In PowerShell, a COM object allows you to interact with software components developed using the Component Object Model, enabling automation and integration with various applications, such as Microsoft Office.
Here’s a simple example of creating an Excel COM object and opening a new workbook:
$excel = New-Object -ComObject Excel.Application
$excel.Visible = $true
$workbook = $excel.Workbooks.Add()
What is a COM Object?
The Component Object Model (COM) is a Microsoft technology that allows software components to communicate and interact with one another regardless of the programming language they were built with. COM is foundational in the Windows ecosystem, providing a standardized way to handle interprocess communication and object creation.
COM objects are crucial in various scenarios, especially for automation tasks, where existing software can be controlled programmatically. Common use cases of COM objects include manipulating Office applications, interacting with browsers, and automating system functions.
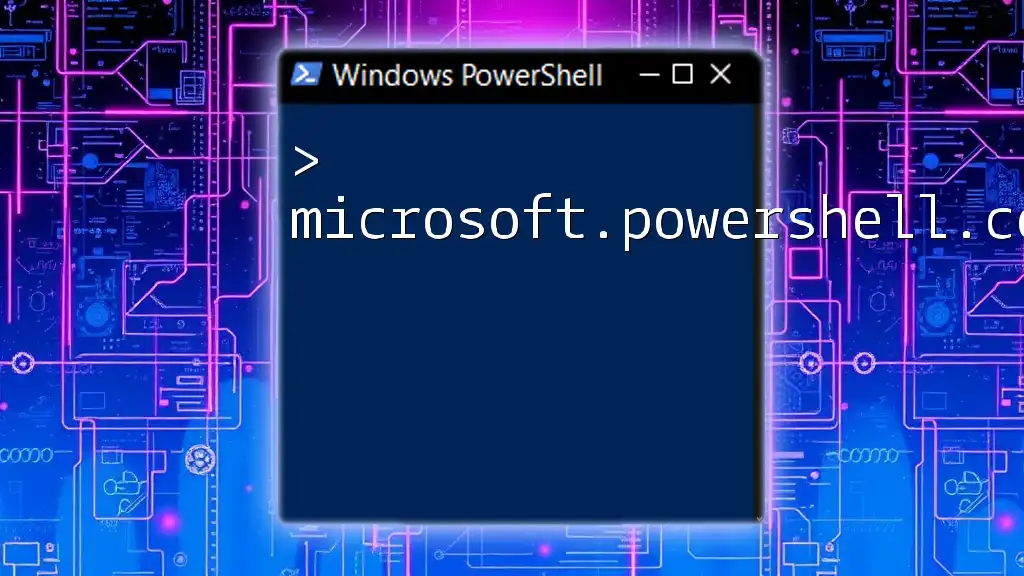
Why Use COM Objects with PowerShell?
Integrating COM objects into PowerShell scripts opens up a world of automation possibilities. Advantages include:
- Seamless integration with applications like Excel, Word, and Internet Explorer.
- The ability to leverage existing software to automate repetitive tasks.
- Enhanced scripting capabilities, allowing users to enhance their productivity through automation.
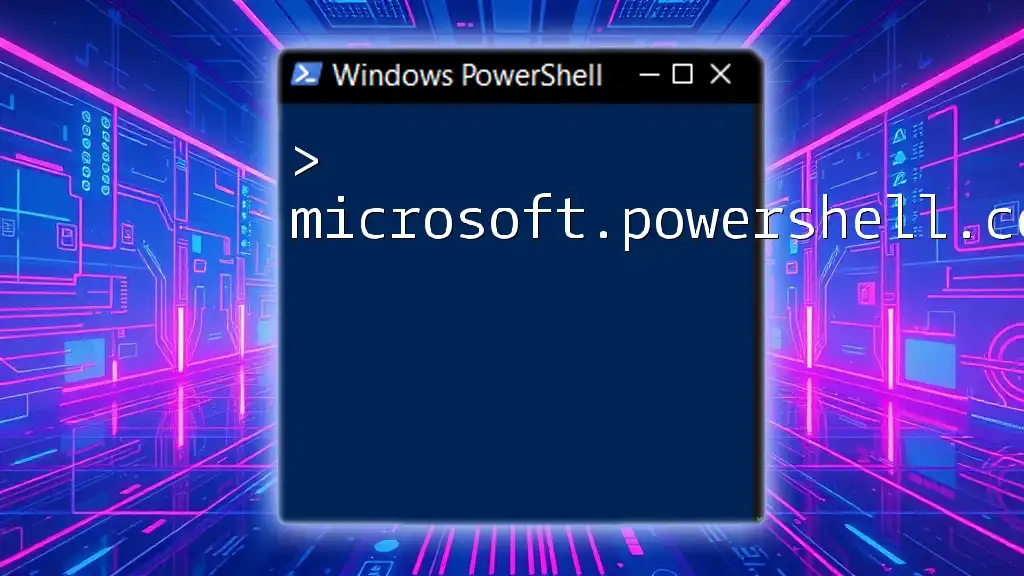
Getting Started with COM Objects in PowerShell
Setting Up Your Environment
Before diving into using COM objects, ensure that your environment is properly set up. Generally, PowerShell 5.0 or later is preferred, but you can check your version with the following command:
$PSVersionTable.PSVersion
Make sure you have Microsoft Office installed if you plan on automating office applications like Excel and Word.
