The "contains" operator in PowerShell is used to determine if a collection includes a specified element, enabling efficient filtering and searching within data sets.
Here is a code snippet demonstrating its usage:
$fruits = @('Apple', 'Banana', 'Cherry')
$fruits -contains 'Banana' # Returns True
Understanding the `Contains` Method in PowerShell
What is the `Contains` Method?
The `Contains` method is a powerful feature in PowerShell that allows users to determine whether a specific element is present within a collection or string. This method checks the existence of the target within the specified context—whether you're dealing with a string or an array.
When utilizing the `Contains` method, it’s essential to understand the context in which you are operating. For example, when working with strings, `Contains` will search for a substring, while in arrays or collections, it will look for a specific item.
PowerShell Data Types and the `Contains`
In PowerShell, `Contains` can be employed with several data types. Common examples include:
- Strings: Used for verifying whether a substring is part of a larger string.
- Arrays: Useful for checking if a particular element exists in an array collection.
- Lists and Hashtables: Flexible structures can also utilize the `Contains` method.
Understanding how `Contains` operates within these data types is crucial for writing efficient scripts.
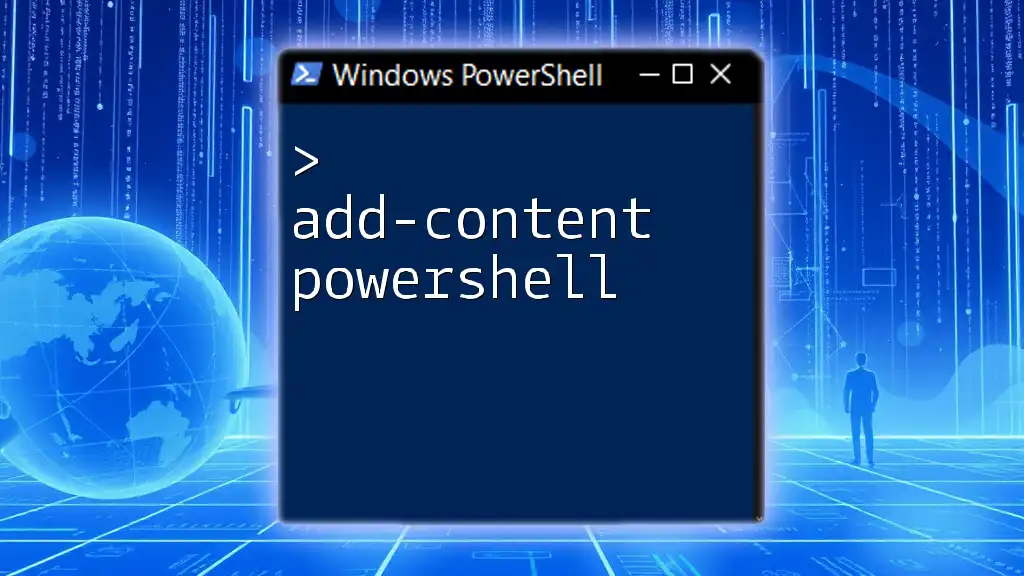
Using `Contains` in PowerShell
The `if` Statement with `Contains`
Using `if` with Strings
You can utilize the `if` statement in conjunction with the `Contains` method on strings to perform conditional actions based on the presence of specific text.
For example, consider the following code snippet:
$text = "Learn PowerShell quickly"
if ($text.Contains("PowerShell")) {
Write-Output "The text contains 'PowerShell'."
}
In this example, the condition checks whether the string $text includes the substring "PowerShell". If true, the script outputs a confirmation message.
It's also worth noting that the `Contains` method in this context is case-sensitive. Therefore, "powershell" (lowercase) would not match "PowerShell". To avoid issues with case sensitivity, you might want to convert both strings to the same case:
if ($text.ToLower().Contains("powershell")) {
Write-Output "The text contains 'PowerShell' (case-insensitive check)."
}
Using `if` with Arrays
When working with arrays, the `Contains` method can effectively verify the presence of an element:
$array = @("Windows", "Linux", "PowerShell")
if ($array.Contains("PowerShell")) {
Write-Output "Array contains 'PowerShell'."
}
In this code, the conditional expression checks if "PowerShell" exists in the array $array. This becomes particularly useful in scenarios where you have large datasets and need to filter or verify specific elements.
