In PowerShell, managing credentials allows you to securely store and retrieve user credentials for authentication purposes, enhancing script security. Here’s an example of how to create a secure credential object:
$credential = Get-Credential -Message "Please enter your credentials"
Understanding Credentials in PowerShell
What Are Credentials?
In PowerShell, credentials refer to the user authentication information, typically composed of a username and a password. They are represented by the Credential object in PowerShell, which encapsulates the necessary information to authenticate a user or service. This object is crucial when executing commands that require elevated privileges or when accessing remote systems.
The primary properties of a Credential object include:
- UserName: The name of the user account.
- Password: A secure string that contains the user’s password. This ensures that sensitive data is not exposed in plain text.
Example: To view the basic structure of a Credential object, you might see something like this after creating it:
$cred = Get-Credential
$cred.UserName
$cred.Password
Why Use Credentials?
Using credentials is essential for maintaining security and access control. In many scenarios, sensitive actions must be restricted to authorized personnel. By employing credentials in your PowerShell scripts, you minimize the risk of unauthorized access.
Additionally, handling credentials safely allows you to develop scripts that can operate across different user contexts without exposing sensitive information directly within your code. This promotes good security practices in your automation tasks.
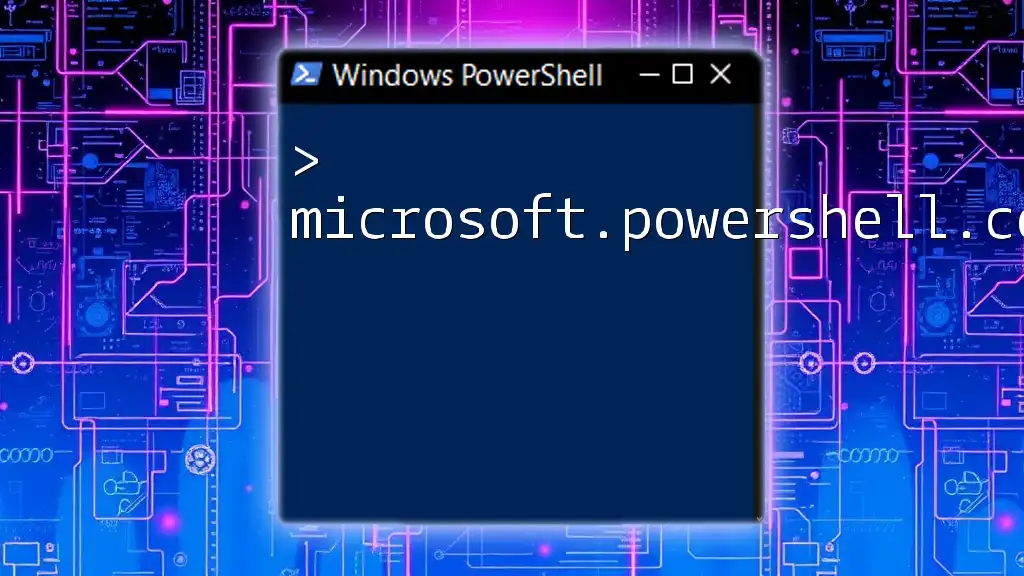
Creating Credentials in PowerShell
Using `Get-Credential`
One of the primary methods to obtain user credentials in PowerShell is the `Get-Credential` cmdlet. This cmdlet prompts the user to enter their username and password through a dialog box.
Code Snippet:
$cred = Get-Credential
When this command is executed, a secure input dialog will appear, asking for the credentials. After user input, `$cred` will hold the Credential object containing the username and the secure password.
Creating Credential Objects Programmatically
In cases where you need to define credentials in your scripts without user interaction, you can create Credential objects programmatically using the New-Object cmdlet.
Using the `New-Object` Cmdlet:
Code Snippet:
$username = "username"
$password = ConvertTo-SecureString "password" -AsPlainText -Force
$cred = New-Object System.Management.Automation.PSCredential($username, $password)
In this code:
- You first define the username.
- The password is converted to a secure string using `ConvertTo-SecureString`, which ensures that plaintext passwords are not stored in memory.
- Finally, the Credential object is created using `New-Object`, allowing you to use it in subsequent commands.
