"Snowflake PowerShell" refers to using PowerShell commands to efficiently interact with Snowflake, a cloud-based data warehousing service, enabling users to perform data operations seamlessly.
Here's a simple example of how to connect to Snowflake using PowerShell:
# Set parameters for Snowflake connection
$connection = "Data Source=myaccount.snowflakecomputing.com;User ID=myuser;Password=mypassword;Database=mydatabase;Schema=myschema;Warehouse=mywarehouse"
# Establish the connection
$connectionString = New-Object System.Data.Odbc.OdbcConnection($connection)
$connectionString.Open()
Write-Host 'Connected to Snowflake successfully!'
$connectionString.Close()
Introduction to Snowflake PowerShell
What is Snowflake?
Snowflake is a powerful cloud-based data platform designed to handle complex data workloads. It provides flexible and scalable data warehousing solutions that enable businesses to manage their analytics and data storage with ease. Some of its key features include automatic scaling, pay-for-what-you-use pricing, and seamless data sharing. By leveraging the cloud, Snowflake allows organizations to focus on data insights rather than infrastructure management.
Why Use PowerShell with Snowflake?
PowerShell is a versatile task automation and configuration management framework. Combining Snowflake with PowerShell allows users to automate repetitive tasks, manage data more efficiently, and integrate Snowflake into broader IT workflows. Use cases include automating data imports/exports, managing Snowflake resources such as warehouses and databases, and executing regular reports.
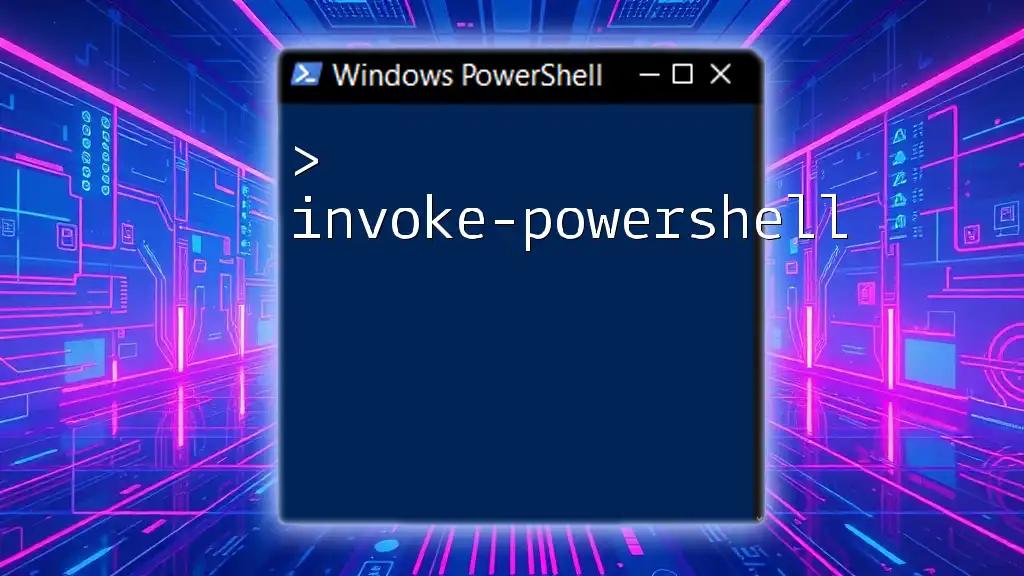
Setting Up PowerShell for Snowflake
Prerequisites
Before diving into using Snowflake with PowerShell, ensure the following software is installed:
- Windows operating system
- PowerShell (version 5.1 or higher preferred)
- SnowSQL (Snowflake’s command-line client)
Additionally, ensure that you have the necessary permissions in your Snowflake account to create, read, update, and delete data.
Installing the Snowflake PowerShell Module
To simplify working with Snowflake, the Snowflake PowerShell module needs to be installed. This can be done easily by executing the following command in PowerShell:
Install-Module -Name Snowflake
This module provides various cmdlets specifically tailored for interfacing with your Snowflake environment, which enhances scripting capabilities and integrates seamlessly with the Snowflake Cloud Data Platform.
