WinSCP PowerShell is a powerful tool that allows users to automate file transfer and management tasks between local and remote servers using WinSCP's command-line features within PowerShell.
Here's a simple code snippet to connect to a remote server and upload a file using WinSCP in PowerShell:
# Import WinSCP .NET assembly
Add-Type -Path "C:\Program Files (x86)\WinSCP\WinSCPnet.dll"
# Set up session options
$sessionOptions = New-Object WinSCP.SessionOptions -Property @{
Protocol = [WinSCP.Protocol]::Sftp
HostName = 'example.com'
UserName = 'username'
Password = 'password'
SshHostKeyFingerprint = 'ssh-rsa 2048 xxxxxxx...='
}
# Create a session and upload a file
$session = New-Object WinSCP.Session
try {
$session.Open($sessionOptions)
$transferOptions = New-Object WinSCP.TransferOptions
$transferOptions.TransferMode = [WinSCP.TransferMode]::Binary
$transferResult = $session.PutFiles("C:\local\path\file.txt", "/remote/path/", $False, $transferOptions)
$transferResult.Check()
Write-Host "Upload successful!"
} finally {
$session.Dispose()
}
What is WinSCP?
WinSCP is a powerful and versatile tool primarily used for transferring files securely over the Internet. It supports several file transfer protocols, including SFTP (SSH File Transfer Protocol), SCP (Secure Copy Protocol), FTP (File Transfer Protocol), and WebDAV.
Some of the key features of WinSCP include:
- Graphical User Interface (GUI): WinSCP offers a user-friendly GUI that simplifies file management and transfers.
- Scripting and Automation: This is where the combination with PowerShell shines, allowing users to automate their file transfer tasks efficiently.
- Highly Configurable: Users can customize settings for connection types, protocols, and transfer parameters depending on their needs.
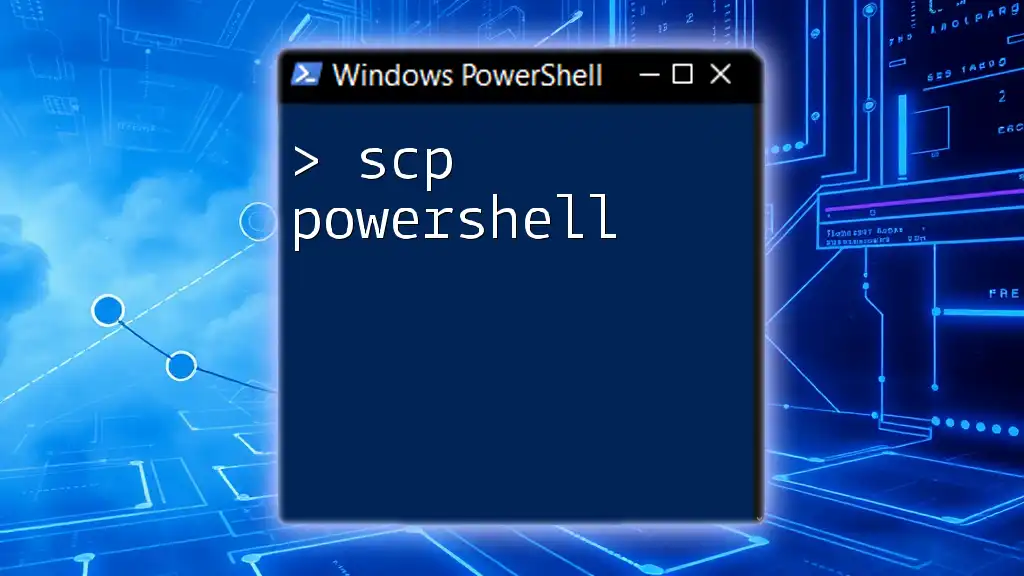
Why Use PowerShell with WinSCP?
Integrating PowerShell with WinSCP provides a robust solution for automating file transfer processes. PowerShell's scripting capabilities can significantly reduce manual effort, save time, and improve accuracy. Here are a few benefits:
- Automation: You can schedule tasks, run scripts, and manage bulk file transfers easily.
