The "touch" equivalent in PowerShell is achieved using the `New-Item` cmdlet, which creates a new file or updates the timestamp of an existing file.
Here’s how you can use it:
New-Item -Path 'C:\path\to\your\file.txt' -ItemType File -Force
This command will create `file.txt` if it doesn't exist, or update its timestamp if it does.
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for task automation and configuration management. Built on the .NET framework, it allows IT professionals and system administrators to manage systems, automate repetitive tasks, and integrate with various services and applications.
Learning PowerShell can significantly improve your efficiency by automating common tasks and enforcing consistent configurations across multiple systems.
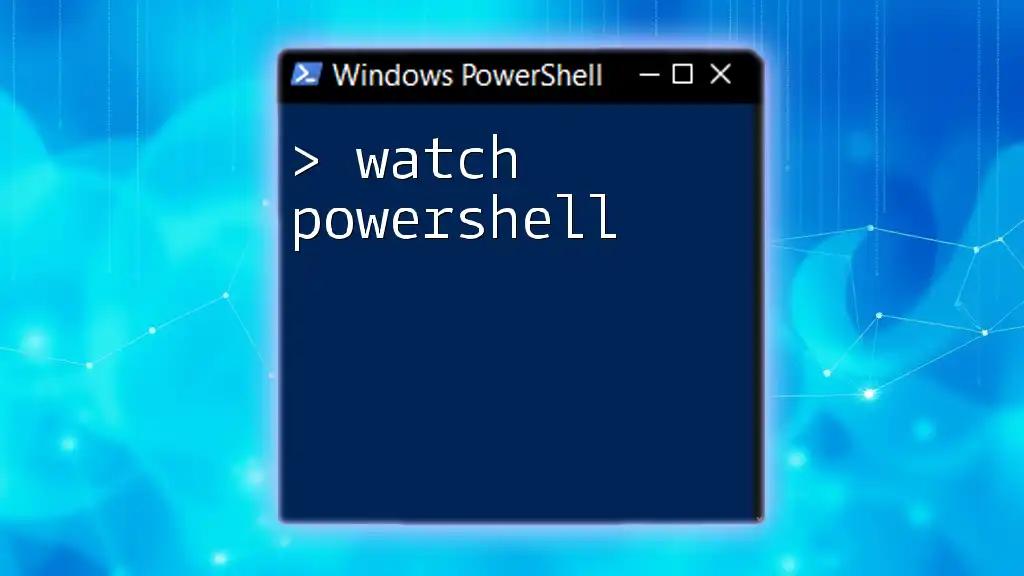
Why Use the Touch Command in PowerShell?
The touch command is a concept that originally comes from Unix/Linux environments, where it serves two primary functions: creating empty files and updating the timestamps of existing files. In PowerShell, the equivalent functionality is crucial for various automation scripts, making it indispensable for efficient file management.
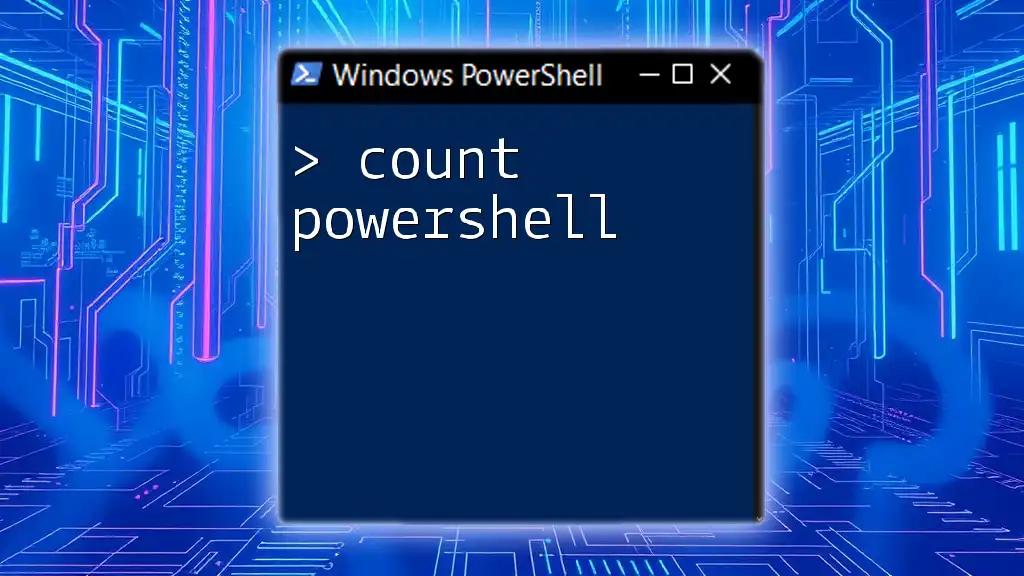
Understanding the Touch Command Concept
Defining Touch in PowerShell
While PowerShell does not have a direct `touch` command like Unix/Linux, you can easily mimic its functionality using existing PowerShell cmdlets. This allows you to either create a new file or update the modification timestamps of existing ones, facilitating better file management within your scripts.
