OpenSSL is a powerful toolkit for the Secure Sockets Layer (SSL) and Transport Layer Security (TLS) protocols, and it can be seamlessly used in PowerShell for tasks such as creating certificates or encrypting files.
Here’s a simple PowerShell command to generate a new RSA private key using OpenSSL:
openssl genpkey -algorithm RSA -out private_key.pem -pkeyopt rsa_keygen_bits:2048
What is OpenSSL?
OpenSSL is a robust, open-source toolkit that implements the Secure Sockets Layer (SSL) and Transport Layer Security (TLS) protocols. It provides various cryptographic functions for securing communications over computer networks, making it crucial for creating secure web applications and implementing various security measures. OpenSSL’s wide range of tools is essential for generating, managing, and verifying digital certificates, keys, and encryption methods.
Using OpenSSL in PowerShell empowers users to leverage the command-line capabilities of OpenSSL in a Windows environment. This combination allows for automation of security-related tasks and efficient handling of certificate operations directly from the PowerShell command line.
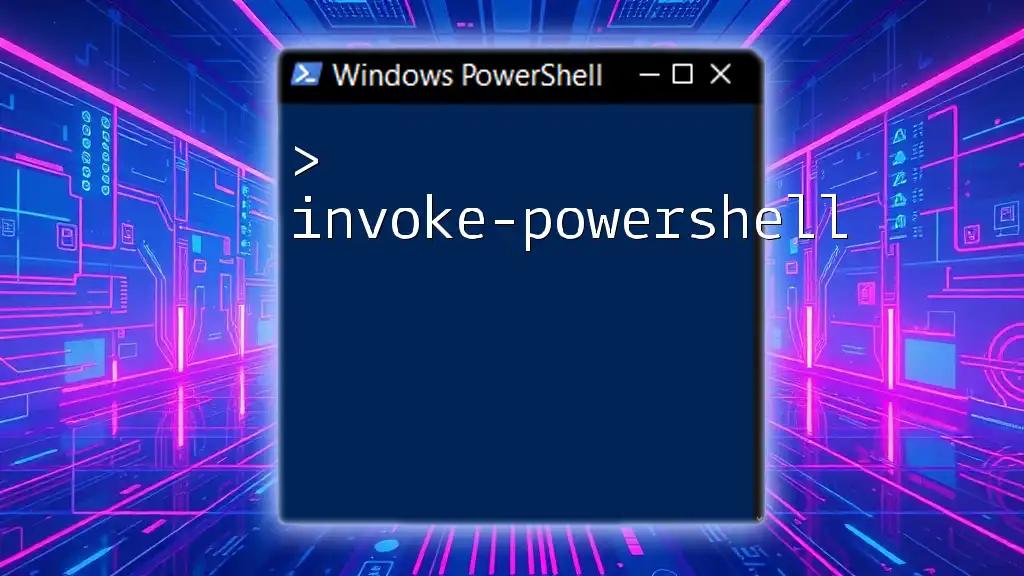
Setting Up OpenSSL in PowerShell
Installation of OpenSSL on Windows
To get started with OpenSSL, the first step is to install it on your Windows machine. Here’s how to do it:
-
Download OpenSSL for Windows: You can find binaries available at sites like [Shining Light Productions](https://slproweb.com/products/Win32OpenSSL.html) or other trusted sources.
-
Run the Installer: Execute the downloaded installer and follow the prompts. Make sure to choose the option that configures OpenSSL as a service.
-
Verify Your Installation: After installation, check if OpenSSL is correctly installed by opening PowerShell and running:
& "C:\OpenSSL-Win32\bin\openssl.exe" version
This should return the installed version of OpenSSL, confirming that it’s ready for use.
Configuring Environment Variables
Setting environment variables allows you to run OpenSSL from any command prompt or PowerShell window without providing the full path to the executable. Follow these steps:
-
Open System Properties: Right-click on 'This PC' or 'My Computer' and select 'Properties'. Then click on 'Advanced system settings'.
-
Environment Variables: In the 'System Properties' window, click on the 'Environment Variables' button.