- Error Handling: PowerShell provides advanced error-handling mechanisms, enabling you to recover gracefully in case of any issues during file transfers.
- Integration with Other Systems: Leverage PowerShell’s compatibility with other Windows features, enabling seamless integration with your overall system architecture.
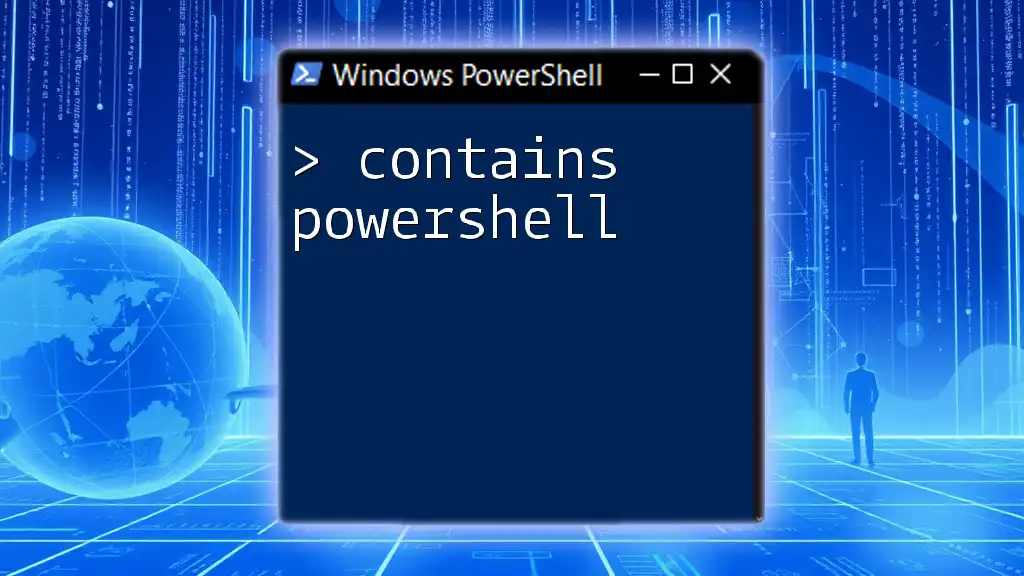
Installing WinSCP
To get started, download and install WinSCP from the official website. Follow these steps:
- Navigate to the WinSCP download page.
- Select the latest version and click to download.
- Run the installer and follow the prompts to complete the installation.
Once installed, you can verify the installation by launching WinSCP and checking its version through the Help menu.
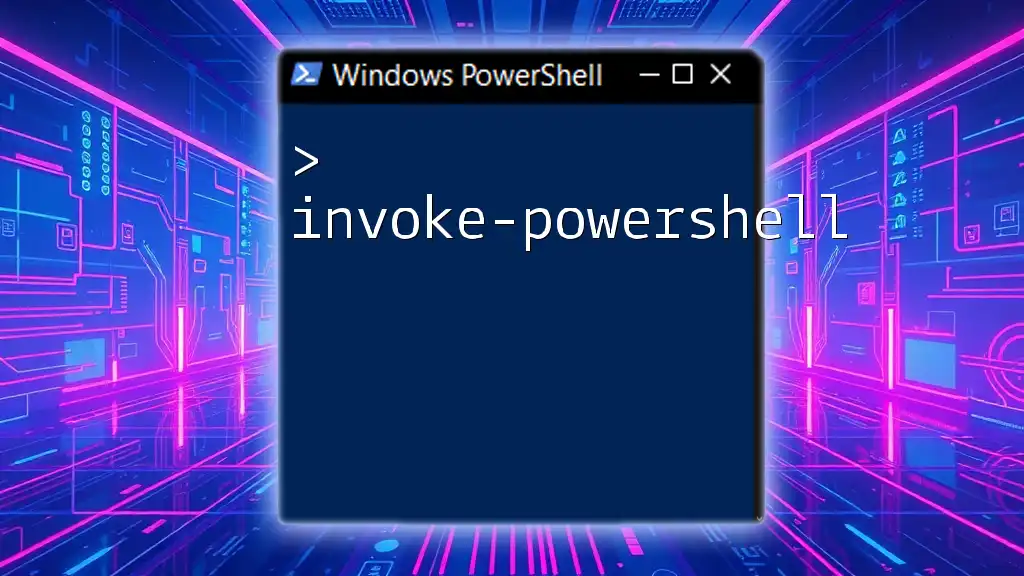
Setting Up PowerShell for WinSCP
To utilize WinSCP in your PowerShell scripts, you need to reference the WinSCP .NET assembly. This allows you to access WinSCP's functionality directly from PowerShell. Here’s how to set it up:
Add-Type -Path "C:\Program Files (x86)\WinSCP\WinSCPnet.dll"
Make sure that the file path to the `WinSCPnet.dll` is accurate based on your machine’s installation directory.
