Certainly! The `scp` (secure copy protocol) is not natively a PowerShell command, but you can use the `scp` functionality within PowerShell to securely transfer files between hosts over SSH.
Here's a code snippet demonstrating how to use `scp` in PowerShell:
scp C:\path\to\your\file.txt username@remotehost:/path/to/destination/
Remember to replace `C:\path\to\your\file.txt`, `username`, `remotehost`, and `/path/to/destination/` with your actual file path, username, and destination details.
Understanding SCP
What is SCP?
SCP, or Secure Copy Protocol, is a network protocol that enables secure file transfers between hosts on a network. Unlike traditional file transfer protocols, SCP provides a robust encryption mechanism for the authentication and the data being transferred, ensuring confidentiality and integrity.
When comparing SCP with other file transfer protocols such as FTP (File Transfer Protocol) or SFTP (SSH File Transfer Protocol), it's essential to note that SCP focuses primarily on simplicity and speed. It operates over SSH (Secure Shell), inheriting its security features. This makes SCP an excellent choice for transferring files securely in environments where data sensitivity is paramount.
How SCP Works
SCP operates by leveraging the SSH protocol to establish a secure connection between the source and destination hosts. Here's a basic rundown of the process:
- Authentication: Before any file transfer, SCP verifies user credentials to ensure they have the appropriate permissions.
- Encryption: All data moved through SCP is encrypted, protecting it from interception during transit. This is crucial in safeguarding sensitive information.
- Copying Data: SCP sends the data streams efficiently over the established SSH connection, making the process relatively quick, especially for large files.
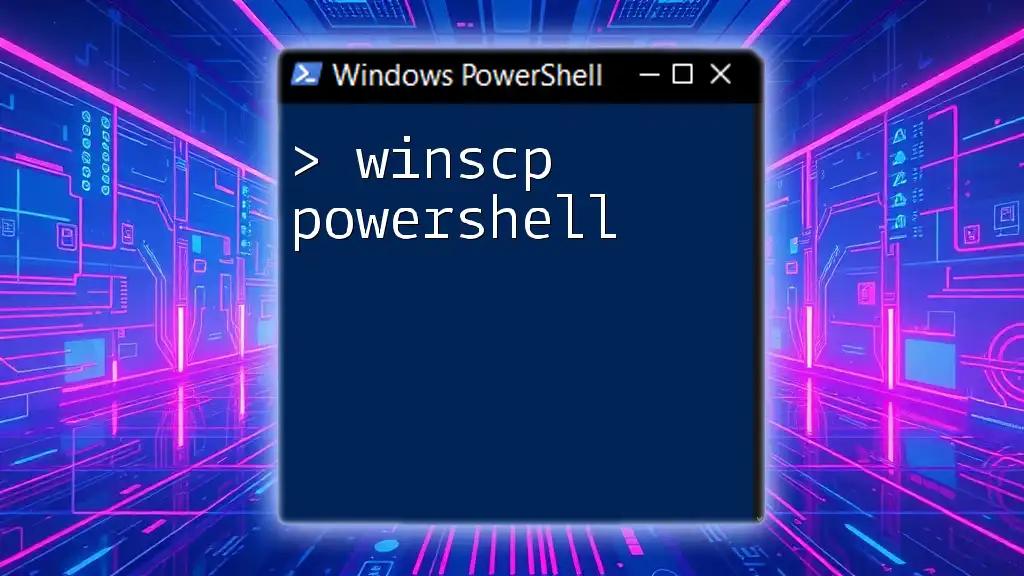
Setting Up Your Environment
Prerequisites
To begin using SCP PowerShell, ensure you have the following prerequisites:
- PowerShell: You should be working with the latest version of PowerShell on your system.
- OpenSSH: This software package must be installed on your machine, as it provides the SSH protocol support necessary for SCP.
Installing OpenSSH on Windows
The Windows 10 and Windows Server systems include OpenSSH as an optional feature. Here’s how to install it:
Using Windows Settings
- Open Settings and navigate to Apps.
- Click on Optional features.
- Select Add a feature.
- Search for OpenSSH Client, then click Install.
- Optionally, install OpenSSH Server if you want to send files to your Windows machine.
Through PowerShell Commands
You can also install OpenSSH using PowerShell with the command:
Add-WindowsCapability -Online -Name OpenSSH.Client~~~~0.0.1.0
Configuring OpenSSH
Once installed, you need to configure OpenSSH:
-
Verify that the installation is successful by opening PowerShell and running:
ssh
-
If you see a prompt with available SSH commands, the installation was successful.
