In PowerShell, a boolean is a data type that represents either `True` or `False`, often used in conditional statements and logical operations.
Here's a simple example:
$trueValue = $true
$falseValue = $false
if ($trueValue -eq $true) {
Write-Host 'This statement is true!'
} else {
Write-Host 'This statement is false!'
}
Understanding Boolean in PowerShell
What is a Boolean?
A Boolean is a data type that can hold one of two values: true or false. This binary nature of Booleans makes them essential in programming and logical operations, as they facilitate decision-making processes. In PowerShell, Booleans allow you to control the flow of your code based on conditional expressions.
The Role of Boolean in PowerShell
In PowerShell, Booleans are used extensively in control structures like if statements, loops, and switch statements. They help determine the execution path of your scripts based on certain conditions, making them a fundamental concept to grasp in order to write effective and efficient code.
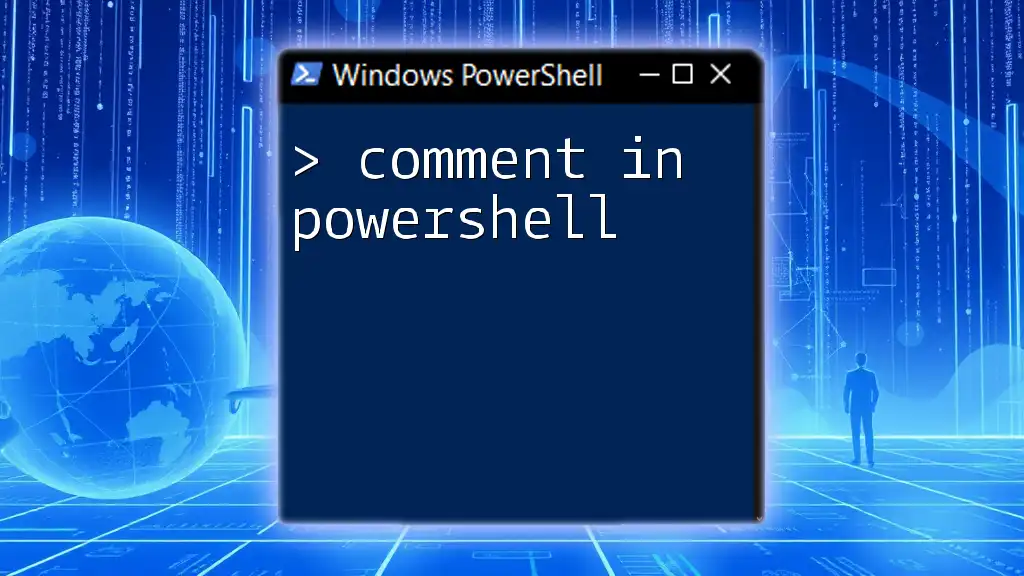
Working with PowerShell Booleans
Creating Boolean Variables
Creating a Boolean variable in PowerShell is straightforward. These variables are typically defined using the `$true` and `$false` keywords.
For example, to create a Boolean variable that represents availability, you can write:
$isAvailable = $true
This creates a variable called `$isAvailable` that holds the value `true`. You can use these Boolean variables to evaluate conditions later in your script.
Evaluating Boolean Expressions
PowerShell also allows you to create Boolean expressions using comparison operators such as `-eq`, `-ne`, `-gt`, and `-lt`. These operators compare values and return Boolean results.
Consider the following example:
$a = 5
$b = 10
$result = $a -lt $b # Returns $true
In this case, `$result` evaluates to `$true` because 5 is indeed less than 10. Understanding these expressions is crucial for using conditional logic effectively in your scripts.
Common Boolean Operations
Logical Operators
PowerShell supports three primary logical operators: `-and`, `-or`, and `-not`. These operators allow you to combine multiple Boolean expressions and are key to building more complex conditions.
Here are some examples:
$condition1 = $true -and $false # Returns $false
$condition2 = $true -or $false # Returns $true
Using Parentheses for Clarity
When constructing complex logical expressions, it's important to use parentheses to clarify precedence. Without them, operations may not yield the expected results.
For instance:
$result = ($a -lt $b) -and ($b -eq 10) # Returns $true if both conditions are met
Using parentheses enhances readability and helps maintain logical clarity.