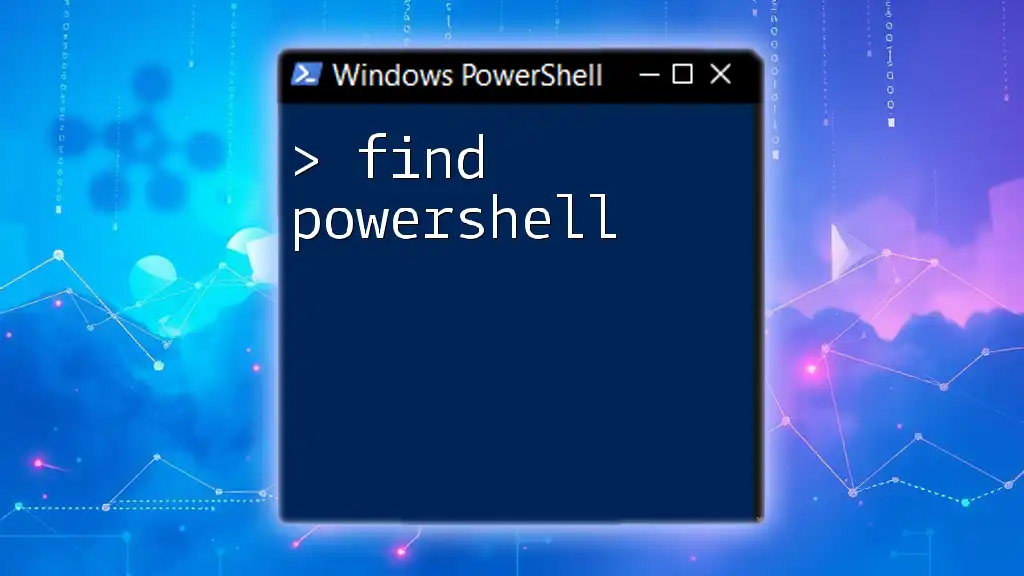
Establishing a Connection
Creating a connection to a server is the first step in any file transfer process. Use the following syntax to set up your connection parameters:
$sessionOptions = New-Object WinSCP.SessionOptions -Property @{
Protocol = [WinSCP.Protocol]::Sftp
HostName = "example.com"
UserName = "user"
Password = "password"
SshHostKeyFingerprint = "ssh-rsa 2048 ...="
}
Replace `"example.com"`, `"user"`, and `"password"` with your actual server credentials. The `SshHostKeyFingerprint` is essential for verifying the server's identity.
