The `if -like` statement in PowerShell is used to test if a particular string matches a specified pattern using wildcard characters.
Here's a code snippet to illustrate its usage:
if ("Hello, World!" -like "Hello*") {
Write-Host 'The string matches the pattern!'
}
The Basics of Conditional Statements in PowerShell
What Are Conditional Statements?
Conditional statements allow scripts to make decisions based on certain conditions. Essentially, they enable the automation of processes by evaluating whether a specified condition is true or false. The most common types of conditional statements in PowerShell are `if`, `else`, and `elseif`. Understanding how to use these statements effectively is critical for anyone looking to harness the full power of PowerShell scripting.
Understanding the `-like` Operator
The `-like` operator is a powerful tool used in PowerShell for pattern matching. Unlike the `-eq` (equal) or `-ne` (not equal) operators that require exact matches, the `-like` operator offers more flexibility by allowing the use of wildcards. This makes it particularly useful when you want to check if a string fits a certain pattern. The `-like` operator is ideal for scenarios where you want to match text with specific prefixes, suffixes, or patterns, providing a versatile way of evaluating conditions in scripts.
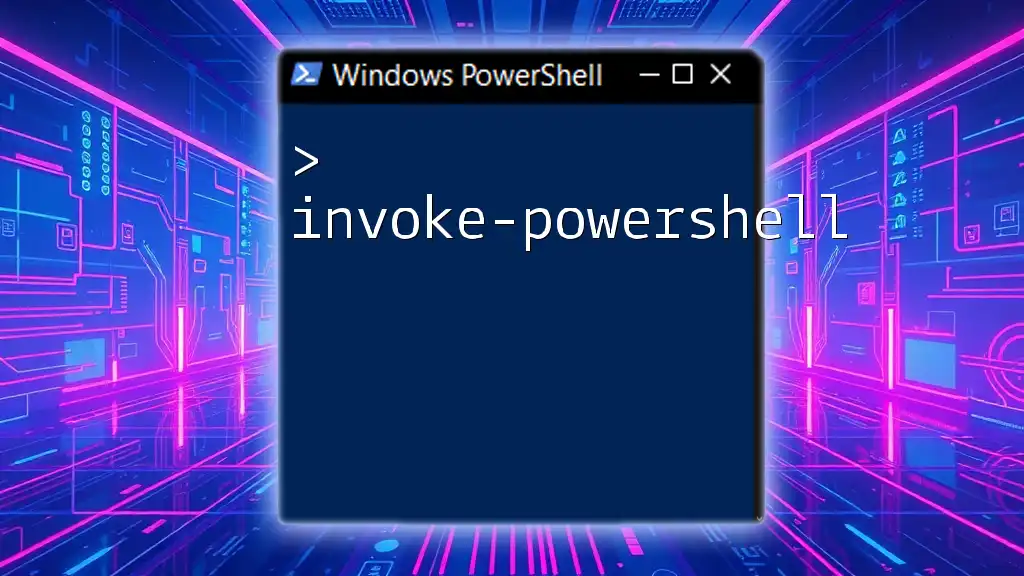
Syntax of the `if -like` Statement
Basic Structure
The general syntax for using the `if -like` statement is straightforward. It follows this structure:
if (<condition> -like <pattern>) {
<code to execute>
}
This structure consists of the `if` keyword, followed by a condition being tested against a pattern using the `-like` operator. If the condition matches the pattern, the code within the curly braces will execute.
Wildcards in Patterns
Wildcards are an integral part of the `-like` operator in PowerShell. The two primary wildcards you can use are:
- `*` – Represents any number of characters.
- `?` – Represents a single character.
For instance, if you want to check whether a name starts with “John,” you can use:
if ($name -like "John*") {
Write-Host "Name starts with John"
}
In this example, `*` allows for any string that follows “John,” making it incredibly flexible for capturing various name formats.
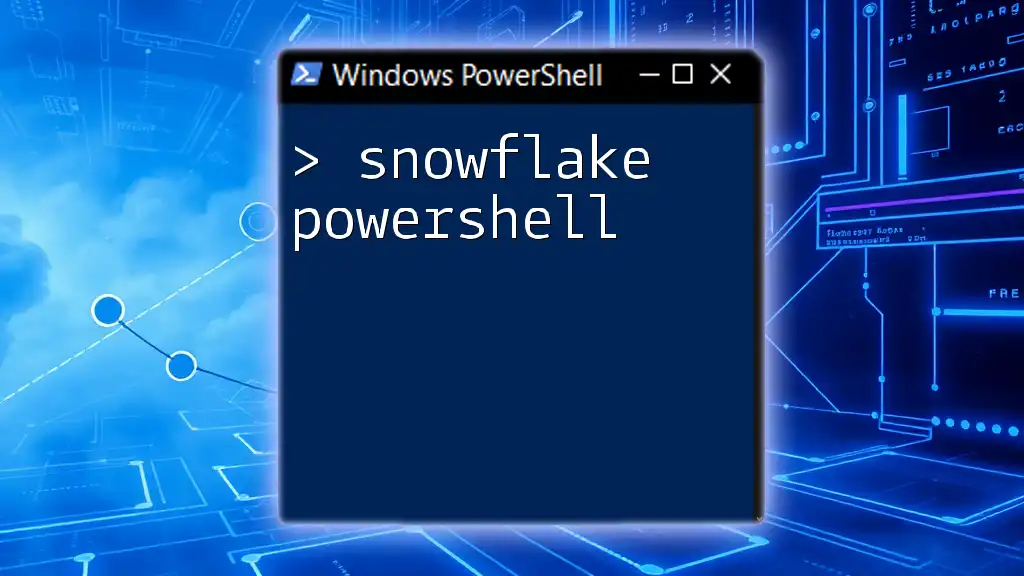
Practical Examples of `if -like`
Example 1: Checking File Extensions
One common use case for the `if -like` statement is in file management. Below is an example where we check if files in a directory have a specific extension.
