The `Get-WmiObject` cmdlet in PowerShell 7 allows you to retrieve management information from local and remote computers, serving as a powerful tool to access system and network data.
Here's a code snippet to demonstrate its usage:
Get-WmiObject -Class Win32_OperatingSystem
Understanding WMI
What is WMI?
Windows Management Instrumentation (WMI) is a core Windows component that provides a standardized method for accessing and managing hardware and software components. It acts as a gateway, enabling scripts and applications to query and manipulate the operating system’s settings, environment, and components. WMI consists of various elements, such as classes, instances, and namespaces, which together facilitate efficient system management.
Why Use Get-WMIObject?
The `Get-WMIObject` cmdlet in PowerShell is a powerful tool for retrieving management information from local or remote computers. It allows users to access a wealth of data on system components and configurations, making it essential for systems administration and automation tasks. The primary advantages of using `Get-WMIObject` include its ability to pull detailed system information without needing complex programming and its compatibility with a wide range of Windows versions.
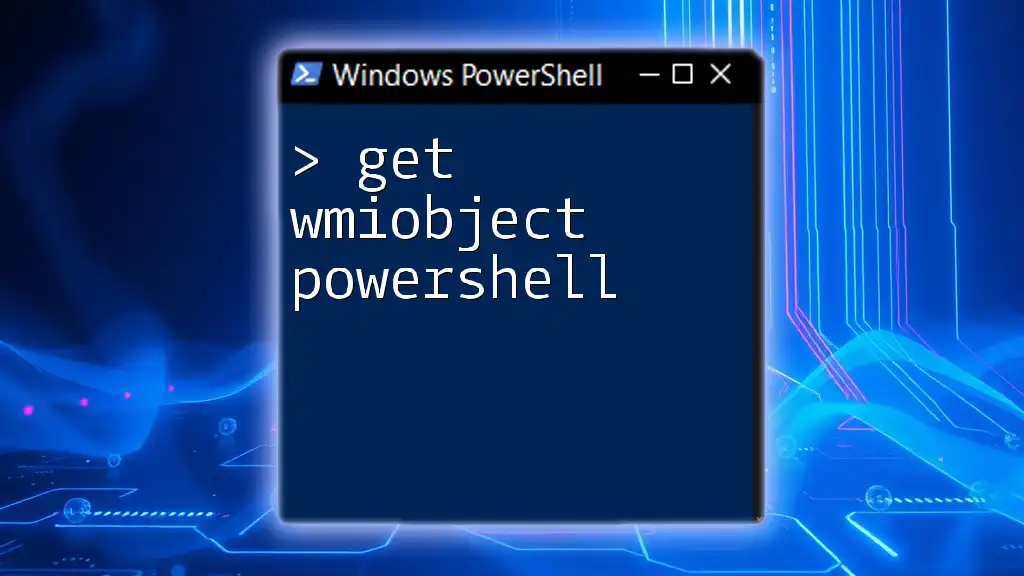
Get-WMIObject in PowerShell 7
Overview of Get-WMIObject
In PowerShell 7, `Get-WMIObject` continues to serve as an integral cmdlet for WMI queries. However, it’s important to note that its use is becoming less common in favor of `Get-CimInstance`, which is more efficient and modern. While both cmdlets perform similar functions, `Get-CimInstance` is designed to utilize WS-Man (Windows Remote Management), making it more suitable for remote queries. Nevertheless, understanding how to use `Get-WMIObject` is vital for managing legacy systems.
Syntax
The basic syntax of `Get-WMIObject` is straightforward:
Get-WMIObject -Class <ClassName> -Namespace <Namespace>
Here, `<ClassName>` refers to the WMI class you want to query, and `<Namespace>` specifies the WMI namespace from which you want to retrieve the information. Example classes include `Win32_OperatingSystem`, `Win32_Processor`, and many more.