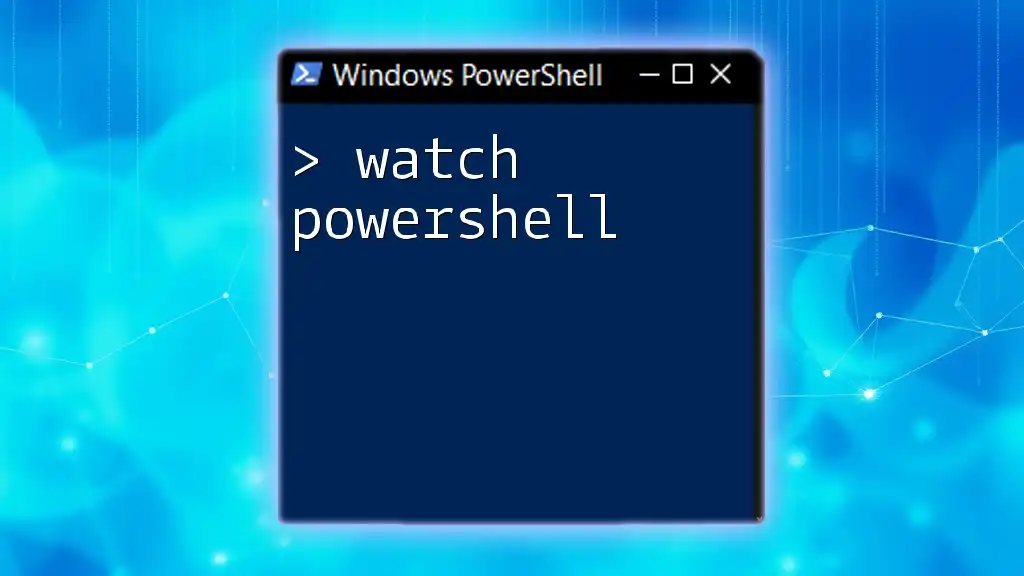
Uploading Files
After establishing a connection, you can upload files to the remote server. To upload a local file, use the following example:
$session = New-Object WinSCP.Session
$session.Open($sessionOptions)
$transferOptions = New-Object WinSCP.TransferOptions
$transferOptions.TransferMode = [WinSCP.TransferMode]::Binary
$transferResult = $session.PutFiles("C:\local\path\file.txt", "/remote/path/", $False, $transferOptions)
In the above code:
- Adjust `C:\local\path\file.txt` and `/remote/path/` to suit your local and remote directory paths.
- The `$False` parameter indicates that you want to skip creating remote directories.
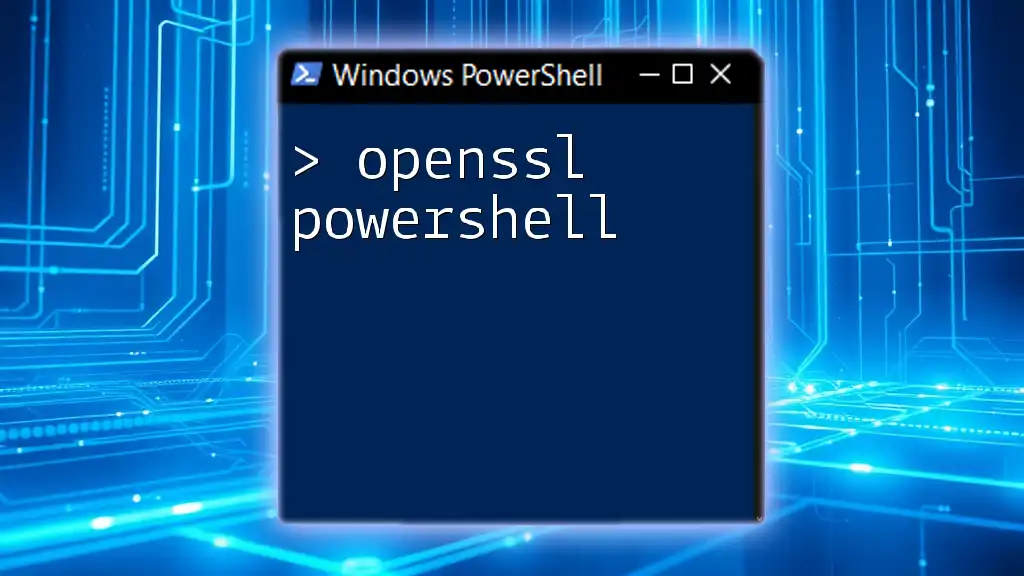
Downloading Files
You can also download files from the server using a similar command structure. Below is an example of how to download a file:
$session.GetFiles("/remote/path/file.txt", "C:\local\path\").Check()
This command will take the remote file located at `/remote/path/file.txt` and save it to `C:\local\path\`.