$files = Get-ChildItem
foreach ($file in $files) {
if ($file.Extension -like "*.txt") {
Write-Host "$file is a text file."
}
}
In the snippet above, the script retrieves all files in the current directory and checks if their extension matches `.txt`. If it does, it confirms that the file is a text file.
Example 2: Validating User Input
Another application of the `if -like` statement is in validating user input. Consider the following example:
$input = Read-Host "Enter your name"
if ($input -like "A*") {
Write-Host "Hello, $input! Your name starts with A."
}
Here, the script prompts the user to enter their name and checks if it starts with the letter “A.” If matched, it provides a personalized greeting.
Example 3: Filtering Arrays
You can also use the `if -like` statement to filter elements in an array. The following example demonstrates this concept:
$fruits = @("Apple", "Banana", "Cherry", "Apricot")
foreach ($fruit in $fruits) {
if ($fruit -like "A*") {
Write-Host "$fruit starts with A."
}
}
This script goes through each fruit in the array and checks whether it starts with "A". The matches will be printed out, illustrating how flexible the `-like` operator can be when handling collections.
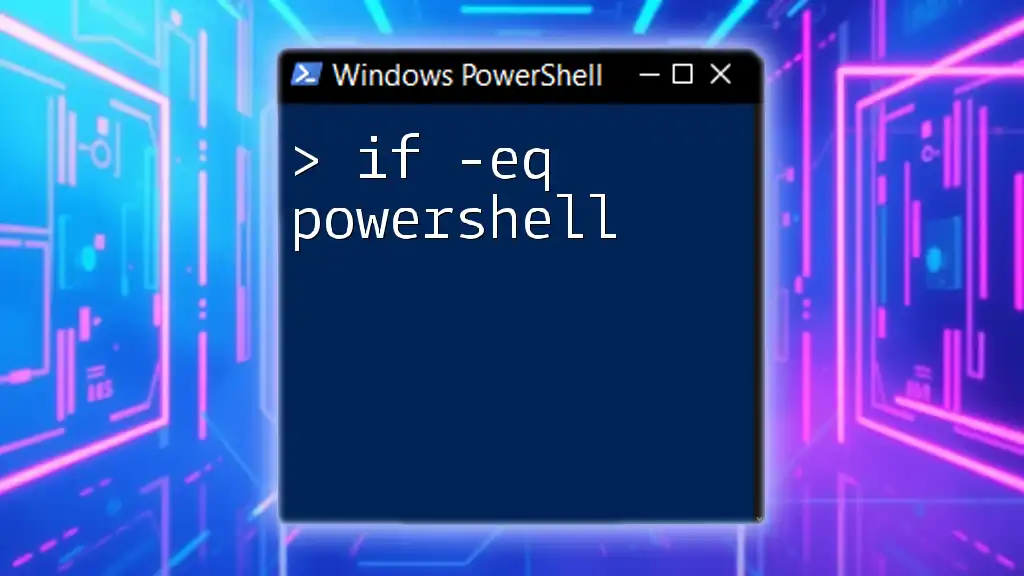
Combining `if -like` with Other Conditional Statements
Using `else` and `elseif`
You can also enhance your logic by combining `if -like` with `else` and `elseif` statements. This allows for comprehensive script behavior based on multiple conditions.
if ($input -like "A*") {
Write-Host "Hello, $input! Your name starts with A."
} elseif ($input -like "B*") {
Write-Host "Hello, $input! Your name starts with B."
} else {
Write-Host "Hello, $input!"
}
In this example, the script provides greetings based on the first letter of the user’s name. It first checks if it starts with "A," then checks for "B," and defaults to a generic greeting otherwise.
Nesting `if` Statements
Nesting `if` statements can add another layer of decision-making in your scripts. It works particularly well when you have specific conditions that require further checks. Consider the following:
if ($name -like "J*") {
if ($name -like "John*") {
Write-Host "It's John!"
} else {
Write-Host "It's someone else with a J!"
}
}
In this script, if the name starts with "J," it further checks whether it’s "John." Depending on the outcome, it provides distinct outputs, showcasing how you can create complex logic flows.
