The `Add-Type` cmdlet in PowerShell allows you to define and add custom .NET types (classes) to your PowerShell session for enhanced functionality.
Add-Type -TypeDefinition "public class Hello { public static void Greet() { System.Console.WriteLine('Hello, World!'); } }"
[Hello]::Greet()
Understanding the Basics of Add-Type
What Does Add-Type Do?
The `Add-Type` cmdlet in PowerShell serves a critical purpose: it allows you to define and create .NET types directly within your PowerShell scripts. This can include defining new classes, structs, interfaces, or enums. By using `Add-Type`, you can enrich your scripts with C# capabilities, making them more powerful and versatile.
For example, you might want to encapsulate complex logic within a custom class, enabling easier reuse and readability in your scripts.
Syntax of Add-Type
The syntax for using `Add-Type` is straightforward, but understanding its parameters is essential for effective implementation:
Add-Type -TypeDefinition <string> [-Language <string>] [-Name <string>] [-Namespace <string>]
- `-TypeDefinition`: This is the core parameter, where you provide a string containing your type definition (which is typically C# code).
- `-Language`: By default, PowerShell assumes C# syntax, but you can specify other languages if needed.
- `-Name`: Use this to define a name for your type.
- `-Namespace`: This allows you to define a custom namespace that helps organize your types effectively.
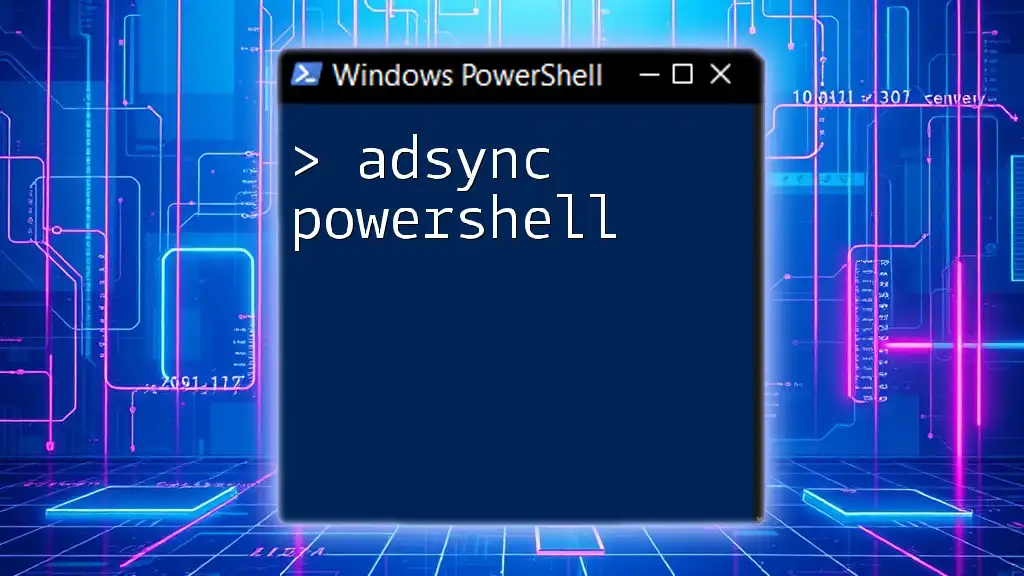
When to Use Add-Type
Creating Custom Classes
One of the most powerful features of `Add-Type` is the ability to create custom classes. This can help encapsulate functionality and make your code more modular.
Example:
$typeDefinition = @"
public class Calculator {
public [int] Add([int] $a, [int] $b) {
return $a + $b;
}
}
"@
Add-Type -TypeDefinition $typeDefinition -Language CSharp
$calc = New-Object Calculator
$result = $calc.Add(5, 3)
Write-Host "Result: $result"
In this example, we defined a simple class called `Calculator` with an `Add` method. When you run this code, it will output:
Result: 8
This shows how `Add-Type` can enable you to define your logic encapsulated within a class.
Using .NET Framework Classes
Another strong use case for `Add-Type` is leveraging existing .NET Framework classes. This allows you to include pre-built functionalities without having to reinvent the wheel.
Example:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.MessageBox]::Show("Hello World!")
This code snippet will display a message box with "Hello World!" when executed. It highlights how `Add-Type` can quickly incorporate powerful .NET functionalities into your scripts.