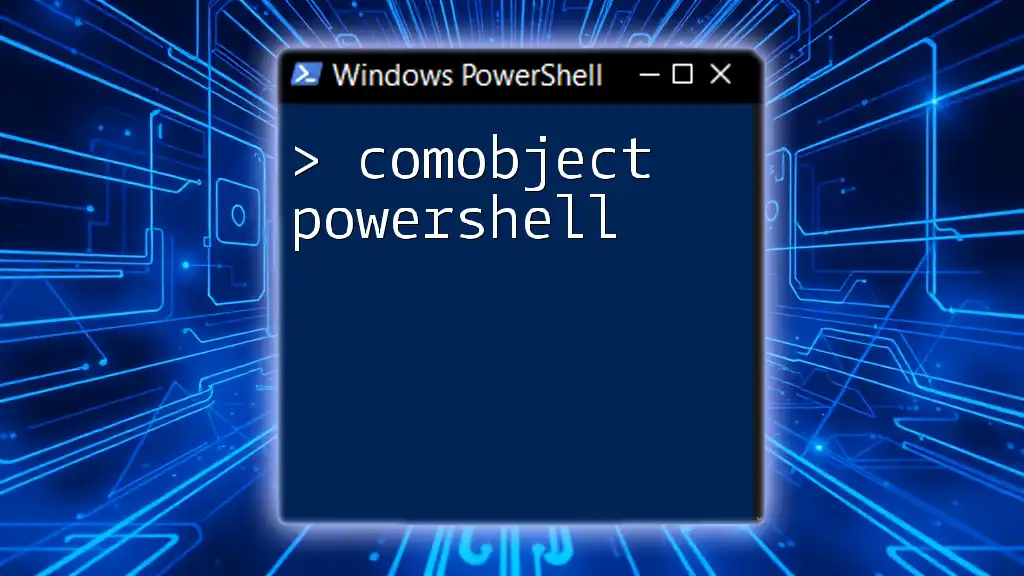
Essential Parameters
Commonly Used Parameters
Understanding the parameters of `Get-WMIObject` is crucial for crafting effective queries.
-Class
The `-Class` parameter specifies the WMI class from which you want to retrieve information. For example, to retrieve operating system details, you can use:
Get-WMIObject -Class Win32_OperatingSystem
-Namespace
WMI classes reside within namespaces. The default namespace is typically `Root\CIMv2`, but we can specify others if needed. If you want to query network adapter information, you might pull it from a different namespace:
Get-WMIObject -Class Win32_NetworkAdapter -Namespace Root\CIMv2
-ComputerName
This parameter allows you to run WMI queries on remote systems. For instance, if you wanted to retrieve process information from a machine named “RemotePC,” you could use:
Get-WMIObject -Class Win32_Process -ComputerName RemotePC
-Filter
Filters help refine your queries, returning only relevant data. If you want to find specific processes, you can filter by name:
Get-WMIObject -Class Win32_Process -Filter "Name='notepad.exe'"
-Credential
For interacting with remote systems, the `-Credential` parameter is essential. It allows you to authenticate using a username and password when querying:
$cred = Get-Credential
Get-WMIObject -Class Win32_ComputerSystem -ComputerName RemotePC -Credential $cred
