The `Set-Acl` cmdlet in PowerShell is used to modify the access control list (ACL) of items such as files or folders, enabling you to set security permissions for users and groups.
Here’s a code snippet demonstrating how to use `Set-Acl` to set permissions on a file:
$acl = Get-Acl "C:\example\file.txt"
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("UserName","FullControl","Allow")
$acl.SetAccessRule($rule)
Set-Acl "C:\example\file.txt" $acl
Understanding ACLs in PowerShell
What is an ACL?
An Access Control List (ACL) is a list of permissions attached to an object in Windows, such as files or folders. These permissions determine who can access, modify, or execute the object. Each entry in an ACL is known as an Access Control Entry (ACE) and defines the specific rights (like Read, Write, or Full Control) that are granted or denied to a user or group.
Why Use PowerShell for Managing ACLs?
PowerShell offers a powerful command line interface that enhances automation and efficiency in managing ACLs. By using `Set-Acl`, you can easily apply changes across multiple objects, significantly reducing the time and effort required for manual updates through the GUI.
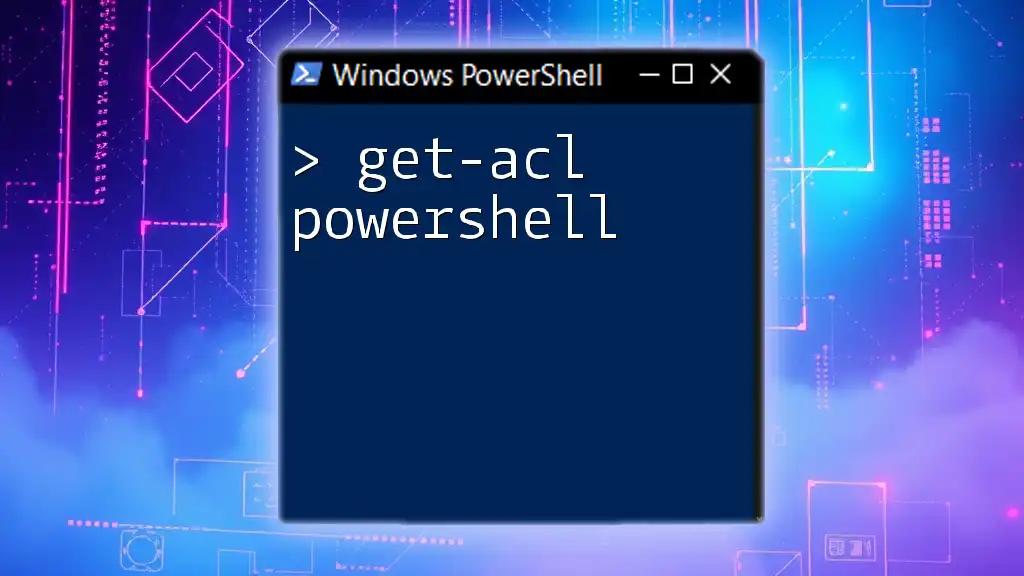
Introduction to the Set-Acl Cmdlet
Overview of Set-Acl
The `Set-Acl` cmdlet is a crucial part of PowerShell, enabling you to change the ACL of a specified file or directory. The basic syntax of the cmdlet is as follows:
Set-Acl -Path <String> -AclObject <Object>
This command allows you to apply specific ACLs that control access to your files and directories.
Key Parameters of Set-Acl
Path
The `-Path` parameter specifies the target file or directory for which you want to set permissions. For example, to set ACLs on a folder called "ExampleFolder," you would use:
Set-Acl -Path "C:\ExampleFolder"
AclObject
The `-AclObject` parameter refers to the ACL object that you want to apply. To obtain this object, you typically retrieve the existing ACL using the `Get-Acl` command.
