The `Add-Content` cmdlet in PowerShell is used to append content to a file without overwriting existing data.
Add-Content -Path "C:\path\to\your\file.txt" -Value "This is the new content."
What is `Add-Content`?
`Add-Content` is a powerful cmdlet in PowerShell that allows users to append data to existing files easily. Its primary purpose is to add textual content or object output to a specified file without overwriting the contents that are already present. This functionality is critical for maintaining logs, collecting outputs from commands, or simply keeping a record of ongoing tasks in a straightforward manner.
The benefits of using `Add-Content` are manifold, including:
- Ease of Use: The command is straightforward, making it accessible for beginners and professionals alike.
- Flexibility: You can append various data types, from strings to arrays and even command outputs, expanding your scripting capabilities in PowerShell.
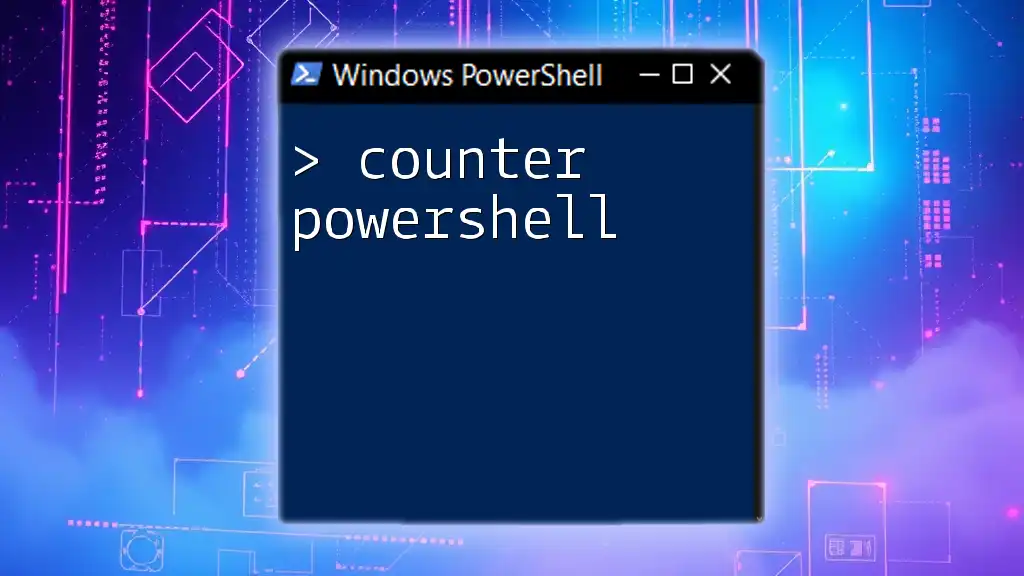
Syntax of `Add-Content`
To effectively utilize the `Add-Content` cmdlet, it is essential to understand its syntax:
Add-Content -Path <string> -Value <object>
Description of Parameters
- `-Path`: This parameter specifies the file's path where you want to add content. The path must include the file name and its extension.
- `-Value`: Here, you provide the actual content you wish to append. The value can be a single object, a string, or even an array.
