In PowerShell, the `ForEach-Object -Parallel` command allows you to execute a block of code for each item in a collection concurrently, significantly speeding up the processing time for large datasets.
Here's a code snippet demonstrating its usage:
$numbers = 1..10
$numbers | ForEach-Object -Parallel {
Start-Sleep -Seconds 1
Write-Host "Processing number $_"
} -ThrottleLimit 5
Understanding the `foreach` Command
What is `foreach`?
The `foreach` command in PowerShell is a loop that allows you to iterate over a collection of items. It provides a succinct way to handle operations on each element within an array or collection.
Key Characteristics of `foreach`
The `foreach` loop is particularly effective due to its simplicity and readability. The basic syntax looks like this:
foreach ($item in $collection) {
# Actions performed on $item
}
This loop can handle arrays, collections, and any enumerable items, making it a versatile tool for PowerShell scripting.
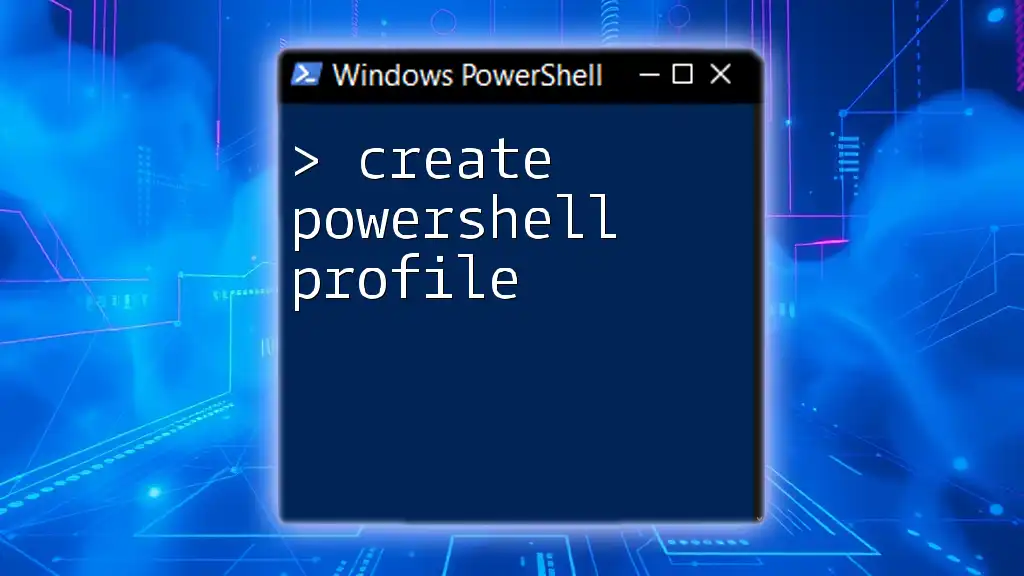
The Need for Parallel Processing
Why Use Parallel Processing?
Parallel processing becomes essential when you need to improve the performance of scripts that involve long-running tasks. By performing operations concurrently, you can significantly reduce the total execution time.
For example, consider a scenario where you need to download multiple files from the internet. If you process these downloads sequentially, each task waits for the previous one to complete. In contrast, using parallel processing enables the simultaneous execution of multiple downloads, leading to substantial time savings.
Drawbacks of Synchronous Processing
In synchronous processing, each task completes before the next one starts, which can lead to delays. Consider a scenario where you use a synchronous `foreach` loop:
$urls = @("http://example.com/file1", "http://example.com/file2")
foreach ($url in $urls) {
Invoke-WebRequest -Uri $url -OutFile ("C:\downloads\" + (Split-Path $url -Leaf))
}
This script downloads each file one after the other, leading to longer wait times, especially when network speed and file sizes vary.
