LINQ (Language Integrated Query) in PowerShell allows you to query and manipulate collections using a simpler and more expressive syntax, similar to queries in SQL.
Here's a code snippet demonstrating how to use LINQ in PowerShell to filter an array of numbers:
$numbers = 1..10
$evenNumbers = [System.Linq.Enumerable]::Where($numbers, { $_ % 2 -eq 0 })
$evenNumbers
Understanding LINQ
LINQ, or Language Integrated Query, is a powerful feature that allows developers to write queries directly within programming languages. Originally introduced in .NET, LINQ streamlines the process of manipulating and querying data, making it intuitive and efficient.
The key concepts of LINQ include:
- Query Syntax vs. Method Syntax: LINQ supports both declarative query syntax and method-based syntax, allowing you to choose the most readable form for your context.
- Queryable Collections and Deferred Execution: LINQ queries can be executed at a later time, preventing unnecessary data retrieval and improving performance.

PowerShell and LINQ
PowerShell is built on the .NET Framework, which provides access to LINQ functionalities natively. By leveraging LINQ, PowerShell scripts can achieve more concise code with improved performance for data manipulation tasks.
Using LINQ in PowerShell allows for:
- Performance Enhancements: LINQ's optimized query execution can outperform traditional looping methods.
- Improved Readability: With fewer lines of code, LINQ enhances the clarity of your scripts.
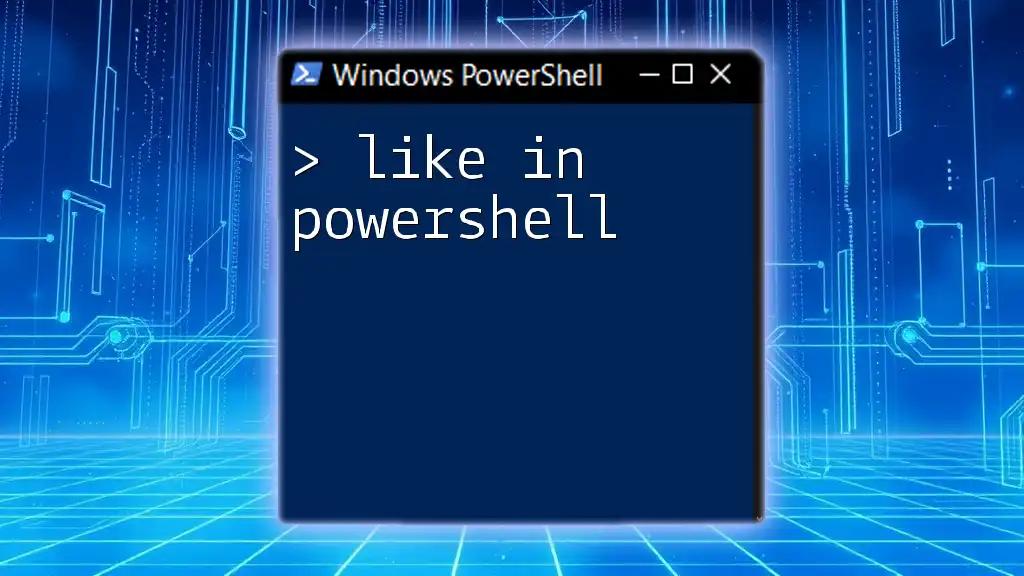
Getting Started with LINQ in PowerShell
Setting Up the Environment
To use LINQ in PowerShell, ensure you are working with PowerShell 5.0 or later, as these versions have improved .NET integration. You can reference the LINQ namespaces directly in your scripts to access the LINQ methods necessary.
Basic LINQ Queries
A great way to start using LINQ is by querying simple data types like arrays. Here's an example illustrating how to select even numbers from an array:
$numbers = 1..10
$evenNumbers = [System.Linq.Enumerable]::Where($numbers, {$_ % 2 -eq 0})
$evenNumbers
In this snippet, we define an array of numbers from 1 to 10 and then filter it using LINQ's Where method. The condition `{$_ % 2 -eq 0}` checks if each number is even, returning only those that meet the criteria.
Filtering Data using LINQ
You can also work with lists of objects for more complex queries. Suppose you have a list of user objects and want to filter them based on their age. The following snippet demonstrates this:
$users = @(
[PSCustomObject]@{Name='Alice'; Age=30},
[PSCustomObject]@{Name='Bob'; Age=25}
)
$filteredUsers = [System.Linq.Enumerable]::Where($users, { $_.Age -gt 26 })
$filteredUsers
Here, we create a collection of user objects and filter out those older than 26. The Where method checks the condition, returning only the user Alice, as she meets the criteria.