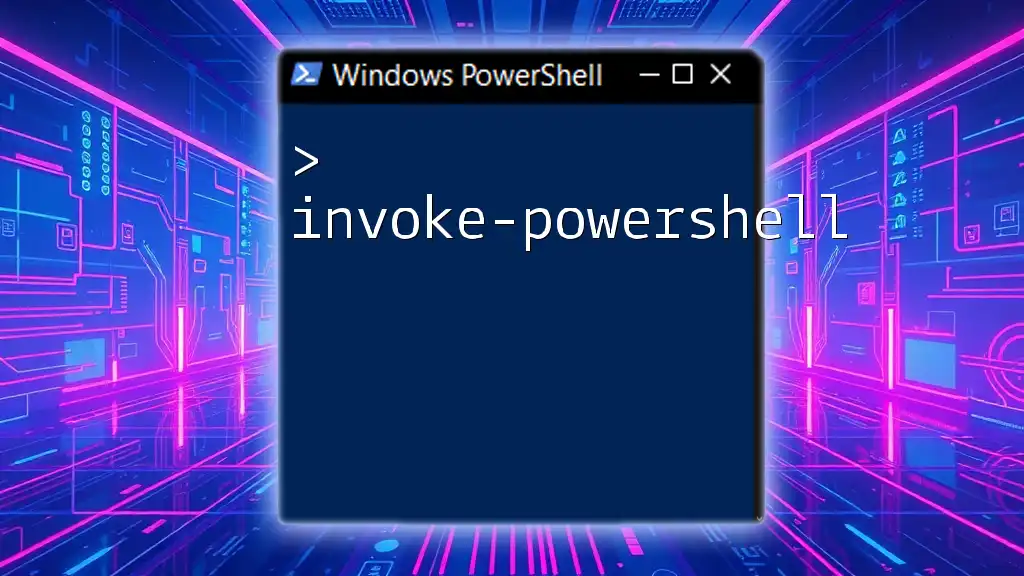
Advanced Usage of Add-Type
Incorporating External Assemblies
Sometimes you may need to use custom .NET assemblies stored in `.dll` files. The `Add-Type` cmdlet makes it easy to accomplish this.
Example:
Add-Type -Path "C:\path\to\your\assembly.dll"
Simply specify the path to the .dll file, and you can use its types and methods right inside your PowerShell script. This is particularly useful when dealing with large projects that have complex structures.
Working with Generic Types
Generics can provide a flexible way to work with various data types while maintaining type-checking.
Example:
$typeDefinition = @"
public class GenericList<T> {
private List<T> items = new List<T>();
public void Add(T item) { items.Add(item); }
public List<T> GetAll() { return items; }
}
"@
Add-Type -TypeDefinition $typeDefinition
$list = New-Object GenericList[string]
$list.Add("Hello")
$list.Add("PowerShell")
Here, we created a generic list class called `GenericList<T>`, allowing us to store any type in a type-safe manner. This showcases the flexibility and power of `Add-Type` with generics.
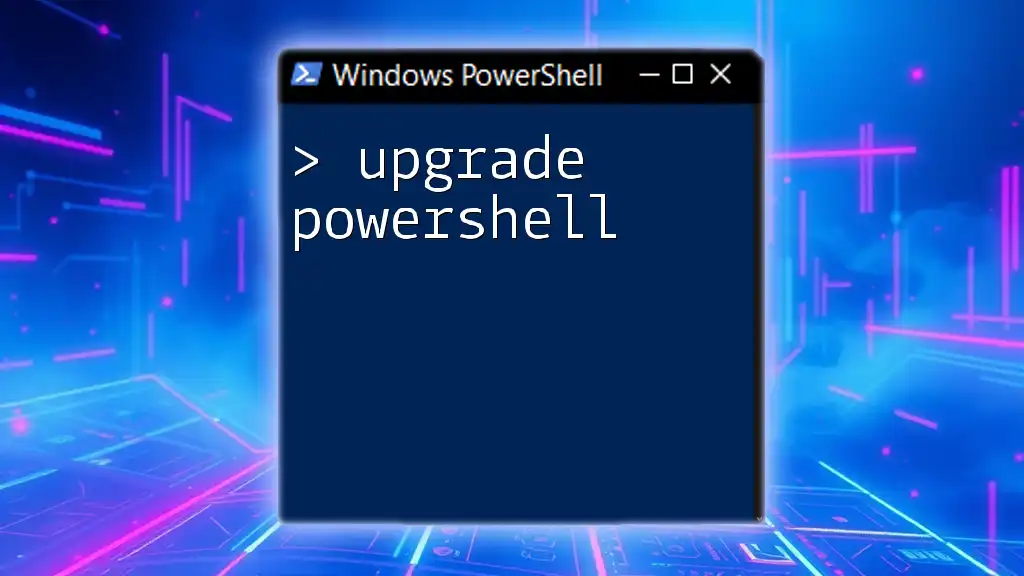
Best Practices for Using Add-Type
Coding Standards
When creating classes with `Add-Type`, adhering to coding standards is crucial. Use meaningful naming conventions and include comments to explain complex logic. This not only improves readability but also maintains your code over time.