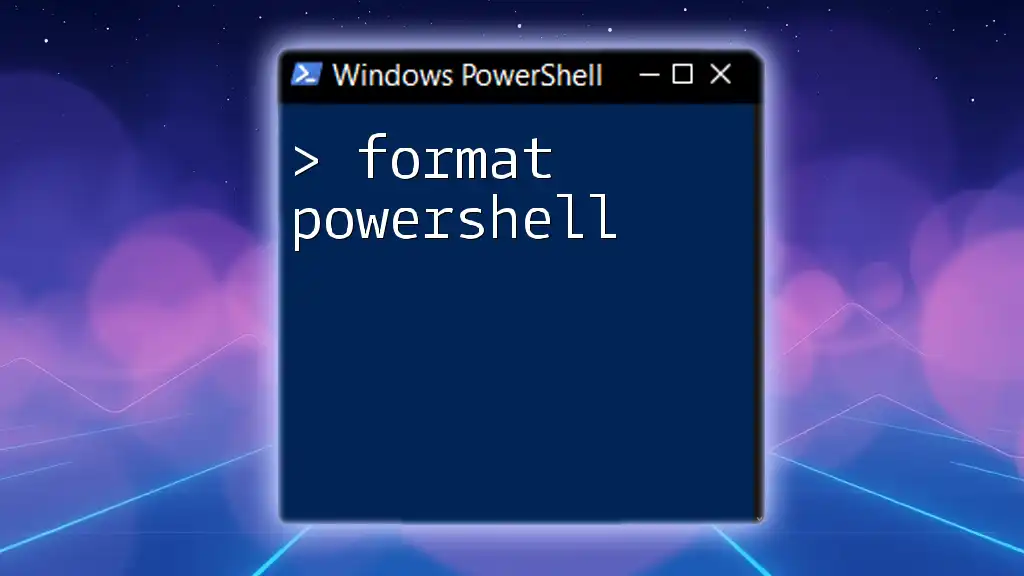
Implementing Parallel Processing with `ForEach-Object`
Introduction to `ForEach-Object`
The `ForEach-Object` cmdlet is designed to allow you to process each element of an input collection. This cmdlet can easily integrate with pipelines, making it useful for processing data streams.
Using the `-Parallel` Parameter
What is the `-Parallel` Parameter?
The `-Parallel` parameter enables parallel execution within the `ForEach-Object` cmdlet, allowing you to specify a script block that runs for each item concurrently.
Syntax Breakdown
The basic syntax for using the `-Parallel` parameter is:
$collection | ForEach-Object -Parallel {
# Commands to execute for each item
}
Example of Parallel Processing
Simple Example: Downloading Files
Here’s a straightforward example that demonstrates the effectiveness of using `foreach powershell parallel`:
$urls = @("http://example.com/file1", "http://example.com/file2")
$urls | ForEach-Object -Parallel {
Invoke-WebRequest -Uri $_ -OutFile ("C:\downloads\" + (Split-Path $_ -Leaf))
}
This script initiates downloads for all specified URLs simultaneously, drastically reducing the overall wait time.
Complex Example: Processing Data
Let’s consider a more complex scenario where we need to simulate a time-consuming task for a large set of data:
$data = 1..100
$data | ForEach-Object -Parallel {
Start-Sleep -Seconds 2 # Simulates a time-consuming task
Write-Output "Processed $_"
} -ThrottleLimit 10
In this example, we process numbers from 1 to 100 in parallel. The `-ThrottleLimit` parameter restricts the number of concurrent tasks to 10, which is useful for managing system resources effectively.