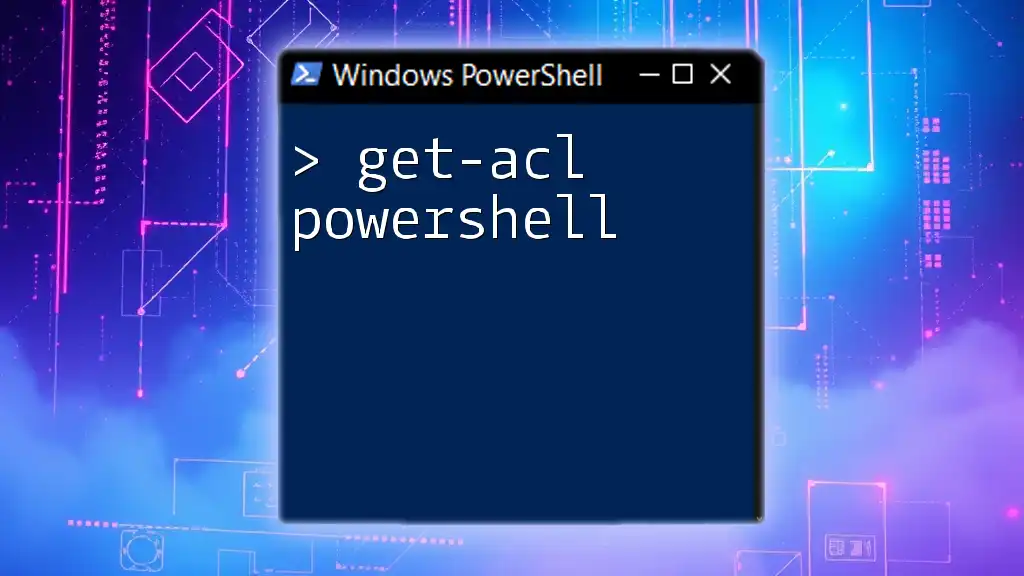
Practical Examples
Retrieving System Information
To get crucial information about the operating system, you can execute:
Get-WMIObject -Class Win32_OperatingSystem
This command provides details such as the OS version, manufacturer, and service pack level.
Accessing Hardware Information
You can use `Get-WMIObject` to retrieve details about the CPU and physical memory. Here are some examples:
Get-WMIObject -Class Win32_Processor
This command provides CPU details such as the number of cores, clock speed, and more.
For memory details, use:
Get-WMIObject -Class Win32_PhysicalMemory
Managing Services
Monitor running Windows services with the following command:
Get-WMIObject -Class Win32_Service | Where-Object { $_.State -eq "Running" }
This command lists all services currently running on the system.
Using Filters Effectively
Creating complex WMI queries can drastically improve performance and relevance. For instance, to find all instances of `notepad.exe`, you can leverage filtering:
Get-WMIObject -Class Win32_Process -Filter "Name='notepad.exe'"
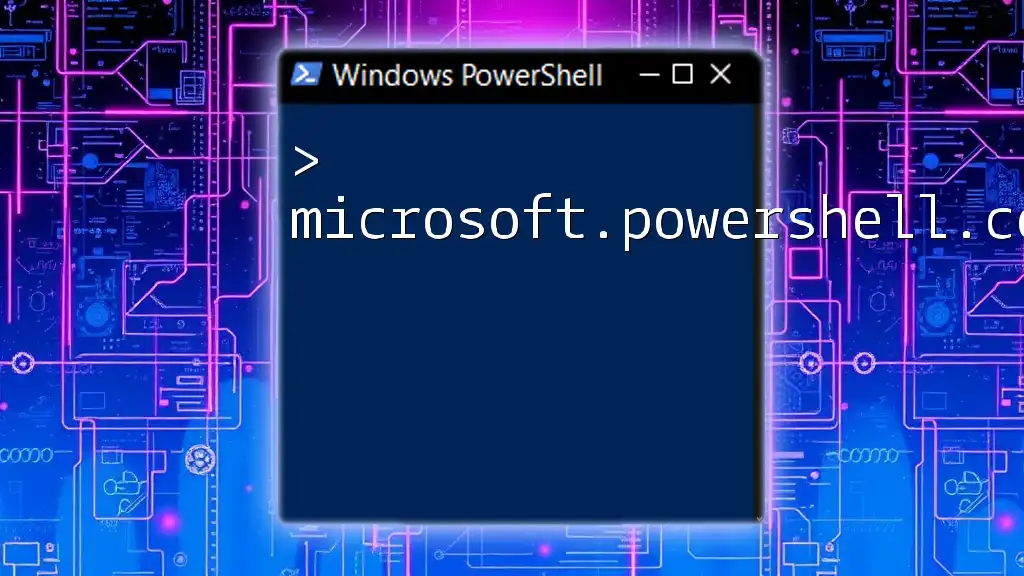
Best Practices
Performance Considerations
While `Get-WMIObject` works well, consider using `Get-CimInstance` when dealing with remote machines for improved performance. CimInstance uses a more efficient protocol and is the recommended option for newer scripts.