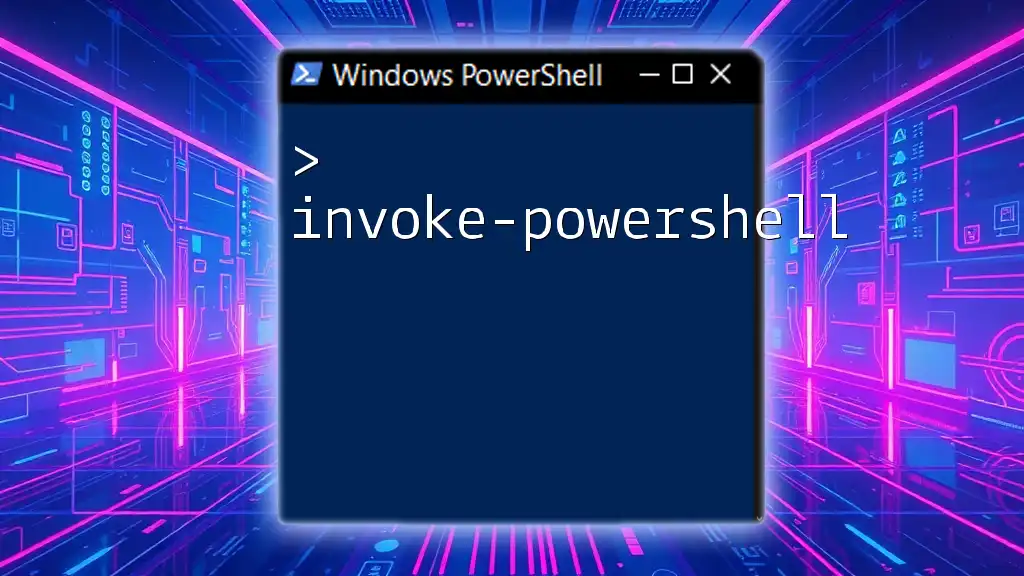
Practical Applications of PowerShell Booleans
Conditional Statements
Using Booleans in If Statements
Conditional statements are a primary means of controlling the flow of execution. Utilizing Booleans within if statements allows you to execute code based on specific conditions.
Here’s an example:
if ($isAvailable) {
Write-Host "Available"
} else {
Write-Host "Not Available"
}
In this case, the script will output "Available" if `$isAvailable` is `true`, and "Not Available" otherwise.
Switch Statements
Switch statements can also leverage Boolean variables. They provide a convenient way to execute different blocks of code based on the values of your conditions.
Loops and Boolean Expressions
While Loop
A typical usage of Booleans in loops is controlling the number of iterations. In a while loop, the condition evaluated at the beginning controls whether the loop continues to run.
Consider the following example:
$count = 0
while ($count -lt 5) {
Write-Host "Count is $count"
$count++
}
This loop will continue to execute as long as `$count` is less than 5. Each iteration displays the current count and increments it.
ForEach Loop
Booleans can also be used to filter iterations in a foreach loop. For instance, if you wanted to execute code based on a condition, you could do something like the following:
$numbers = 1..10
foreach ($num in $numbers) {
if ($num -gt 5) {
Write-Host "$num is greater than 5"
}
}
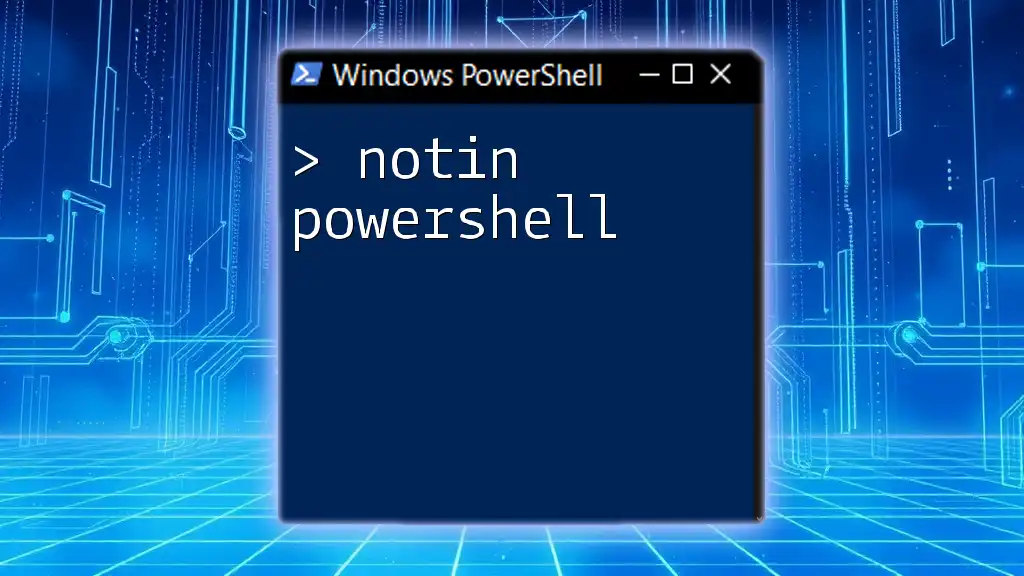
Advanced Boolean Concepts
Boolean Arrays
In PowerShell, you can also create Boolean arrays that store multiple Boolean values. This can be useful for handling a collection of conditions.
