In PowerShell, the "like" operator is used for pattern matching with wildcard characters, allowing you to filter strings and variables efficiently.
Get-ChildItem | Where-Object { $_.Name -like '*.txt' }
What is the `-like` Operator?
The `-like` operator in PowerShell is a powerful tool for performing string comparison, allowing you to determine if a given string matches a specified pattern. At its core, it offers a straightforward way to compare strings using wildcards, making it particularly useful in scenarios where you need to filter or validate data.
Definition of the `-like` Operator
The `-like` operator checks if a string matches a specified pattern. Unlike the equality operator `-eq`, which checks for an exact match, `-like` allows for flexibility with pattern matching through wildcards.
When you write a condition using `-like`, the syntax follows this format:
string -like pattern
This operator returns `True` if the string matches the pattern and `False` otherwise.
Syntax of the `-like` Operator
To utilize the `-like` operator effectively, it’s essential to understand its syntax:
"example" -like "ex*"
In this example, "example" matches the pattern "ex*", meaning any string that starts with "ex" followed by zero or more characters is acceptable. The components consist of the string being evaluated, the `-like` operator, and the pattern to match.

Pattern Matching with Wildcards
Introduction to Wildcards in PowerShell
Wildcards are special characters that help in pattern matching. In PowerShell, the primary wildcards are `*` and `?`.
- `*`: Represents zero or more characters. It can match any sequence of characters.
- `?`: Represents a single character. It matches exactly one character in the string.
Examples of Using Wildcards in `-like` Operator
To understand how wildcards operate, consider the following examples:
-
A simple match utilizing the asterisk:
"Hello World" -like "Hello*" # Returns True
This code confirms that the string starts with "Hello" and can be followed by any characters.
-
Using the question mark wildcard:
"Hello" -like "He?lo" # Returns True
Here, the string "Hello" matches "He?lo" because the `?` wildcard can represent one character.

Practical Scenarios for Using `-like`
Filtering Strings in Arrays
The `-like` operator excels in filtering data, especially strings within arrays. For example, when you want to filter items based on a specific pattern:
$arr = @("apple", "banana", "apricot", "blueberry")
$arr | Where-Object {$_ -like "a*"} # Returns apple, apricot
In this case, the command filters through the array, returning only items that start with "a".
Matching File Names
Another common use case for the `-like` operator is filtering file names within a directory. For instance, if you want to list all text files:
Get-ChildItem | Where-Object { $_.Name -like "*.txt" } # Gets all .txt files
This command retrieves all files in the current directory that have a `.txt` extension, demonstrating the operator's utility in file operations.
User Input Validation
The `-like` operator is also beneficial for validating user input against specific patterns. For example, if you want to prompt a user for their name and verify if it starts with 'A':
$input = Read-Host "Enter your name"
if ($input -like "A*") {
"Your name starts with A!"
}
This snippet checks if the entered name begins with an "A" and responds accordingly.