-
Edit PATH Variable: Find the 'Path' variable in the 'System variables' section and click 'Edit'. Click 'New' and add the path to the OpenSSL `bin` directory, typically `C:\OpenSSL-Win32\bin`.
-
Save and Exit: Confirm your changes and close all windows. You can verify that the path is set by running the following command in PowerShell:
$Env:Path -split ';' | Where-Object { $_ -match "OpenSSL-Win32" }
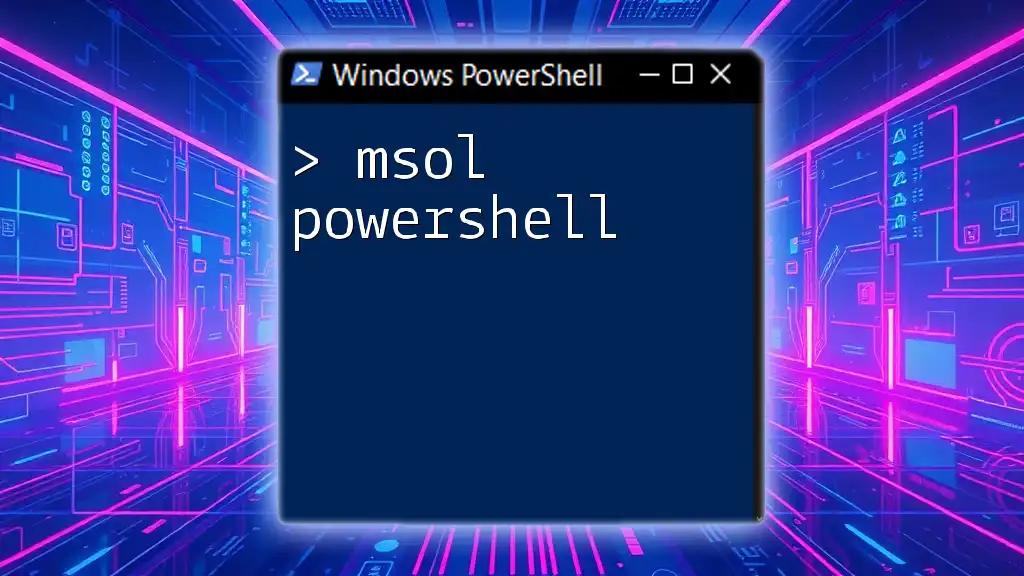
Using OpenSSL Commands in PowerShell
Now that OpenSSL is installed and configured, you can invoke its commands directly from PowerShell. Use the call operator `&` to execute OpenSSL commands.
Common OpenSSL Commands
Generating a Private Key
Generating a private key is a fundamental step in securing communications. Below is how to create a private key in PEM format:
& "C:\OpenSSL-Win32\bin\openssl.exe" genrsa -out mykey.pem 2048
Here, the `genrsa` command generates a new RSA private key of 2048 bits and saves it as `mykey.pem`.
Creating a Certificate Signing Request (CSR)
After generating a private key, you can create a Certificate Signing Request (CSR). This is often needed to obtain an SSL/TLS certificate from a Certificate Authority (CA). Execute the following command:
& "C:\OpenSSL-Win32\bin\openssl.exe" req -new -key mykey.pem -out mycsr.csr
This command prompts you for information about your organization, such as the Common Name (CN), which typically is your domain name.
Self-Signing a Certificate
For development or internal testing, you might want to create a self-signed certificate. Use this command:
& "C:\OpenSSL-Win32\bin\openssl.exe" x509 -req -days 365 -in mycsr.csr -signkey mykey.pem -out mycert.pem
This will create a self-signed certificate valid for 365 days.
