To convert a string to lowercase in PowerShell, you can use the `ToLower()` method, which easily transforms your text into all lowercase letters.
Here’s a simple code snippet to demonstrate this:
$originalString = 'HELLO, WORLD!'
$lowercaseString = $originalString.ToLower()
Write-Host $lowercaseString
Understanding Lowercase in PowerShell
What is Case Sensitivity?
When working with text in programming and scripting, case sensitivity refers to how a language treats uppercase and lowercase letters differently. In many programming languages, including PowerShell, the case of characters can significantly affect how they are interpreted. This distinction is particularly important when dealing with variables, function names, and string comparisons.
Importance of Lowercase Data
Using lowercase consistently can improve readability and ensure that scripts are easier to follow and understand. For example, when developers adhere to a specific casing style, it can eliminate confusion about variable names or function calls. Additionally, in databases and various data management scenarios, maintaining case consistency can be crucial for data integrity. When data is fetched from or stored in a case-sensitive environment, discrepancies in case can lead to unexpected results or errors.
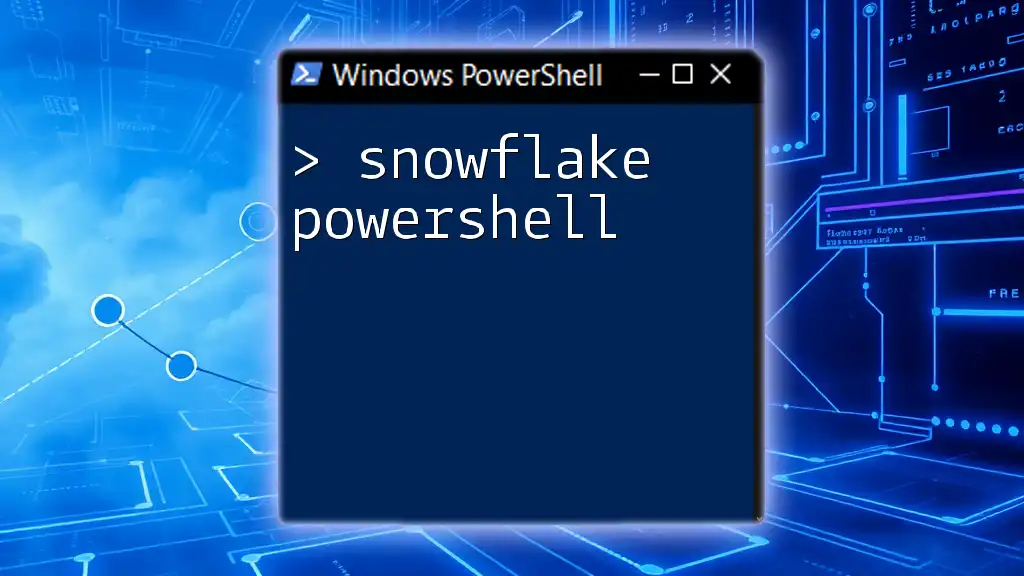
PowerShell's `ToLower` Method
Overview of the `ToLower` Method
The `ToLower` method in PowerShell is designed to convert all uppercase letters in a string to their lowercase counterparts. This method is a key tool for ensuring that text data is uniform, especially when comparability is required.
Syntax of `ToLower`
The syntax for the `ToLower` method is straightforward and appears as follows:
$stringVariable.ToLower()
This simple command calls the `ToLower` method on the string variable, allowing for seamless conversion.
Example Usage of `ToLower`
Here's a basic example of how to use the `ToLower` method:
$myString = "Hello World"
$lowerString = $myString.ToLower()
Write-Output $lowerString # Outputs: hello world
In this example, the string `"Hello World"` is transformed into `"hello world"` using the `ToLower` method. The output demonstrates the effectiveness and simplicity of using this method in PowerShell scripts.
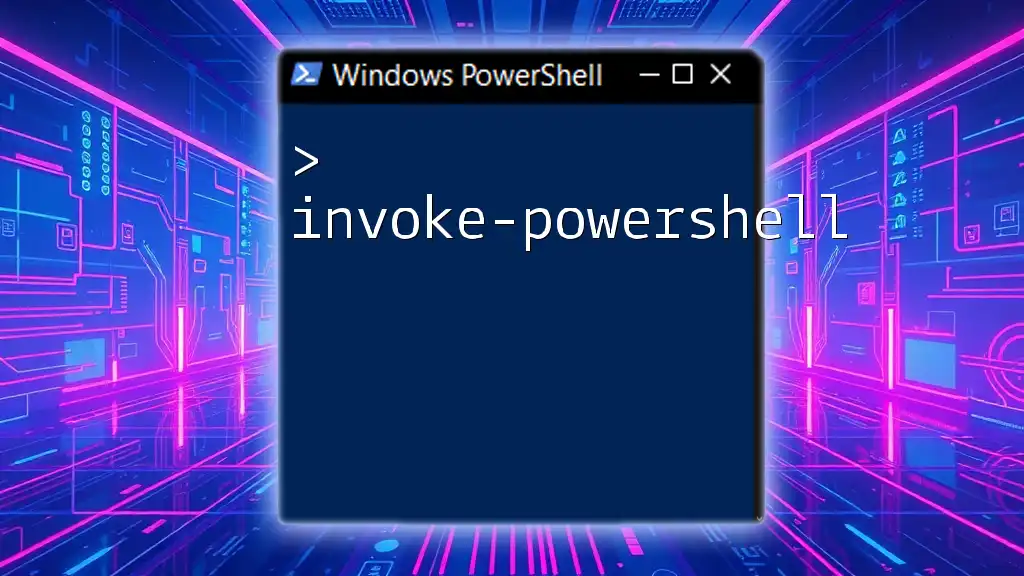
Working with PowerShell Strings
Creating and Modifying Strings
PowerShell allows the creation of strings using either single or double quotes. For instance, you can define a string as follows:
$string = "PowerShell Scripting"
To convert this string to lowercase, the following command can be utilized:
$lowerString = $string.ToLower()
This command effectively converts the entire content of `$string` to lowercase, enhancing its usability for various operations.
