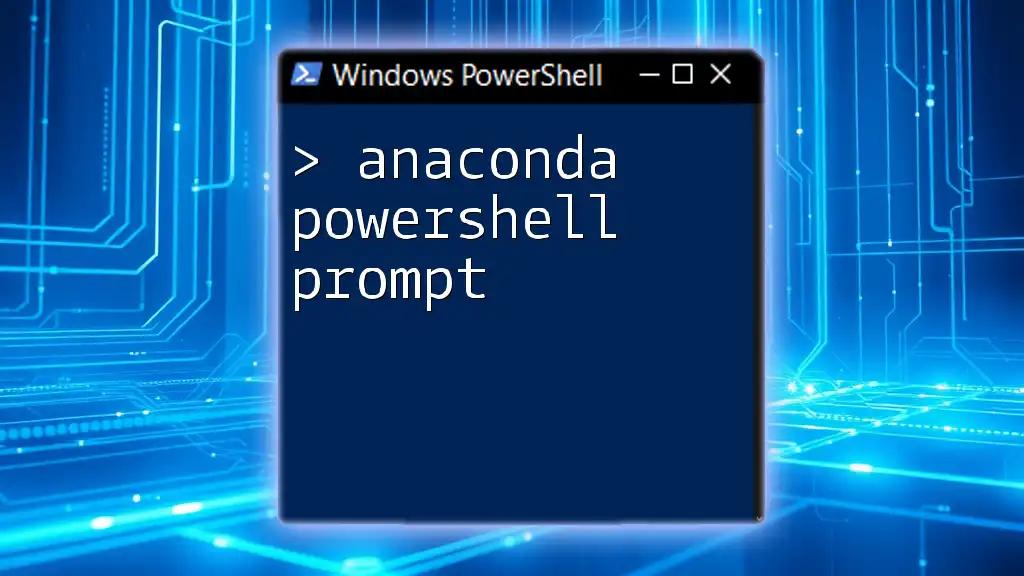
Troubleshooting Common Issues
Debugging Function Calls with Parameters
When working with functions and parameters, you may encounter various issues. Some common pitfalls include:
- Omitting mandatory parameters, which results in an error.
- Passing incorrect data types, which can lead to unexpected behavior.
To aid in debugging these issues, you can sprinkle your functions with `Write-Host` or `Write-Verbose` statements to track the flow of data. For instance:
function Debug-Sample {
param (
[string]$InputData
)
Write-Host "Received Input: $InputData"
}
Resources for Learning More
To further enhance your understanding of PowerShell functions and parameters, consider exploring additional resources like online tutorials, books, and community forums dedicated to PowerShell scripting. Engaging with the PowerShell community can provide valuable insights and best practices.
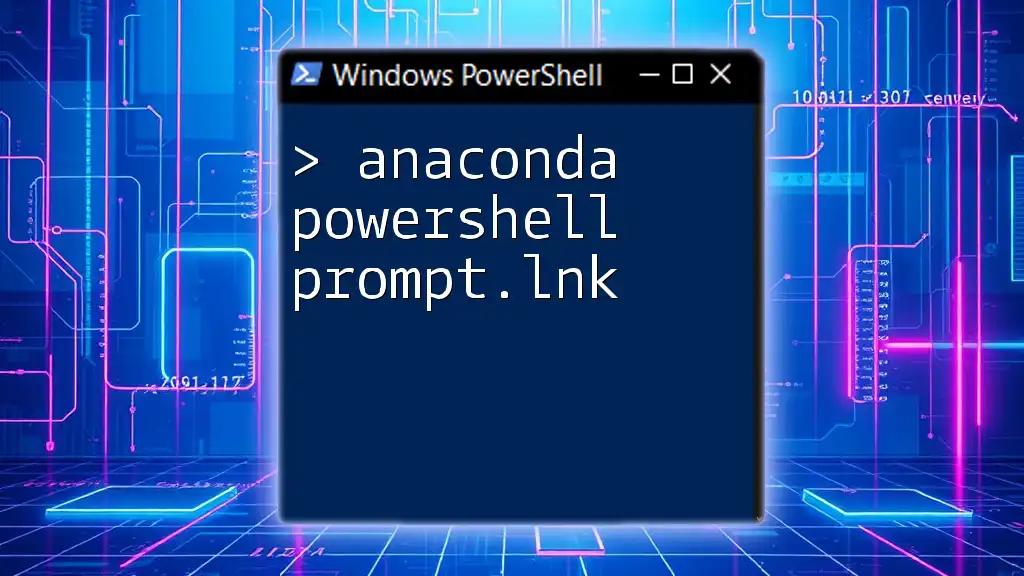
Conclusion
Mastering the function in PowerShell with parameters is essential for effective scripting. Functions encapsulate logic, promote code reuse, and allow passing data seamlessly. By understanding the structure of functions alongside parameter usage and validation techniques, you can create more powerful and flexible scripts. As you practice creating and utilizing functions, you'll gain confidence and improve your proficiency in PowerShell scripting.

Additional Examples
Real-World Use Cases for Functions with Parameters
Consider using parameters in functions to automate tasks, such as managing user accounts or fetching system information. These real-world applications underscore the value of functions in streamlining administrative tasks and enhancing overall productivity.