Filtering in PowerShell allows users to selectively retrieve and display specific data from a collection of objects based on defined criteria.
Here’s a code snippet that demonstrates how to filter a list of processes to show only those with a CPU usage greater than 100:
Get-Process | Where-Object { $_.CPU -gt 100 }
Understanding PowerShell Filters
What are Filters in PowerShell?
Filters in PowerShell are essential tools that allow users to manage and manipulate data by retrieving only the objects or items that meet specific criteria. By applying filters, you can streamline your data retrieval process, making it more efficient and effective. For instance, instead of pouring through a long list of objects, you can apply filters to extract only those that are relevant to your current task.
The Role of Pipeline in Filtering
The PowerShell pipeline is a core component of filtering methods. In PowerShell, commands can pass the output of one command as input to another, creating a seamless flow of data. This pipeline allows you to filter data dynamically, making it easy to perform complex queries without the need for intermediate steps.

Common PowerShell Filters
Using the `Where-Object` Cmdlet
Introduction to `Where-Object`
The `Where-Object` cmdlet is one of the most powerful and commonly used filtering methods in PowerShell. It evaluates each object in the pipeline against a specified condition, allowing you to filter the results based on properties of the objects.
Syntax Breakdown
The basic syntax for `Where-Object` appears as follows:
Where-Object { <condition> }
Example Usage
Consider a scenario where you want to see all processes that are consuming more than 100 CPU seconds. You can achieve this using the following command:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this code snippet:
- `Get-Process` retrieves a list of all currently running processes.
- The pipeline (`|`) sends this list to `Where-Object`.
- The condition `{ $_.CPU -gt 100 }` filters the list to include only those processes that use more than 100 CPU seconds.
Filtering with Comparison Operators
Common Comparison Operators
PowerShell provides several comparison operators that enable fine-tuned filtering of your data. These include:
- `-eq` (equal)
- `-ne` (not equal)
- `-gt` (greater than)
- `-lt` (less than)
- `-ge` (greater than or equal to)
- `-le` (less than or equal to)
Code Snippets
Here’s how you might use these operators in filtering:
Get-Service | Where-Object { $_.Status -eq 'Running' }
This command lists all services that are currently running. The filter checks the `Status` property of each service object against the string 'Running', returning only those services that match.
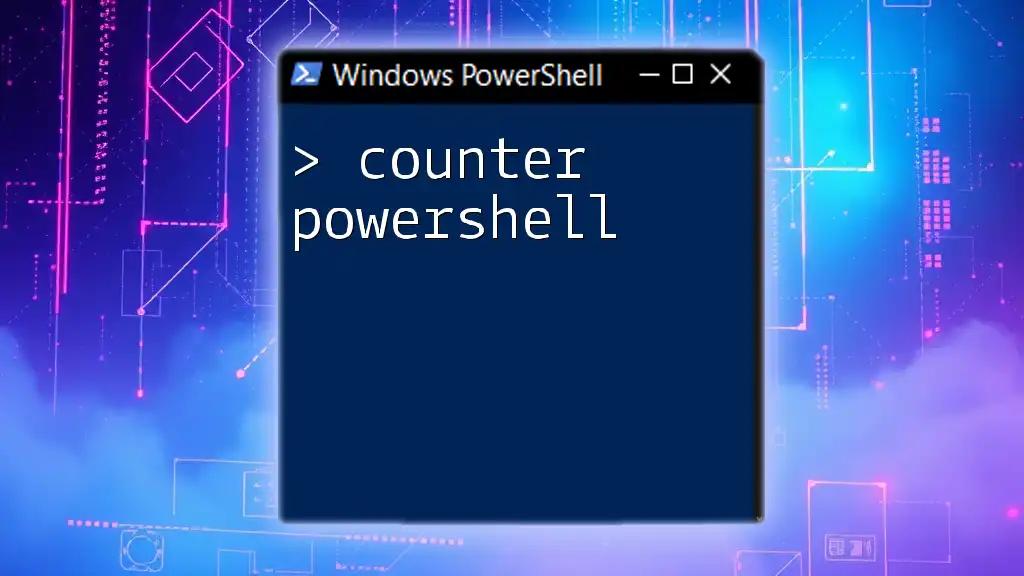
Advanced Filtering Techniques
Using `Select-Object` for Property Filtering
Select-Object works in conjunction with filtering to streamline the output. It allows you to specify which properties to display from the filtered results, enhancing readability and focus.