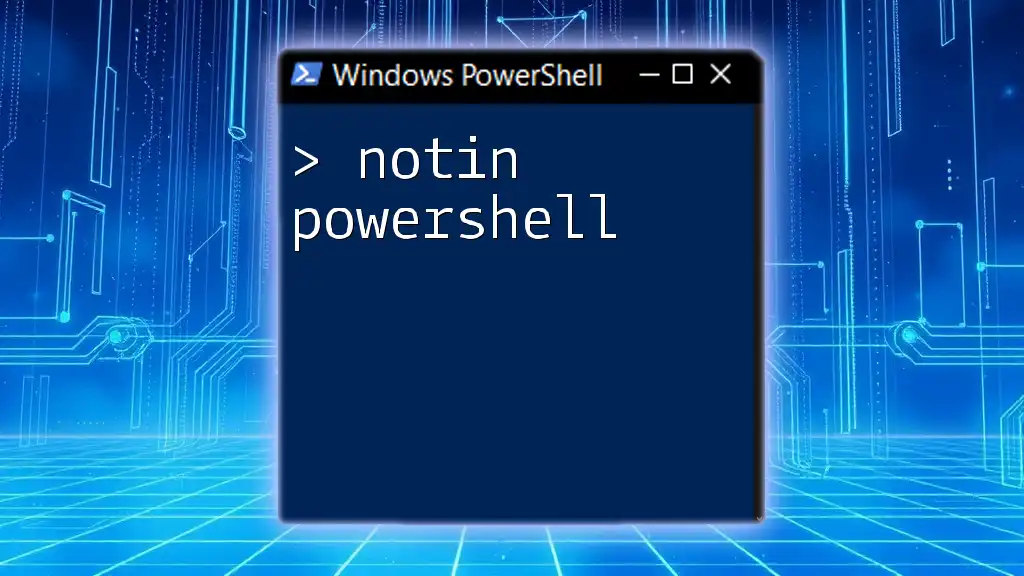
Synchronizing Directories
WinSCP allows you to synchronize directories between your local machine and the remote server seamlessly. Here’s how you can do it:
$session.SynchronizeDirectories([WinSCP.SynchronizationMode]::Local, "C:\local\path", "/remote/path")
This command will ensure that the local directory and the remote directory stay in sync, with the local directory mirroring the changes made.
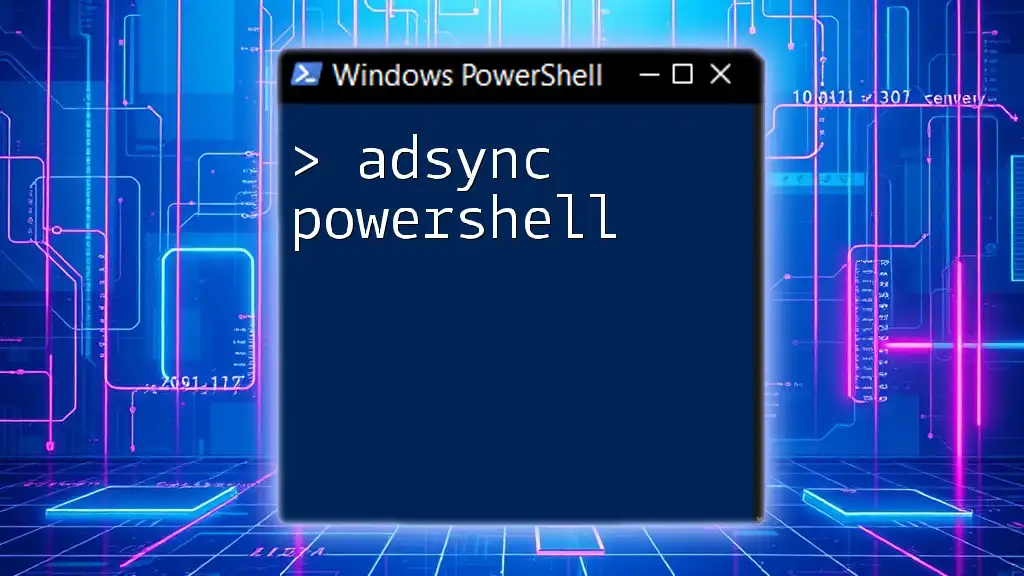
Handling Errors and Logs
In scripting, it's crucial to handle errors effectively. Utilizing try-catch blocks will allow you to catch any exceptions during the file transfer processes. Here's an example of how to implement error handling:
try {
# Your transfer code here
} catch {
Write-Host "Error occurred: $_"
}
Using `Write-Host`, you can print the error message to the console, allowing for easier debugging and monitoring.