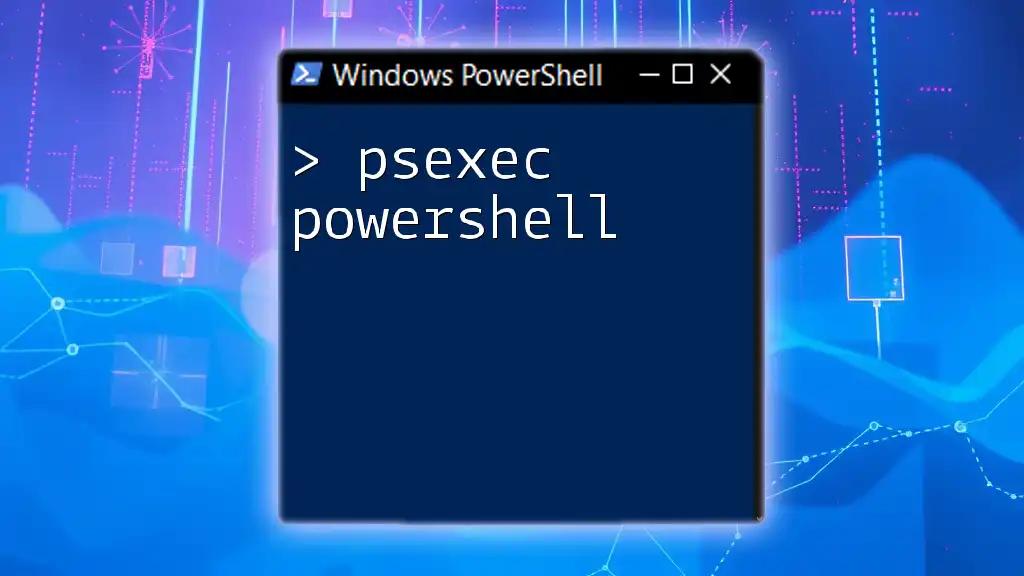
Step-by-Step Guide to Using Set-Acl
Step 1: Retrieve Current ACLs
Using Get-Acl
Before making any changes, it's vital to review the current ACL for your object. You can do this using the `Get-Acl` cmdlet:
$acl = Get-Acl -Path "C:\ExampleFolder"
$acl | Format-List
This command retrieves and displays the existing access settings in a structured format, making it easy to analyze.
Step 2: Modify ACLs
Creating a New ACL Rule
To modify an ACL, you need to create a new access rule. This is done using the `System.Security.AccessControl.FileSystemAccessRule` object. For example, to grant a user named "User" modify permissions, you would use:
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("DOMAIN\User", "Modify", "Allow")
Applying the New Rule to an ACL Object
Once you've created the new rule, you must add it to your ACL object:
$acl.SetAccessRule($rule)
This command appends the specified rule to the current list of permissions.
Step 3: Set the Modified ACL
Finally, apply the modified ACL back to the target file or directory using `Set-Acl`:
Set-Acl -Path "C:\ExampleFolder" -AclObject $acl
This command commits your changes, ensuring the specified permissions are now in effect.
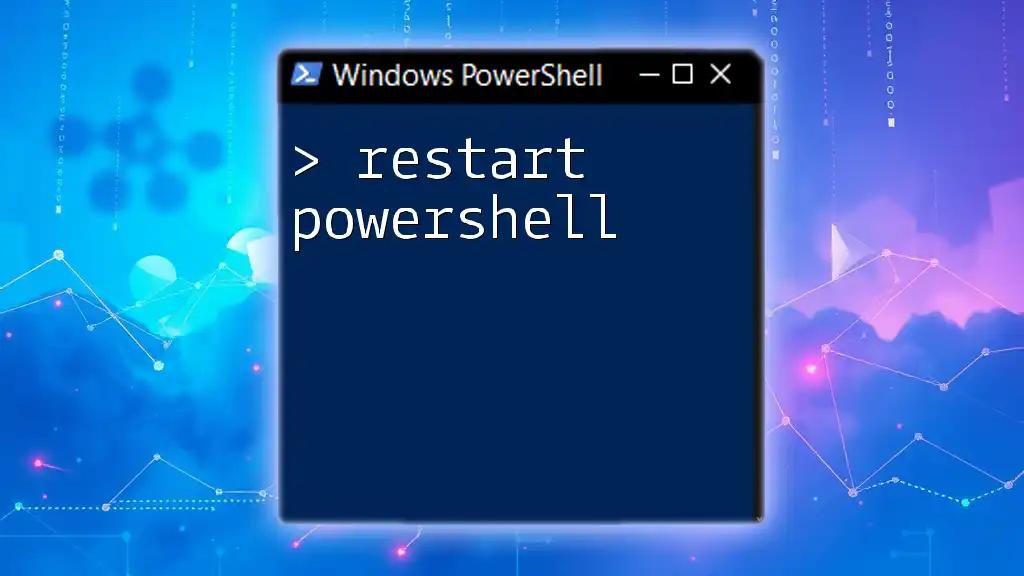
Common Scenarios and Use Cases
Granting Read Access to a User
To grant read access to a user, you can create a rule similar to the following:
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("DOMAIN\User", "Read", "Allow")
This command allows the specified user to view files in the folder.