Establishing a Connection to Snowflake
To start interacting with Snowflake from PowerShell, you will need to establish a connection. Here’s how to do that:
$Connection = Connect-Snowflake -Account '<your_account>' -Username '<your_username>' -Password '<your_password>'
Replace `<your_account>`, `<your_username>`, and `<your_password>` with your respective Snowflake account details. If successful, this command will create a connection object that can be used for subsequent commands. If you encounter issues, check your credentials and network settings.
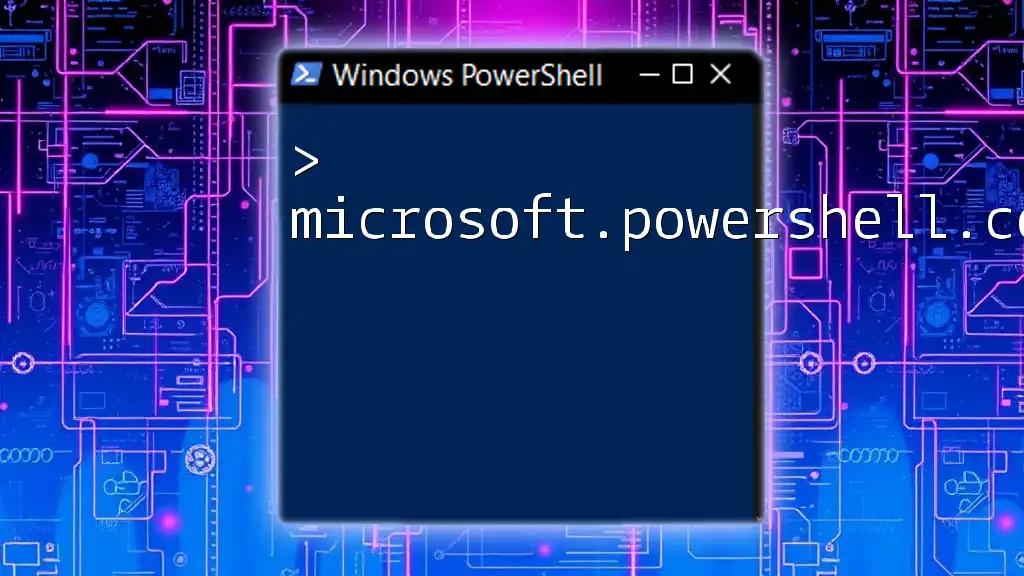
Basic PowerShell Commands for Snowflake
Querying Data from Snowflake
One of the primary tasks you'll perform using PowerShell with Snowflake is querying data. You can execute SQL statements directly from your PowerShell session. For example, to retrieve all records from a table, you would use:
$Query = "SELECT * FROM my_table"
Invoke-SnowflakeQuery -Connection $Connection -Query $Query
After executing this command, you’ll receive the query results—often in a structured format—allowing you to manipulate or display the data as needed.
Inserting Data into Snowflake
To add data to a Snowflake table, you can utilize the `INSERT` SQL command. Here’s how it works:
$InsertQuery = "INSERT INTO my_table (column1, column2) VALUES ('value1', 'value2')"
Invoke-SnowflakeQuery -Connection $Connection -Query $InsertQuery
This command will insert a new record into `my_table`. Consider performing batch insertions for larger datasets to optimize performance and reduce execution time.