For example, if you want to see only the process names and their CPU usage for processes exceeding a specific CPU threshold, you can use:
Get-Process | Where-Object { $_.CPU -gt 100 } | Select-Object Name, CPU
This command not only filters processes based on CPU usage but also restricts the output to just the necessary properties, `Name` and `CPU`.
Combining Filters
Combining multiple filters can provide even more powerful results. Logical operators such as `-and` and `-or` allow you to layer your conditions effectively. For example:
Get-Process | Where-Object { $_.CPU -gt 100 -and $_.Memory -lt 500MB }
In this snippet, processes are filtered based on two conditions: using more than 100 CPU seconds and less than 500 MB of memory.

Filtering Lists in PowerShell
Using Arrays and Collections
PowerShell enables filtering on arrays, which can be particularly useful when managing lists of items. For example, if you wanted to filter a range of numbers for only the even integers, you could do so like this:
$numbers = 1..100
$filteredNumbers = $numbers | Where-Object { $_ % 2 -eq 0 }
This command defines an array of numbers from 1 to 100, and then filters it, using the modulus operator to return only even numbers.
Using Filtering with Hash Tables
PowerShell also allows for filtering within hash tables, which are collections of key-value pairs. For example:
$users = @(
@{Name="John"; Age=30},
@{Name="Doe"; Age=25}
)
$filteredUsers = $users | Where-Object { $_.Age -lt 30 }
In this case, the command filters the users based on their age, returning only those that are younger than 30.
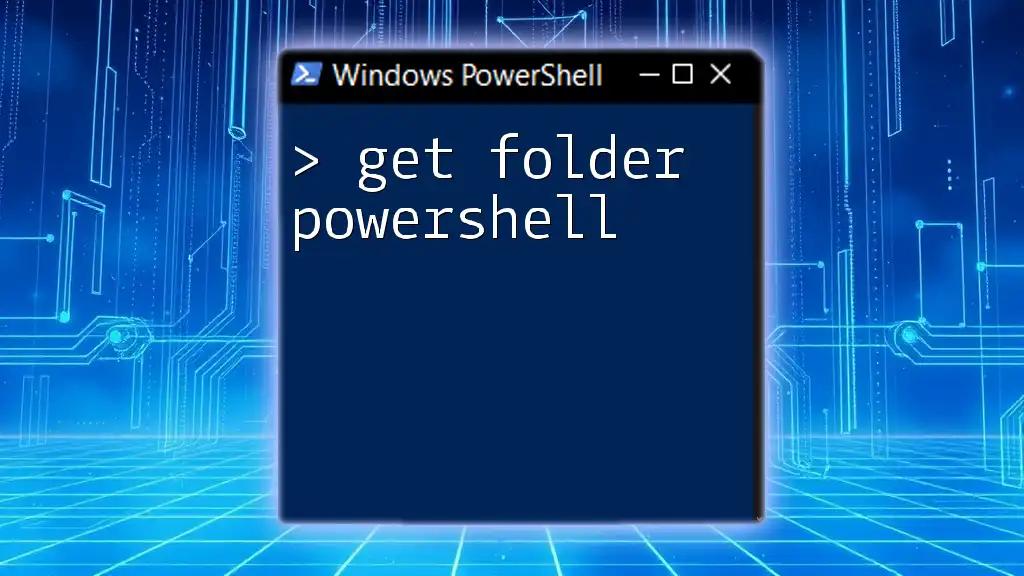
Practical Applications of Filtering in PowerShell
Use Cases in System Administration
Filtering plays a vital role in system administration tasks. Here are a few scenarios where filter Powershell can be beneficial:
-
Filtering Event Logs: Admins can filter system or application event logs to identify specific entries related to errors or warnings.
-
Filtering Installed Software: Quickly see which software is installed on a machine based on version or publisher.
Example Scenarios
A practical example of filtering event logs might look like this:
Get-EventLog -LogName System | Where-Object { $_.EventID -eq 6006 }
This retrieves entries from the System log where the Event ID is 6006, which commonly signifies a system shutdown event.
