In PowerShell, the `-eq` operator is used to check if two values are equal, returning `$true` if they are and `$false` otherwise.
Here’s a code snippet demonstrating its usage:
$number1 = 5
$number2 = 5
if ($number1 -eq $number2) {
Write-Host 'The numbers are equal!'
} else {
Write-Host 'The numbers are not equal.'
}
Understanding Conditional Statements in PowerShell
What is a Conditional Statement?
A conditional statement is a fundamental programming concept that allows a script to execute specific blocks of code based on whether certain conditions are true or false. In scripting languages, including PowerShell, conditional statements play a vital role in controlling the flow of execution, enabling automation and decision-making in scripts. Understanding how these statements work is essential for efficient scripting.
Introduction to Comparison Operators
Comparison operators are symbols that are used to compare two values. In PowerShell, these operators return a Boolean value of either true or false, which can then be used with conditional statements such as `if`. Among the available comparison operators, you’ll find `-eq`, `-ne`, `-lt`, `-gt`, and more, with `-eq` being specifically used to evaluate equality. Mastering these operators is crucial for effective decision-making in your scripts.
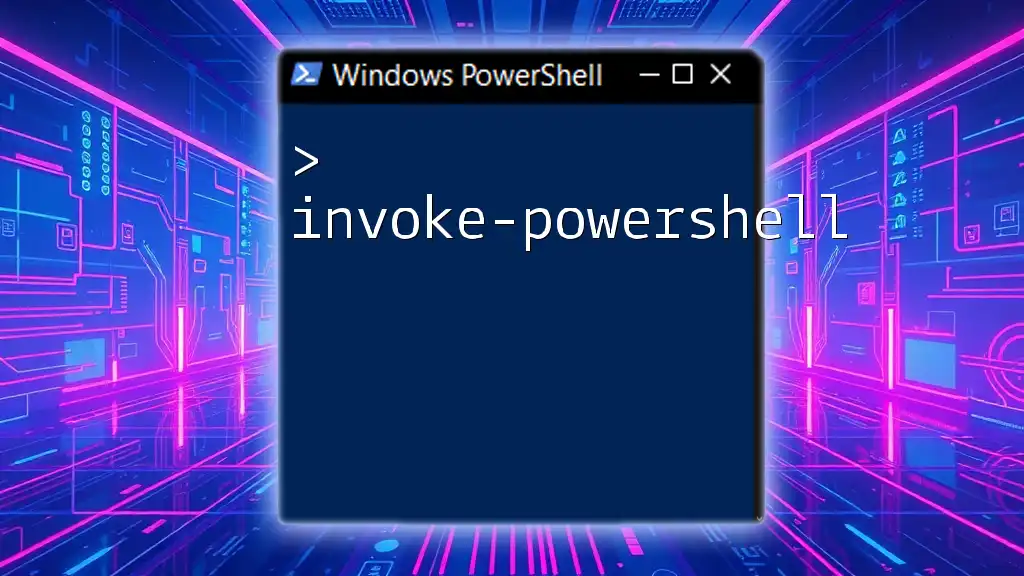
The -eq Operator in PowerShell
What does -eq Mean?
The `-eq` operator stands for "equals" and is primarily used to compare two values for equality in PowerShell. When you use the `-eq` operator, PowerShell checks if the values on its left and right sides are the same. If they are equal, `-eq` returns true; if they are not equal, it returns false. Understanding how to leverage this operator is critical for building logical conditions in scripts.
Syntax Overview
The basic syntax for using the `if` statement in PowerShell looks like this:
if (condition) {
# code to execute if condition is true
}
Here, you replace `condition` with your `-eq` expression.
How to Use -eq in an if Statement
Basic Structure
Using the `-eq` operator within an `if` statement is straightforward. It allows you to execute a block of code based on whether two values are equal.
For example:
if ($a -eq $b) {
"They are equal"
}
In this simple code snippet, if the variable `$a` is equal to the variable `$b`, PowerShell executes the code within the curly braces.
Example: Comparing Strings
Comparing strings demonstrates the `-eq` operator in action. Let’s look at a practical example:
$a = "Hello"
$b = "Hello"
if ($a -eq $b) {
Write-Output "The strings are equal."
} else {
Write-Output "The strings are not equal."
}
In this case, since both strings are identical, the output will be “The strings are equal.” This showcases how `-eq` works effectively with strings.
Example: Comparing Numbers
You can also use the `-eq` operator to compare numeric values. Here is another straightforward example:
$x = 10
$y = 20
if ($x -eq $y) {
Write-Output "Numbers are equal."