Error Handling
Including error-handling mechanisms in your scripts helps manage unexpected failures gracefully. Utilizing try-catch blocks, like below, improves robustness:
try {
Get-WMIObject -Class Win32_LogicalDisk -ErrorAction Stop
} catch {
Write-Host "Error: $_"
}
Security Considerations
Ensure that permissions are properly set when accessing WMI data. Using the right credentials and maintaining secure practices is essential, especially when interacting with remote systems.
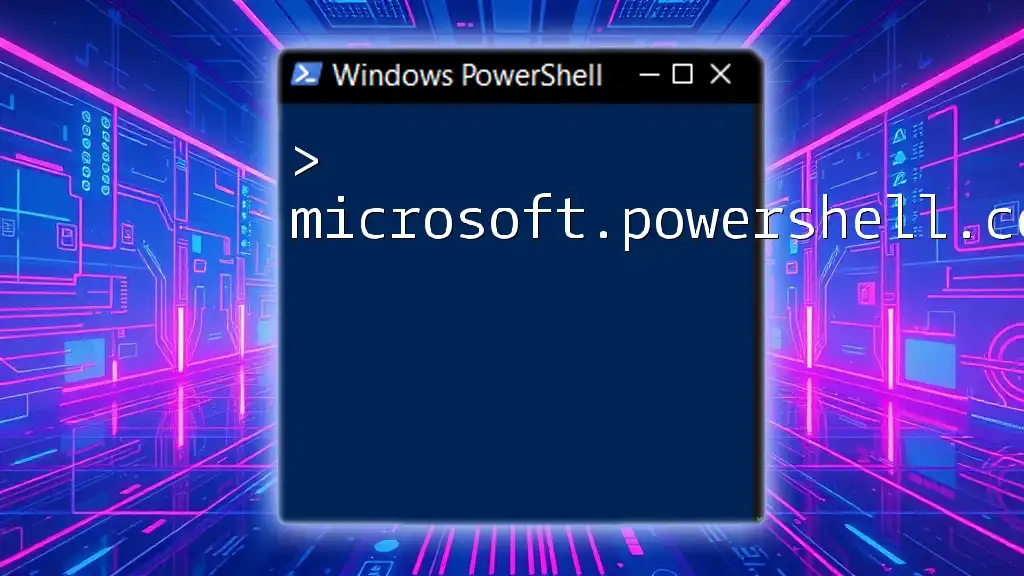
Common Issues and Troubleshooting
Connectivity Issues
Common problems include remote access being blocked, firewall configurations, or permission issues. Ensuring that the target machine allows WMI queries is vital.
Query Failures
When queries fail, common culprits include incorrect class names, namespaces, or filter syntax. Always verify that you are using the correct parameters and values. Debugging with simple queries can help isolate the issue.