Basic Syntax of Creating a COM Object
To create a COM object in PowerShell, you’ll primarily use the `New-Object` cmdlet. The syntax is straightforward:
New-Object -ComObject "ProgID"
ProgID refers to the programmatic identifier associated with the particular COM object you want to create. For example, to create an instance of Excel, you can use:
$excel = New-Object -ComObject Excel.Application
This command initializes the Excel application, allowing further manipulation through PowerShell.
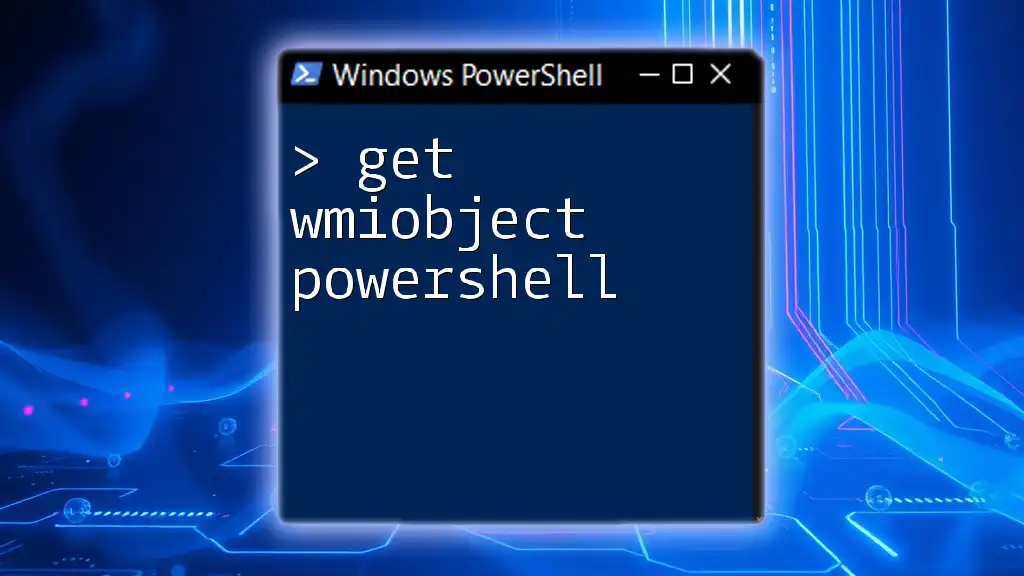
Working with Common COM Objects
Automating Excel with PowerShell
Excel is one of the most commonly automated applications using COM objects. Here’s how you can create and manipulate an Excel spreadsheet:
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Add()
$worksheet = $workbook.Worksheets.Item(1)
$worksheet.Cells.Item(1, 1) = "Hello, COM!"
$excel.Visible = $true
In this example, we’re creating a new Excel application, adding a workbook, inserting a greeting in the first cell, and finally making Excel visible to the user. This highlights the ease with which you can automate office tasks.
Interacting with Word Documents
PowerShell can also be used to automate Word. Here’s how to create and edit a document:
$word = New-Object -ComObject Word.Application
$doc = $word.Documents.Add()
$doc.Content.Text = "Welcome to PowerShell with COM Objects!"
$word.Visible = $true
This snippet initializes Word, creates a new document, adds a text, and makes Word visible. Whether generating reports or creating templates, using PowerShell with Word can significantly boost productivity.
Controlling Internet Explorer
PowerShell can automate Internet Explorer, allowing actions like web browsing or data extraction. Here’s an example of navigating to a webpage:
$ie = New-Object -ComObject InternetExplorer.Application
$ie.Visible = $true
$ie.Navigate("http://www.example.com")
By creating an instance of the InternetExplorer COM object, you can interact with web pages programmatically—a useful feature for scraping data or testing web applications.