} else {
Write-Output "Numbers are not equal."
}
In this scenario, the output will be “Numbers are not equal.” since 10 is not equal to 20. This illustrates the versatility of the `-eq` operator in handling numerical comparisons.
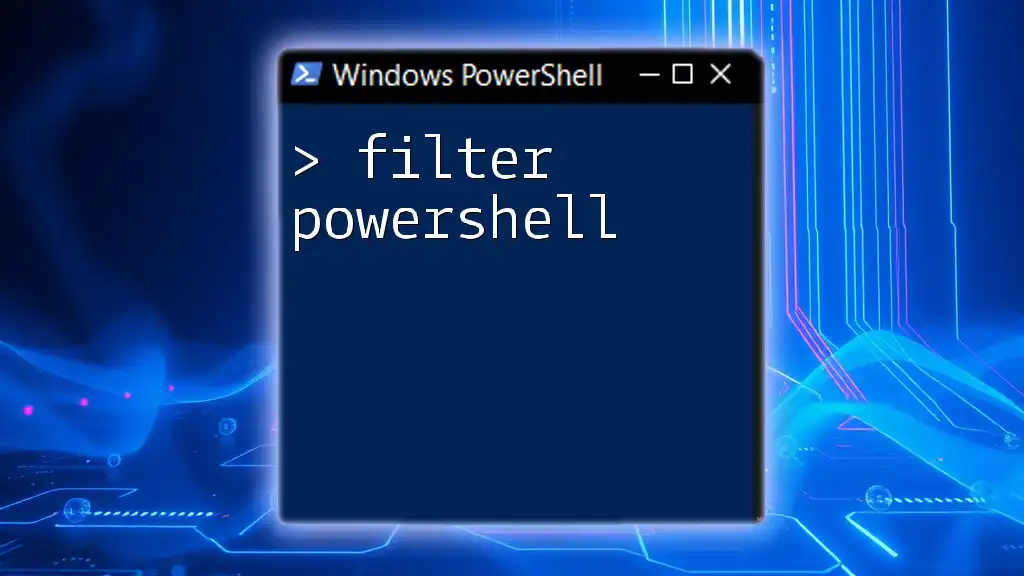
Using -eq with Other Comparison Operators
The Power of Combining Operators
Combining the `-eq` operator with other comparison operators enhances the decision-making capabilities within your scripts. You can create complex conditional expressions that reflect multiple conditions.
Example: Multiple Conditions
Consider the following code snippet that employs both `-eq` and `-lt` in conjunction:
$a = 5
$b = 5
$c = 10
if (($a -eq $b) -and ($b -lt $c)) {
Write-Output "First condition is true."
}
In this case, the `-and` operator is used to ensure that both conditions must be true. If they are, PowerShell will output “First condition is true.” This example underscores the importance of logic in crafting robust condition checks.
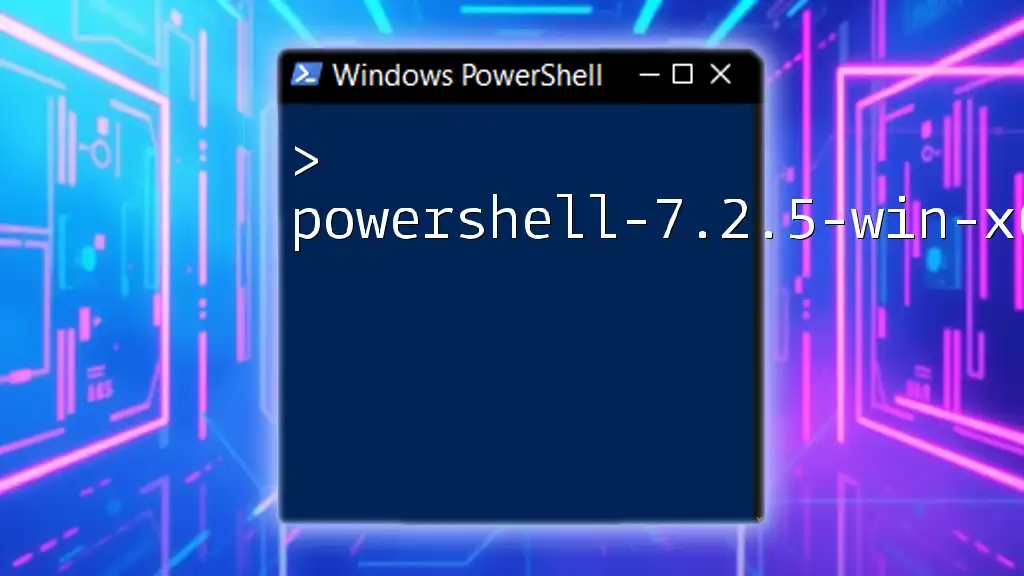
Common Mistakes When Using -eq
Case Sensitivity
One common mistake when using the `-eq` operator is overlooking case sensitivity. By default, PowerShell performs case-sensitive comparisons. This can lead to unexpected results when comparing strings.