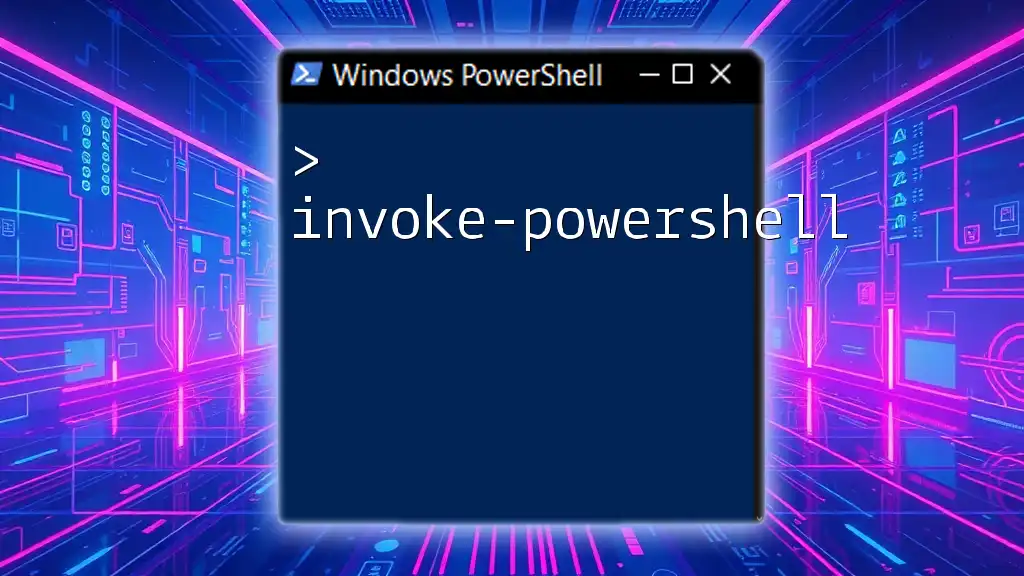
Conclusion
In conclusion, the `Get-WMIObject` cmdlet remains a powerful tool in PowerShell 7 for querying system and hardware information. Though it has evolved alongside PowerShell, mastering this cmdlet is essential for effective systems management, especially in environments with legacy systems. Embrace practical exploration and implementation to take full advantage of PowerShell's capabilities.
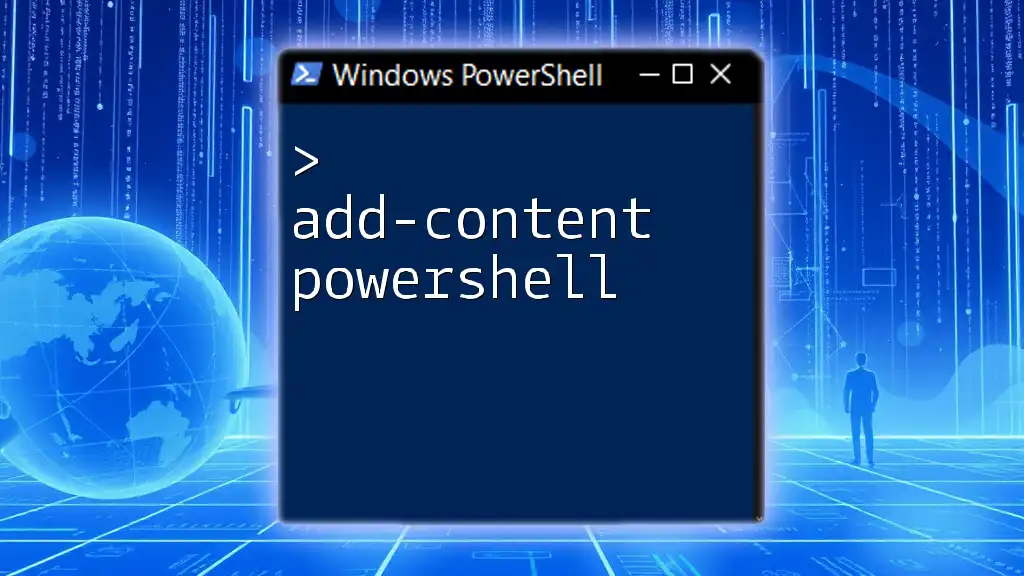
Additional Resources
For deeper learning, explore the official Microsoft documentation on [WMI](https://docs.microsoft.com/en-us/windows/win32/wmisdk/wmi-start-page) and [PowerShell](https://docs.microsoft.com/en-us/powershell/scripting/learn/overview?view=powershell-7.1). Consider following various PowerShell blogs and forums for ongoing knowledge and community support.