"Watch PowerShell" refers to the ability to monitor and observe real-time updates or changes in processes, files, or system states using PowerShell commands.
Here’s a simple code snippet to watch a file's changes:
Get-Content 'C:\path\to\your\file.txt' -Wait
This command will continuously display new lines added to the specified file as they occur.
What is PowerShell and Why Use It?
PowerShell is a task automation framework designed for system administrators. It combines a command-line shell with a scripting language to help manage and automate the Windows operating system and other applications. One of the key advantages of using PowerShell is its ability to streamline complex tasks, enabling users to manage systems in a more efficient manner.
The real-time monitoring of system states is particularly vital in modern IT environments. With the capability to observe changes continuously, administrators can act quickly on critical issues such as resource bottlenecks, service failures, or unauthorized access.
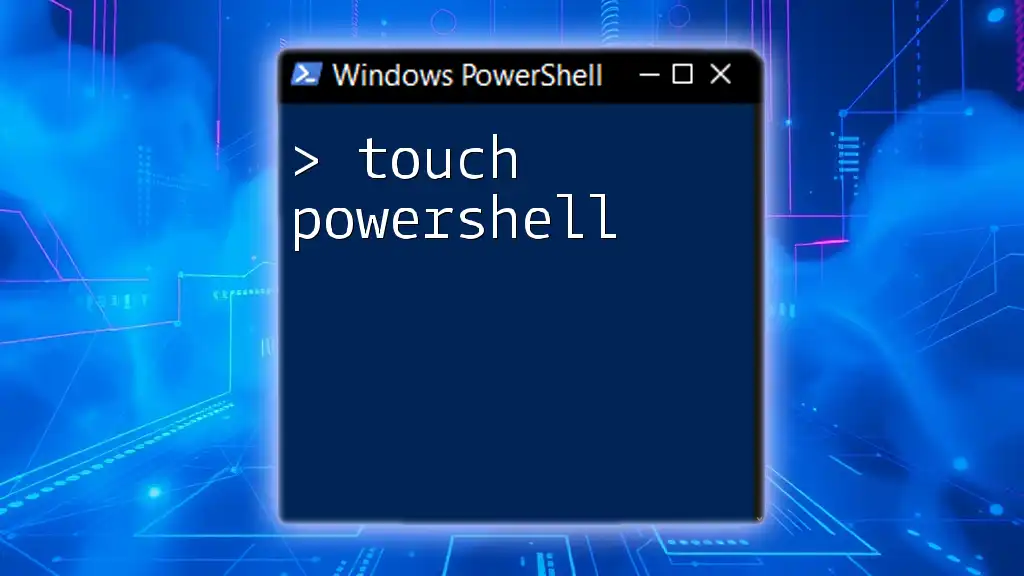
Understanding the Concept of "Watch" in PowerShell
When we talk about "watching" in PowerShell, we refer to the practice of continuously monitoring system processes, services, or files. This capability allows users to ensure optimal performance and immediate detection of issues.
Some use cases for "watching" in PowerShell include:
- Monitoring processes to identify performance-related problems.
- Keeping an eye on services to ensure they are running as expected.
- Observing file changes in specific directories to detect unauthorized modifications or errors.
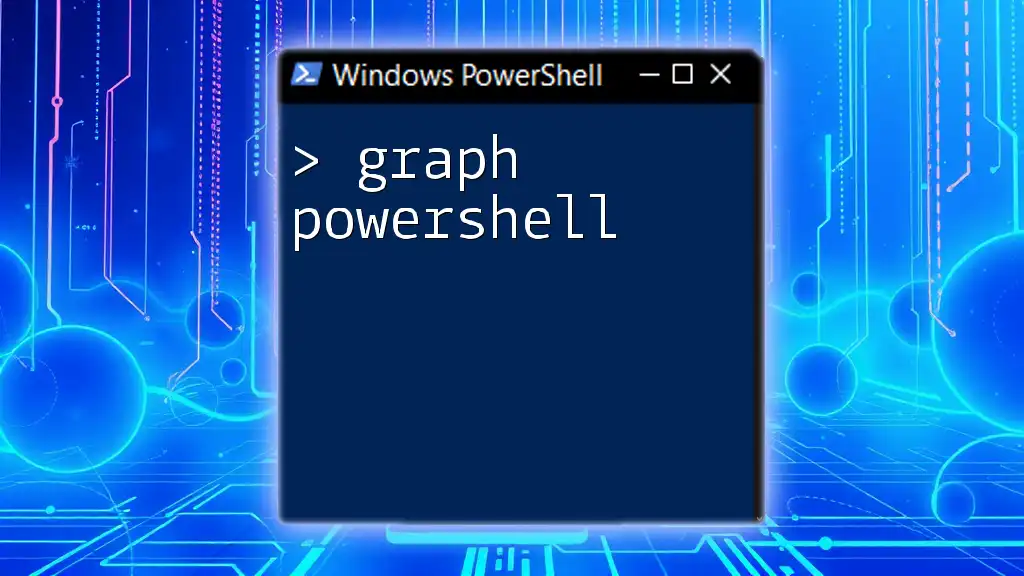
PowerShell `Watch` Equivalent
PowerShell does not have a direct equivalent to the Unix/Linux `watch` command, which repeatedly executes a command at set intervals. However, several approaches can mimic its behavior within the PowerShell environment.
