In PowerShell, the `-notin` operator is used to determine if a value does not exist within a specified collection or array.
$fruit = 'Apple', 'Banana', 'Cherry'
if ('Orange' -notin $fruit) {
Write-Host 'Orange is not in the fruit list.'
}
Understanding "notin" in PowerShell
What is "notin"?
The `notin` operator in PowerShell is a logical operator that allows you to check if a given value is not present in a specified collection or array. It essentially serves as a negation of the `-in` operator, which checks for inclusion. Understanding how to use `notin` is crucial, especially when you want to filter out unwanted items from your data sets or conditions.
Why Use "notin"?
Using the `notin` operator is vital for exclusionary logic in PowerShell scripts. For instance, if you are managing users and need to filter out those who do not belong to a certain group, `notin` becomes indispensable. This operator is particularly useful for enhancing the efficiency and clarity of your scripts.
Consider a scenario where you're processing a list of operations and need to ignore certain commands or processes. `notin` allows you to streamline your checks, ensuring that only relevant data is processed.
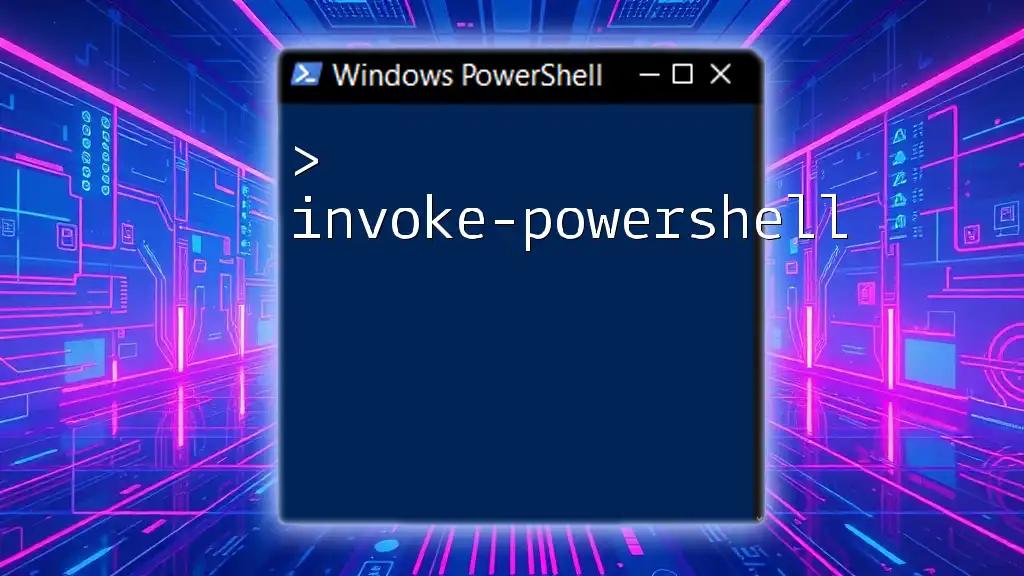
Syntax of "notin"
Basic Syntax Structure
The syntax for using `notin` is straightforward. The basic structure looks like this:
$value -notin $collection
Where:
- `$value` is the item you want to check.
- `$collection` is an array or collection that you are checking against.
Example of "notin" Syntax
Here’s a simple code snippet that illustrates how the `notin` operator can be applied:
$array = 1, 2, 3, 4, 5
if (-not(3 -in $array)) {
"3 is not in the array."
}
In this example, the code checks if the number 3 is not part of the array. Since 3 is included in the array, the message will not display.

Implementing "notin" in PowerShell Scripts
Using "notin" with Arrays
The `notin` operator can be used effectively with arrays. When you want to check the absence of an element in an array, `notin` simplifies your code.
For example:
$fruits = "apple", "banana", "cherry"
if ("grape" -notin $fruits) {
"Grape is not in the fruits array."
}
In this case, since "grape" is not found in the `$fruits` array, the statement will print confirming that grape is indeed not part of the collection.
Combining "notin" with Conditional Statements
You can integrate the `notin` operator into `if` statements to control script flow based on inclusion criteria. This is particularly useful in scenarios with user permissions or specific command exclusions.
For instance:
$allowedUsers = "admin", "manager"
$currentUser = "guest"
if ($currentUser -notin $allowedUsers) {
"Access denied for $currentUser."
}
Here, the script checks if the `$currentUser` is not in the allowed list. If the user is not authorized, they receive an access denial message.