Denying Access to a User
If you need to deny permissions, you can use:
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("DOMAIN\User", "FullControl", "Deny")
This rule removes all permissions for the specified user, adding an extra layer of security.
Removing an Existing Access Rule
To remove a rule that is no longer applicable, the `RemoveAccessRule` method comes into play:
$acl.RemoveAccessRule($rule)
This will delete the rule from the ACL, reflecting the updated permissions.
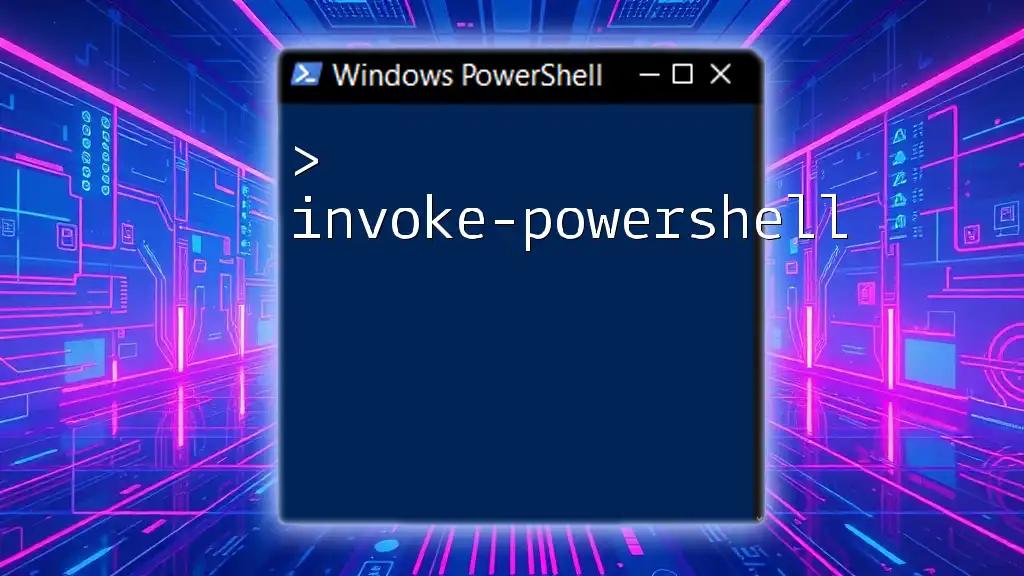
Best Practices for Using Set-Acl
Testing Before Committing Changes
Before applying changes to your ACLs, it’s a best practice to preview what the modifications will look like. You can create a snapshot of existing permissions by utilizing `Get-Acl`. This allows you to ensure you're making the right adjustments.
Backup and Restore ACLs
Creating a backup of your current ACLs before any modifications is essential for disaster recovery. You can export the ACL to an XML file like this:
$acl | Export-Clixml -Path "C:\Backup\ExampleFolderACL.xml"
This action provides you with a restore point should anything go wrong during the modification process.