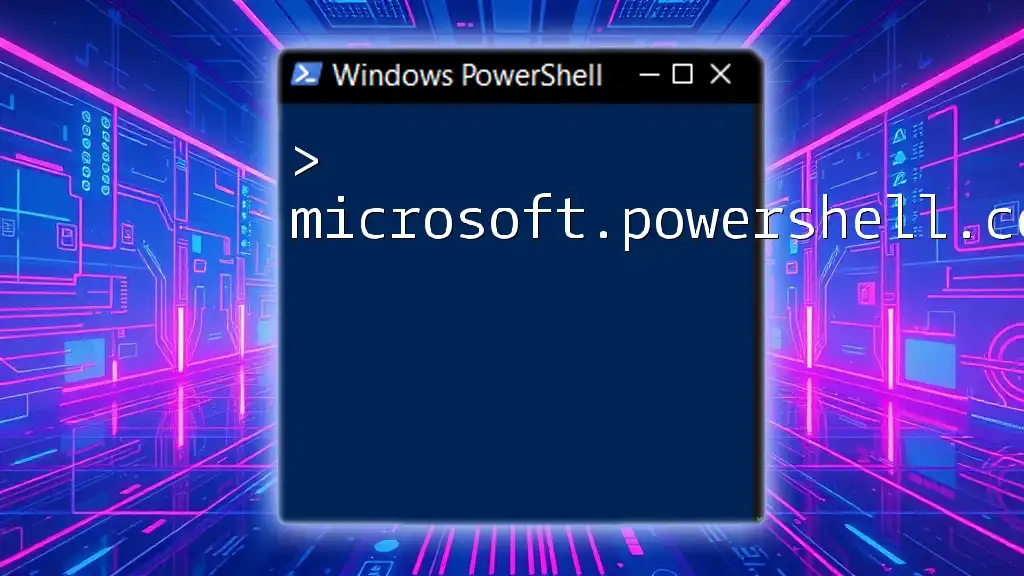
Storing Credentials Securely
Using the Windows Credential Manager
Windows provides a secure way to store and retrieve user credentials via the Windows Credential Manager. This feature allows users to save credentials securely so they can be accessed later without needing to re-enter them.
To store credentials securely, you can pass the Credential object to the Credential Manager.
Code Snippet: Storing a Credential object to an XML file:
$cred | Export-Clixml -Path "C:\path\to\credential.xml"
Storing credentials this way allows you to retrieve them in a secure format later, ensuring they are protected from unauthorized access.
Alternatives for Storing Credentials
While storing credentials in the Windows Credential Manager or as encrypted files is generally recommended, you can also store them in plaintext formats for convenience. However, this approach has significant security risks.
Best Practice: Always opt for encrypted formats over plaintext whenever possible. This minimizes the chances of exposing sensitive information.
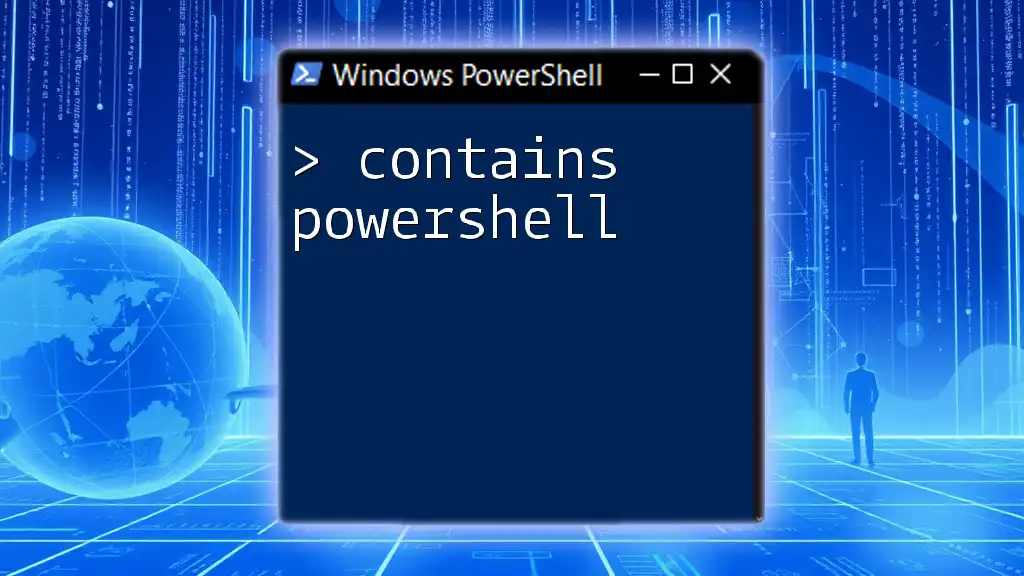
Using Credentials in PowerShell Cmdlets
Common Cmdlets That Require Credentials
Many PowerShell cmdlets require the use of credentials for actions such as remoting or accessing secured resources. Common cmdlets that utilize credentials include:
- `Invoke-Command`
- `Enter-PSSession`
- `Test-Connection`
Code Snippet: Using credentials with `Invoke-Command`:
Invoke-Command -ComputerName "RemotePC" -Credential $cred -ScriptBlock { Get-Process }
In this command:
- Invoke-Command allows you to run commands on a remote machine.