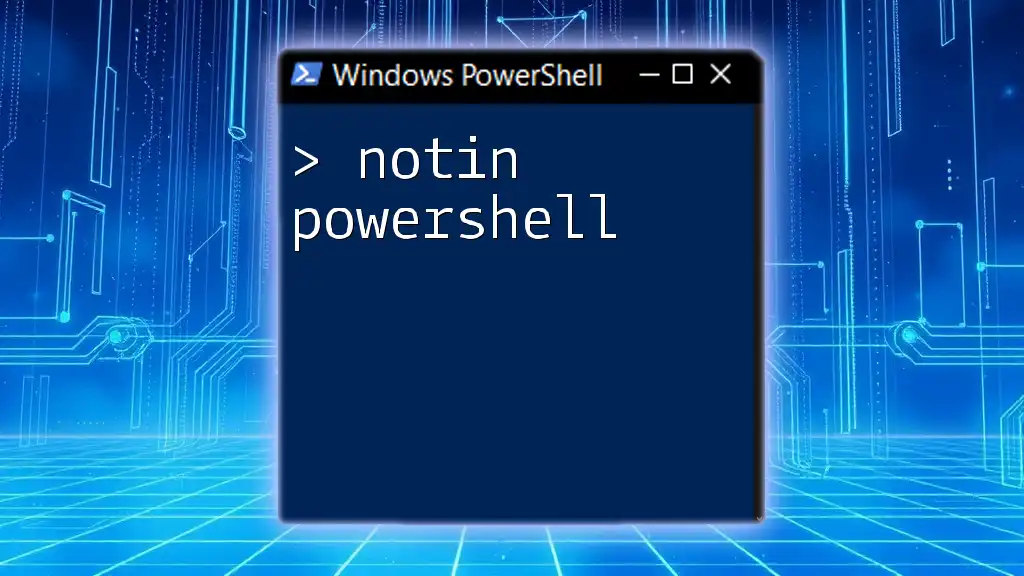
Where-Object with `Contains`
Filtering with `Where-Object`
The `Where-Object` cmdlet can be paired with `Contains` to filter collections based on specific criteria. This combination is powerful for dealing with data filtering tasks.
For instance, this code snippet demonstrates how to use it:
$users = @("Alice", "Bob", "Charlie", "David")
$matched = $users | Where-Object { $_.Contains("a") }
$matched
Here, Where-Object filters the user names and returns only those that contain the letter "a". The underscore (`$_`) represents the current object in the pipeline, making it versatile for processing multiple entries.
This method could be applied practically in situations such as managing user accounts, where you may want to segment users based on specific characteristics.
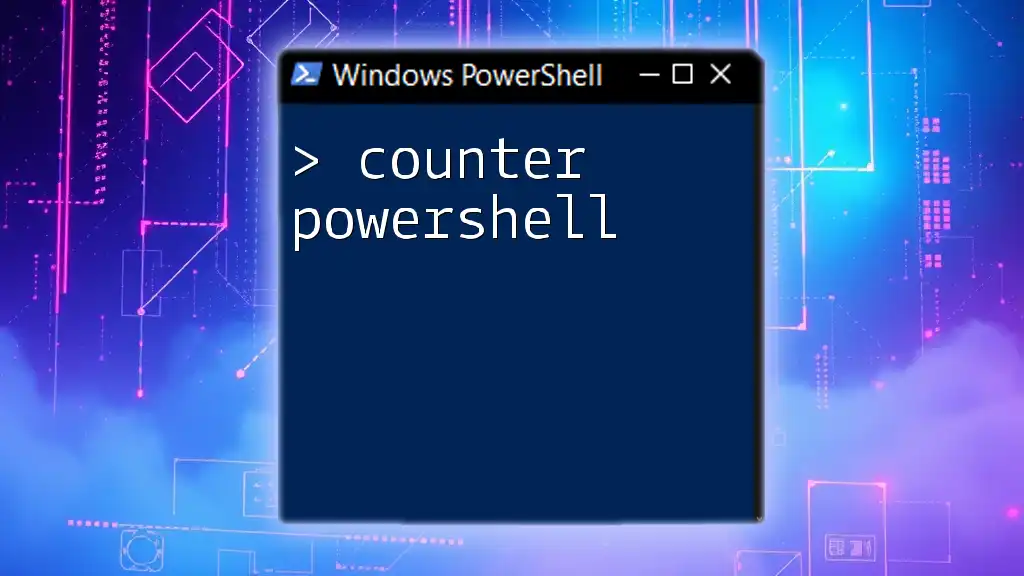
Advanced `Contains` Usage
Multiple Conditions with `if Contains`
Enhancing your PowerShell scripts with multiple conditions can lead to more complex and useful checks. For example:
$teams = @("TeamA", "TeamB", "Development", "Marketing")
if ($teams.Contains("Development") -and $teams.Contains("Marketing")) {
Write-Output "Both teams are present."
}
This snippet evaluates whether both "Development" and "Marketing" teams are included in the $teams array. Such logical operators enable powerful validations in your scripts.
Using Regex with `Contains`
While `Contains` is straightforward, sometimes you may require more flexibility—this is where regular expressions (regex) come into play. Regular expressions can perform more complex pattern matching than the simple `Contains` method.
For instance, consider the following example:
$text = "PowerShell is a powerful scripting language."
if ($text -match "Power.*") {
Write-Output "The text contains a match for Power."
}
In this case, the `-match` operator checks for any occurrences that start with "Power" followed by any characters. This method is useful when dealing with flexible text patterns that need validation.