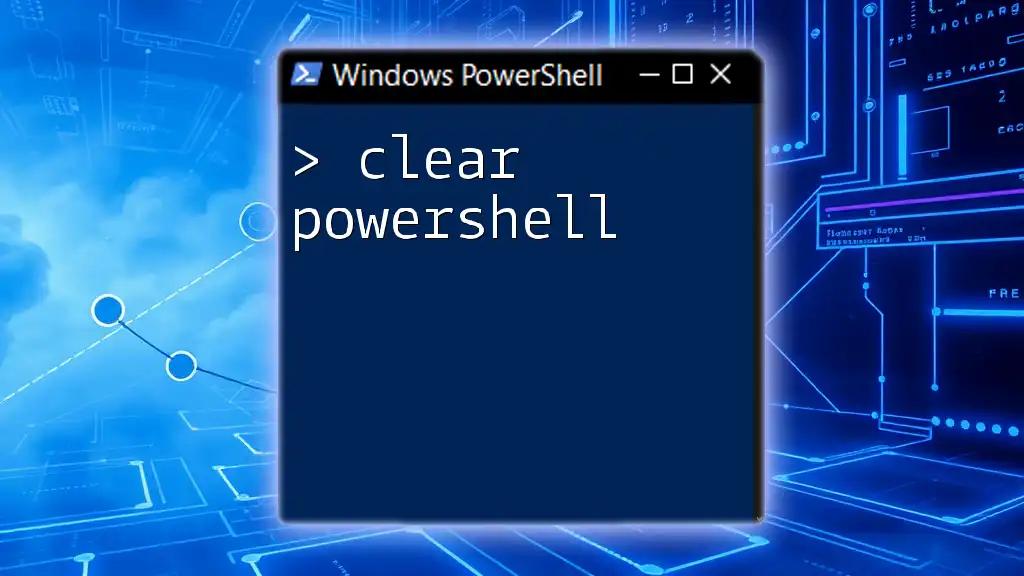
Conclusion
Mastering filtering in PowerShell is a crucial skill that greatly enhances your data management capabilities. By effectively using commands like `Where-Object`, adopting various comparison operators, and combining filters, you can retrieve precisely the information you seek with minimal effort. Practice with these techniques to boost your efficiency and streamline your PowerShell workflows.
As you dive deeper into PowerShell, this skill will prove invaluable, offering you the tools to tackle complex tasks with ease. Explore further and refine your skills to become proficient at leveraging filters in PowerShell!
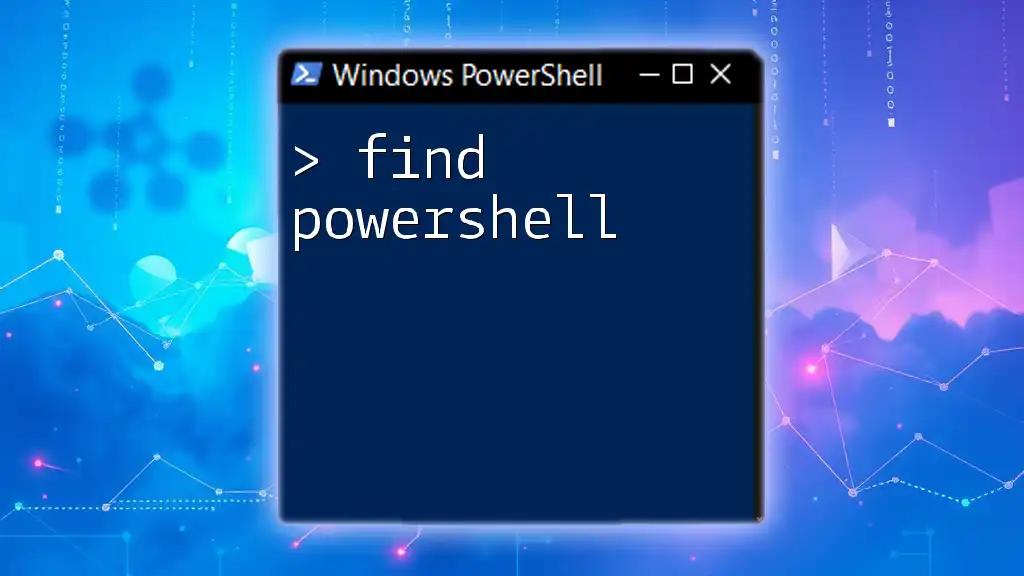
Additional Resources
For those interested in further learning, refer to the official PowerShell documentation or explore online tutorials to deepen your understanding of filtering techniques and their applications in various administrative tasks.