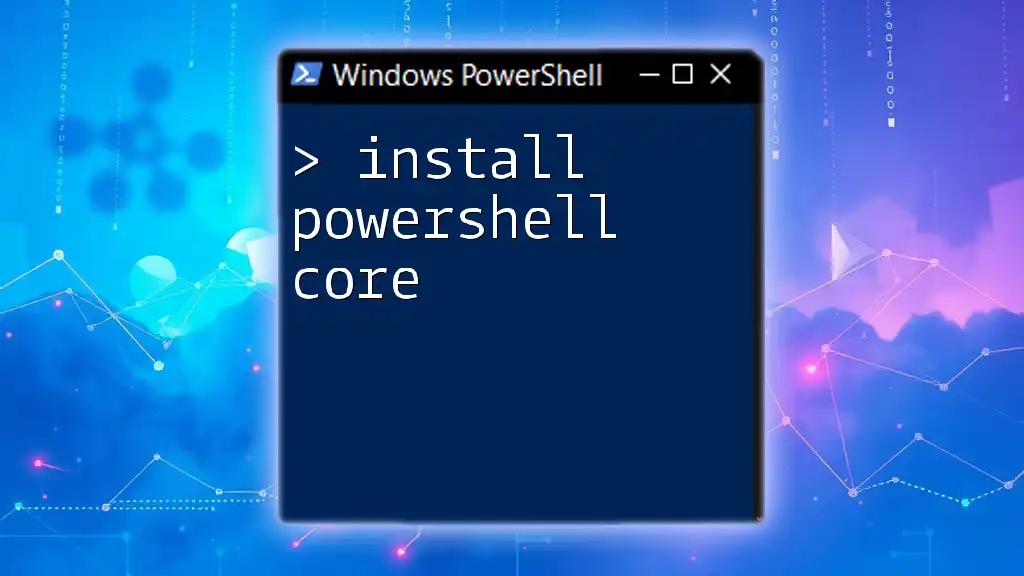
Troubleshooting Common Issues
Access Denied Errors
Encountering "Access Denied" errors is not uncommon when attempting to change ACLs. This is typically due to insufficient rights. You may need to run PowerShell as an Administrator or modify the permission settings on the parent directories.
Understanding Inheritance
ACLs can inherit permissions from parent objects, which may complicate changes. It’s crucial to comprehend how inheritance works. If you need to manage inherited permissions, you can use specific parameters while creating your ACL rules.
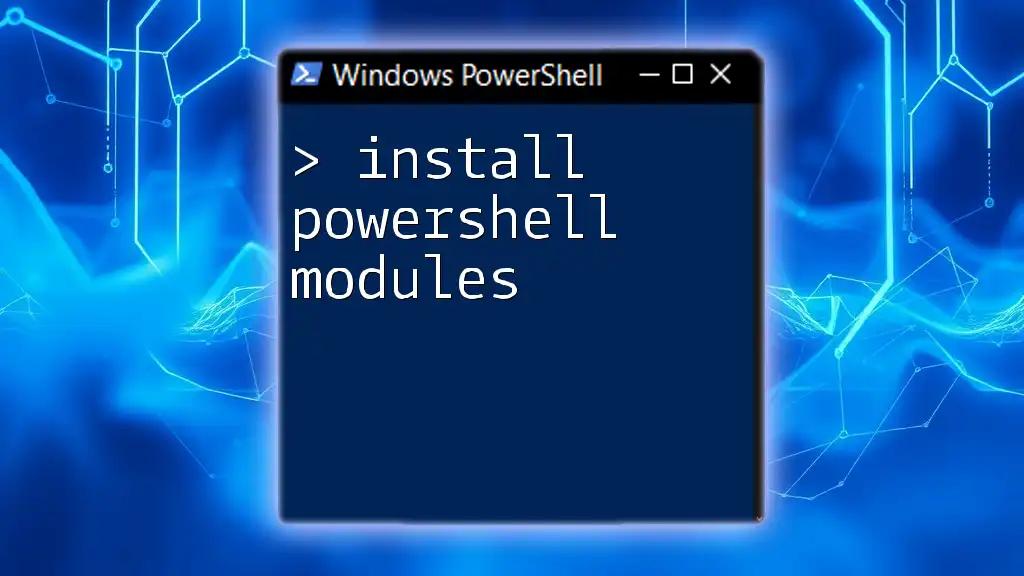
Conclusion
By mastering the `Set-Acl PowerShell` cmdlet, you can efficiently control access to files and directories within your system. Understanding the nuances of ACLs allows for better security and management of resources in any Windows environment. Keep exploring the potent capabilities of PowerShell for a more automated and streamlined approach to systems administration, and don't hesitate to delve deeper into ACL management techniques.