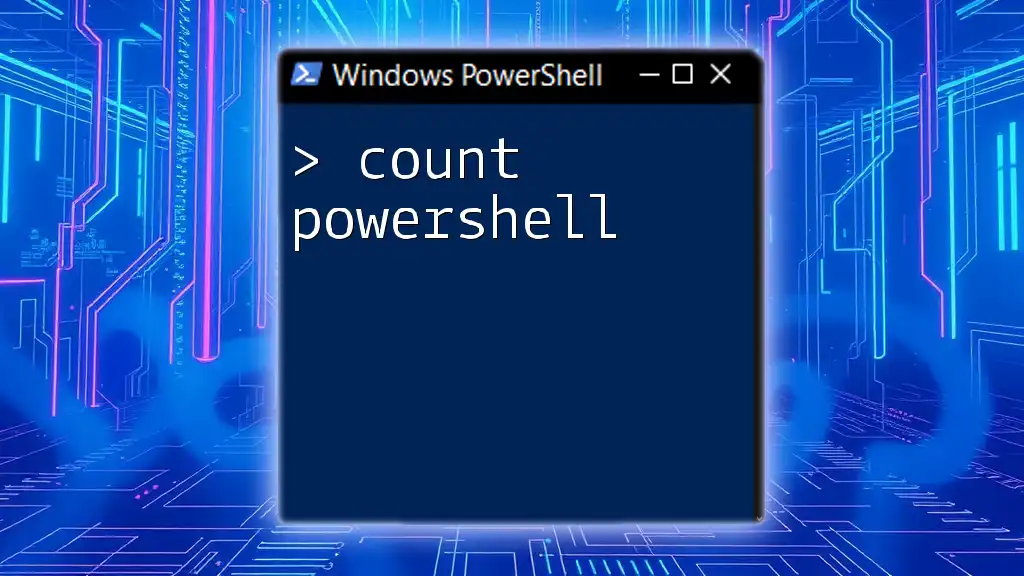
Common Pitfalls and Troubleshooting
Case Sensitivity Issues
As mentioned previously, the `Contains` method is inherently case-sensitive when used with strings. If this is not handled correctly, it can lead to unexpected results.
Always consider using `.ToLower()` or `.ToUpper()` on both your target string and the search string to mitigate case sensitivity issues.
Performance Considerations
When checking large datasets with `Contains`, be mindful of performance—particularly in scripts executed in real-time environments. For large arrays or collections, `Contains` may introduce noticeable delays.
To optimize performance, try limiting the size of the dataset before performing checks, or consider more efficient data structures if applicable.
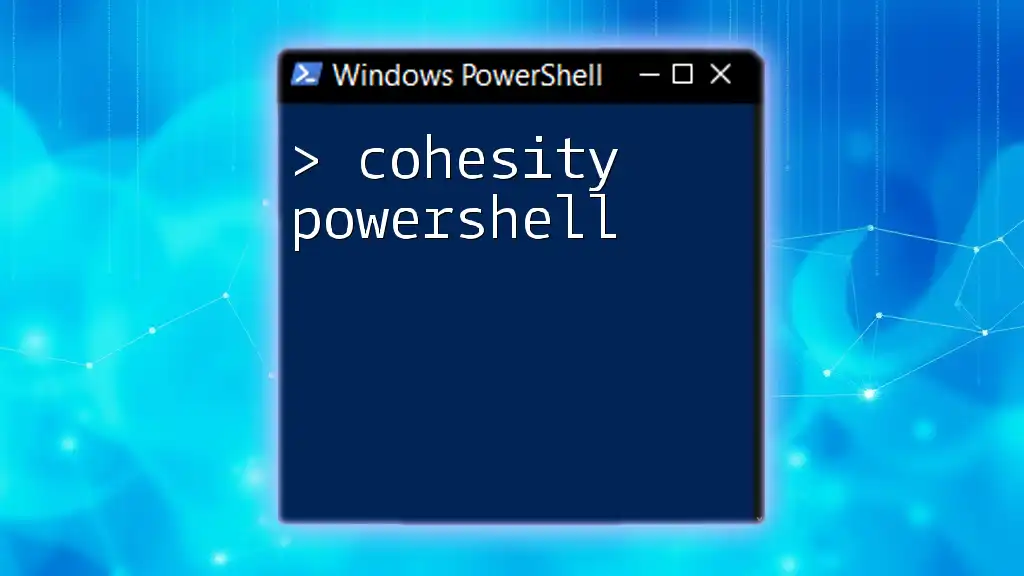
Conclusion
In conclusion, understanding how to use the `Contains` method in PowerShell can significantly enhance your scripting capabilities. As demonstrated, whether you're checking string values or filtering arrays, leveraging the power of `Contains` allows for concise and effective programming.
Now that you’re equipped with these methods and insights, experiment with them in your own scripts to become proficient in utilizing `Contains in PowerShell`. Keep pushing your PowerShell skills forward!