PowerShell Methods for Monitoring:
Using a Loop with `Start-Sleep`
One traditional method of monitoring in PowerShell is to create a loop combined with `Start-Sleep`, which pauses the execution of a script for a specified period. This method can effectively simulate the functionality of `watch`.
Here’s a basic example to continuously display the list of running processes:
while ($true) {
Get-Process
Start-Sleep -Seconds 5
}
In this example, the script runs indefinitely, refreshing the process list every 5 seconds. While this method is straightforward, it can become resource-intensive if used excessively.
Using the `Get-Process` Cmdlet
The `Get-Process` cmdlet allows users to monitor specific processes easily. For instance, if you want to ensure that the `powershell` process is running, you can use:
Get-Process -Name 'powershell' -ErrorAction SilentlyContinue
This command checks for the `powershell` process and returns its status. The `-ErrorAction SilentlyContinue` parameter ensures that no error is shown if the process isn’t found.
Using the `Get-Service` Cmdlet
PowerShell also allows real-time monitoring of service statuses through the `Get-Service` cmdlet. Below is an example of how to use it to monitor all running services:
while ($true) {
Get-Service | Where-Object { $_.Status -eq 'Running' }
Start-Sleep -Seconds 10
}
This command checks for running services every 10 seconds. Monitoring services can be crucial for maintaining system health, as it enables you to identify and address issues before they escalate.
Using Advanced Tools for Monitoring
Introducing `WMI` (Windows Management Instrumentation)
WMI is a powerful framework that provides a standardized way to access system management information. It can be particularly useful for monitoring system performance. To check for CPU usage, you can execute:
Get-WmiObject Win32_Processor | Measure-Object -Property LoadPercentage -Average
This command queries the processor load percentage and allows administrators to gather essential data about system performance.
Leveraging `Measure-Command` for Performance Monitoring
The `Measure-Command` cmdlet captures the time taken to run a particular command, which can be useful for performance evaluations. For example:
Measure-Command { Get-Process }
This command measures the time taken to execute `Get-Process`, providing insight into how quickly the system responds to requests.
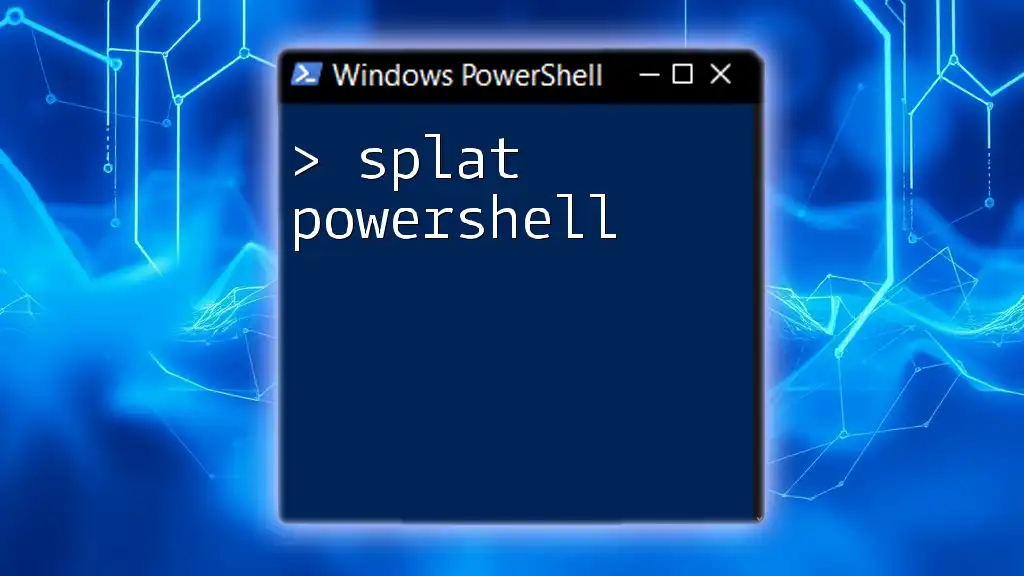
Real-Time File Monitoring in PowerShell
Using FileSystemWatcher for Directory Monitoring
The `FileSystemWatcher` class allows for real-time monitoring of file system changes. By setting it up in PowerShell, you can observe a specific directory for any activity, like modifications or deletions.