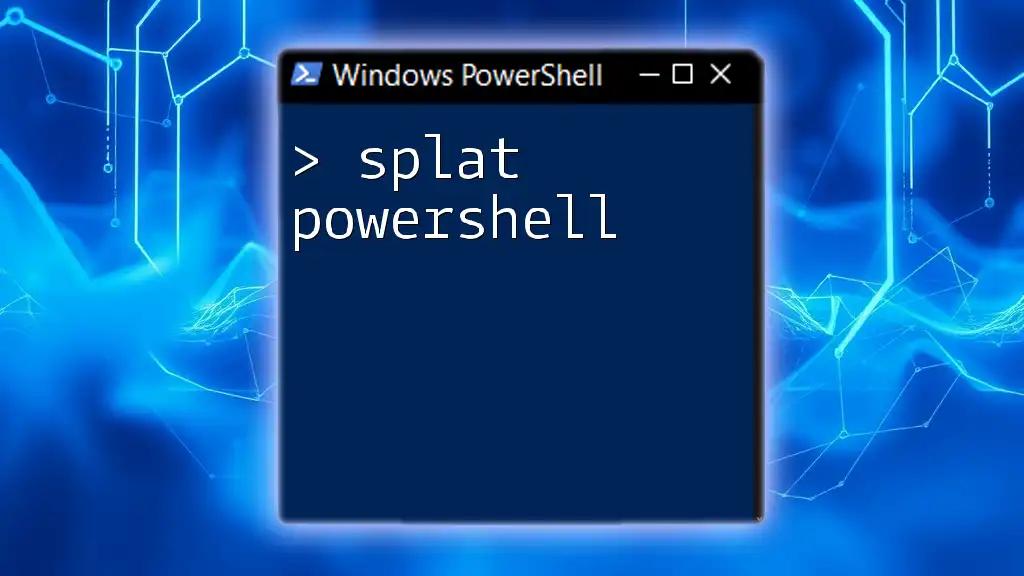
Advanced Snowflake PowerShell Usage
Managing Snowflake Warehouses
Snowflake's architecture includes warehouses that provide the compute resources needed to manage and process queries. You can manage these warehouses directly from PowerShell, allowing for scripting automated environments. For example, to resume a warehouse, execute:
Invoke-SnowflakeQuery -Connection $Connection -Query "ALTER WAREHOUSE my_warehouse RESUME;"
This command is invaluable for automating workloads where consistent resource availability is critical, such as during peak business hours.
Working with Snowflake Stages
Snowflake stages allow you to store data files for loading or unloading. To upload a local file to a Snowflake stage, use:
Invoke-SnowflakeQuery -Connection $Connection -Query "PUT file://path/to/local/file @my_stage;"
This command uploads the specified file directly to `my_stage`. It’s essential to correctly reference file paths and ensure your permissions allow for file operations.
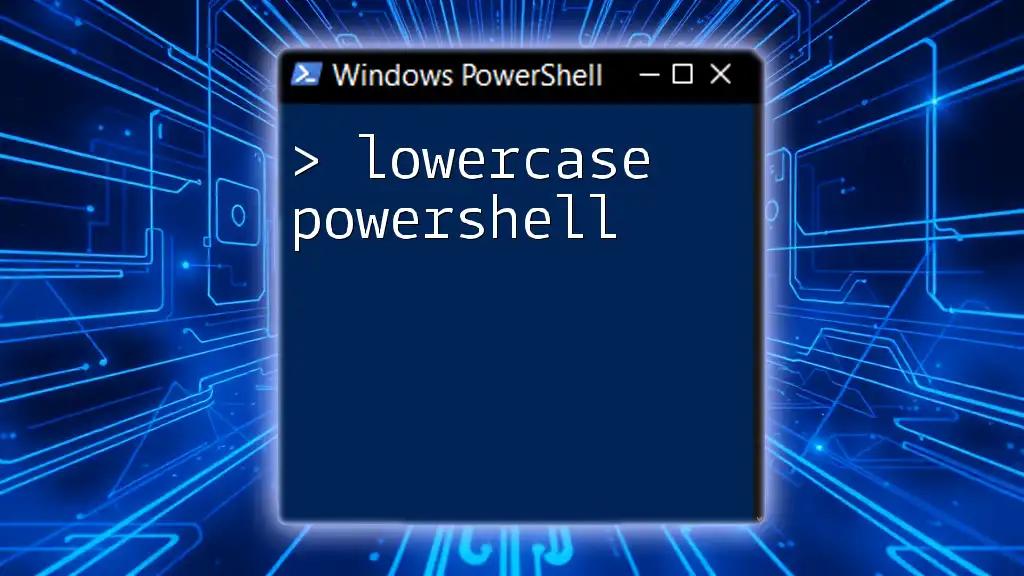
Practical Examples and Use Cases
Using PowerShell Scripts for ETL Processes
PowerShell scripts can efficiently manage Extract, Transform, Load (ETL) processes in Snowflake. By integrating with Snowflake and other data sources, you can automate data cleaning, transformation, and loading tasks. An example of such automation would involve using PowerShell to scrape data from an API and load it into Snowflake daily.