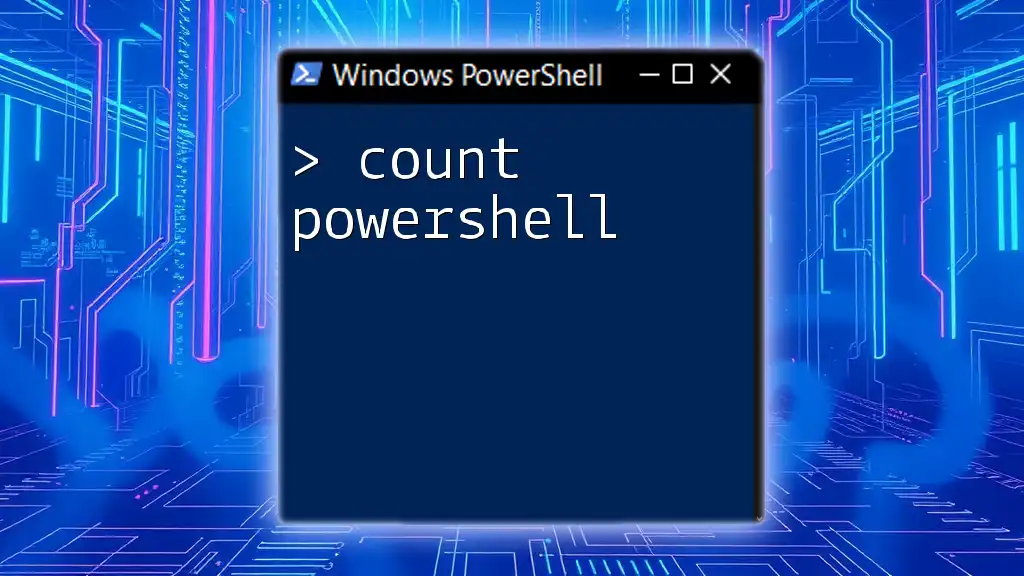
Basic Usage of `Add-Content`
To get started with `Add-Content`, here are some foundational examples that illustrate its usage.
Example 1: Appending a Simple String to a Text File
To append a simple string, use the following command:
Add-Content -Path "C:\example\sample.txt" -Value "Hello, World!"
This command will add "Hello, World!" to the end of `sample.txt`. If the file doesn’t exist, PowerShell will create it automatically, demonstrating a user-friendly feature of this cmdlet.
Example 2: Appending Multiple Lines of Text
You can also append multiple lines at once. For instance:
$lines = "First line`nSecond line"
Add-Content -Path "C:\example\sample.txt" -Value $lines
The backtick (`n) is a special character that denotes a new line, allowing you to append text in an organized manner.
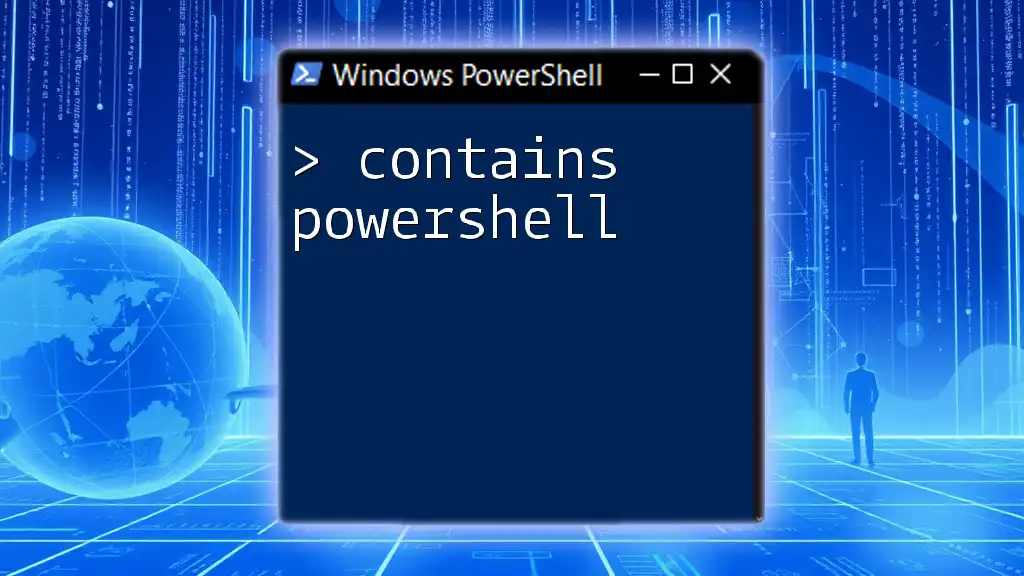
Appending Different Data Types
One of the remarkable aspects of `Add-Content` is its ability to handle different data types seamlessly.