Converting Multiple Strings
When working with multiple strings, PowerShell makes it easy to apply the `ToLower` method across arrays of strings. Below is an illustration of how you can achieve this:
$stringArray = @("Hello", "WORLD", "PowerShell")
$lowerArray = $stringArray | ForEach-Object { $_.ToLower() }
Write-Output $lowerArray # Outputs: hello, world, powershell
In this case, an array of strings is processed, and each element is transformed into its lowercase version. This not only showcases the functionality but also the power of the pipeline in PowerShell.
String Properties and Methods
PowerShell offers a variety of string methods that developers can leverage. Alongside `ToLower`, some common methods include `ToUpper`, which converts strings to uppercase, and `Trim`, which removes whitespace from the beginning and end of strings. Familiarizing oneself with these methods can greatly expand a developer's toolkit and enhance string manipulation capabilities in scripts.
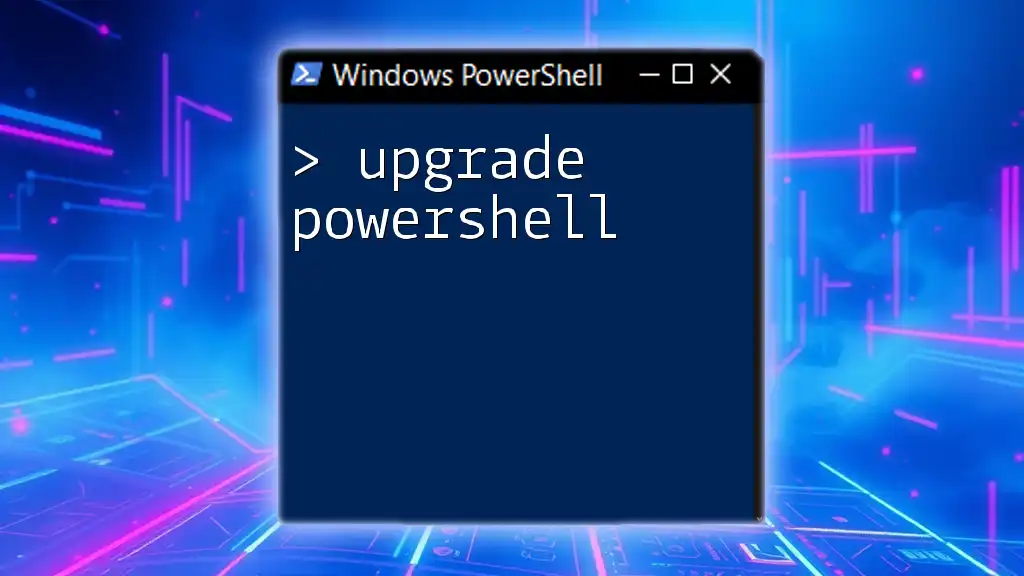
Advanced Use Cases
Working with Data Objects
In situations where you're dealing with objects, the `ToLower` method can play an essential role in standardizing data. For instance:
$users = Get-ADUser -Filter * | Select-Object -Property Name, Email
$lowerUsers = $users | ForEach-Object {
$_.Name = $_.Name.ToLower()
$_
}
In this example, we retrieve a list of Active Directory users, transforming each user's name to lowercase. This practice can be vital when conducting searches or comparisons where case might otherwise disrupt intended outcomes.
Using `ToLower` in Conditional Logic
When it comes to conditional logic, the `ToLower` method can be tremendously helpful for case insensitive comparisons. The following example demonstrates this:
$input = Read-Host "Enter a Command"
if ($input.ToLower() -eq "exit") {
Write-Output "Exiting the script."
}
In this case, the input collected from the user is converted to lowercase before comparison, ensuring that regardless of how the user types the command (e.g., "Exit", "EXIT", or "exit"), the script will recognize it appropriately.