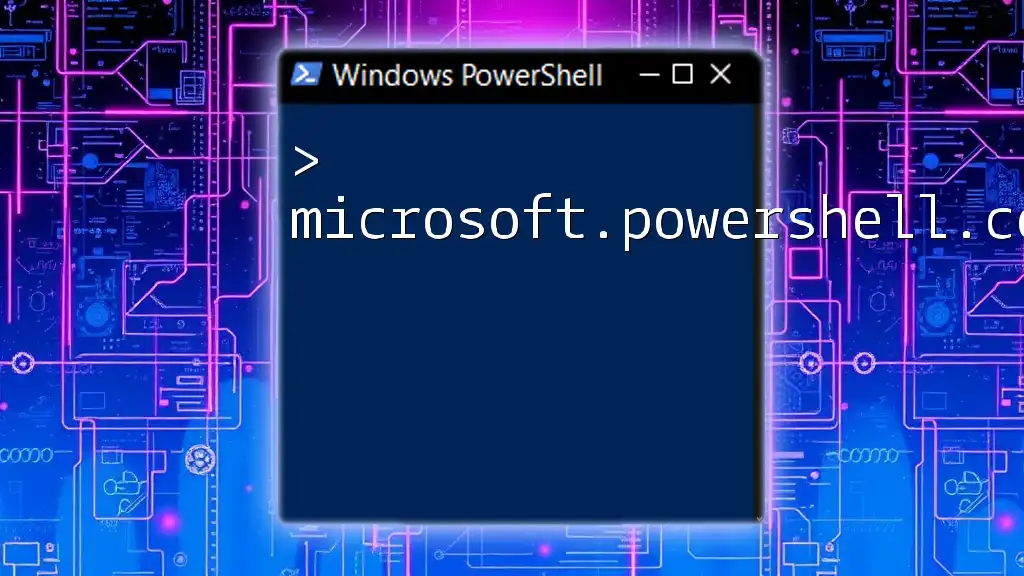
Best Practices for Using `if -like`
Clear and Concise Code
Writing clear and concise code is critical in scripting. Always strive for readability by using meaningful variable names and maintaining a consistent format. The use of comments can greatly enhance understanding, explaining the purpose of complex conditional structures. A well-structured script is easier to maintain and troubleshoot.
Avoiding Common Mistakes
There are some common pitfalls to avoid when using the `-like` operator. One common mistake is failing to use wildcards correctly. For example:
if ($name -like "J") { # This won’t match names like "John"
Write-Host "It might be a match."
}
In this scenario, the condition will fail to capture most cases. Ensure that your patterns are accurate and appropriately use wildcards for effective matching.
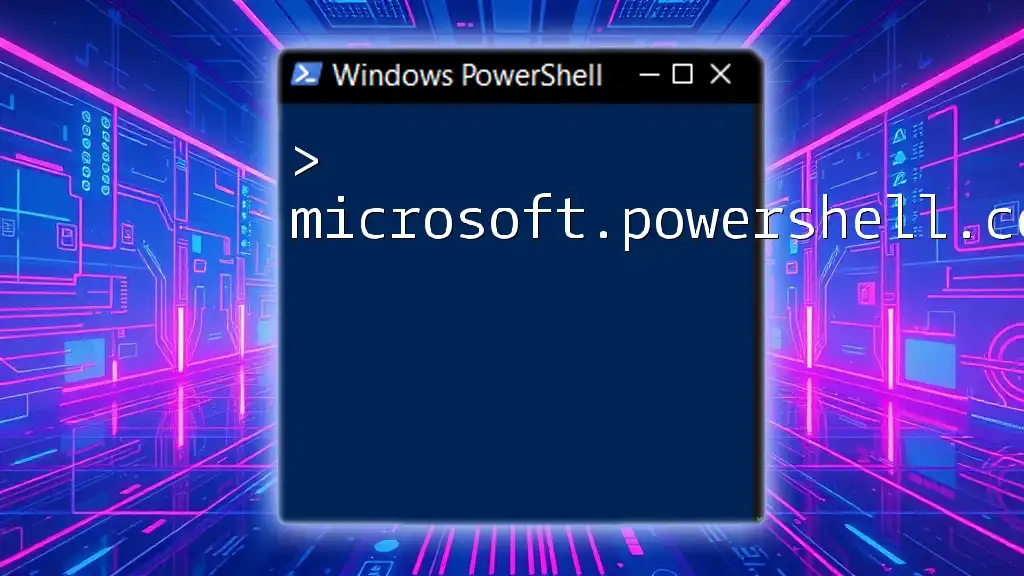
Conclusion
The `if -like` statement in PowerShell is a valuable tool for pattern matching and conditional evaluations. By mastering this operator and understanding how to implement it effectively in scripts, you open up a world of possibilities for automating tasks and decision-making processes. Practice using the `if -like` statement in various scenarios to reinforce your understanding and develop your PowerShell skills further. Utilizing these techniques will make your scripting more efficient and productive.