Example 3: Appending the Output of a Command
You can append the output of a command to a file directly. For example:
Get-Process | Add-Content -Path "C:\example\processes.txt"
This command retrieves the list of currently running processes and appends that list to `processes.txt`. It's effective for monitoring and logging system activities.
Example 4: Appending an Array of Strings
Appending arrays is just as easy. For instance:
$array = "Item 1", "Item 2", "Item 3"
Add-Content -Path "C:\example\array.txt" -Value $array
In this case, each item in the array will be appended as a new line in `array.txt`. This flexibility allows for structured and organized data logs.
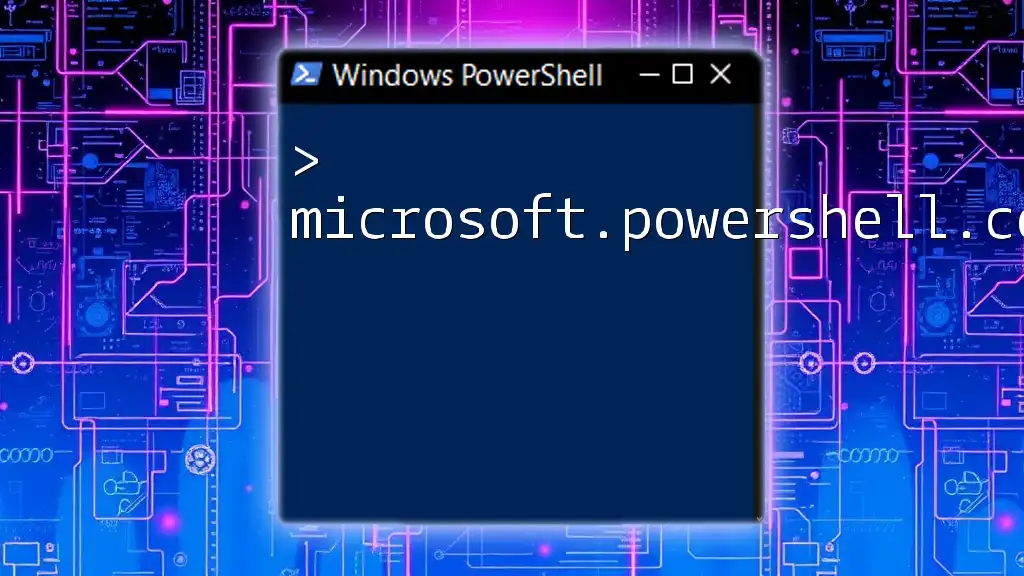
Working with File Paths
When working with `Add-Content`, using correct file paths is crucial. The path you provide can be absolute or relative.
- Absolute Path: Specifies the complete path from the root of the file system, e.g., `C:\example\sample.txt`.
- Relative Path: This path is relative to the current working directory, like `.\sample.txt`.
To ensure a smooth operation, verify that the target path exists. If you try to append to a file in a non-existing directory, PowerShell will return an error.