Here’s an example of how to monitor changes in a directory:
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = "C:\YourDirectoryPath"
$watcher.EnableRaisingEvents = $true
Register-ObjectEvent $watcher "Changed" -Action {
Write-Host "File $($Event.SourceEventArgs.FullPath) was changed."
}
This script will alert you in the console whenever a file is changed in the specified directory. Using `FileSystemWatcher` is efficient for responding to immediate file system changes rather than running periodic checks.
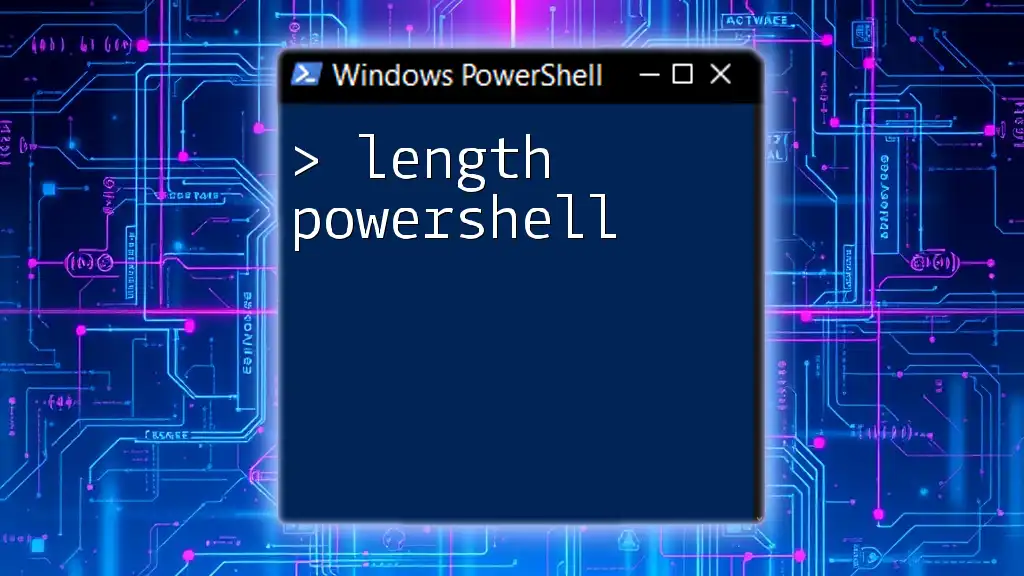
Combining and Customizing Watch Commands
Creating custom monitoring scripts in PowerShell can greatly enhance your administration capabilities. By combining multiple monitoring commands into a single script, you can keep tabs on various aspects of your environment without running multiple scripts concurrently.
For example, a script that combines several commands might look like this:
while ($true) {
Get-Process | Where-Object { $_.CPU -gt 200 }
Get-Service | Where-Object { $_.Status -eq 'Stopped' }
Start-Sleep -Seconds 10
}
This command will monitor processes consuming more than 200 CPU units while checking for any stopped services every 10 seconds. This holistic approach can help in identifying performance bottlenecks and service failures simultaneously.