PowerShell `-like` vs `-match`
Differences Between `-like` and `-match`
While both `-like` and `-match` perform pattern comparisons, they differ significantly. The `-like` operator targets simple patterns with wildcards, suitable for straightforward string evaluations.
Conversely, the `-match` operator employs regular expressions, providing more advanced and flexible matching capabilities. Depending on your needs, you'll choose one over the other.
Examples of `-match`
Here’s a clear example of how `-match` differs:
"Hello World" -match "Hello" # Returns True
This demonstrates a regex-based match that confirms the string contains "Hello".
When to Use Each Operator
Determining when to use `-like` or `-match` hinges on your requirements:
- Use `-like` when dealing with simple patterns and you want rapid results without the complexity of regex.
- Use `-match` when you need the power of regex for complex patterns, such as validating email formats or other detailed string requirements.
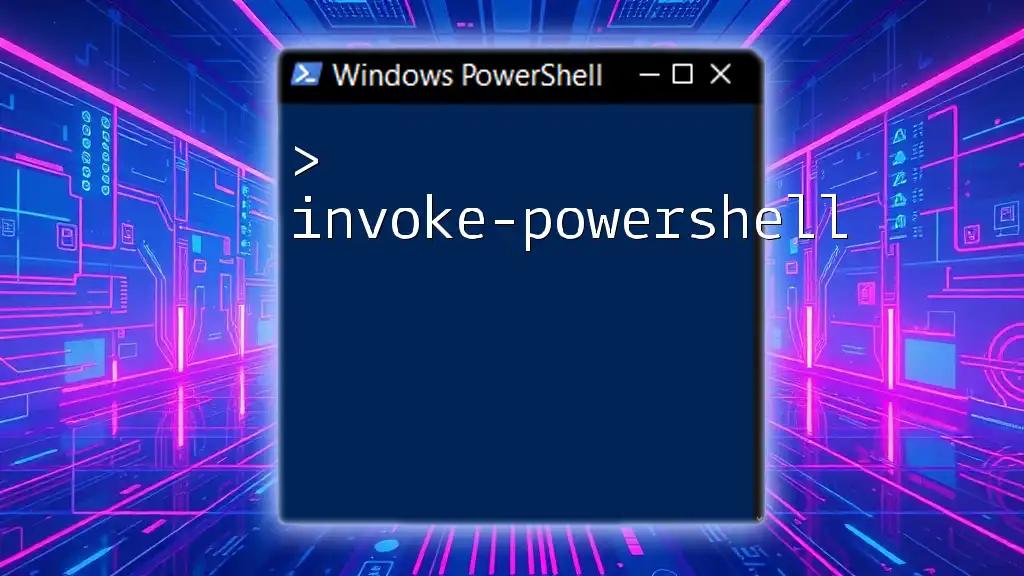
Common Pitfalls to Avoid with `-like`
Case Sensitivity Issues
A common misunderstanding involves the case sensitivity of the `-like` operator. By default, `-like` is case-insensitive. However, if there's any confusion, simply remember:
"Hello" -like "hello" # Returns False
This example illustrates that in cases where exact casing is implicative in the match, it can return erroneous results.
Misunderstanding Wildcards
It’s also crucial to grasp how wildcards work within patterns. For example, using them incorrectly can lead to surprising outputs. If you combine both wildcards improperly, you may not achieve the match you expect.

Conclusion
In conclusion, understanding how to utilize the `-like` operator in PowerShell can significantly enhance your scripting efficiency. Its pattern matching capabilities are vital for filtering data, validating input, and much more.
As you become more familiar with `like in PowerShell`, experimenting with various patterns and scenarios will only deepen your comprehension and effectiveness. Feel free to explore related operators, such as `-match`, to expand your PowerShell skills further.
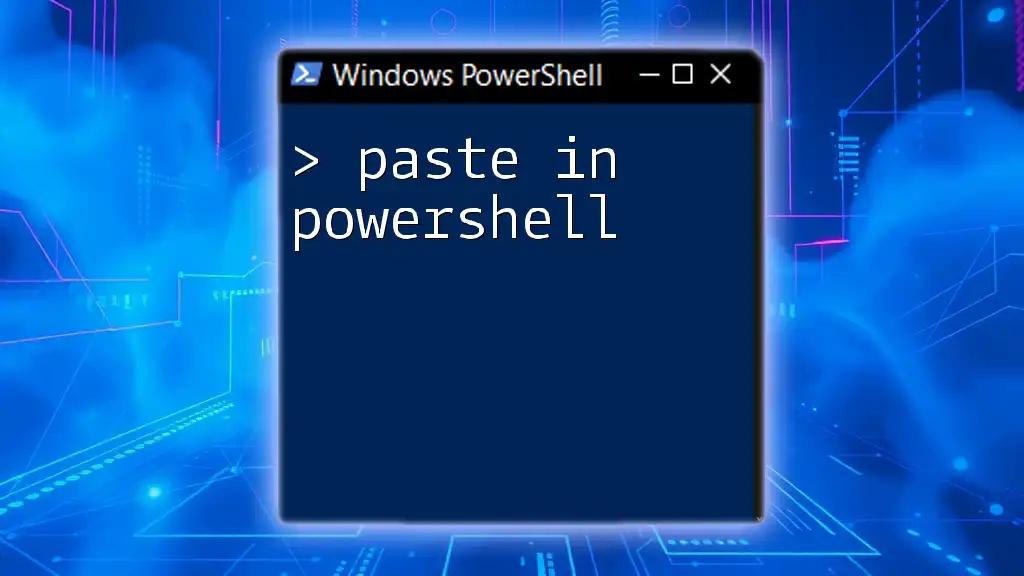
Additional Resources
For comprehensive information, always refer to the [official PowerShell documentation](https://docs.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.2) and consider checking out online forums and communities dedicated to PowerShell scripting.

Call to Action
Are you eager to enhance your PowerShell skills? Join our course designed to teach you concise and effective techniques for mastering PowerShell commands. We also encourage you to reach out with any questions or feedback to further enrich our learning community.