-
If you installed the OpenSSH Server, you'll also need to start the service:
Start-Service sshd
-
Optionally, set it to start automatically with Windows:
Set-Service -Name sshd -StartupType 'Automatic'
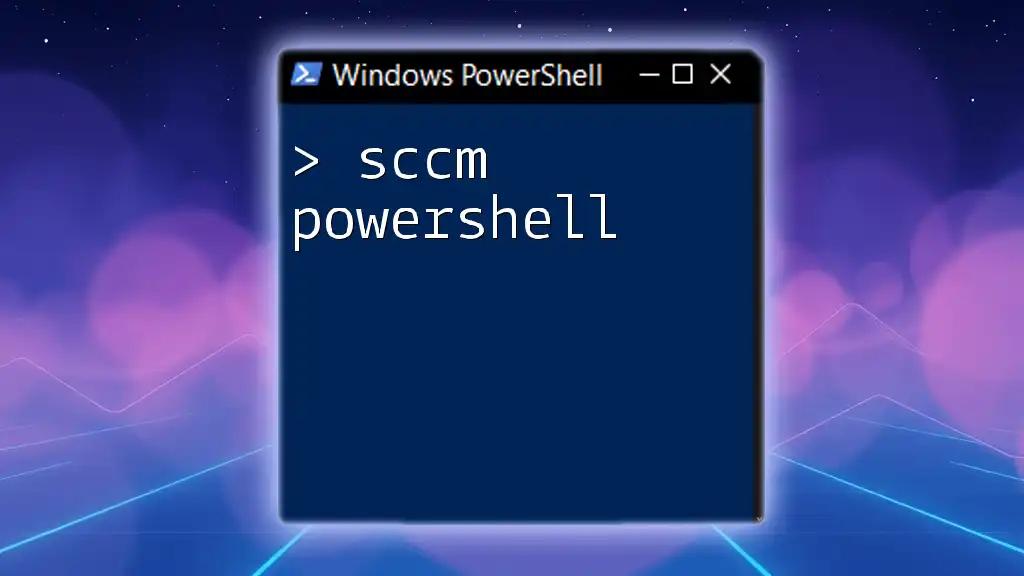
Using SCP in PowerShell
Basic Syntax of SCP Command
When using SCP PowerShell, understanding the syntax is crucial. The general format of the SCP command is:
scp [options] [source] [destination]
Here’s a breakdown:
- options: Additional flags to modify the behavior (such as `-r` for recursive copying).
- source: The file or directory you want to copy. This can be local or remote.
- destination: The destination path, which can also be local or remote.
Common SCP Commands and Examples
Copying Files from Local to Remote
To copy a file from your local machine to a remote server, use the command:
scp C:\localfile.txt user@remotehost:/remote/directory/
- In this example, `C:\localfile.txt` is the source file, and `user@remotehost:/remote/directory/` specifies the destination on the remote server.
- After entering your password, the file will be securely transferred.
Copying Files from Remote to Local
You can also transfer files from a remote server to your local machine using:
scp user@remotehost:/remote/file.txt C:\local\directory\
- Here, `user@remotehost:/remote/file.txt` indicates the file on the remote server, while `C:\local\directory\` is where it will be saved locally.
Copying Directories
To copy an entire directory recursively, the `-r` option is used:
scp -r C:\local\directory user@remotehost:/remote/directory/
- This command copies the local directory and its contents to the specified location on the remote server.

Advanced SCP Features
Using SCP with Key-Based Authentication
One advanced feature of SCP is the ability to use key-based authentication, which enhances security while simplifying authentication. Here’s how to set it up:
-
Generate SSH keys using PowerShell:
ssh-keygen
- You'll be prompted to enter a filename and passphrase. You can press Enter for default settings.
-
Once the keys are generated, copy the public key to the remote server. Use the following command while replacing `user@remotehost` with your remote server login:
ssh-copy-id user@remotehost
-
This key will now authenticate user access without requiring a password for future SCP commands.
SCP Transfer Options
SCP offers several options that can customize your transfer:
- -P: Specify a port if you're using a non-standard port (like `-P 2222`).
- -i: Use a specific private key file when authenticating.
- -v: Enables verbose mode to show more details about the transfer process.