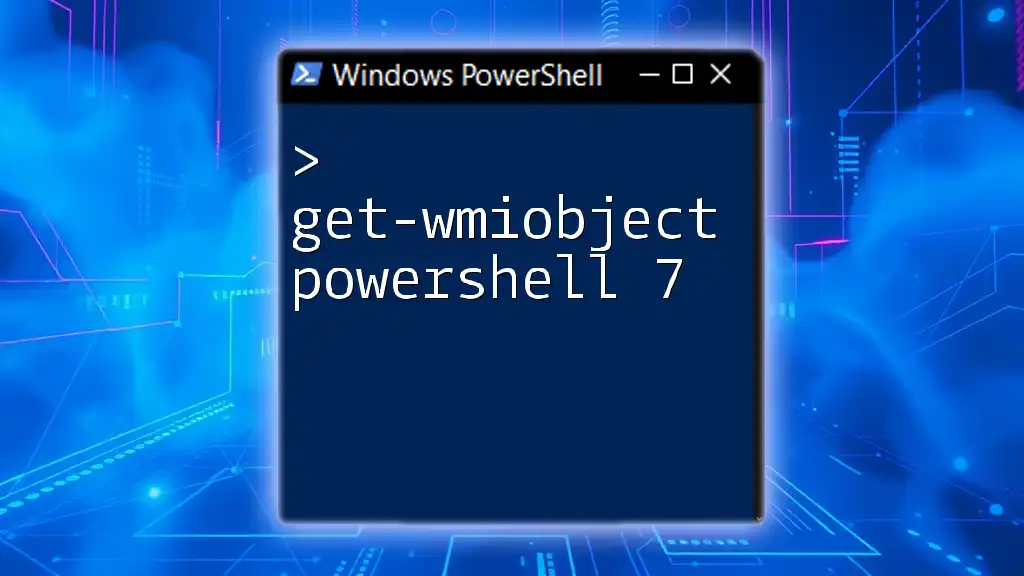
Advanced COM Object Operations
Working with COM Properties and Methods
Once you've created a COM object, accessing its properties and methods is essential for thorough manipulation. You can both get and set properties as needed.
$excel.Visible = $true # Set property
$version = $excel.Version # Get property
In this example, we set Excel to be visible and retrieve its version. Understanding how to interact with properties and methods unlocks the full potential of COM objects in PowerShell.
Handling COM Errors
Working with COM objects can sometimes lead to errors. Handling these gracefully ensures reliability in your scripts. To manage errors effectively, use `Try-Catch` blocks:
try {
$excel = New-Object -ComObject Excel.Application
} catch {
Write-Host "Error: $_"
}
In this scenario, if an error occurs while creating the Excel object, your script will catch and display the error message instead of crashing.
Cleaning Up COM Objects
Failure to release COM objects can lead to memory leaks and stability issues. After you’re done using a COM object, ensure it’s properly disposed of:
$excel.Quit()
[System.Runtime.Interopservices.Marshal]::ReleaseComObject($excel)
This practice of cleaning up COM objects is crucial for maintaining optimal performance and resource management in your PowerShell scripts.
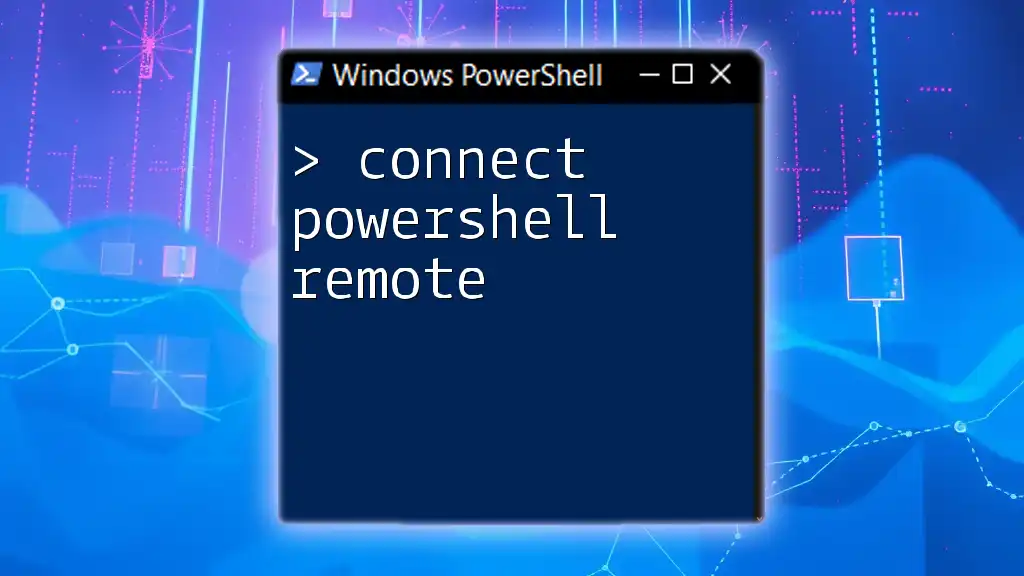
Best Practices for Using COM Objects
Optimization Techniques
Performance can vary significantly when using COM objects. To optimize your scripts, consider the following:
- Minimize object creation: Reuse existing COM objects when possible instead of creating new ones unnecessarily.