To schedule these scripts, you can utilize the Windows Task Scheduler, giving you the ability to run your ETL processes at predefined intervals, ensuring data is always up to date.
Monitoring Snowflake Usage with PowerShell
Monitoring your Snowflake environment is crucial for optimizing performance and managing costs. You can query the background processes like this:
Invoke-SnowflakeQuery -Connection $Connection -Query "SELECT * FROM table(query_history());"
This command retrieves recent query execution details, allowing you to monitor usage patterns, potential bottlenecks, and resource consumption.
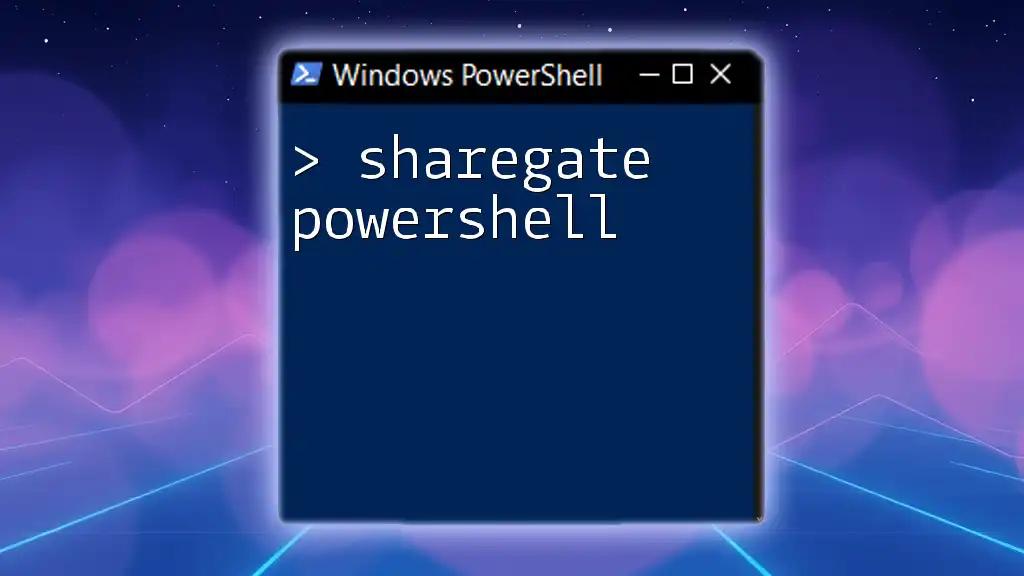
Best Practices for Using PowerShell with Snowflake
Error Handling and Logging in PowerShell
When working with scripts, it’s vital that you implement error handling. This allows your scripts to gracefully manage exceptions and keep you informed of issues. Here’s a simple error handling structure:
try {
Invoke-SnowflakeQuery -Connection $Connection -Query $Query
} catch {
Write-Error "An error occurred: $_"
}
Using `try-catch` blocks ensures that any errors encountered during execution are logged, which can significantly aid in debugging.
Optimizing Performance
To enhance performance when executing commands, consider optimizing SQL queries for better response times. Additionally, using pipeline commands efficiently can significantly reduce processing time, as you can stream data directly from Snowflake into PowerShell variables without needing to load it all at once.