Here’s an example using multiple options:
scp -P 2222 -i "C:\path\to\keyfile" C:\localfile.txt user@remotehost:/remote/directory/
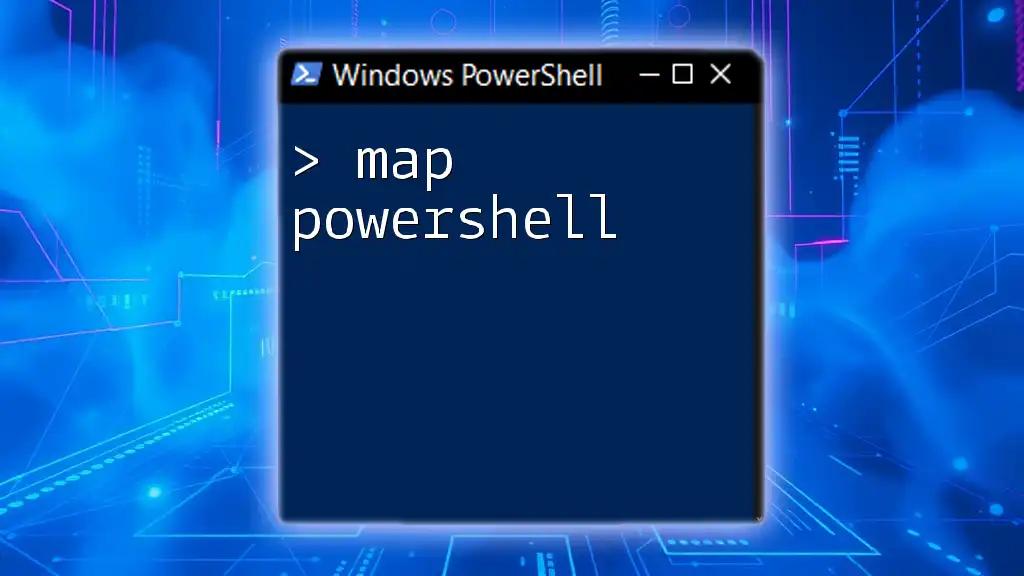
Troubleshooting Common Issues
Connecting Failures
You might encounter connection failures due to several reasons:
- Firewall Settings: Ensure that your firewall settings aren't blocking the SSH ports (usually port 22).
- Incorrect Credentials: Double-check your username and password.
- Host Verification: If it’s your first connection, you might need to verify the remote host's authenticity.
Permission Denied Errors
Receiving a permission denied error typically indicates:
- The user account does not have write permissions to the targeted directory.
- The remote server might have strict access controls. Verify your access rights by contacting the server administrator.
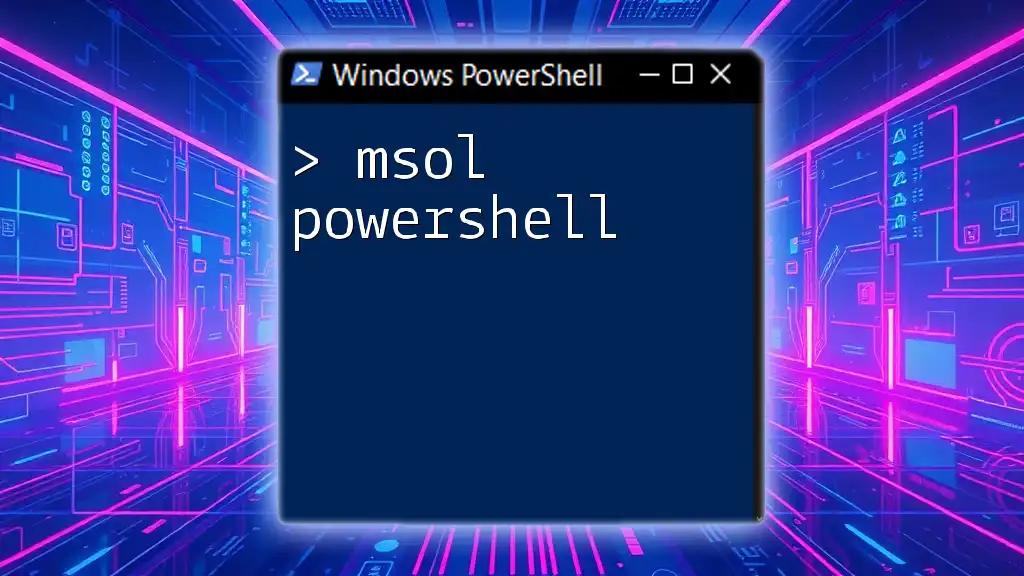
Security Considerations
When utilizing SCP for file transfers, maintaining security is paramount to protecting sensitive data. Here are some best practices:
- Always use strong passwords or key-based authentication.
- Regularly update your software, including PowerShell and the OpenSSH client, to mitigate vulnerabilities.