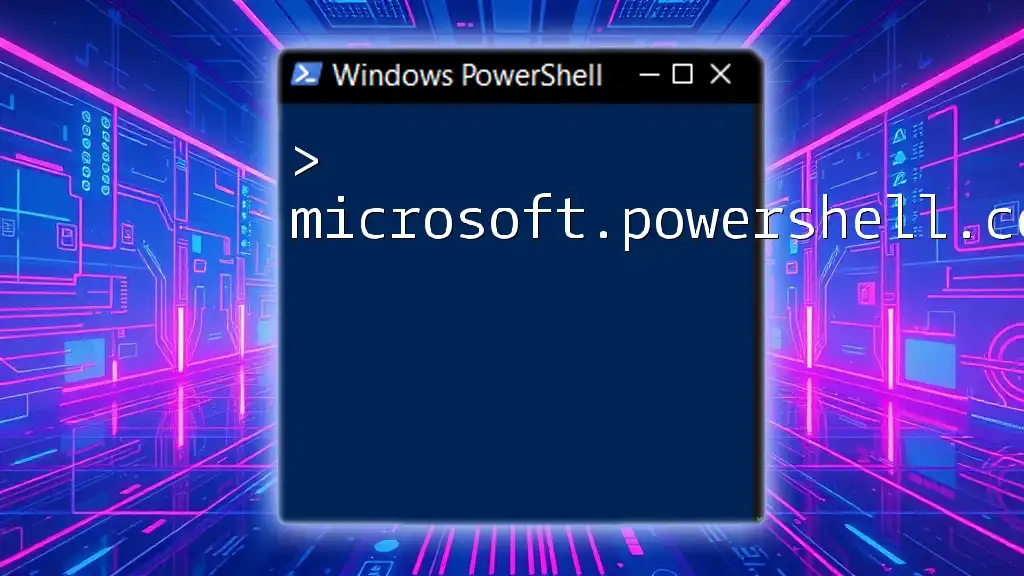
Using `Add-Content` with Text Files
When working exclusively with text files, there are a few guidelines to consider for optimal file management.
Example 5: Appending Collections of Data
For instance, to append a collection of strings to a text file:
$data = "Item A", "Item B", "Item C"
Add-Content -Path "C:\example\data.txt" -Value $data
This will add each item in the `$data` array to `data.txt`, one per line, effectively keeping your records well-organized.
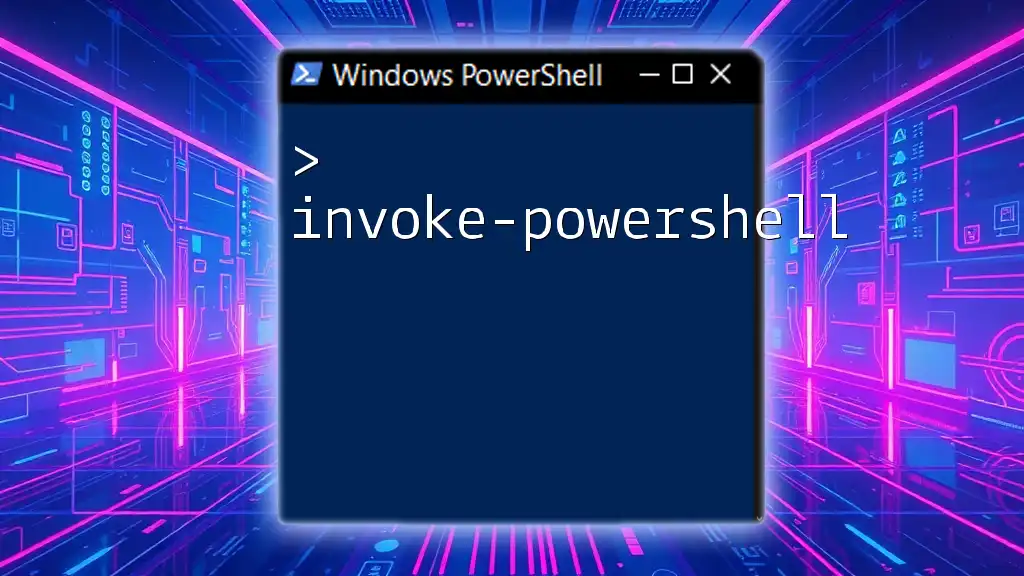
Error Handling with `Add-Content`
As with any command, it's essential to handle errors that may arise during usage.
Common errors include:
- File Permission Issues: You may not have the necessary permissions to write to a specific location.
- Non-Existing Paths: If the path specified does not exist, an error will occur.
Example 6: Using `Try-Catch` to Manage Errors
To gracefully handle errors when using `Add-Content`, consider employing error handling mechanisms. For instance:
Try {
Add-Content -Path "C:\nonexistent\file.txt" -Value "Hello!"