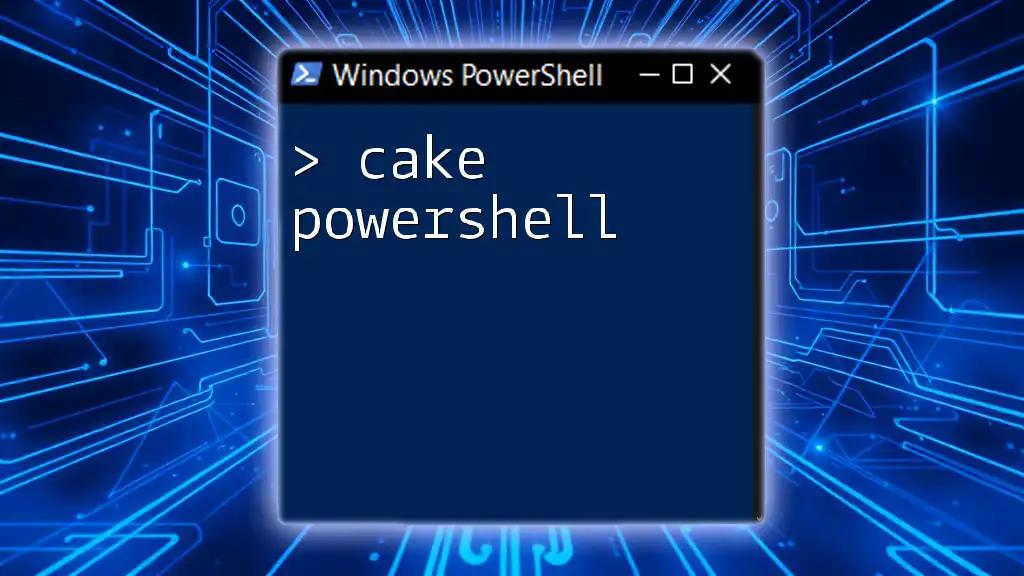
Conclusion
Snowflake PowerShell is an immensely powerful toolset that allows users to manage cloud data warehousing efficiently and automate complex processes with relative ease. By understanding how to set up, execute, and manage PowerShell commands with Snowflake, you can streamline your data operations dramatically. As you practice creating your own scripts, keep the learning resources handy and experiment with different commands to become proficient in automating your Snowflake environment.
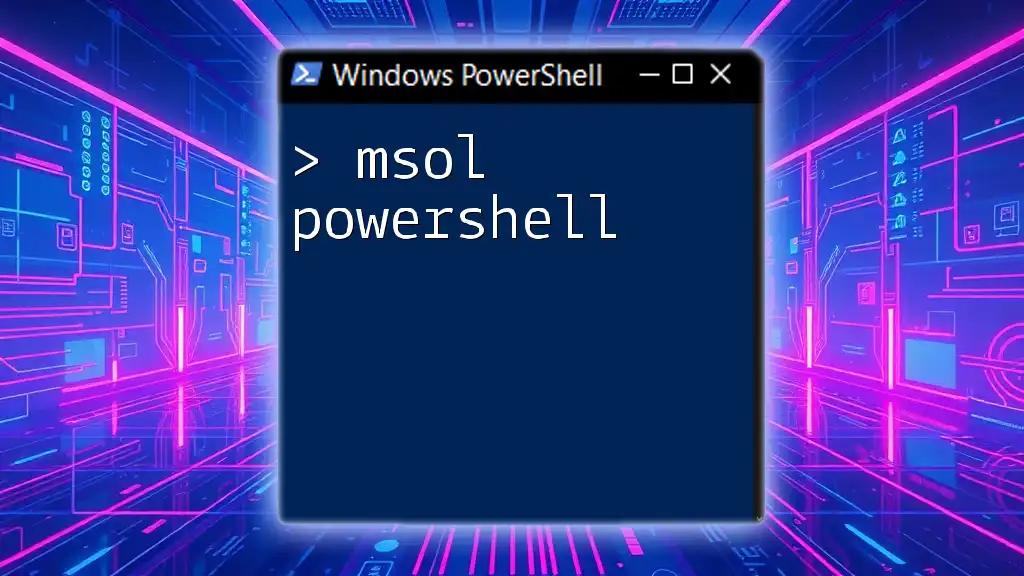
Additional Resources
- Official Snowflake Documentation: For detailed usage and feature explanations.
- PowerShell Community Forums: Engage with other PowerShell users for tips and support.
- Recommended Books and Courses on PowerShell and Snowflake: Explore further learning options to deepen your knowledge and skills.