Here’s how you might define a Boolean array:
$boolArray = @($true, $false, $true)
You can then iterate through this array to evaluate or utilize conditions based on its values.
Boolean Values in Functions
PowerShell functions can return and accept Boolean values as parameters. This is an excellent way to create reusable logic that checks conditions.
Here’s a simple function that evaluates if a number is greater than 10:
function Test-Condition {
param (
[int]$value
)
return $value -gt 10
}
When you call this function with an integer, it will return `$true` if the value exceeds 10, and `$false` otherwise.
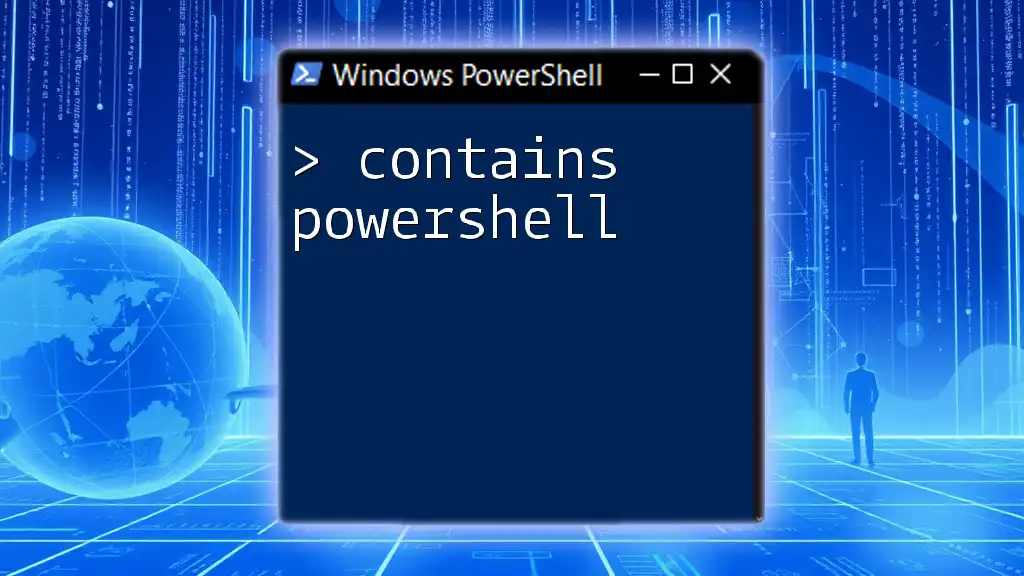
Best Practices When Using Booleans in PowerShell
Clarity and Readability
When working with Booleans, ensuring clarity in your code is paramount. Use descriptive variable names and clear logical expressions to enhance readability. This will make it easier for you and others to understand your code at a glance.
Performance Considerations
While Booleans are generally efficient, it’s important to consider their impact within larger scripts. Complex Boolean logic might slow down execution if overused in loops or extensive condition checks. Optimize conditions to improve performance and efficiency.