- Implement restrictive user permissions on both local and remote machines to minimize exposure.
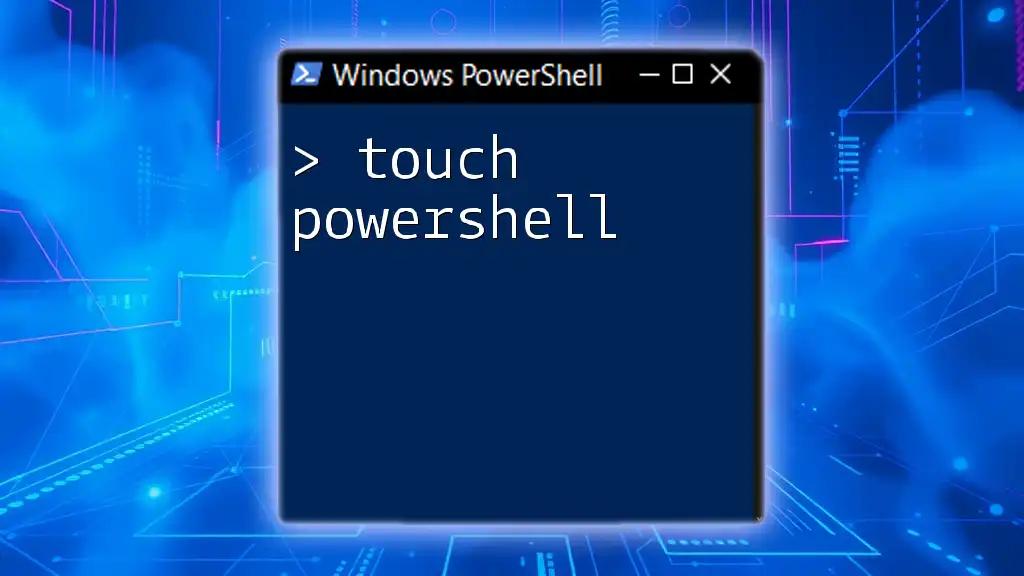
Conclusion
Using SCP PowerShell provides a powerful and secure way to transfer files over a network. By leveraging the simplicity of SCP along with the robust features of PowerShell, users can efficiently manage file transfers with confidence.
Explore and experiment with various SCP commands and options to enhance your file management skills! To stay updated with more PowerShell tips and tutorials, consider subscribing to our mailing list.
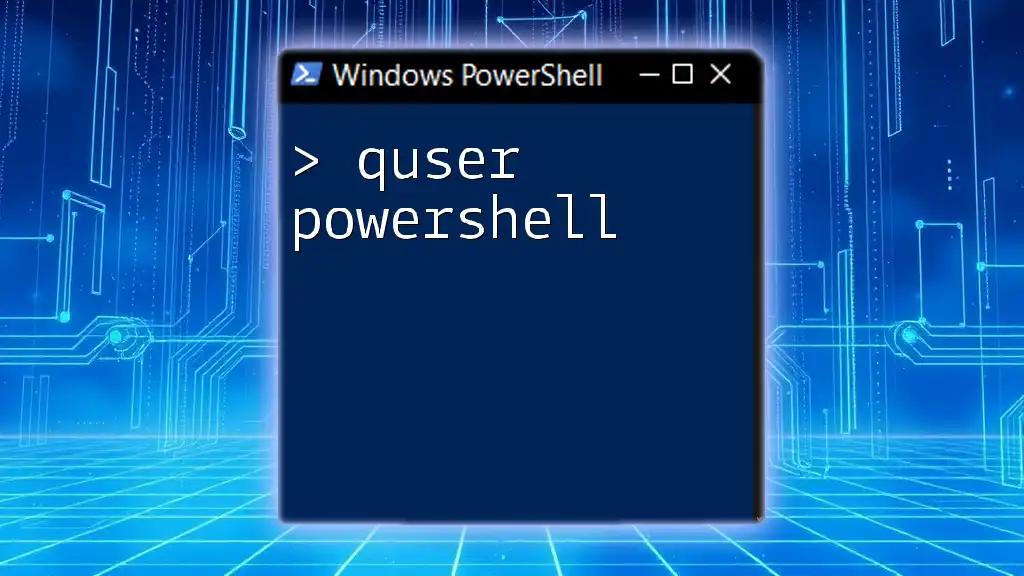
Additional Resources
For further assistance, refer to the official documentation for SCP and OpenSSH. Additionally, communities like Stack Overflow offer excellent support for any specific questions or troubleshooting you may need.