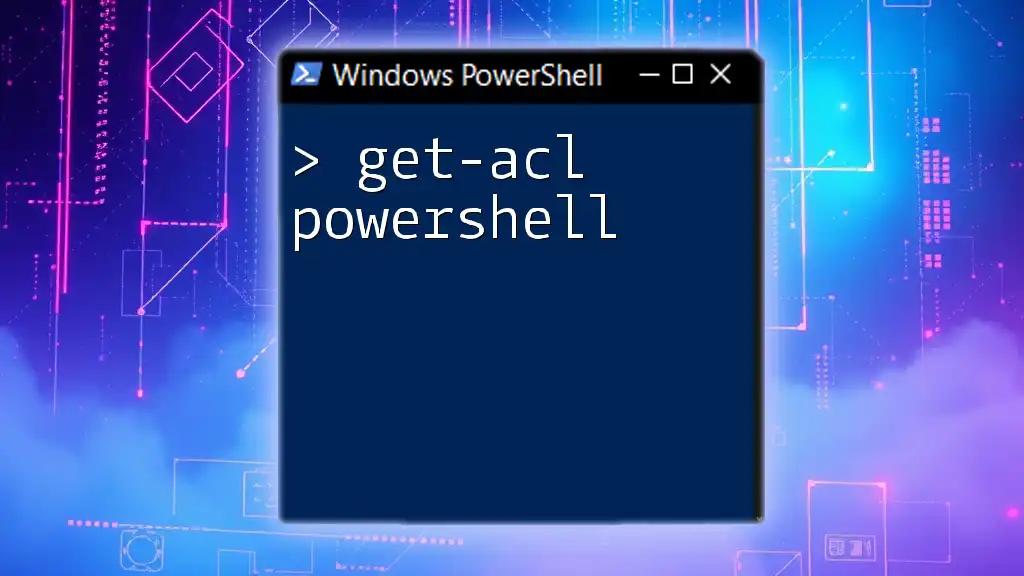
Creating Files with PowerShell
Using New-Item Cmdlet
The `New-Item` cmdlet in PowerShell is essential for creating new files or folders. Its flexibility allows you to specify different types for various use cases.
Example: Creating a new text file
To create a text file named `myfile.txt` in the directory `C:\example`, you can simply use:
New-Item -Path "C:\example\myfile.txt" -ItemType File
In this command:
- `-Path` specifies the location and name of the file.
- `-ItemType` is used to indicate that you want to create a file.
Using Out-File Cmdlet
PowerShell’s `Out-File` cmdlet is particularly useful when you want to create a new file from the output of another command.
Example: Creating a new file from command output
If you want to capture the list of running processes and save it to a text file, you can use:
Get-Process | Out-File -FilePath "C:\example\processes.txt"
In this instance, `Get-Process` gathers the currently running processes, and `Out-File` redirects this output into the specified file.

Modifying Timestamps of Files in PowerShell
Using Set-ItemProperty Cmdlet
The `Set-ItemProperty` cmdlet is vital for modifying file attributes, including last modified and creation dates.
Example: Updating creation date
If you want to set the creation date of `myfile.txt` to the current date and time, you can execute:
Set-ItemProperty -Path "C:\example\myfile.txt" -Name CreationTime -Value (Get-Date)
This command sets the creation time of `myfile.txt` to the current date and time retrieved by `Get-Date`, demonstrating how easy it is to manipulate file metadata in PowerShell.