You can use `-ieq` for a case-insensitive comparison. Here’s how that looks:
Example: Case Sensitivity Issue
$a = "test"
$b = "TEST"
if ($a -eq $b) {
Write-Output "Strings are equal."
} else {
Write-Output "Strings are not equal."
}
In this example, the output will be “Strings are not equal.” This is because the comparison is case-sensitive. To avoid such issues, consider using the `-ieq` operator when dealing with string comparisons that might differ in case.
Using -eq with Variables
Another frequent pitfall occurs when comparing uninitialized or undefined variables. If you attempt to use `-eq` without properly declaring your variables, PowerShell may throw an error or behave unexpectedly.
Example: Uninitialized Variables
if ($x -eq $y) {
Write-Output "Both variables are equal."
} else {
Write-Output "One or both variables are not initialized."
}
In this scenario, if `$x` or `$y` is not initialized, the output could be misleading. Always ensure variables are defined before using them in comparisons to maintain script integrity.
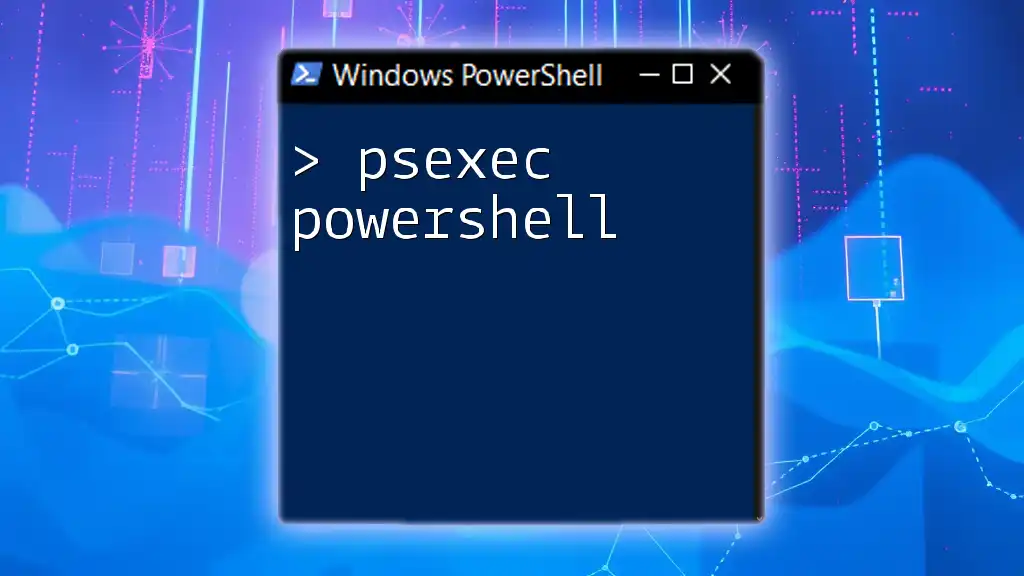
Practical Applications of the -eq Operator
Scripting Automation Tasks
The `-eq` operator can be extremely useful in various automation tasks, especially in administrative scripts where decision-making is critical. Automating repetitive tasks by checking conditions can save time and reduce errors.
Example: File Existence Check
For example, you might want to check the existence of a file before performing an operation on it:
$filePath = "C:\example.txt"
if (Test-Path $filePath -eq $true) {
Write-Output "File exists."
} else {
Write-Output "File does not exist."
}
By using `-eq` to check the result of `Test-Path`, you can ensure that your operations are only attempted on files that actually exist, helping avoid runtime errors.
Interactive User Prompts
You can also use the `-eq` operator to make your scripts interactive and user-friendly. This can enhance user experience by providing personalized responses based on user input.
Example: User Input Comparison
Here's how you might use user input with `-eq`:
$userInput = Read-Host "Enter your name"
if ($userInput -eq "Admin") {
Write-Output "Welcome Admin!"
} else {
Write-Output "Welcome User!"