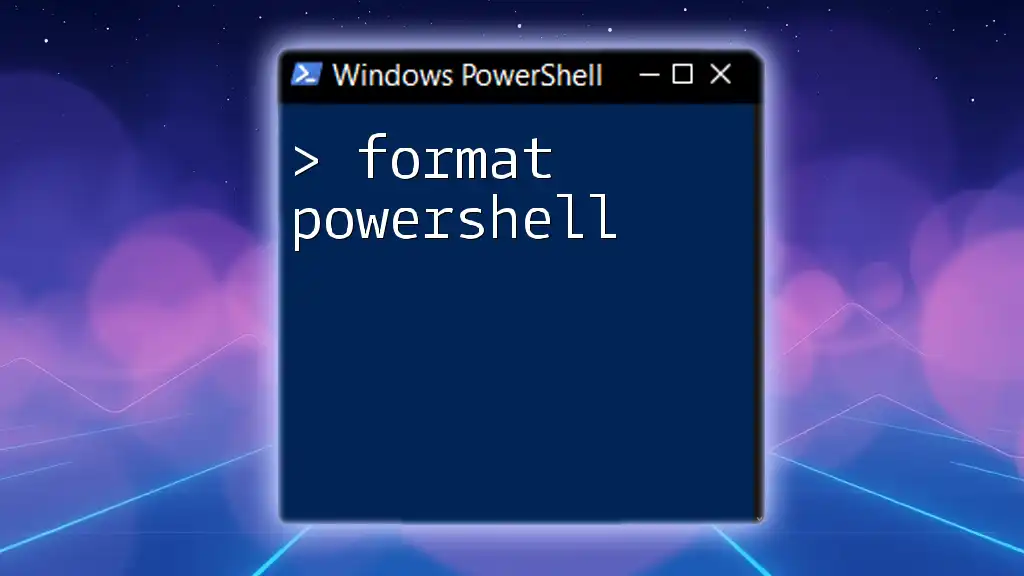
Common Pitfalls and Best Practices
Avoiding Too Frequent Queries
A critical consideration when executing monitoring scripts is avoiding overloading the system with too frequent queries. Excessive polling can consume valuable system resources. It’s essential to strike a balance between monitoring frequency and system performance.
Using Error Handling Best Practices
Incorporating robust error handling is essential for ensuring that your monitoring scripts run smoothly. Utilizing `Try/Catch` blocks can enhance your scripts’ reliability, enabling them to gracefully handle unexpected situations without crashing.
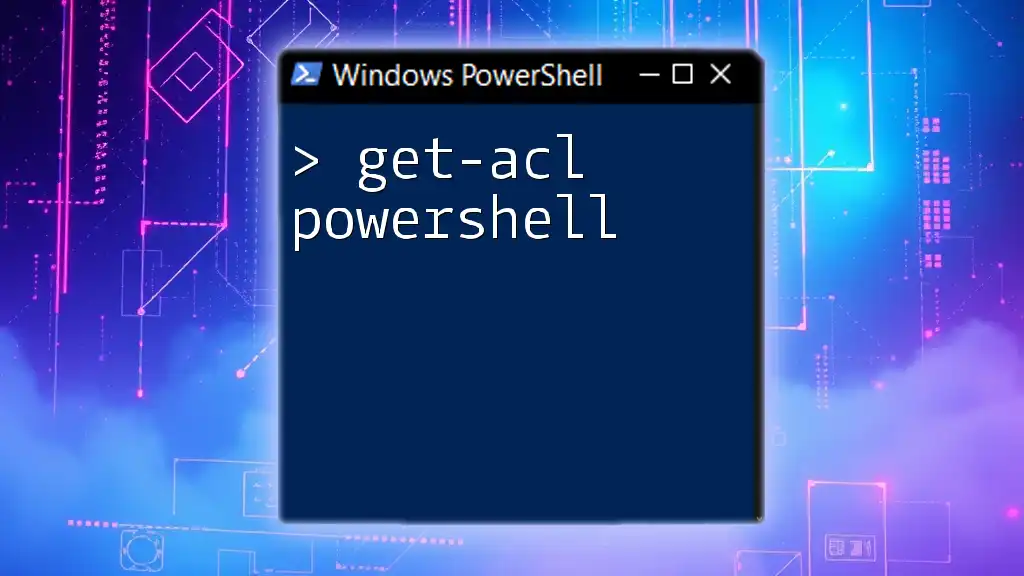
Conclusion
Real-time monitoring using PowerShell is a powerful technique for maintaining efficient system operations. With methods ranging from simple loops to advanced tools like WMI and `FileSystemWatcher`, administrators have a variety of options to keep systems running smoothly. By understanding and implementing these techniques, you can ensure that you stay one step ahead in managing your environments effectively.