Using Get-ChildItem and Get-Date
You can retrieve file properties using `Get-ChildItem`, and update timestamps in a straightforward manner.
Example: Changing file timestamps
To update the last modified time for `myfile.txt`, the following command does the trick:
$file = Get-ChildItem "C:\example\myfile.txt"
$file.LastWriteTime = (Get-Date)
This approach utilizes PowerShell's object-oriented capabilities, allowing you to fetch file information and modify it as needed.
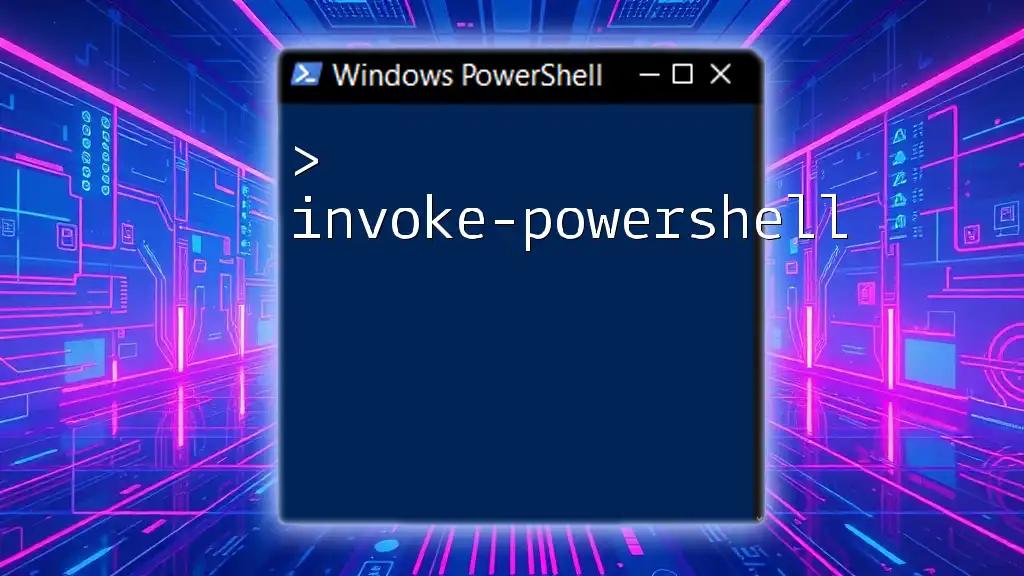
Creating a Custom Function to Simulate Touch
Defining the Function
If you’d like to streamline your file-management process, you can create a custom function that simulates the touch command in PowerShell. This function checks if a file exists; if not, it creates one, and then updates the timestamp of the file.
Here's how to define the function:
function Touch {
param (
[string]$Path
)
if (!(Test-Path $Path)) {
New-Item -ItemType File -Path $Path | Out-Null
}
(Get-Item $Path).LastWriteTime = Get-Date
}
Example Usage of Custom Touch
After defining the function, you can use it like so:
Touch -Path "C:\example\customTouchFile.txt"
This command will create `customTouchFile.txt` if it doesn't exist, and update its last write time to the current date, effectively simulating the Unix/Linux `touch` command.