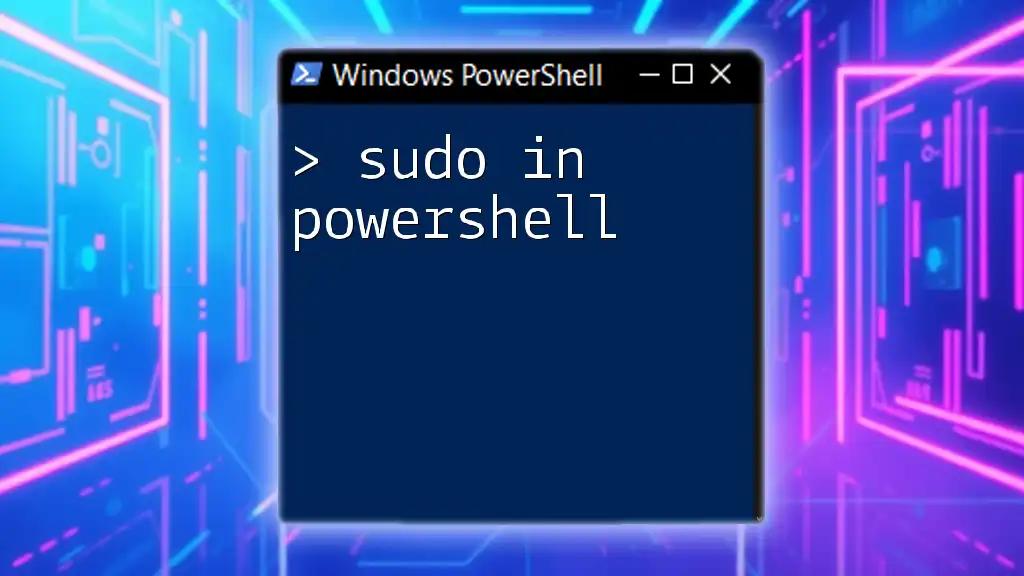
Complex Use Cases for "notin"
Filtering Data with "notin"
One of the strongest use cases for `notin` is filtering data. It becomes especially powerful when used with cmdlets that return collections, such as `Get-Process`.
Consider the following example that filters out unwanted processes:
$excludedProcesses = "explorer", "powershell"
Get-Process | Where-Object { $_.Name -notin $excludedProcesses }
In this code, `Get-Process` retrieves all running processes, but the `Where-Object` clause ensures that any processes named explorer or powershell are excluded.
Using "notin" in Functions
Creating reusable functions that leverage the `notin` operator can enhance scripting efficiency. Functions allow for modular and organized code, improving readability and maintainability:
function Test-UserAccess {
param (
[string[]]$allowedUsers,
[string]$currentUser
)
if ($currentUser -notin $allowedUsers) {
return "$currentUser is not an allowed user."
}
return "$currentUser has access."
}
This function checks if a current user is part of an allowed list. It returns a tailored message based on the outcome of the `notin` check.

Common Mistakes and Troubleshooting
Common Misconceptions About "notin"
A frequent error in using the `notin` operator arises from confusion with the `-eq` operator. Users might mistakenly use `notin` for singular comparisons, which do not warrant a collection context.
Understanding that `notin` is intended for checks against arrays or collections will alleviate most common pitfalls.
Debugging "notin" Statements
To effectively debug statements that incorporate `notin`, it’s essential to break down the conditions. Utilizing `Write-Host` or logging the values can help you see what exactly is being checked at any given time.
For example:
$currentUser = "guest"
$allowedUsers = "admin", "manager"
Write-Host "Checking if $currentUser is allowed..."
if ($currentUser -notin $allowedUsers) {
Write-Host "$currentUser is not an allowed user."
}
This clarity in logging allows you to trace through the logic seamlessly.