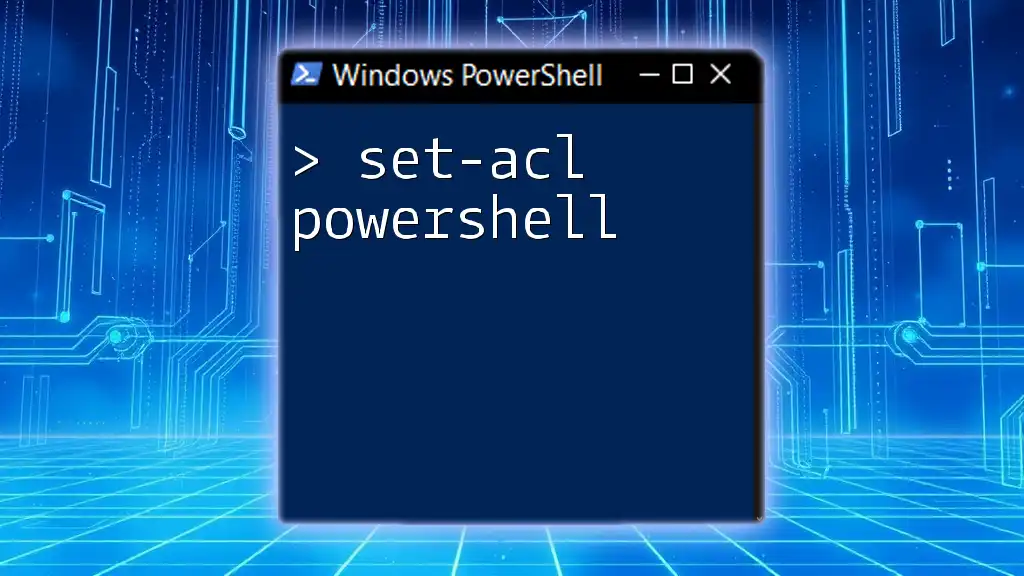
Call to Action
We invite you to share your experiences with PowerShell monitoring. What techniques have you found most effective? If you're interested in mastering PowerShell commands, check out our company’s resources and courses designed to enhance your skills in PowerShell scripting and automation.
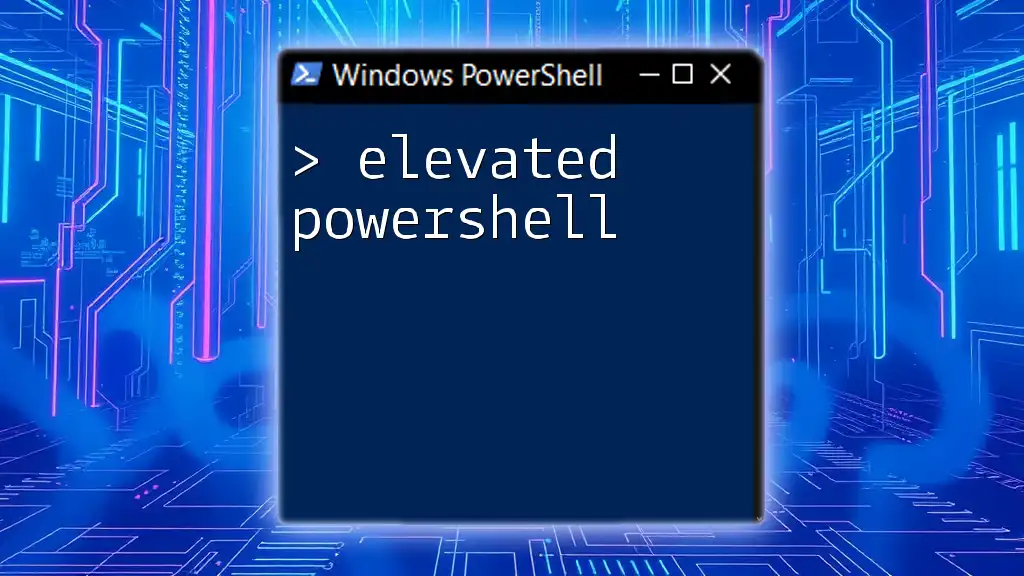
Additional Resources
For further learning, explore additional materials related to PowerShell automation, online forums, and documentation. Whether you are a beginner or advanced user, there’s always more to learn in the world of PowerShell!