- The `-ComputerName` parameter specifies the target machine, and the `-Credential` parameter provides the necessary authentication.
The ScriptBlock `{ Get-Process }` executes a command remotely, retrieving a list of running processes on the remote system.
Passing Credentials Between Cmdlets
One effective method of utilizing credentials is passing them across multiple cmdlets within a single script. Once you acquire a Credential object, you can reuse it seamlessly in subsequent commands.
Example:
$cred = Get-Credential
Invoke-Command -ComputerName "PC1" -Credential $cred -ScriptBlock { Get-Service }
Invoke-Command -ComputerName "PC2" -Credential $cred -ScriptBlock { Get-Process }
In this example, the same Credential object `$cred` is reused to execute commands on two different remote computers, demonstrating efficiency and reducing the need for redundant credentials prompts.
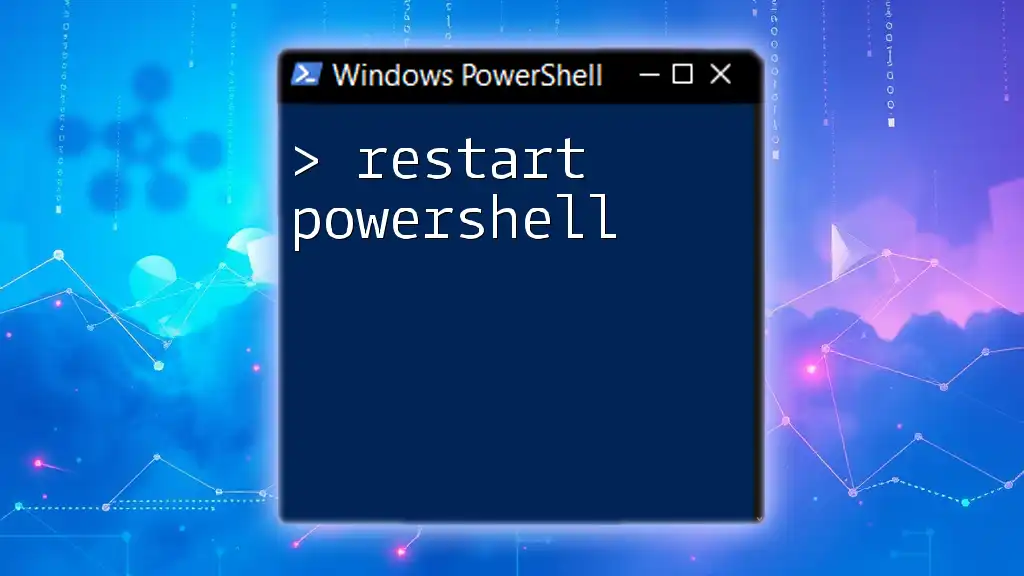
Troubleshooting Credential Issues
Common Errors Related to Credentials
When working with credentials in PowerShell, users may encounter various errors, such as:
- Access Denied: This typically occurs when the provided credentials do not have the required permissions.
- Invalid Credentials: This indicates that the username or password was entered incorrectly.
Steps to Resolve These Issues:
- Double-check the username and password for typos.
- Ensure that the user account is enabled and has the necessary access rights.
- Verify that the target system allows connections from the user account.
Best Practices for Handling PowerShell Credentials
To ensure that your scripts maintain a high degree of security when dealing with credentials, consider the following best practices:
- Limit Credential Exposure: Avoid exposing credentials directly in your scripts; use secure methods to prompt for input or store securely.
- Use Least Privilege Principle: Always provide the minimum permissions necessary for the task at hand.
- Regularly Rotate Credentials: To mitigate risks, regularly update and rotate your credentials to ensure security.