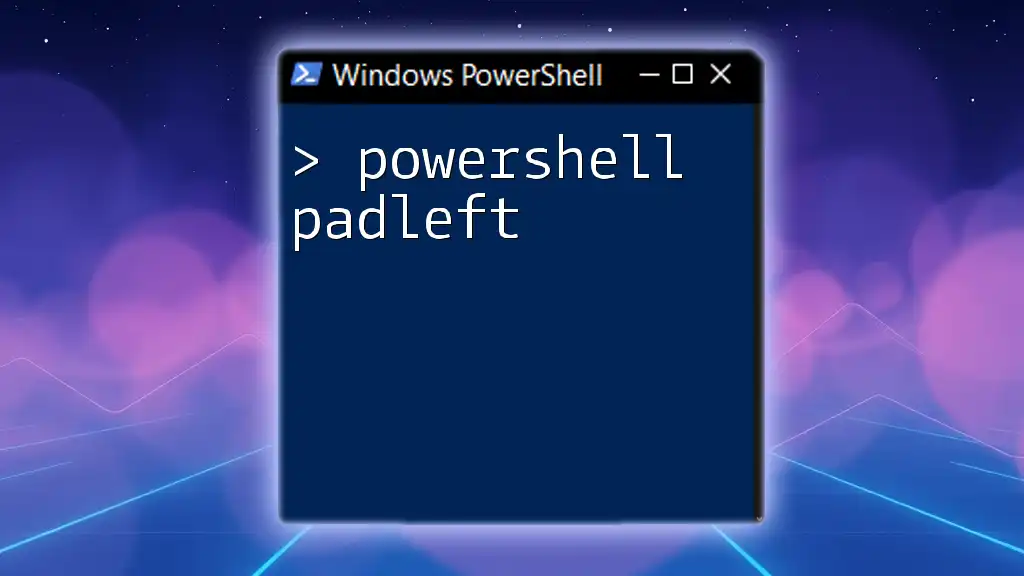
Real-World Applications of `foreach` Parallel
Data Processing Tasks
Parallel processing shines when you're dealing with large datasets. For instance, utilizing PowerShell scripts for log file analysis or bulk data processing can yield significant time savings. By executing tasks in parallel, you can analyze multiple log files or records without waiting for each to finish before starting the next.
Automation Scripts
Many automation scripts benefit from parallel execution. Tasks like system backups, remote administration commands, or software deployments can be significantly accelerated when using parallel processing.
Azure and Cloud Services
Cloud resources management can also be enhanced through parallel processing. For example, when automating the provisioning of virtual machines in Azure, using parallel processing can expedite resource allocation and configuration, optimizing the entire deployment process.
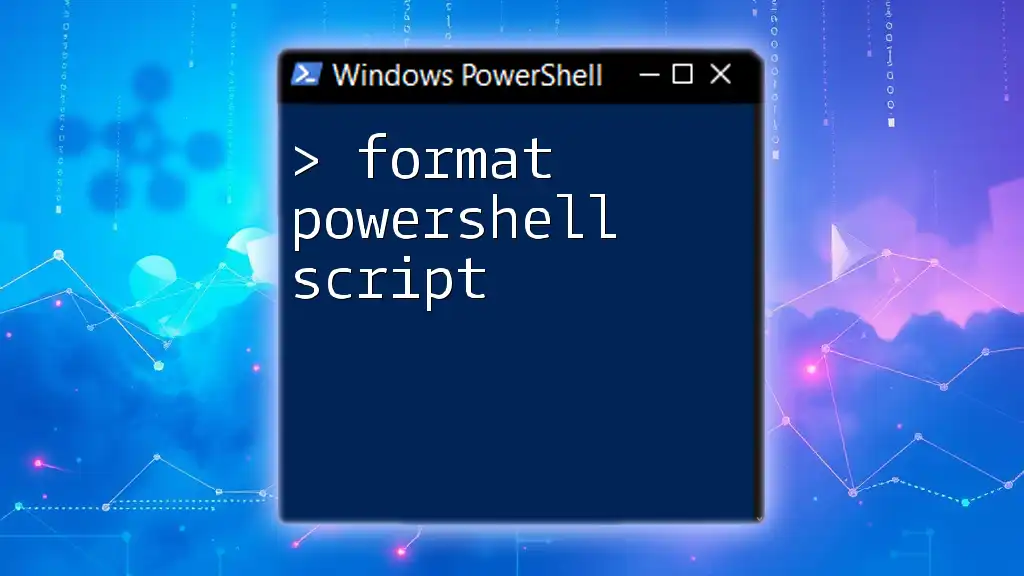
Best Practices for Using `foreach` in Parallel
Understanding Resource Limits
Before diving into parallel processing, it's crucial to understand your system's capability limits. Running too many simultaneous tasks can lead to resource starvation and potential failures. Always gauge the optimal number of concurrent operations based on your system's specifications.