- Batch operations: If you are performing multiple actions, try to batch them together to reduce the overhead of frequent calls.
Security Considerations
Using COM objects can introduce security risks, particularly with automation tasks involving user input. Always validate input and restrict the execution context to trusted sources.
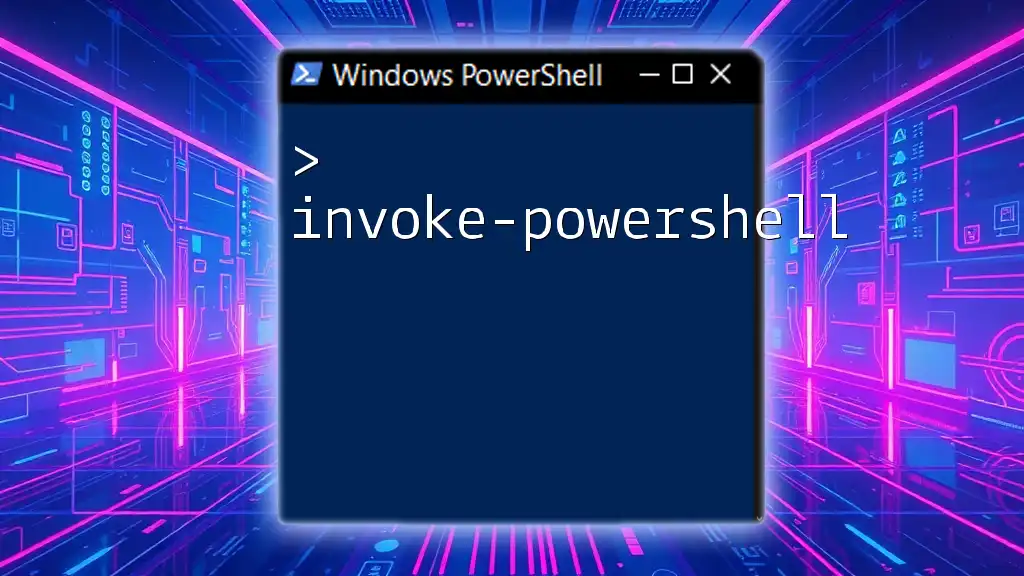
Conclusion
In conclusion, leveraging COM objects in PowerShell opens up vast automation capabilities, especially when working with commonly used applications like Excel and Word. Understanding the nuances of creation, manipulation, and cleanup ensures that your scripts run efficiently and effectively.
Further Resources
To deepen your knowledge about COM objects and PowerShell, consider exploring Microsoft's official documentation or engaging with community forums. These platforms often provide valuable insights and real-world applications that can enhance your skills further.
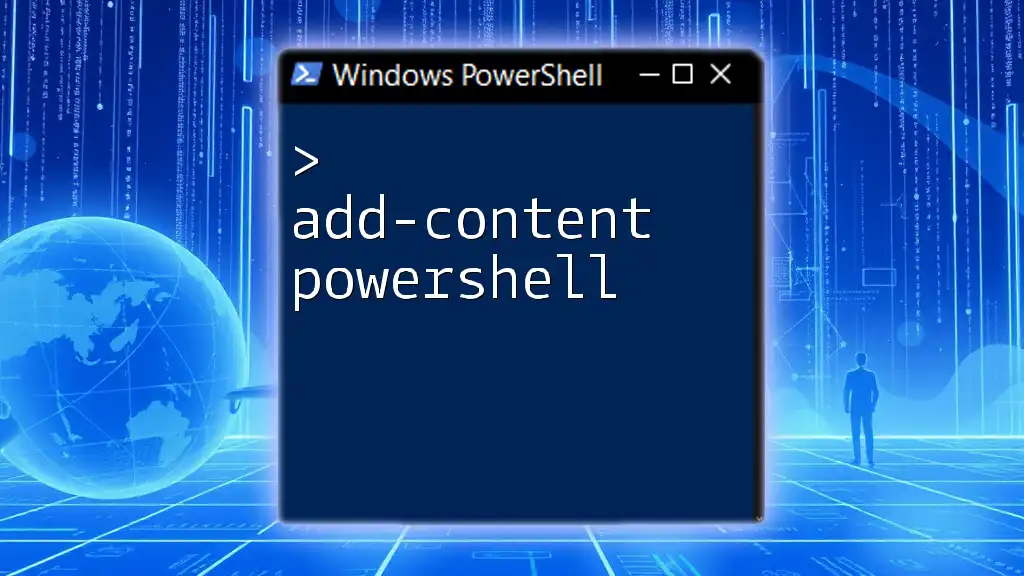
Call to Action
Now that you’re equipped with a comprehensive understanding of using COM objects in PowerShell, it’s time to put your knowledge into practice! Try creating your own scripts to automate tasks and share your experiences or questions in the comments. Happy scripting!