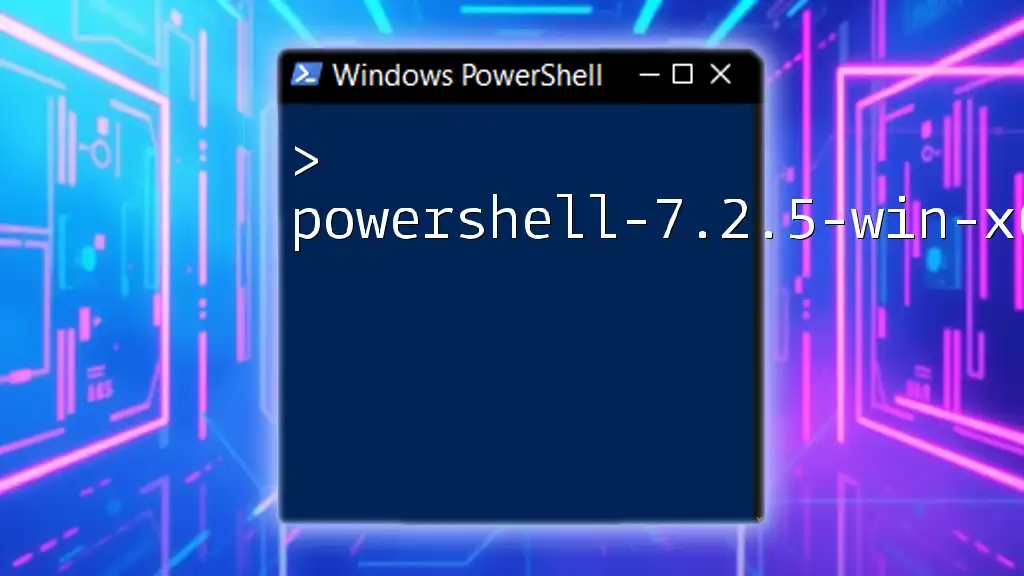
Using Command Line Options
WinSCP also allows you to run scripts via the command line. This is particularly useful for batch operations where GUI usage is not practical. The following command illustrates how to execute a script using PowerShell:
& "C:\Program Files (x86)\WinSCP\WinSCP.com" /script=C:\path\to\script.txt
This method enables running predefined commands through your PowerShell scripts, further enhancing automation capabilities.