Performance Considerations
Repeated use of `Add-Type` in your scripts can have performance implications. Consider defining your types once and reusing them where necessary. This avoids overhead and improves script execution speed.
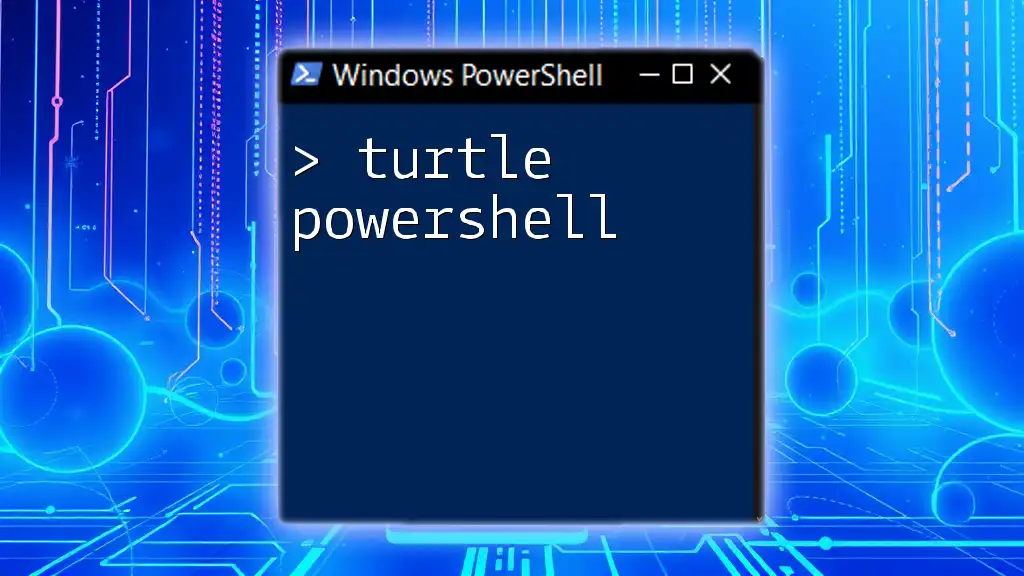
Debugging Add-Type
Common Errors and Solutions
Even as a powerful tool, `Add-Type` is prone to common errors. Here are various issues you might face and how to solve them:
- Syntax Errors: If your C# code is incorrect, you will receive a syntax error. Always ensure your type definition is valid C# code.
- Assembly Not Found: If attempting to load an assembly fails, double-check the path and permissions. Ensure you are using the correct assembly name.
To debug, consider using `Try-Catch` blocks around your `Add-Type` calls to capture and handle any exceptions gracefully.
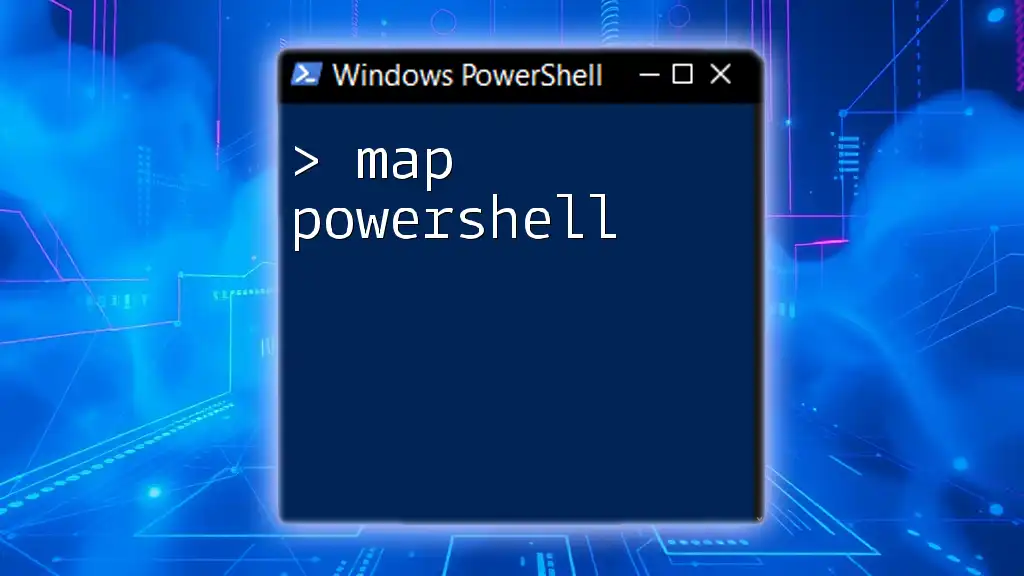
Conclusion
The `Add-Type` cmdlet in PowerShell opens up a myriad of possibilities for defining and using custom .NET types directly within your scripts. Whether you are creating custom classes, leveraging existing assemblies, or working with generics, understanding how to effectively utilize `Add-Type` is crucial for any PowerShell user.