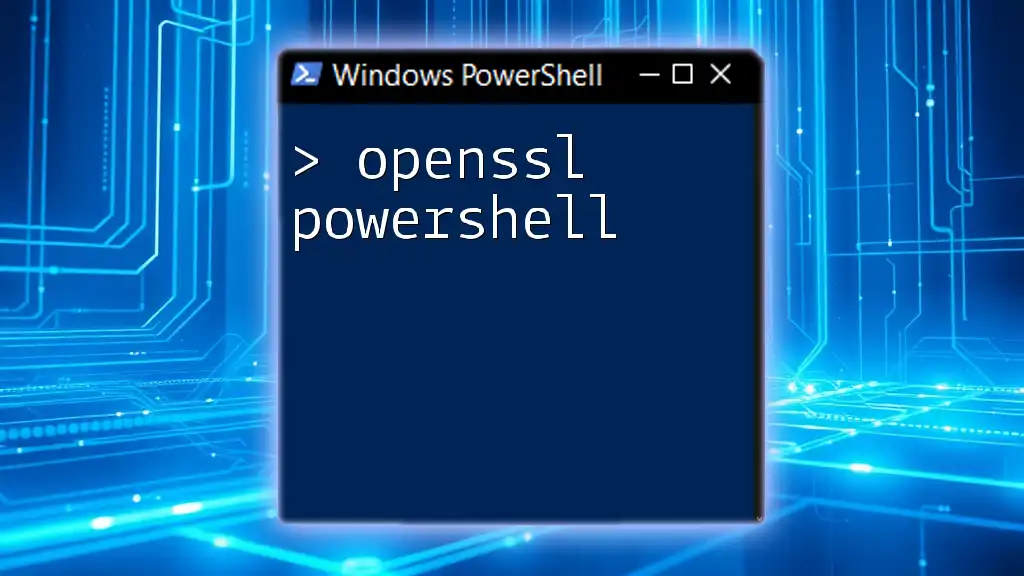
Conclusion
In summary, Booleans are a foundational aspect of programming in PowerShell. They enable logical decision-making and control the flow of scripts through conditional statements, loops, and functions. Understanding how to use Booleans effectively will undoubtedly enhance your PowerShell scripting capabilities. As you work with them, don’t hesitate to experiment and explore their complexities to become a more proficient PowerShell user.
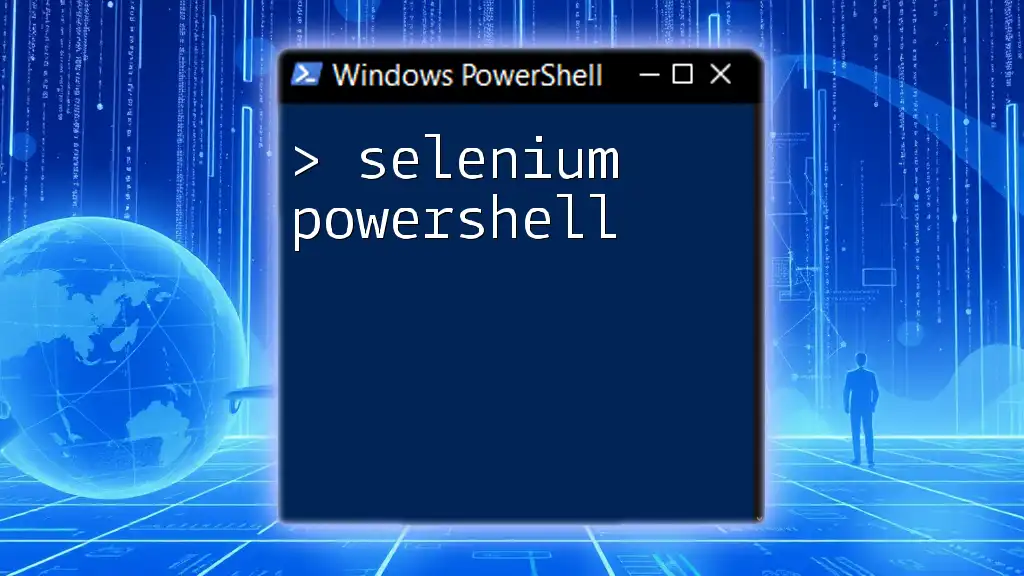
Additional Resources
Recommended Reading and Tools
For further learning, consider checking out the official Microsoft documentation on PowerShell and participating in community forums for tips and tricks.
FAQs about Booleans in PowerShell
If you have questions regarding your experiences with PowerShell Booleans, researching FAQs and engaging with the community can provide invaluable insights.