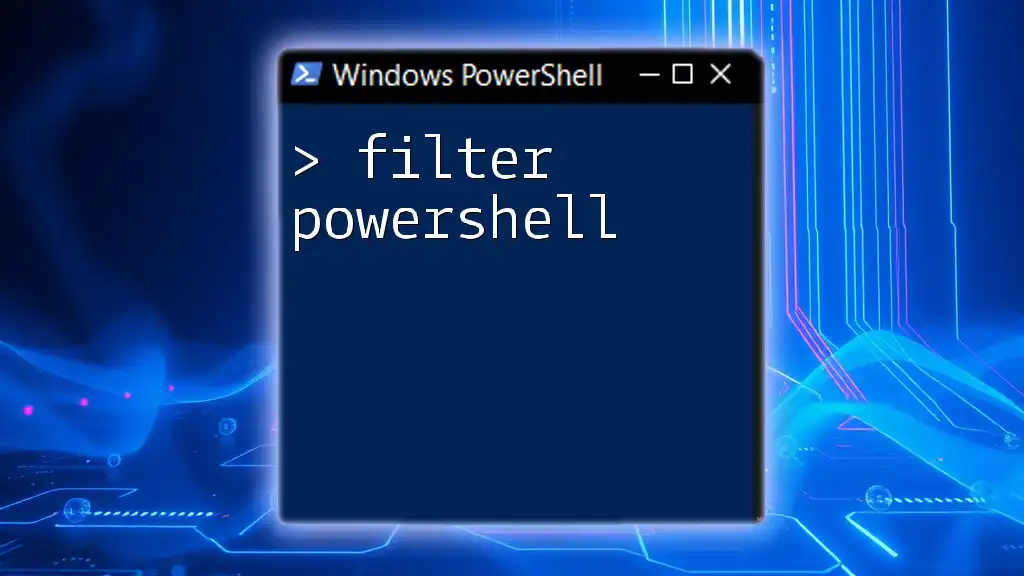
Creating Scheduled Tasks with PowerShell
To automate the file transfer processes, you can create scheduled tasks in Windows Task Scheduler. With PowerShell, this can be accomplished with the following example:
$action = New-ScheduledTaskAction -Execute "powershell.exe" -Argument "-File C:\path\to\YourScript.ps1"
This command will ensure that your PowerShell script runs at the specified schedule, making file transfers automatic and timely.
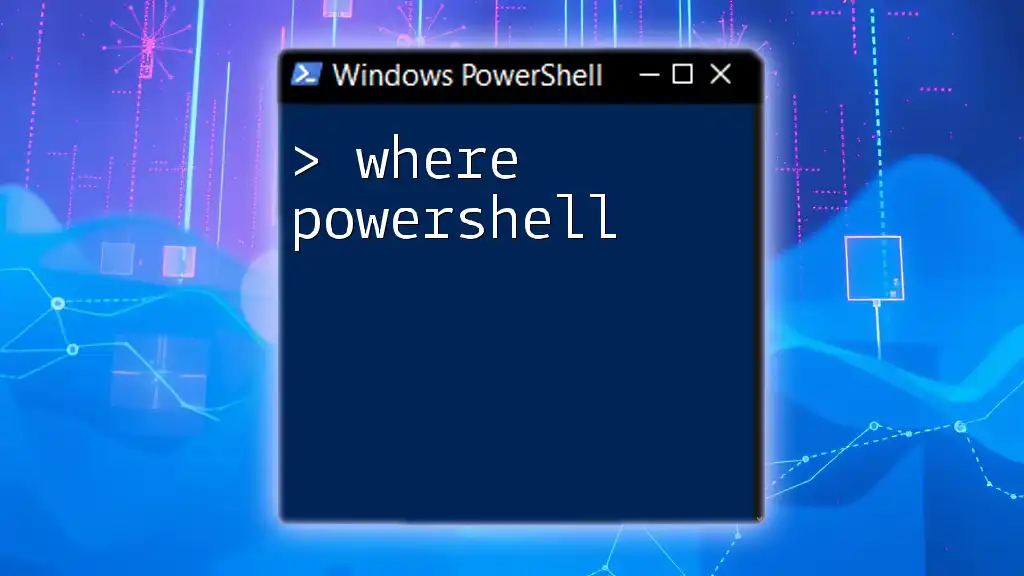
Recap of PowerShell WinSCP Usage
In this comprehensive guide, you learned how to integrate WinSCP with PowerShell effectively. From setting up connections and transferring files to handling errors and automating routines, the discussed concepts empower you to streamline your file management tasks with confidence.