Advanced LINQ Techniques
Ordering Data with LINQ
Sorting data is another powerful application of LINQ. If you want to sort users by age, you could do this:
$orderedUsers = [System.Linq.Enumerable]::OrderBy($users, { $_.Age })
$orderedUsers
In this example, the OrderBy method arranges the users in ascending order based on their ages, with output displaying Bob followed by Alice.
Grouping Data with LINQ
Grouping is essential when you need to categorize data points. Let's look into grouping users by age:
$groupedUsers = [System.Linq.Enumerable]::GroupBy($users, { $_.Age })
This allows you to see how many users exist for each age group. Grouping makes it easier to perform aggregate calculations and analyses based on specific attributes of your data.
Joining Data with LINQ
LINQ also allows you to join multiple collections, similar to SQL joins. For instance, if you had two collections—users and their associated roles—you could leverage LINQ to consolidate this information for further analysis.
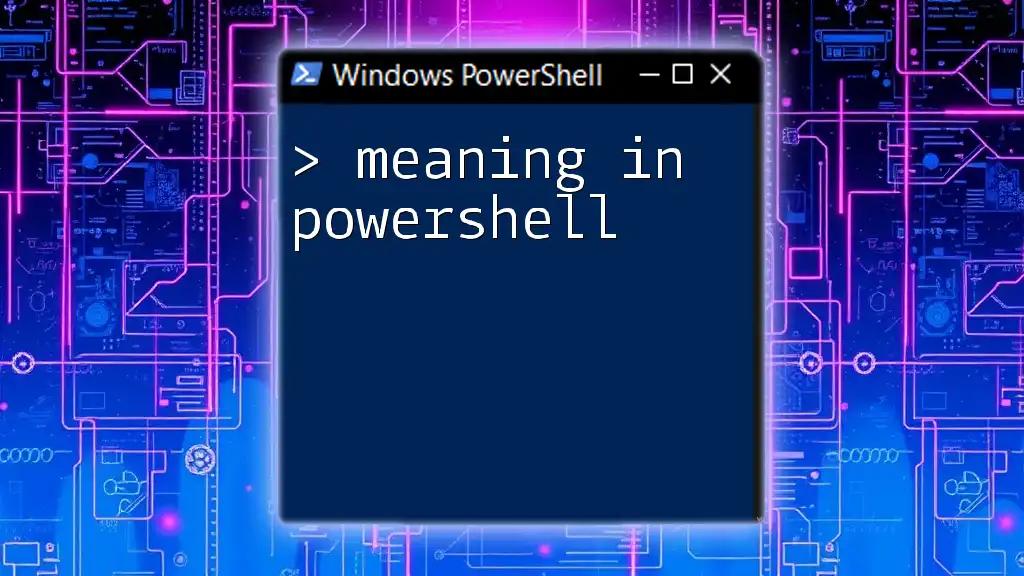
Performance Considerations
When it comes to performance, LINQ often surpasses traditional iterative loops. Implementing LINQ can lead to cleaner code and better performance, especially for large datasets. However, it's essential to understand when to use LINQ effectively.
For instance, if you're working with a small collection, traditional methods might be just as efficient. However, as the data set grows, the optimizations inherent in LINQ become invaluable.
Best Practices for Optimizing LINQ Queries
- Avoid unnecessary computations: Utilize deferred execution to limit performance hits.
- Choose the right method: Understand the difference between Where and FirstOrDefault to prevent pulling unnecessary data.
- Profile performance: Always profile your LINQ queries to ensure they're meeting your performance needs.