} Catch {
Write-Host "An error occurred: $_"
}
This code snippet attempts to append content to a file and, if it fails, catches the error and displays a message. This approach can save time and improve the resilience of your scripts.
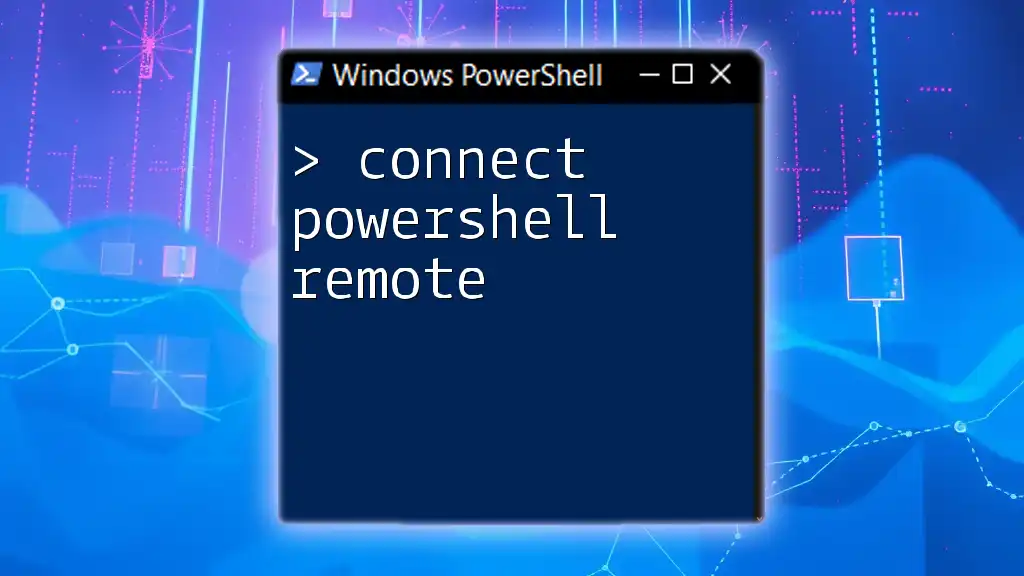
Performance Considerations
When appending large amounts of data using `Add-Content`, performance can become a concern. Some tips for optimizing its usage include:
- Minimizing Multiple Calls: If you need to append large data, it's more efficient to collect it all first and use a single `Add-Content` call.
- Using Batching Techniques: Instead of appending line-by-line, gather your lines in an array and append them at once.
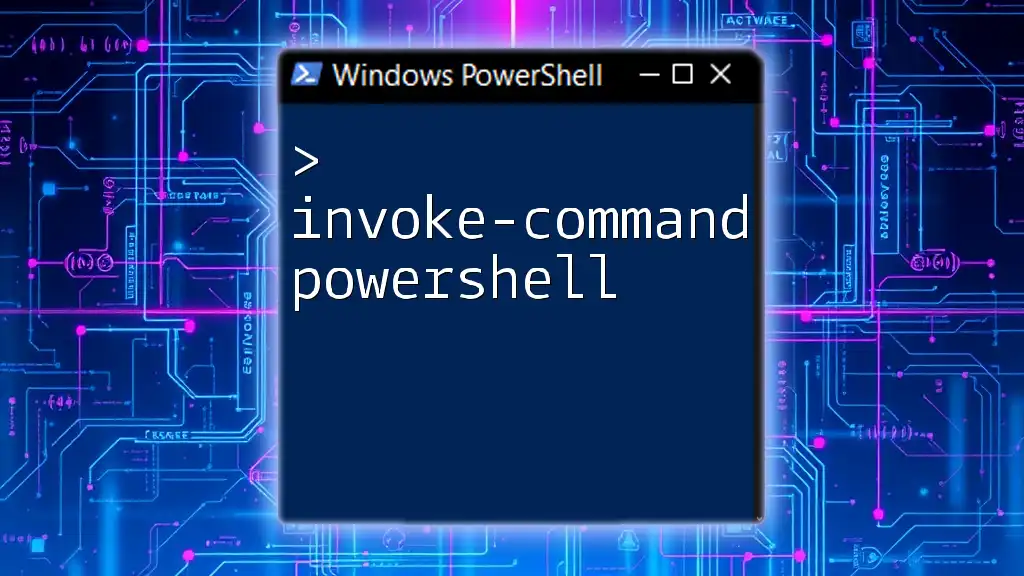
Alternatives to `Add-Content`
While `Add-Content` is potent for appending data, there are alternatives to consider depending on your needs:
-
`Set-Content`: This cmdlet is used for replacing existing content rather than appending, so if overwriting is your goal, `Set-Content` is appropriate.