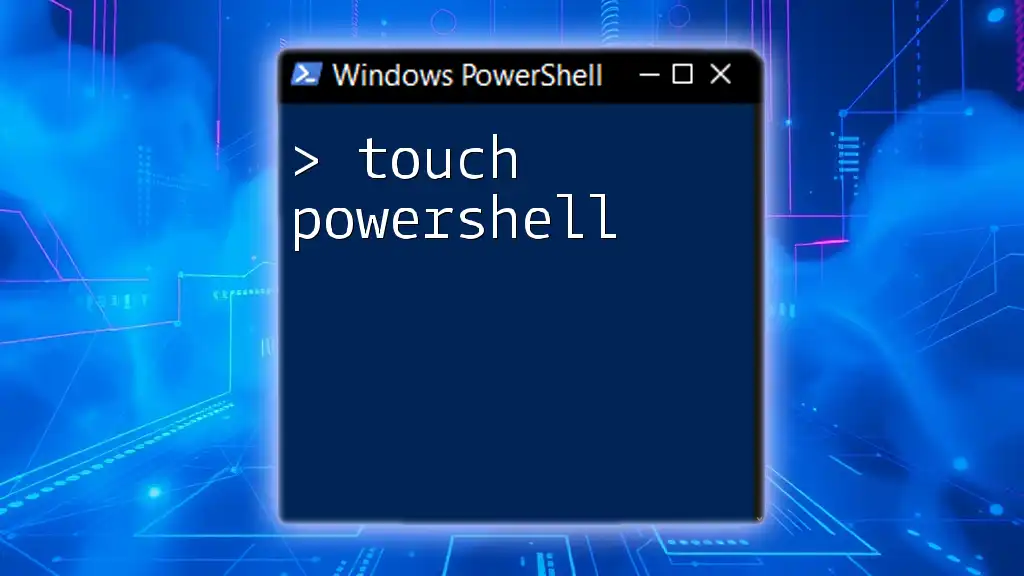
Further Learning Resources
To deepen your knowledge, consider exploring the WinSCP documentation and several PowerShell forums where users share best practices and troubleshooting tips. There are numerous online communities that focus on automation and scripting which can be invaluable to your learning journey.
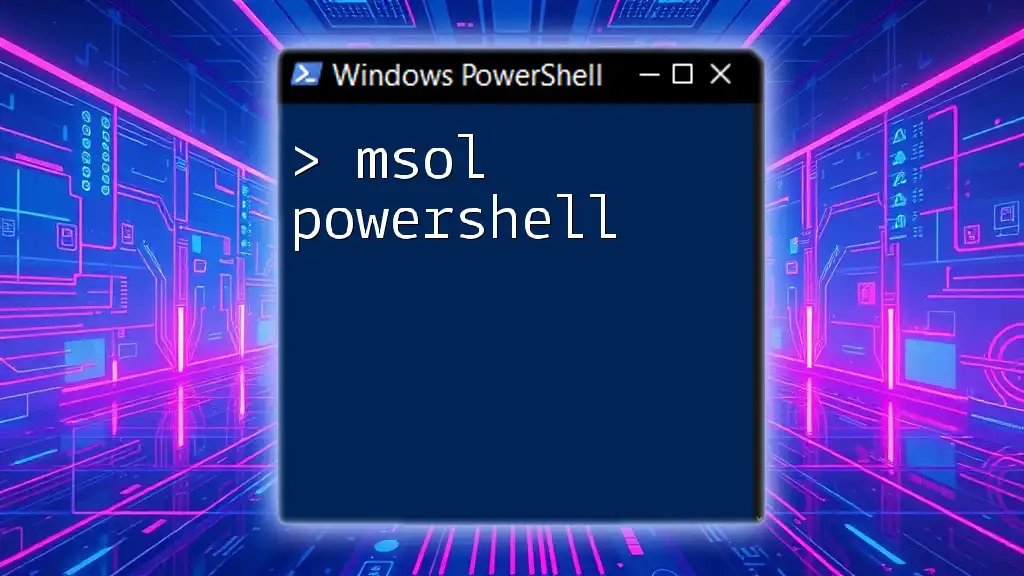
Join Our Community
We encourage you to engage with others learning PowerShell and WinSCP. Share your experiences, ask questions, and learn together. Subscribe to our platform for more tutorials, insights, and tips on using PowerShell for efficient system management.