}
In this example, the script welcomes different users based on their names. The ability to customize responses based on input is one of the many practical applications of the `-eq` operator.
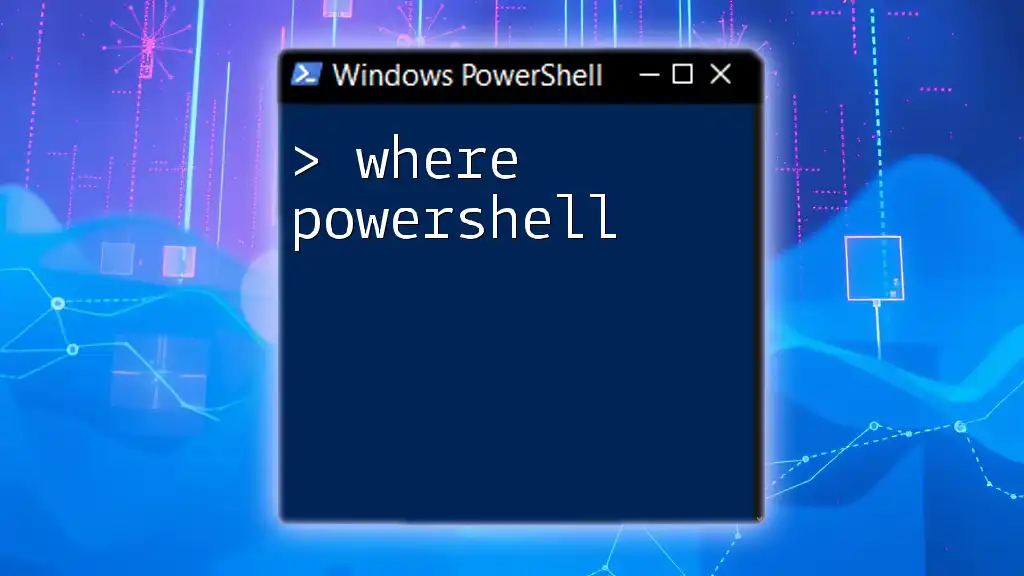
Conclusion
In summary, the `if -eq` syntax in PowerShell is a powerful tool for comparison and logical decision-making. It allows scripts to perform different actions based on variable equality, making it essential for effective scripting. By understanding the nuances of `-eq`, along with its cases and potential pitfalls, you can significantly enhance the robustness and effectiveness of your PowerShell scripts.
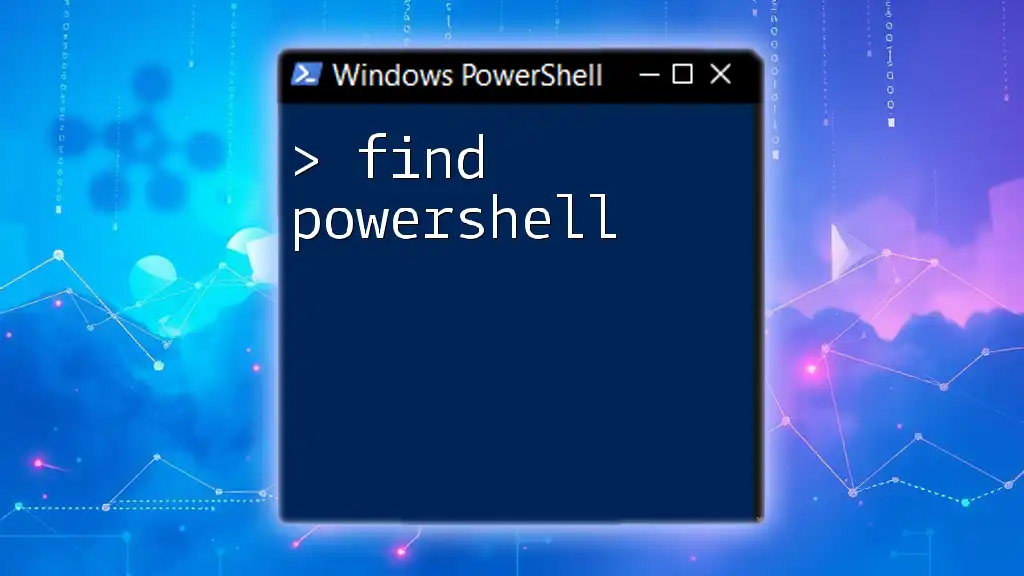
Additional Resources
To further enhance your knowledge and skills in PowerShell scripting, consider exploring additional resources such as official documentation, community forums, and tutorials that provide more advanced use cases and best practices. Happy scripting!