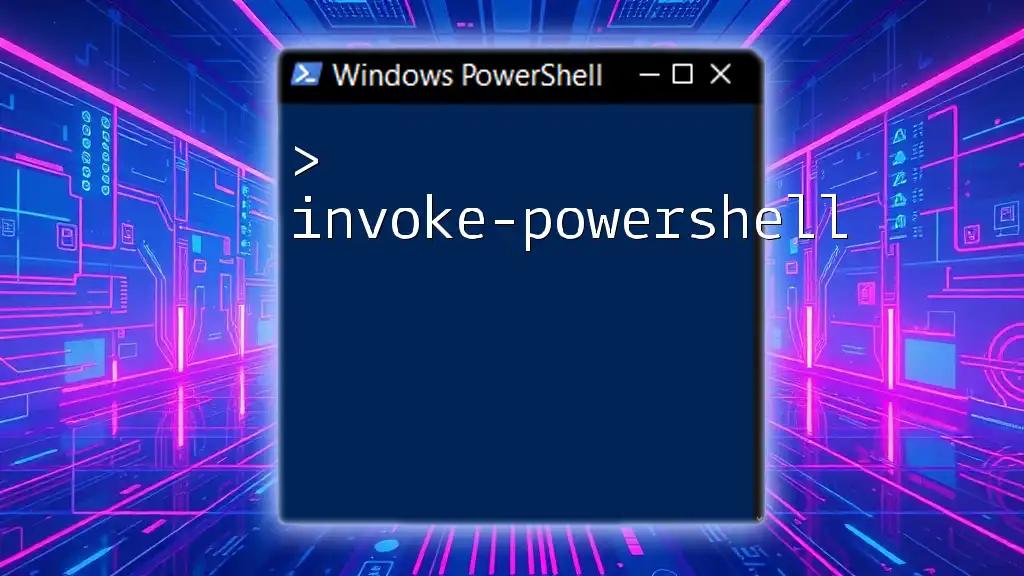
Common LINQ Patterns in PowerShell
FirstOrDefault and SingleOrDefault
Two pivotal methods in LINQ are FirstOrDefault and SingleOrDefault. These methods allow you to retrieve items based on specific criteria:
- FirstOrDefault returns the first element that matches a given condition or a default value if no elements are found.
- SingleOrDefault expects that there will be either one or zero elements that satisfy the criteria—throwing an error if there's more than one match.
Aggregate Functions
LINQ also provides several aggregate functions like Sum, Count, and Average. For example, if you want to count how many users are older than a specific age, you could run:
$userCountAbove30 = [System.Linq.Enumerable]::Count($users, { $_.Age -gt 30 })
$userCountAbove30
This simplicity is one of the many advantages of utilizing LINQ in your PowerShell scripts.
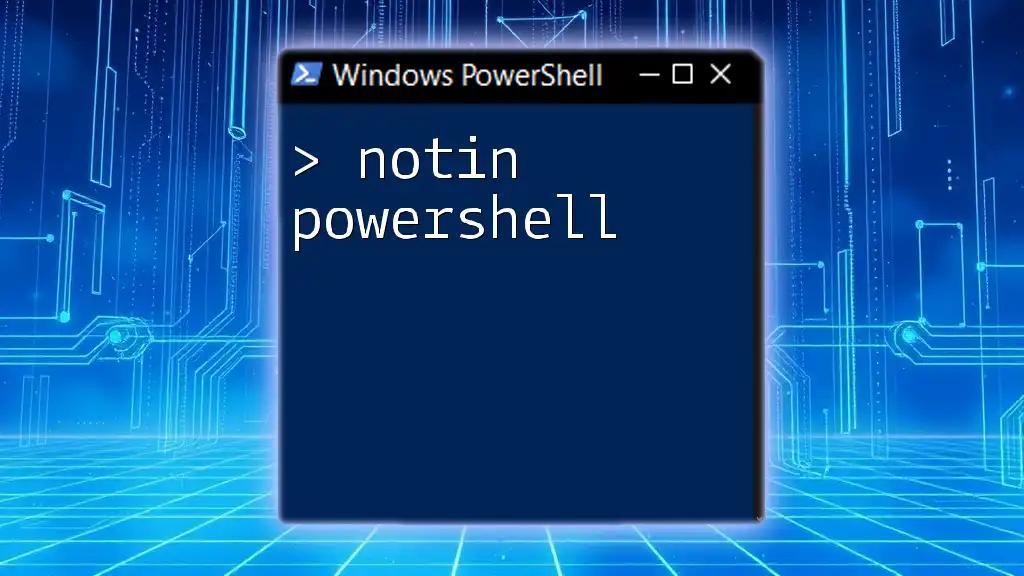
Conclusion
By understanding and utilizing LINQ in PowerShell, you equip yourself with a powerful toolkit for efficient data manipulation. The benefits of performance, readability, and maintainability make LINQ an essential skill for anyone looking to enhance their PowerShell capabilities. As you continue to explore LINQ, practice with different data sets, and challenge yourself with more complex queries, you'll find that the skills you develop will significantly improve your scripting prowess.

Further Reading and Resources
For those looking to expand their knowledge, various resources exist both online and in print. Consider exploring Microsoft documentation for PowerShell and LINQ, forums for community support, and books focused on mastering these technologies.