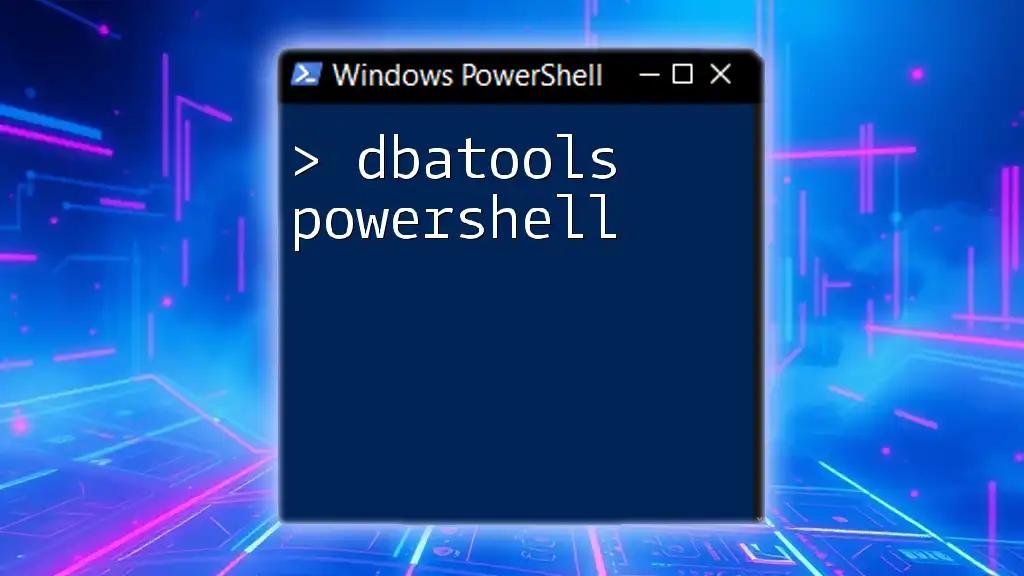
Best Practices and Common Mistakes
Best Practices for File Management in PowerShell
- Organize scripts logically: Maintain a clear structure and organization of your scripts to facilitate their reusability and maintenance.
- Implement error handling: Use try/catch blocks to manage exceptions and improve your script's reliability.
Common Mistakes to Avoid
- Forgetting to specify the correct path: Always double-check file paths, as incorrect paths can lead to unexpected errors.
- Neglecting to handle existing files: Make sure your scripts properly account for existing files to avoid overwriting critical data.
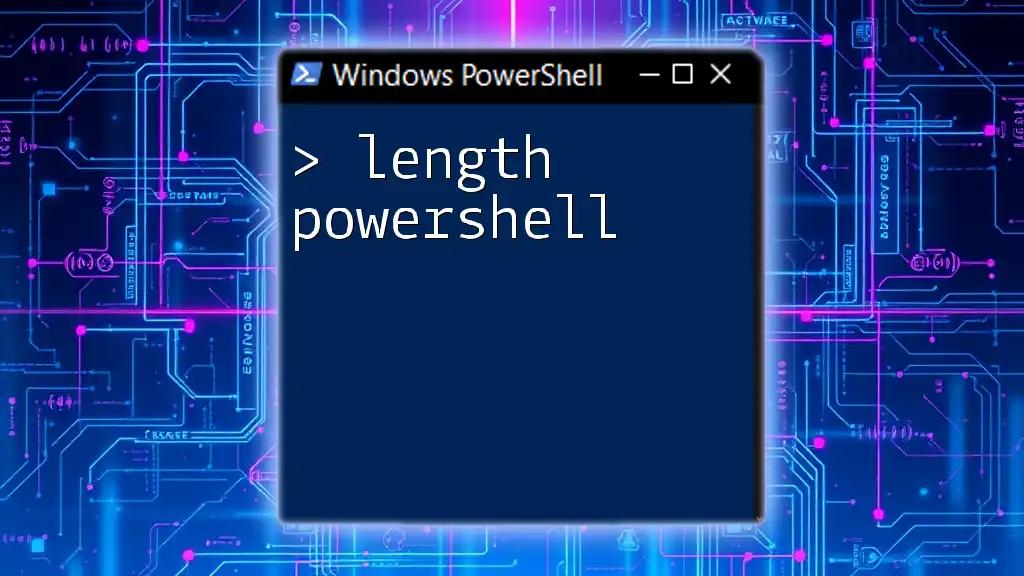
Conclusion
The touch functionality in PowerShell, while not as straightforward as in Unix/Linux systems, can be effectively simulated using a combination of built-in cmdlets. By leveraging commands like `New-Item`, `Set-ItemProperty`, and custom functions, you can automate file creation and modification processes. This not only enhances your efficiency but empowers you to manage file systems effectively within your PowerShell scripts.