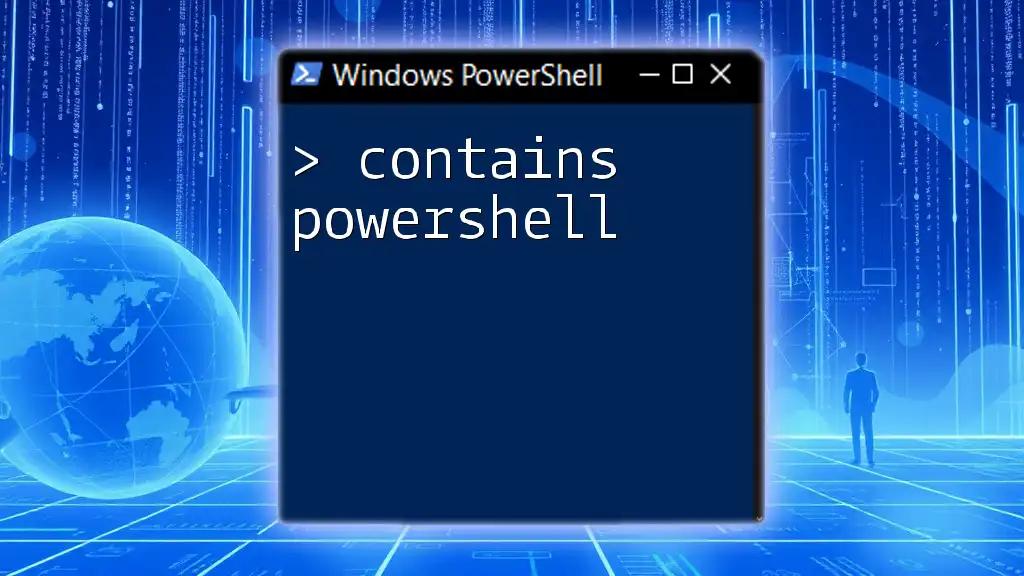
Best Practices When Using "notin"
Efficiency in Scripting
To write optimal scripts using `notin`, ensure that the collections you're working with are well-defined. Using arrays that are initialized properly aids in preventing unexpected behavior. Leveraging `notin` effectively minimizes script clutter while improving execution speed by short-circuiting unnecessary conditions.
Real-World Applications of "notin"
In industry applications, `notin` has been utilized in security scripts, user management, and data filtration operations. For instance, a network security script might exclude certain IPs from logging to improve focus on suspicious activity while ignoring regular traffic.
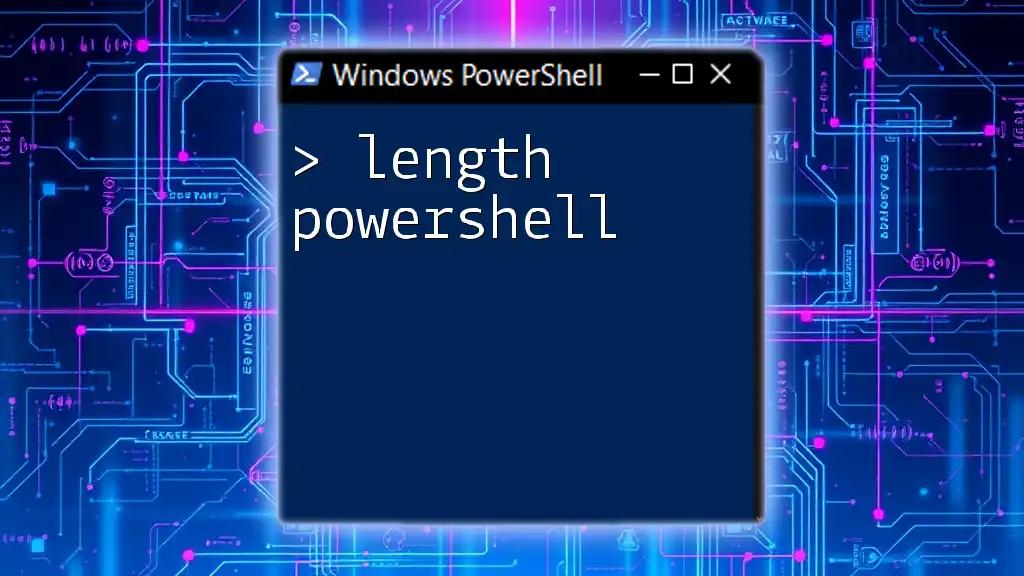
Conclusion
The `notin` operator in PowerShell is an essential tool that fosters more precise scripting, allowing users to flirtatiously touch upon exclusion within their code. By mastering `notin`, PowerShell users can significantly streamline their commands and enhance data management capabilities, ensuring that their scripts remain both efficient and effective.
Further Learning Resources
To deepen your understanding of the `notin` operator and its applications, exploring additional resources like the official Microsoft documentation on PowerShell operators will be invaluable. Engaging with communities and tutorials will further enrich your learning journey.