-
`Out-File`: This offers more options for output formatting and is beneficial when exporting content for reports or logs.
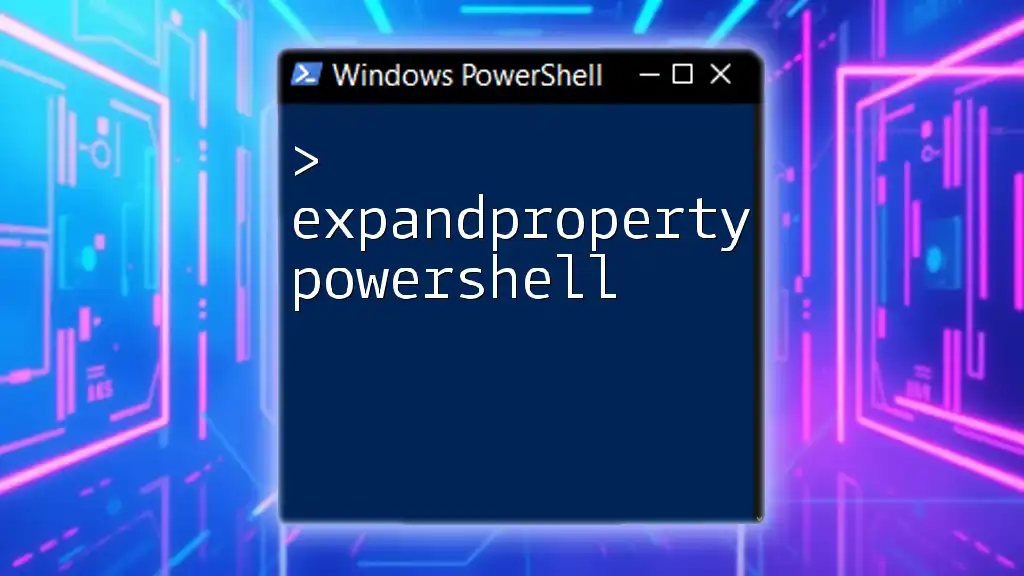
Best Practices for Using `Add-Content`
To maximize the effectiveness of using `Add-Content`, implement best practices:
- Effective File Management: Organize your files logically and separate different types of logs and outputs.
- Clarity in Scripts: Ensure your scripts are clear by using descriptive file paths and commenting where necessary.
- Documentation: Document your code effectively to future-proof your scripts and make it easier for others to understand.