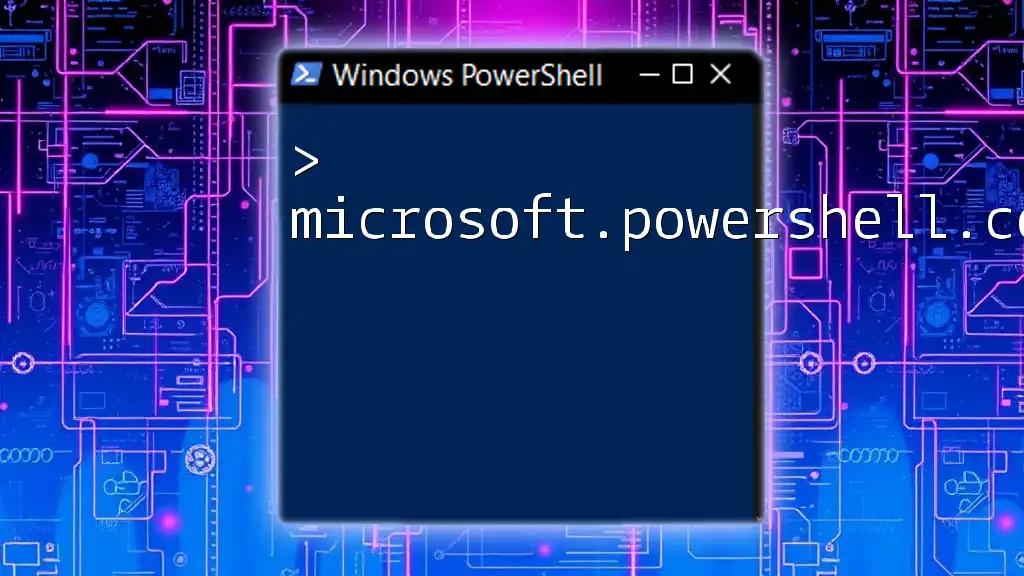
Additional Resources
For further exploration of PowerShell, consider checking out the official Microsoft PowerShell documentation, participating in online forums, or enrolling in comprehensive courses focused on mastering PowerShell.
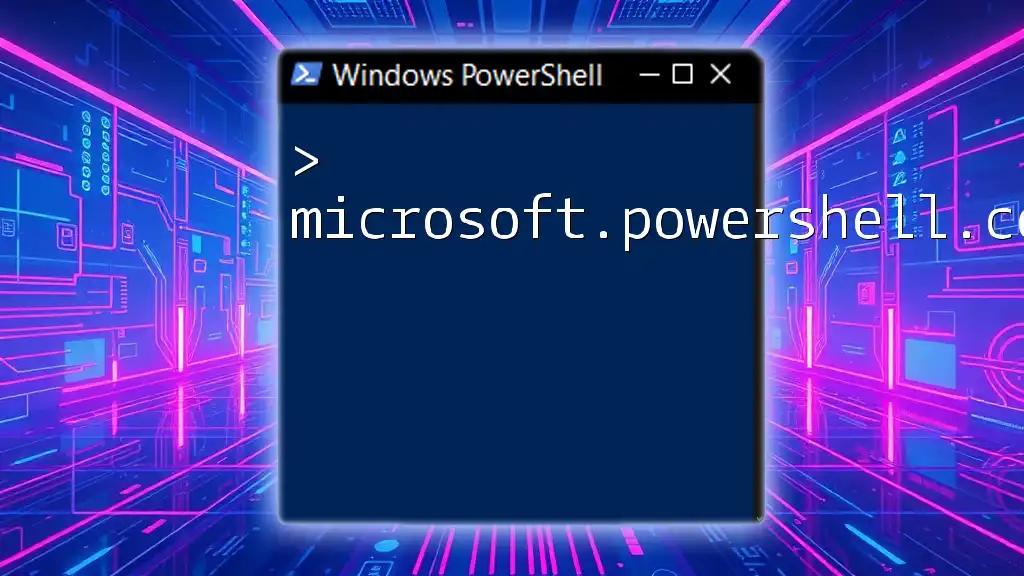
FAQs
Can I use PowerShell to modify file timestamps on remote systems?
Yes, you can use PowerShell remoting to execute commands on remote systems, allowing you to modify file timestamps as needed.
What are alternative ways to create files in PowerShell?
In addition to `New-Item` and `Out-File`, you can use redirection operators (`>` or `>>`) to create files in PowerShell quickly.
How does file handling in PowerShell differ from CMD?
PowerShell uses an object-oriented approach, allowing for more complex manipulations with files, unlike CMD, which is more suited for basic command-line tasks.
By mastering these concepts, you will significantly enhance your proficiency and workflow in managing files with PowerShell.