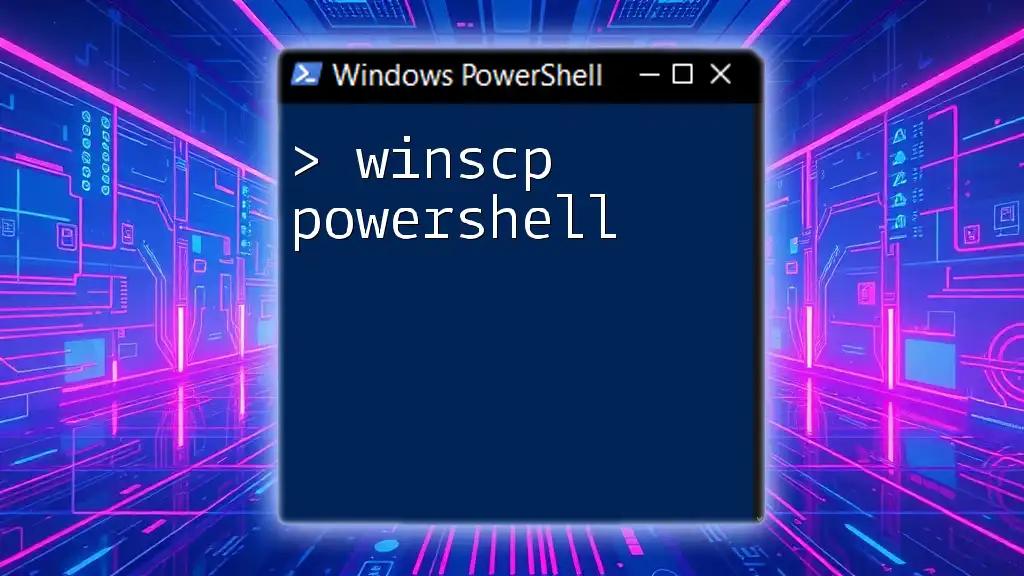
Common Tasks with OpenSSL in PowerShell
Converting Certificate Formats
Different applications and systems may require certificates in specific formats. For example, you might need to convert a certificate from PEM to DER format. Use the following command:
& "C:\OpenSSL-Win32\bin\openssl.exe" x509 -in mycert.pem -outform DER -out mycert.der
This command converts `mycert.pem` into DER format, saving it as `mycert.der`.
Verifying Certificates
You should regularly verify your certificates to ensure they are valid. The following command displays information about a certificate:
& "C:\OpenSSL-Win32\bin\openssl.exe" x509 -in mycert.pem -text -noout
This command outputs the details of the certificate, helping you check its validity, expiration, and public key.
Extracting Public Keys from Certificates
Sometimes, you might need the public key from your certificate. Use this command to extract it:
& "C:\OpenSSL-Win32\bin\openssl.exe" x509 -in mycert.pem -pubkey -noout
This command will output the public key associated with `mycert.pem`.
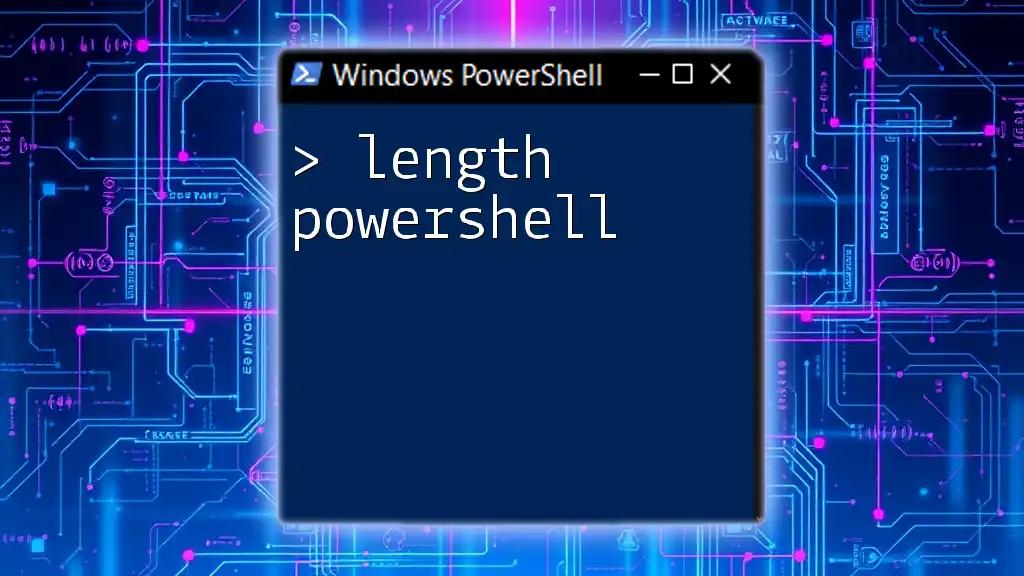
Troubleshooting Common Issues with OpenSSL in PowerShell
Common Errors and Their Solutions
-
Failed to Load OpenSSL DLLs: This often occurs if the environment variables weren't set correctly. Double-check to ensure that the OpenSSL `bin` path is included in your `PATH` variable.