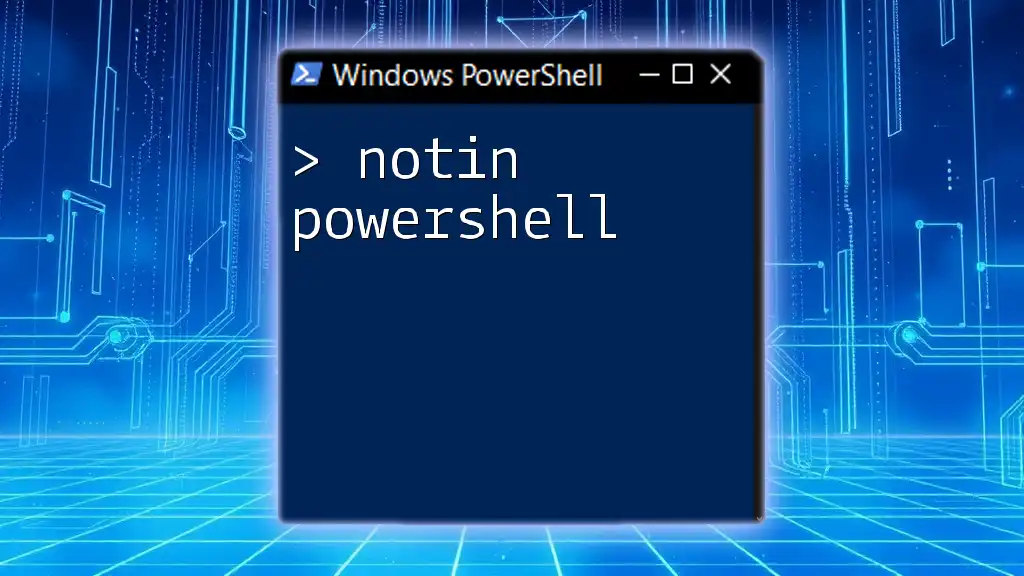
Conclusion
Understanding `Add-Content` in PowerShell is essential for anyone looking to manage file content efficiently. Its ease of use and versatility make it an invaluable tool for scripting and automation. Regular practice will enhance your skills and help you integrate this cmdlet into your daily tasks seamlessly. Consider exploring further PowerShell concepts to enrich your knowledge base and scripting abilities.
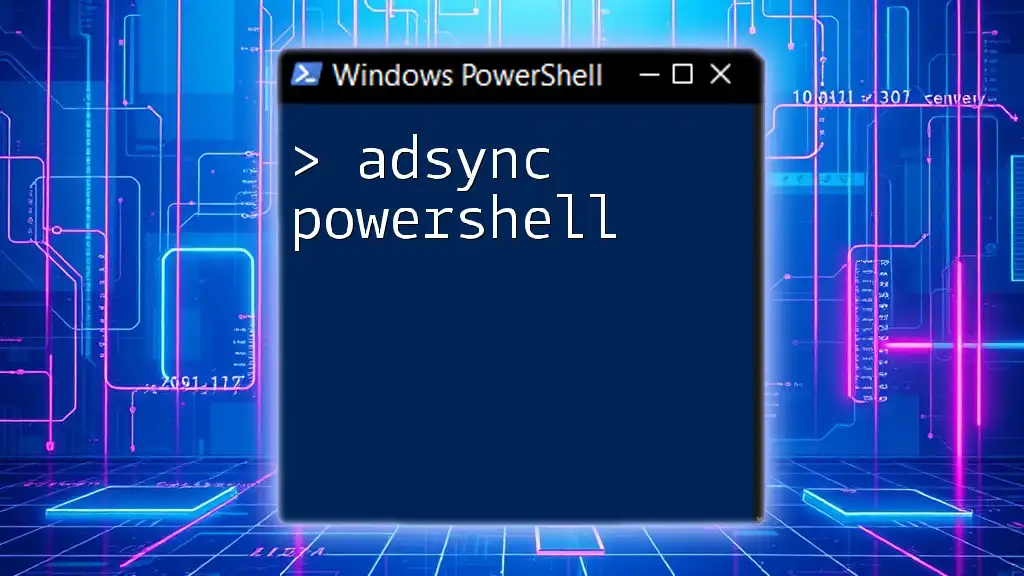
Additional Resources
To delve deeper into `Add-Content` and its capabilities, check out the official Microsoft documentation, PowerShell community forums, and recommended tutorials. Engaging with the community can bring valuable insights and assist you in mastering PowerShell scripting.