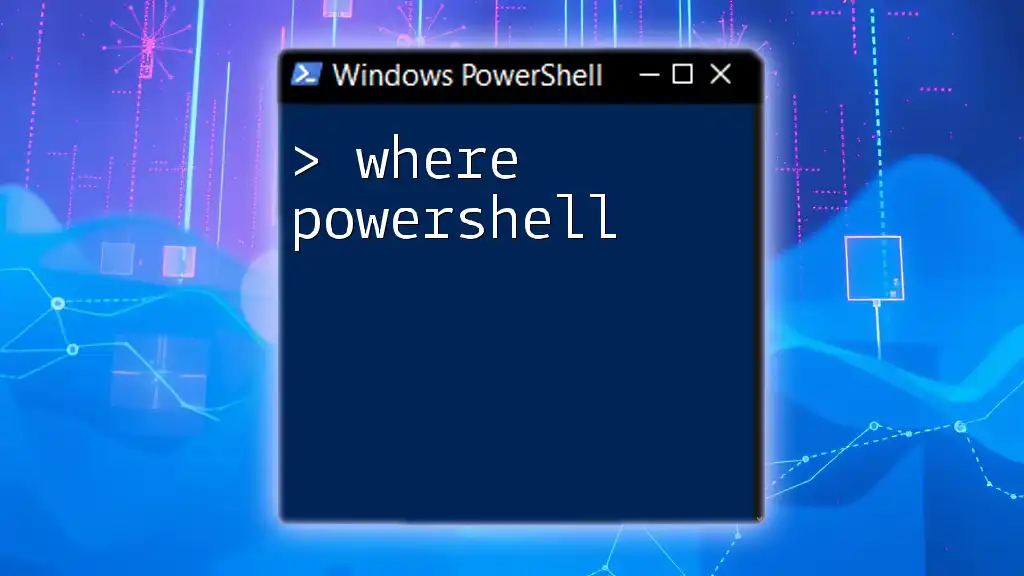
Best Practices for Using Lowercase in PowerShell
Tips for Effective Scripting
For effective PowerShell scripting, one important best practice involves the consistent use of casing. By adhering to a specific casing standard throughout your scripts, you can enhance maintainability and readability. It's also wise to document your code adequately, providing comments that clarify the intent behind specific lines, especially those involving string manipulation.
Common Mistakes to Avoid
One common mistake developers often make is forgetting to call `ToLower` when needed. This oversight can lead to cases where comparisons fail or unexpected results occur. Staying aware of the necessity to standardize case can save a lot of debugging time.
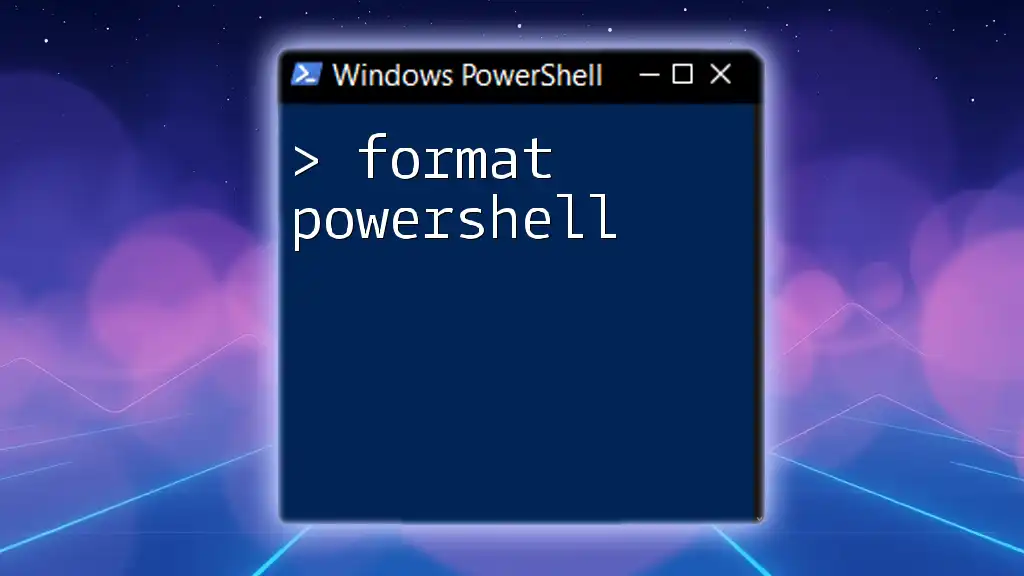
Conclusion
To effectively utilize PowerShell and navigate its capabilities, understanding the `ToLower` method and the implications of lowercase data is paramount. By mastering these concepts, you can write clear, effective scripts that enhance usability and ensure data consistency in your PowerShell endeavors.
Additional Resources
For those seeking to delve deeper into PowerShell string manipulation and case sensitivity, a wealth of materials is available online, from official documentation to community forums and dedicated tutorials.
Call to Action
Practice using `ToLower` and embrace it as a tool in your scripting arsenal. As you refine your skills, consider how these techniques can apply to larger projects and scripts. Happy scripting!