Error Handling in Parallel Execution
Handling Errors Gracefully
When executing tasks in parallel, managing errors effectively becomes vital. You can catch exceptions within the `-Parallel` block to ensure they do not cause the entire operation to fail. Use try-catch blocks within your script block for robust error handling:
$data | ForEach-Object -Parallel {
try {
# Operation that might fail
} catch {
Write-Output "Error processing $_: $_"
}
}
Performance Monitoring
As with any automation task, monitoring performance is essential. You can utilize PowerShell’s built-in commands or external tools to measure execution time and resource usage. Observing these metrics allows you to fine-tune your scripts for improved efficiency.
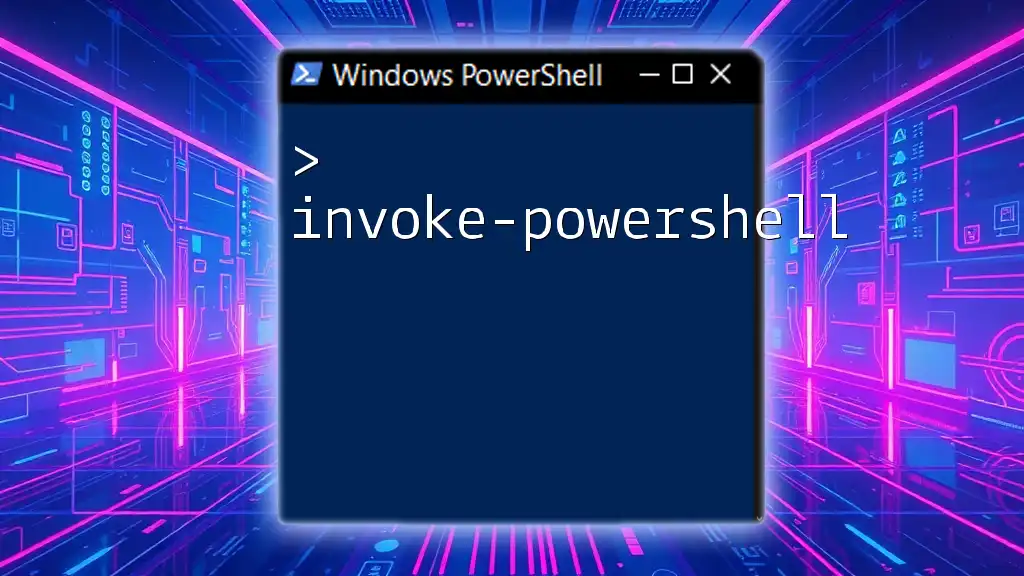
Conclusion
Using foreach powershell parallel execution can dramatically enhance script performance, especially for tasks that involve multiple items. By utilizing the `ForEach-Object -Parallel` cmdlet, you can leverage the power of multi-threading effectively. Implementing best practices such as resource management, error handling, and performance monitoring will ensure a smooth and efficient scripting experience.