-
Incorrect Configuration of Environment Variables: Make sure the paths are specified correctly without typos.
Tips for Effective Debugging
Using verbose modes can help identify issues during execution. You can enable verbose output for OpenSSL commands with the `-v` option, which provides detailed information on the workings of the command.
Additionally, checking for version compatibility between PowerShell and OpenSSL can prevent unexpected behaviors.

Best Practices When Using OpenSSL in PowerShell
To ensure the highest level of security and efficiency while working with OpenSSL in PowerShell, consider the following best practices:
-
Regularly Update OpenSSL: New vulnerabilities are discovered frequently; thus, keeping OpenSSL up to date is essential for maintaining security.
-
Use Strong Encryption Algorithms and Key Lengths: When generating keys, prefer using at least 2048-bit keys when possible, as shorter lengths are at risk of being compromised.
-
Properly Manage Private Keys and Certificates: Protect your private keys by setting appropriate file permissions and avoiding unnecessary exposure through public repositories.
-
Keep Track of Expiration Dates for Certificates: Implement a system or tool to alert you when certificates are nearing expiration to avoid unexpected downtimes.

Conclusion
Using OpenSSL in PowerShell provides a powerful toolset for managing certificates, securing communications, and performing cryptographic operations. By integrating OpenSSL with PowerShell’s command-line capabilities, users can streamline their security workflows effectively. Regular practice with these commands ensures familiarity and proficiency in handling real-world security tasks.
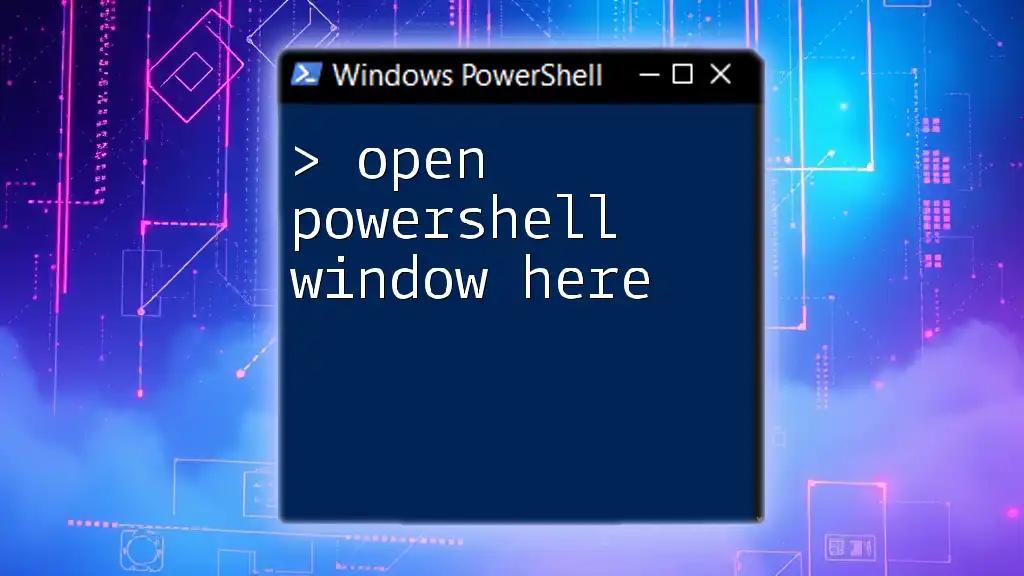
Additional Resources
For further learning and exploration, consider diving into the following resources:
- Official OpenSSL Documentation
- PowerShell Documentation
- Community forums and support channels for PowerShell users
With diligent practice and understanding, you will harness the full potential of OpenSSL in your PowerShell environment.