Call to Action
Now that you have a comprehensive understanding of `Add-Type`, experiment with it in your own scripts and explore the vast opportunities it offers in enhancing your PowerShell capabilities.
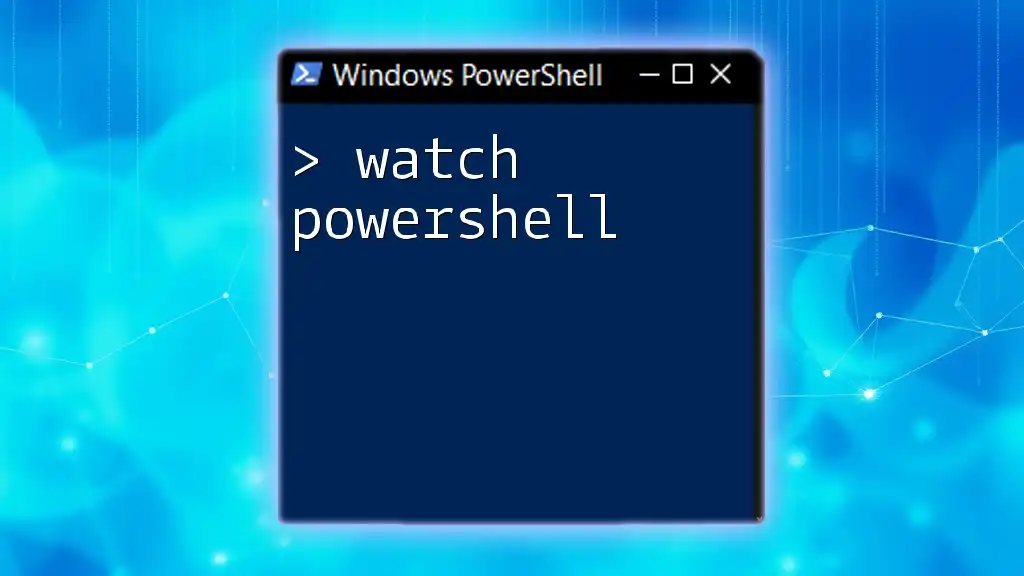
Resources for Further Reading
- PowerShell Documentation: `Add-Type`
- Official Microsoft .NET Documentation
- Online courses and communities focusing on PowerShell scripting.
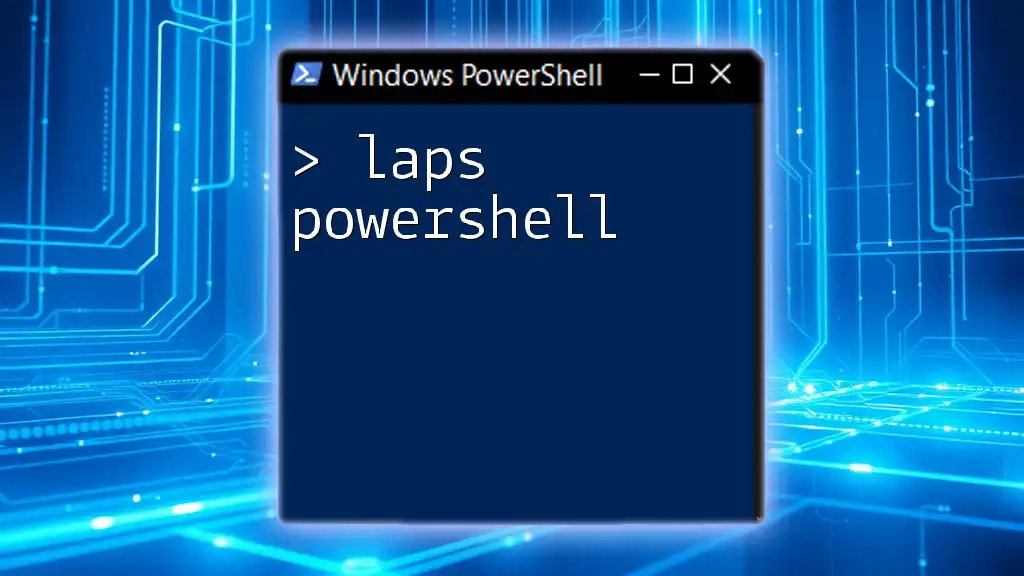
About Us
At [Your Company Name], we are dedicated to helping individuals master PowerShell through concise and effective training. Join us to enhance your scripting skills and unlock the potential of PowerShell in your daily tasks.