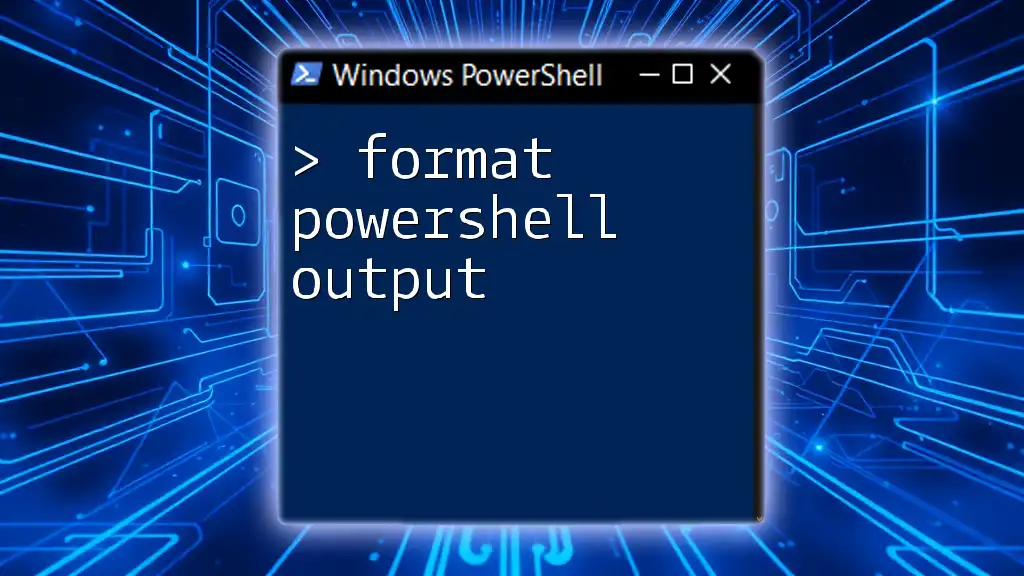
Additional Resources
Documentation Links
For more information, check the official PowerShell documentation on [ForEach-Object](https://learn.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/foreach-object) and [parallel processing](https://learn.microsoft.com/en-us/powershell/scripting/developer/cmdlet/parallel-processing).
Further Reading
Look into recommended PowerShell books, articles, and tutorials to deepen your understanding of parallel processing.
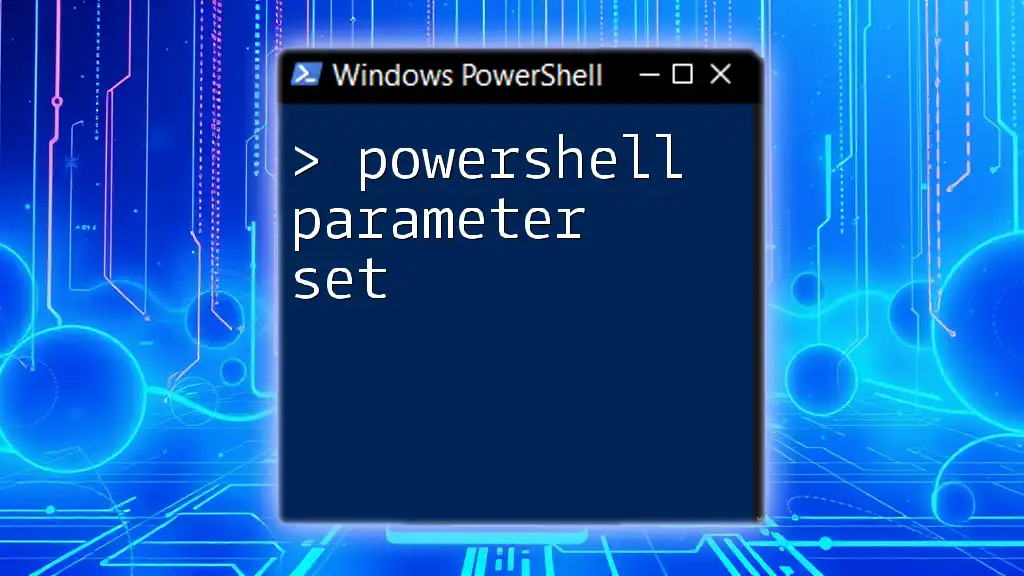
Call to Action
Start practicing parallel processing with `foreach` in your scripts today! Don't hesitate to share your experiences or ask questions in the comments section. Your insights and queries can contribute to a richer learning experience for everyone.