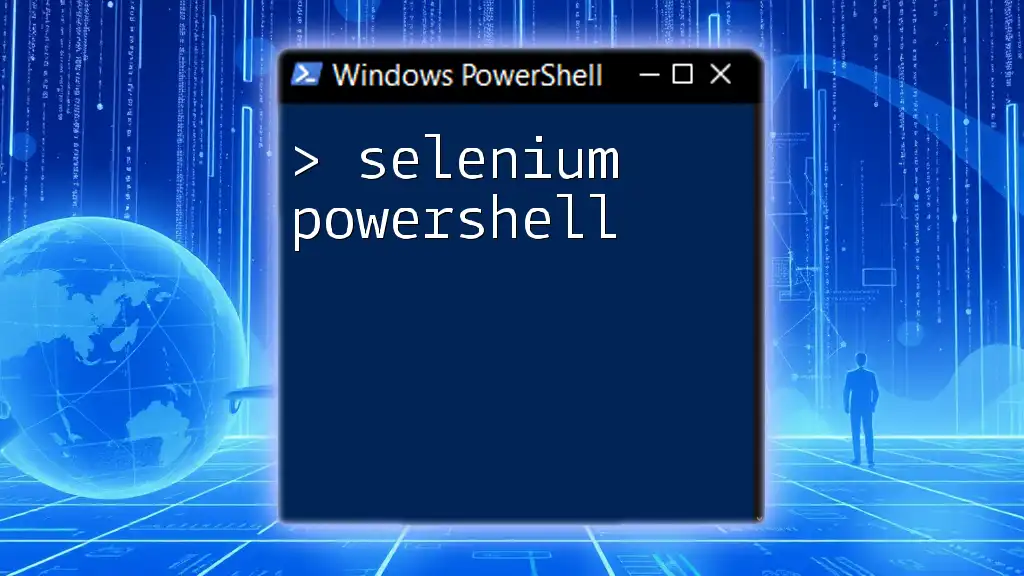
Conclusion
In the world of automation and scripting, effectively managing credentials in PowerShell is critical for maintaining security and access control. From creating and storing credentials to utilizing them in cmdlets, the methodologies discussed here provide a solid foundation for secure scripting practices.
By following the best practices and understanding how to work with credentials in PowerShell, you can elevate your automation scripts and safeguard sensitive information, ultimately leading to more robust and secure PowerShell operations.
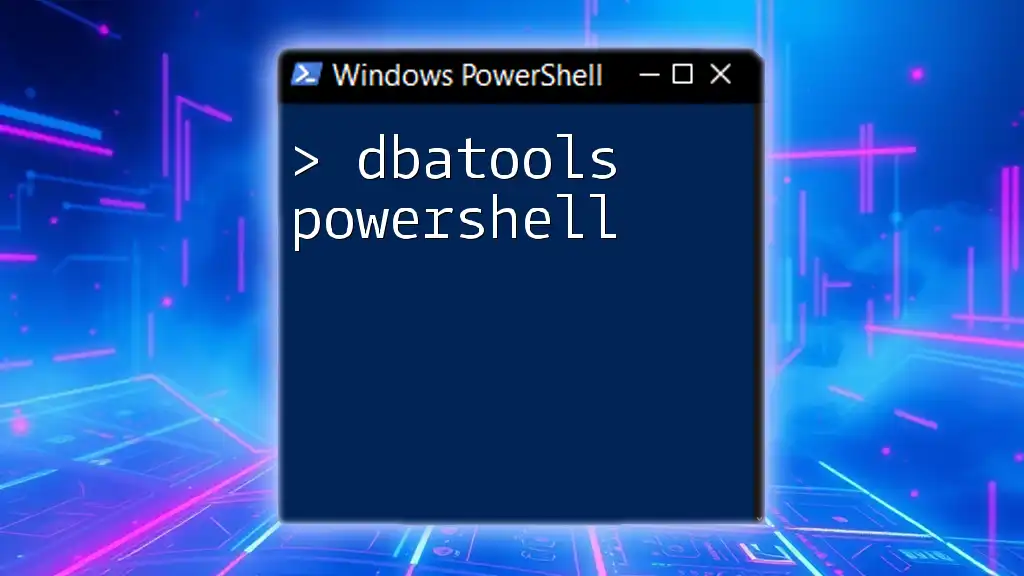
Additional Resources
For in-depth learning, consider exploring the official Microsoft documentation on security and credentials. This will enhance your understanding and provide more advanced techniques for credential management in PowerShell.